人脸识别引擎SeetaFaceEngine中Identification模块使用的测试代码
人脸识别引擎SeetaFaceEngine中Identification模块用于比较两幅人脸图像的相似度,以下是测试代码:
int test_recognize()
{const std::string path_images{ "E:/GitCode/Face_Test/testdata/recognization/" };seeta::FaceDetection detector("E:/GitCode/Face_Test/src/SeetaFaceEngine/FaceDetection/model/seeta_fd_frontal_v1.0.bin");seeta::FaceAlignment alignment("E:/GitCode/Face_Test/src/SeetaFaceEngine/FaceAlignment/model/seeta_fa_v1.1.bin");seeta::FaceIdentification face_recognizer("E:/GitCode/Face_Test/src/SeetaFaceEngine/FaceIdentification/model/seeta_fr_v1.0.bin");detector.SetMinFaceSize(20);detector.SetMaxFaceSize(200);detector.SetScoreThresh(2.f);detector.SetImagePyramidScaleFactor(0.8f);detector.SetWindowStep(4, 4);std::vector<std::vector<seeta::FacialLandmark>> landmards;// detect and alignmentfor (int i = 0; i < 20; i++) {std::string image = path_images + std::to_string(i) + ".jpg";//fprintf(stderr, "start process image: %s\n", image.c_str());cv::Mat src_ = cv::imread(image, 1);if (src_.empty()) {fprintf(stderr, "read image error: %s\n", image.c_str());continue;}cv::Mat src;cv::cvtColor(src_, src, CV_BGR2GRAY);seeta::ImageData img_data;img_data.data = src.data;img_data.width = src.cols;img_data.height = src.rows;img_data.num_channels = 1;std::vector<seeta::FaceInfo> faces = detector.Detect(img_data);if (faces.size() == 0) {fprintf(stderr, "%s don't detect face\n", image.c_str());continue;}// Detect 5 facial landmarks: two eye centers, nose tip and two mouth cornersstd::vector<seeta::FacialLandmark> landmard(5);alignment.PointDetectLandmarks(img_data, faces[0], &landmard[0]);landmards.push_back(landmard);cv::rectangle(src_, cv::Rect(faces[0].bbox.x, faces[0].bbox.y,faces[0].bbox.width, faces[0].bbox.height), cv::Scalar(0, 255, 0), 2);for (auto point : landmard) {cv::circle(src_, cv::Point(point.x, point.y), 2, cv::Scalar(0, 0, 255), 2);}std::string save_result = path_images + "_" + std::to_string(i) + ".jpg";cv::imwrite(save_result, src_);}int width = 200;int height = 200;cv::Mat dst(height * 5, width * 4, CV_8UC3);for (int i = 0; i < 20; i++) {std::string input_image = path_images + "_" + std::to_string(i) + ".jpg";cv::Mat src = cv::imread(input_image, 1);if (src.empty()) {fprintf(stderr, "read image error: %s\n", input_image.c_str());return -1;}cv::resize(src, src, cv::Size(width, height), 0, 0, 4);int x = (i * width) % (width * 4);int y = (i / 4) * height;cv::Mat part = dst(cv::Rect(x, y, width, height));src.copyTo(part);}std::string output_image = path_images + "result_alignment.png";cv::imwrite(output_image, dst);// crop imagefor (int i = 0; i < 20; i++) {std::string image = path_images + std::to_string(i) + ".jpg";//fprintf(stderr, "start process image: %s\n", image.c_str());cv::Mat src_img = cv::imread(image, 1);if (src_img.data == nullptr) {fprintf(stderr, "Load image error: %s\n", image.c_str());return -1;}if (face_recognizer.crop_channels() != src_img.channels()) {fprintf(stderr, "channels dismatch: %d, %d\n", face_recognizer.crop_channels(), src_img.channels());return -1;}// ImageData store data of an image without memory alignment.seeta::ImageData src_img_data(src_img.cols, src_img.rows, src_img.channels());src_img_data.data = src_img.data;// Create a image to store crop face.cv::Mat dst_img(face_recognizer.crop_height(), face_recognizer.crop_width(), CV_8UC(face_recognizer.crop_channels()));seeta::ImageData dst_img_data(dst_img.cols, dst_img.rows, dst_img.channels());dst_img_data.data = dst_img.data;// Crop Faceface_recognizer.CropFace(src_img_data, &landmards[i][0], dst_img_data);std::string save_image_name = path_images + "crop_" + std::to_string(i) + ".jpg";cv::imwrite(save_image_name, dst_img);}dst = cv::Mat(height * 5, width * 4, CV_8UC3);for (int i = 0; i < 20; i++) {std::string input_image = path_images + "crop_" + std::to_string(i) + ".jpg";cv::Mat src_img = cv::imread(input_image, 1);if (src_img.empty()) {fprintf(stderr, "read image error: %s\n", input_image.c_str());return -1;}cv::resize(src_img, src_img, cv::Size(width, height), 0, 0, 4);int x = (i * width) % (width * 4);int y = (i / 4) * height;cv::Mat part = dst(cv::Rect(x, y, width, height));src_img.copyTo(part);}output_image = path_images + "result_crop.png";cv::imwrite(output_image, dst);// extract featureint feat_size = face_recognizer.feature_size();if (feat_size != 2048) {fprintf(stderr, "feature size mismatch: %d\n", feat_size);return -1;}float* feat_sdk = new float[feat_size * 20];for (int i = 0; i < 20; i++) {std::string input_image = path_images + "crop_" + std::to_string(i) + ".jpg";cv::Mat src_img = cv::imread(input_image, 1);if (src_img.empty()) {fprintf(stderr, "read image error: %s\n", input_image.c_str());return -1;}cv::resize(src_img, src_img, cv::Size(face_recognizer.crop_height(), face_recognizer.crop_width()));// ImageData store data of an image without memory alignment.seeta::ImageData src_img_data(src_img.cols, src_img.rows, src_img.channels());src_img_data.data = src_img.data;// Extract featureface_recognizer.ExtractFeature(src_img_data, feat_sdk + i * feat_size);}float* feat1 = feat_sdk;// varify(recognize)for (int i = 1; i < 20; i++) {std::string image = std::to_string(i) + ".jpg";float* feat_other = feat_sdk + i * feat_size;// Caculate similarityfloat sim = face_recognizer.CalcSimilarity(feat1, feat_other);fprintf(stdout, "0.jpg -- %s similarity: %f\n", image.c_str(), sim);}delete[] feat_sdk;return 0;
}
从网上找了20张图像,前19张为周星驰,最后一张为汤唯,用于测试此模块,测试结果如下:detect/alignment结果如下:
crop结果如下:
取上图中最左上图为标准图,与其它19幅图作验证,测试结果如下:
GitHub:https://github.com/fengbingchun/Face_Test
相关文章:
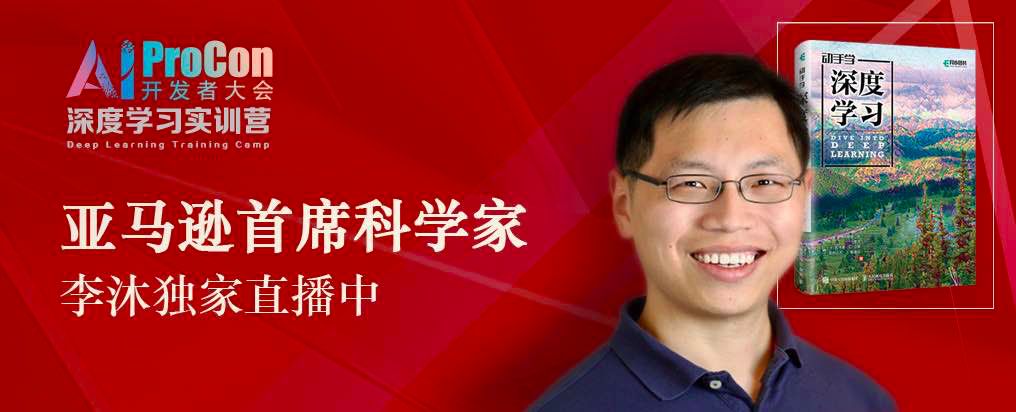
李沐亲授加州大学伯克利分校深度学习课程移师中国,现场资料新鲜出炉
2019 年 9 月 5 日,AI ProCon 2019 在北京长城饭店正式拉开帷幕。大会的第一天,以亚马逊首席科学家李沐面对面亲自授课完美开启!“大神”,是很多人对李沐的印象。除了是亚马逊首席科学家李,李沐还拥有多重身份…
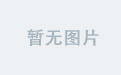
对Python课的看法
学习Python已经有两周的时间了,我是计算机专业的学生,我抱着可以多了解一种语言的想法报了Python的选修课,从第一次听肖老师的课开始,我便感受到一种好久没有感受到的课堂氛围,感觉十分舒服,不再是那种高中…
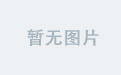
维护学习的一点体会与看法
学习维护的知识也有2个月了,对于知识的学习也有一定的看法。接下来我就说一下我对学习的看法。首先,你要学会自学,无论是看书还是上网查资料,维护的知识很多很杂,想要人一下子来教是不可能。只能是自己慢慢的学。其次&…
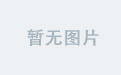
Dlib简介及在windows7 vs2013编译过程
Dlib是一个C库,包含了许多机器学习算法。它是跨平台的,可以应用在Windows、Linux、Mac、embedded devices、mobile phones等。它的License是Boost Software License 1.0,可以商用。Dlib的主要特点可以参考官方网站:http://dlib.ne…
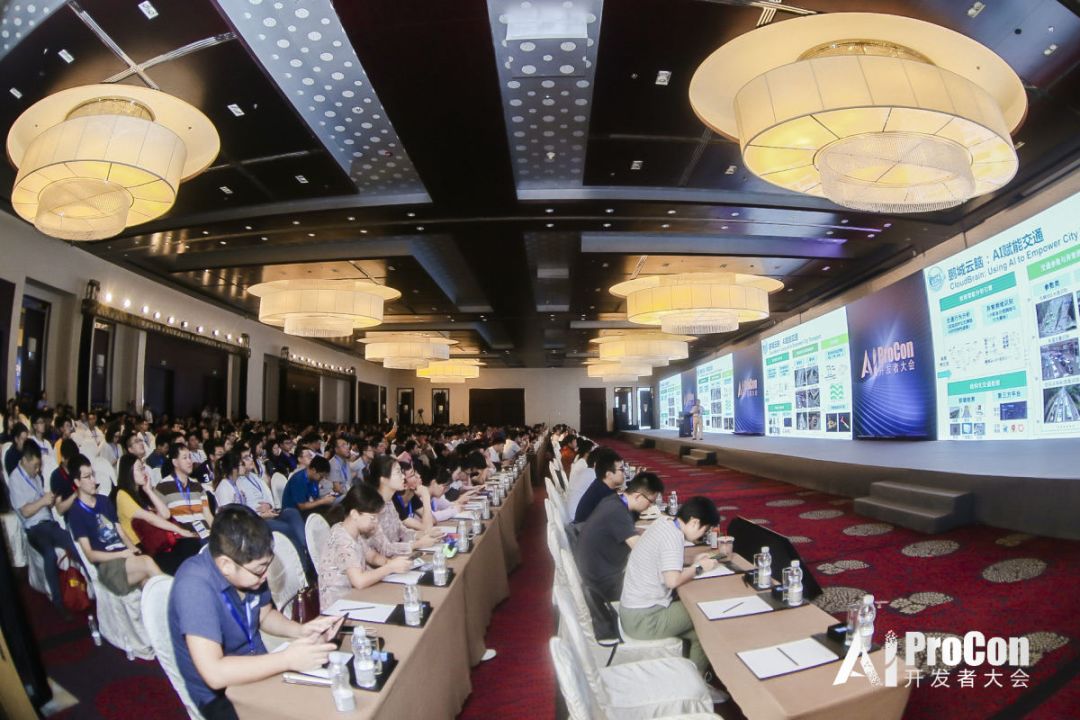
六大主题报告,四大技术专题,AI开发者大会首日精华内容全回顾
9月6-7日,2019中国AI开发者大会(AI ProCon 2019) 在北京拉开帷幕。本次大会由新一代人工智能产业技术创新战略联盟(AITISA)指导,鹏城实验室、北京智源人工智能研究院支持,专业中文IT技术社区CSD…
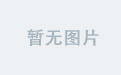
Git安装配置(Linux)
使用yum安装Git yum install git -y 编译安装 # 安装依赖关系 yum install curl-devel expat-devel gettext-devel openssl-devel zlib-devel # 编译安装 tar -zxf git-2.0.0.tar.gz cd git-2.0.0 make configure ./configure --prefix/usr make make install 配置Git…
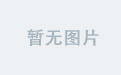
卷积神经网络(CNN)代码实现(MNIST)解析
在http://blog.csdn.net/fengbingchun/article/details/50814710中给出了CNN的简单实现,这里对每一步的实现作个说明:共7层:依次为输入层、C1层、S2层、C3层、S4层、C5层、输出层,C代表卷积层(特征提取),S代表降采样层…
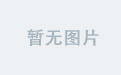
一点感悟和自勉
来学习linux,其实目的很简单,也很现实,就是为了学成之后找一份好的工作,能够养家糊口。 接触了几天,感觉挺难的,可能是因为我没有基础的原因吧,以前电脑只是用来打游戏和看电影,第一…
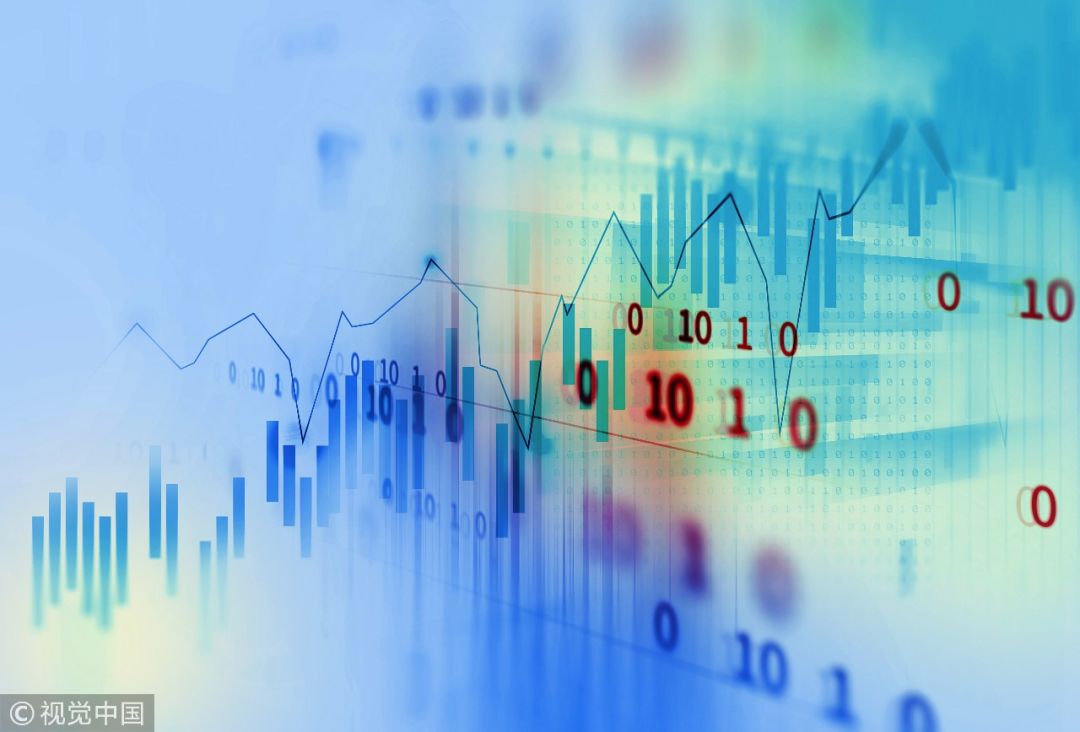
入门数据分析师,从了解元数据中心开始
作者丨凯凯连编辑丨Zandy来源 | 大数据与人工智能(ID:ai-big-data)【导读】上一篇文章,我们简单讲解了数据仓库的概念,并介绍了它的分层架构设计,相信大家对数据仓库体系已经有一定的了解了。那么ÿ…
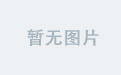
PhpMyAdmin的简单安装和一个mysql到Redis迁移的简单例子
1.phpmyadmin的安装 sudo apt-get install phpmyadmin 之后发现http://localhost/phpmyadmin显示没有此url,于是想到本机上能显示的网页都在/var/www文件夹内,因此执行命令行:sudo ln /usr/share/phpmyadmin /var/www 这样就能使用了; 之…

聊聊flink的HistoryServer
为什么80%的码农都做不了架构师?>>> 序 本文主要研究一下flink的HistoryServer HistoryServer flink-1.7.2/flink-runtime-web/src/main/java/org/apache/flink/runtime/webmonitor/history/HistoryServer.java public class HistoryServer {private st…
深度学习开源库tiny-dnn的使用(MNIST)
tiny-dnn是一个基于DNN的深度学习开源库,它的License是BSD 3-Clause。之前名字是tiny-cnn是基于CNN的,tiny-dnn与tiny-cnn相关又增加了些新层。此开源库很活跃,几乎每天都有新的提交,因此下面详细介绍下tiny-dnn在windows7 64bit …
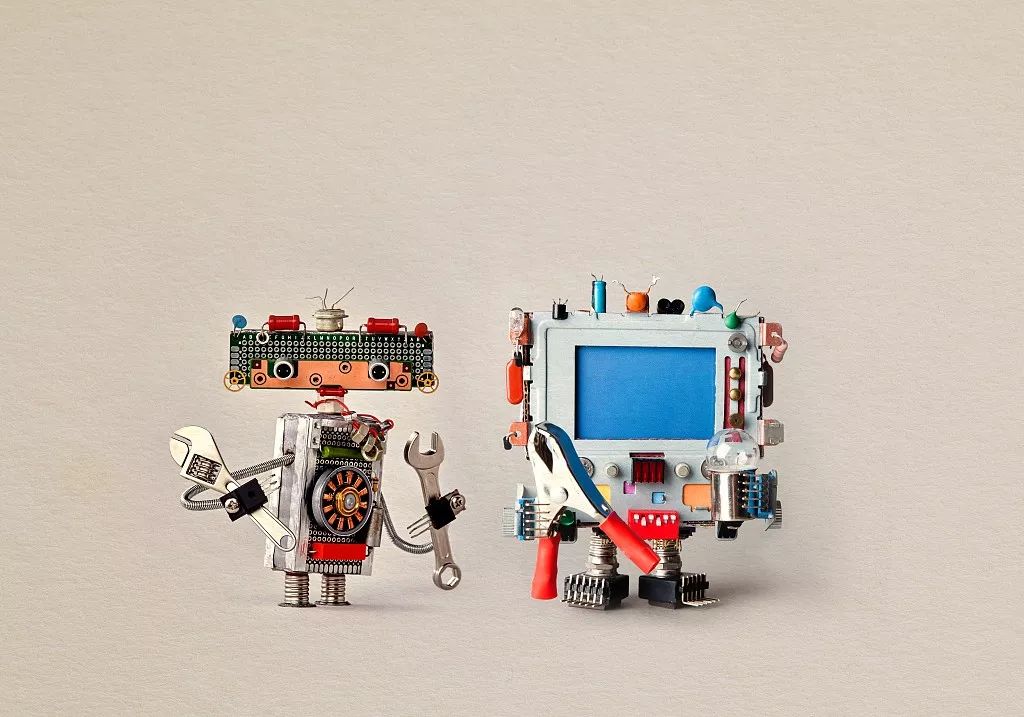
如何学习SVM?怎么改进实现SVM算法程序?答案来了
编辑 | 忆臻来源 | 深度学习这件小事(ID:DL_NLP)【导读】在 3D 动作识别领域,需要用到 SVM(支持向量机算法),但是现在所知道的 SVM 算法很多很乱,相关的程序包也很多,有什…

跟着石头哥哥学cocos2d-x(三)---2dx引擎中的内存管理模型
2019独角兽企业重金招聘Python工程师标准>>> 2dx引擎中的对象内存管理模型,很简单就是一个对象池引用计数,本着学好2dx的好奇心,先这里开走吧,紧接上面两节,首先我们看一个编码场景代码: hello…
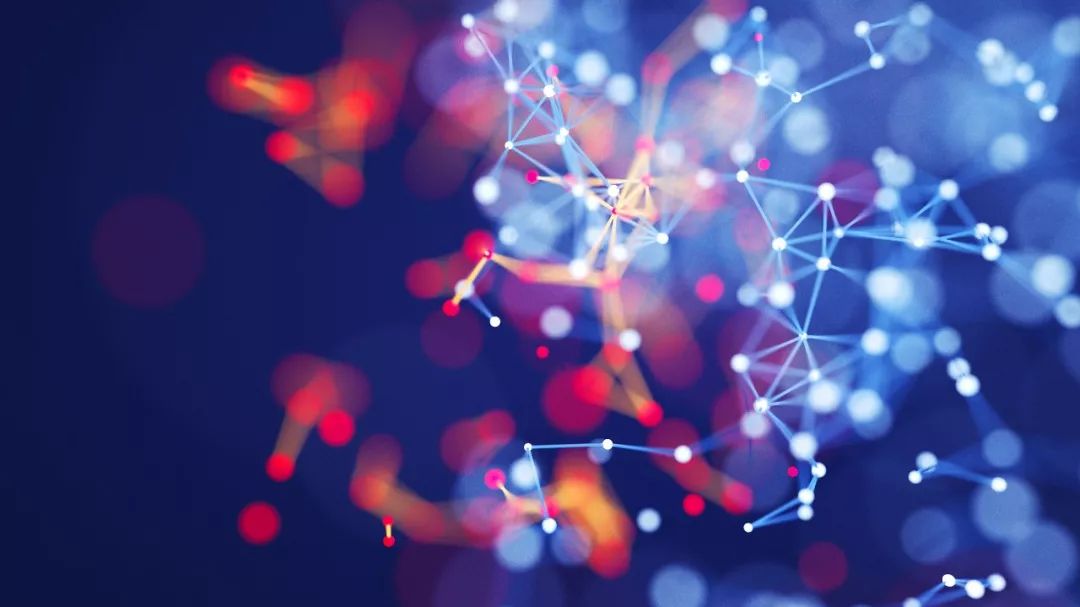
读8篇论文,梳理BERT相关模型进展与反思
作者 | 陈永强来源 | 微软研究院AI头条(ID:MSRAsia)【导读】BERT 自从在 arXiv 上发表以来获得了很大的成功和关注,打开了 NLP 中 2-Stage 的潘多拉魔盒。随后涌现了一大批类似于“BERT”的预训练(pre-trained)模型,有…
Dlib库中实现正脸人脸检测的测试代码
Dlib库中提供了正脸人脸检测的接口,这里参考dlib/examples/face_detection_ex.cpp中的代码,通过调用Dlib中的接口,实现正脸人脸检测的测试代码,测试代码如下:#include "funset.hpp" #include <string>…
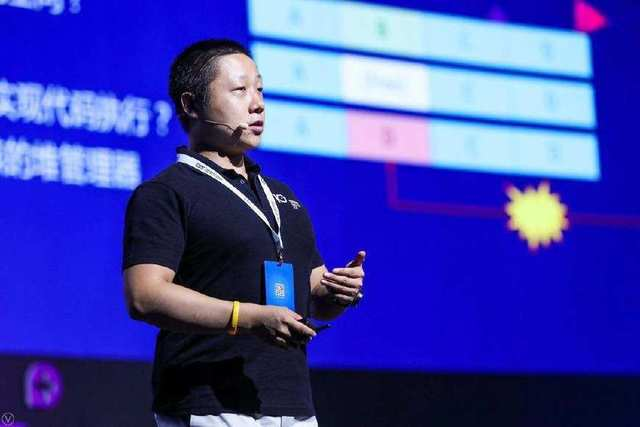
20189317 《网络攻防技术》 第二周作业
一.黑客信息 (1)国外黑客 1971年,卡普尔从耶鲁大学毕业。在校期间,他专修心理学、语言学以及计算机学科。也就是在这时他开始对计算机萌生兴趣。他继续到研究生院深造。20世纪60年代,退学是许多人的一个选择。只靠知识…
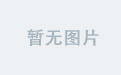
centos 6.4 SVN服务器多个项目的权限分组管理
根据本博客中的cent OS 6.4下的SVN服务器构建 一文,搭建好SVN服务器只能管理一个工程,如何做到不同的项目,多个成员的权限管理分配呢?一 需求开发服务器搭建好SVN服务器,不可能只管理一个工程项目,如何做到…
cifar数据集介绍及到图像转换的实现
CIFAR是一个用于普通物体识别的数据集。CIFAR数据集分为两种:CIFAR-10和CIFAR-100。The CIFAR-10 and CIFAR-100 are labeled subsets of the 80 million tiny images dataset. They were collected by Alex Krizhevsky, Vinod Nair, and Geoffrey Hinton.CIFAR-10由…
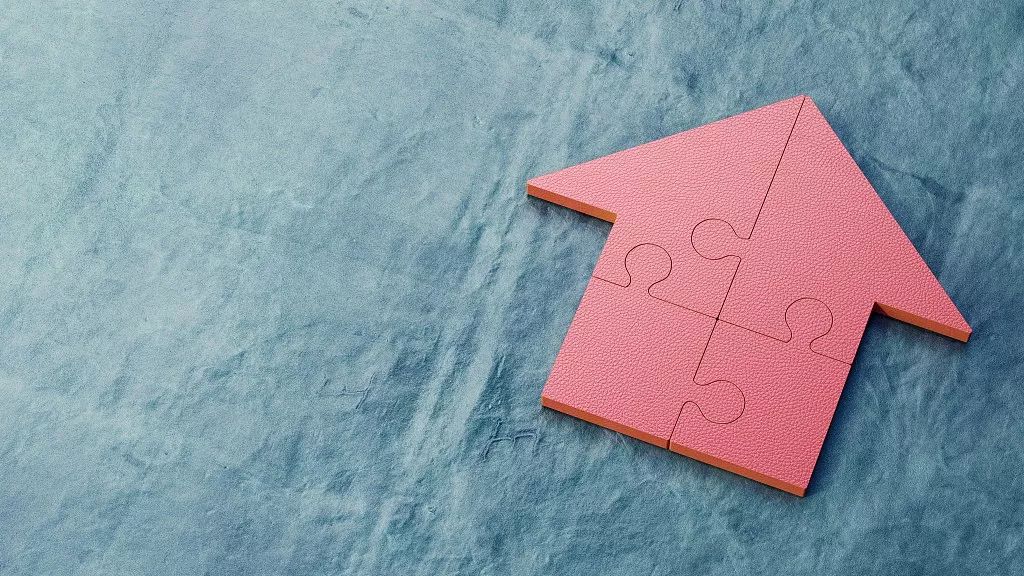
取代Python?Rust凭什么
作者 | Nathan J. Goldbaum译者 | 弯月,责编 | 屠敏来源 | CSDN(ID:CSDNnews)【导语】Rust 也能实现神经网络?在前一篇帖子中,作者介绍了MNIST数据集以及分辨手写数字的问题。在这篇文章中,他将…

【Mac】解决「无法将 chromedriver 移动到 /usr/bin 目录下」问题
问题描述 在搭建 Selenium 库 ChromeDriver 爬虫环境时,遇到了无法将 chromedriver 移动到 /usr/bin 目录下的问题,如下图: 一查原来是因为系统有一个 System Integrity Protection (SIP) 系统完整性保护,如果此功能不关闭&#…
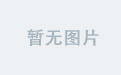
【译文】怎样让一天有36个小时
作者:Jon Bischke原文地址:How to Have a 36 Hour Day 你经常听人说“真希望一天能多几个小时”或者类似的话吗?当然,现实中我们每天只有24小时。这么说吧,人和人怎样度过这24个小时是完全不同的。到现在这样的说法已经…
Dlib库中实现正脸人脸关键点(landmark)检测的测试代码
Dlib库中提供了正脸人脸关键点检测的接口,这里参考dlib/examples/face_landmark_detection_ex.cpp中的代码,通过调用Dlib中的接口,实现正脸人脸关键点检测的测试代码,测试代码如下:/* reference: dlib/examples/face_l…
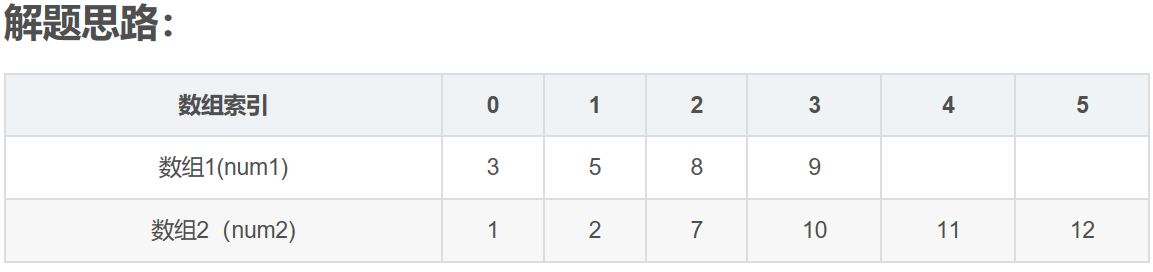
LeetCode--004--寻找两个有序数组的中位数(java)
转自https://blog.csdn.net/chen_xinjia/article/details/69258706 其中,N14,N26,size4610. 1,现在有的是两个已经排好序的数组,结果是要找出这两个数组中间的数值,如果两个数组的元素个数为偶数,则输出的是中间两个元…
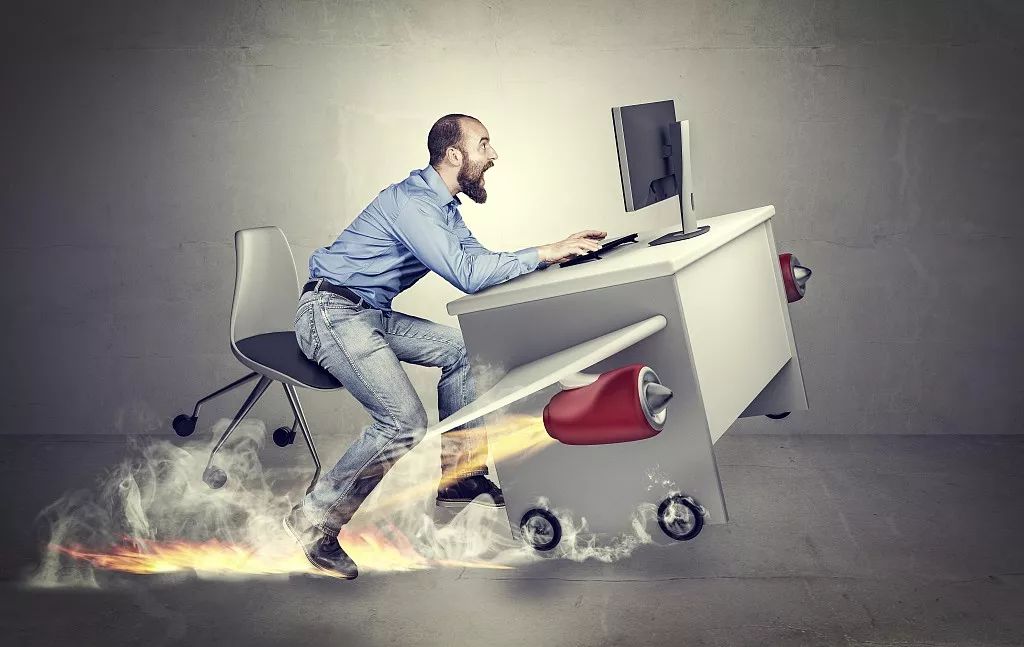
开源sk-dist,超参数调优仅需3.4秒,sk-learn训练速度提升100倍
作者 | Evan Harris译者 | Monanfei编辑 | Jane 出品 | AI科技大本营(ID:rgznai100)【导语】这篇文章为大家介绍了一个开源项目——sk-dist。在一台没有并行化的单机上进行超参数调优,需要 7.2 分钟,而在一百多个核心的 Spark 群集…
Windows和Linux下通用的线程接口
对于多线程开发,Linux下有pthread线程库,使用起来比较方便,而Windows没有,对于涉及到多线程的跨平台代码开发,会带来不便。这里参考网络上的一些文章,整理了在Windows和Linux下通用的线程接口。经过测试&am…
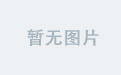
MySQL 性能调优的10个方法
MYSQL 应该是最流行了 WEB 后端数据库。WEB 开发语言最近发展很快,PHP, Ruby, Python, Java 各有特点,虽然 NOSQL 最近越來越多的被提到,但是相信大部分架构师还是会选择 MYSQL 来做数据存储。MYSQL 如此方便和稳定,以…
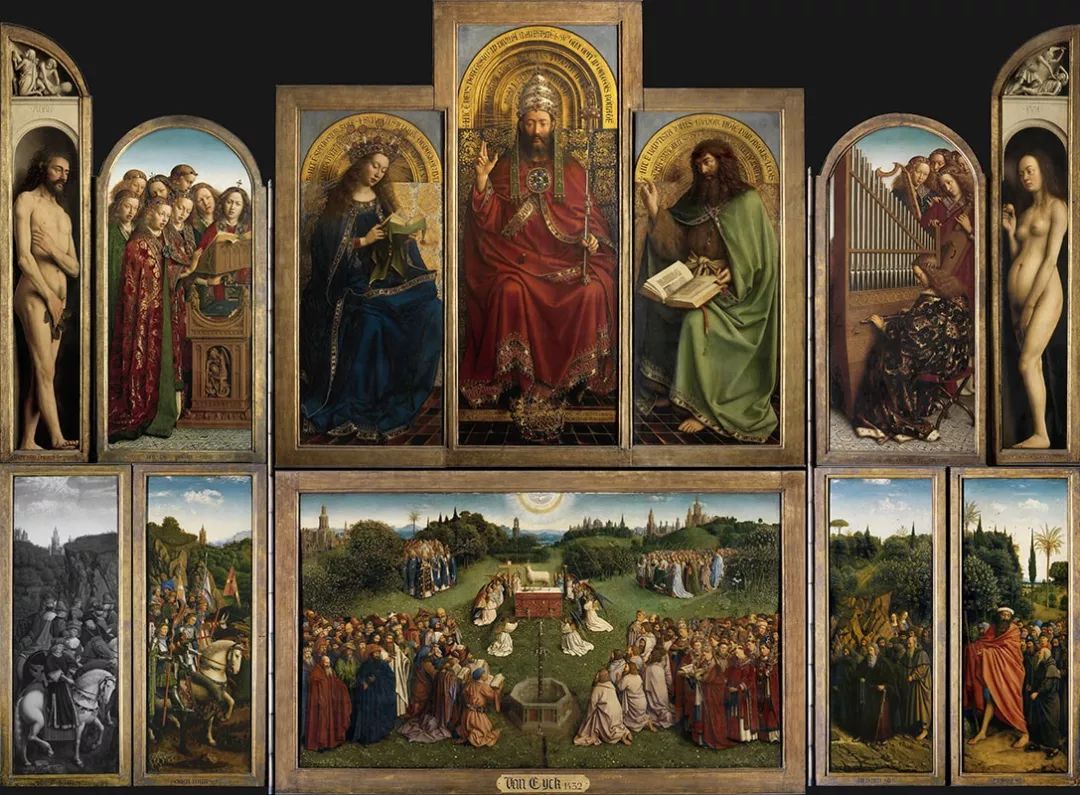
他们用卷积神经网络,发现了名画中隐藏的秘密
作者 | 神经小刀来源 |HyperAI超神经( ID: HyperAI)导语:著名的艺术珍品《根特祭坛画》,正在进行浩大的修复工作,以保证现在的人们能感受到这幅伟大的巨制,散发出的灿烂光芒。而随着技术的进步,…
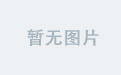
机器学习公开课~~~~mooc
https://class.coursera.org/ntumlone-001/class/index
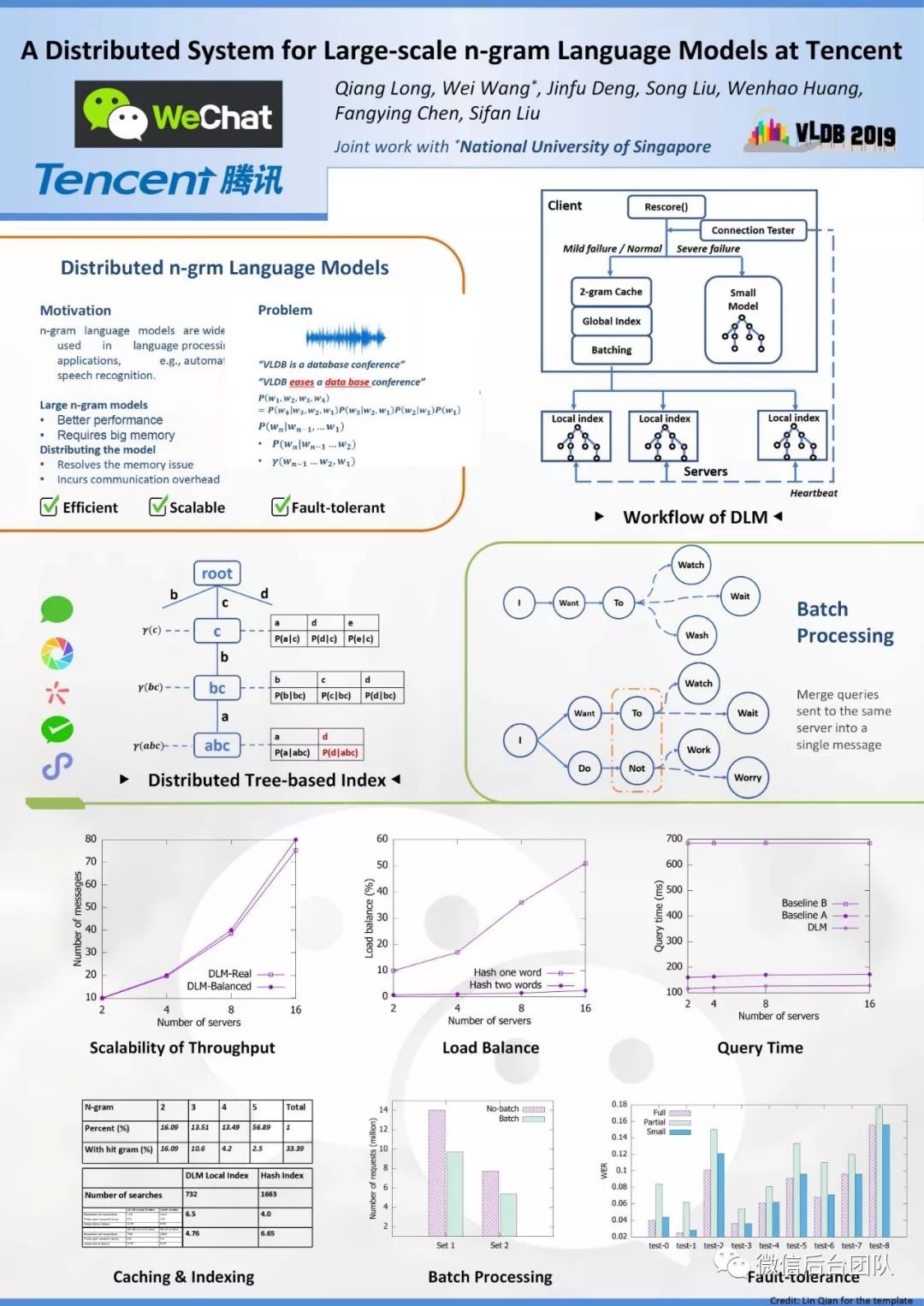
DLM:微信大规模分布式n-gram语言模型系统
来源 | 微信后台团队Wechat & NUS《A Distributed System for Large-scale n-gram Language Models at Tencent》分布式语言模型,支持大型n-gram LM解码的系统。本文是对原VLDB2019论文的简要翻译。摘要n-gram语言模型广泛用于语言处理,例如自动语音…