MNIST数据库介绍及转换
MNIST数据库介绍:MNIST是一个手写数字数据库,它有60000个训练样本集和10000个测试样本集。它是NIST数据库的一个子集。
MNIST数据库官方网址为:http://yann.lecun.com/exdb/mnist/ ,也可以在windows下直接下载,train-images-idx3-ubyte.gz、train-labels-idx1-ubyte.gz等。下载四个文件,解压缩。解压缩后发现这些文件并不是标准的图像格式。这些图像数据都保存在二进制文件中。每个样本图像的宽高为28*28。
以下为将其转换成普通的jpg图像格式的代码:
#include "funset.hpp"
#include <iostream>
#include <fstream>
#include <vector>
#include <opencv2/opencv.hpp>static int ReverseInt(int i)
{unsigned char ch1, ch2, ch3, ch4;ch1 = i & 255;ch2 = (i >> 8) & 255;ch3 = (i >> 16) & 255;ch4 = (i >> 24) & 255;return((int)ch1 << 24) + ((int)ch2 << 16) + ((int)ch3 << 8) + ch4;
}static void read_Mnist(std::string filename, std::vector<cv::Mat> &vec)
{std::ifstream file(filename, std::ios::binary);if (file.is_open()) {int magic_number = 0;int number_of_images = 0;int n_rows = 0;int n_cols = 0;file.read((char*)&magic_number, sizeof(magic_number));magic_number = ReverseInt(magic_number);file.read((char*)&number_of_images, sizeof(number_of_images));number_of_images = ReverseInt(number_of_images);file.read((char*)&n_rows, sizeof(n_rows));n_rows = ReverseInt(n_rows);file.read((char*)&n_cols, sizeof(n_cols));n_cols = ReverseInt(n_cols);for (int i = 0; i < number_of_images; ++i) {cv::Mat tp = cv::Mat::zeros(n_rows, n_cols, CV_8UC1);for (int r = 0; r < n_rows; ++r) {for (int c = 0; c < n_cols; ++c) {unsigned char temp = 0;file.read((char*)&temp, sizeof(temp));tp.at<uchar>(r, c) = (int)temp;}}vec.push_back(tp);}}
}static void read_Mnist_Label(std::string filename, std::vector<int> &vec)
{std::ifstream file(filename, std::ios::binary);if (file.is_open()) {int magic_number = 0;int number_of_images = 0;int n_rows = 0;int n_cols = 0;file.read((char*)&magic_number, sizeof(magic_number));magic_number = ReverseInt(magic_number);file.read((char*)&number_of_images, sizeof(number_of_images));number_of_images = ReverseInt(number_of_images);for (int i = 0; i < number_of_images; ++i) {unsigned char temp = 0;file.read((char*)&temp, sizeof(temp));vec[i] = (int)temp;}}
}static std::string GetImageName(int number, int arr[])
{std::string str1, str2;for (int i = 0; i < 10; i++) {if (number == i) {arr[i]++;str1 = std::to_string(arr[i]);if (arr[i] < 10) {str1 = "0000" + str1;} else if (arr[i] < 100) {str1 = "000" + str1;} else if (arr[i] < 1000) {str1 = "00" + str1;} else if (arr[i] < 10000) {str1 = "0" + str1;}break;}}str2 = std::to_string(number) + "_" + str1;return str2;
}int MNISTtoImage()
{// reference: http://eric-yuan.me/cpp-read-mnist/// test images and test labels// read MNIST image into OpenCV Mat vectorstd::string filename_test_images = "E:/GitCode/NN_Test/data/database/MNIST/t10k-images.idx3-ubyte";int number_of_test_images = 10000;std::vector<cv::Mat> vec_test_images;read_Mnist(filename_test_images, vec_test_images);// read MNIST label into int vectorstd::string filename_test_labels = "E:/GitCode/NN_Test/data/database/MNIST/t10k-labels.idx1-ubyte";std::vector<int> vec_test_labels(number_of_test_images);read_Mnist_Label(filename_test_labels, vec_test_labels);if (vec_test_images.size() != vec_test_labels.size()) {std::cout << "parse MNIST test file error" << std::endl;return -1;}// save test imagesint count_digits[10];std::fill(&count_digits[0], &count_digits[0] + 10, 0);std::string save_test_images_path = "E:/GitCode/NN_Test/data/tmp/MNIST/test_images/";for (int i = 0; i < vec_test_images.size(); i++) {int number = vec_test_labels[i];std::string image_name = GetImageName(number, count_digits);image_name = save_test_images_path + image_name + ".jpg";cv::imwrite(image_name, vec_test_images[i]);}// train images and train labels// read MNIST image into OpenCV Mat vectorstd::string filename_train_images = "E:/GitCode/NN_Test/data/database/MNIST/train-images.idx3-ubyte";int number_of_train_images = 60000;std::vector<cv::Mat> vec_train_images;read_Mnist(filename_train_images, vec_train_images);// read MNIST label into int vectorstd::string filename_train_labels = "E:/GitCode/NN_Test/data/database/MNIST/train-labels.idx1-ubyte";std::vector<int> vec_train_labels(number_of_train_images);read_Mnist_Label(filename_train_labels, vec_train_labels);if (vec_train_images.size() != vec_train_labels.size()) {std::cout << "parse MNIST train file error" << std::endl;return -1;}// save train imagesstd::fill(&count_digits[0], &count_digits[0] + 10, 0);std::string save_train_images_path = "E:/GitCode/NN_Test/data/tmp/MNIST/train_images/";for (int i = 0; i < vec_train_images.size(); i++) {int number = vec_train_labels[i];std::string image_name = GetImageName(number, count_digits);image_name = save_train_images_path + image_name + ".jpg";cv::imwrite(image_name, vec_train_images[i]);}// save big imagsstd::string images_path = "E:/GitCode/NN_Test/data/tmp/MNIST/train_images/";int width = 28 * 20;int height = 28 * 10;cv::Mat dst(height, width, CV_8UC1);for (int i = 0; i < 10; i++) {for (int j = 1; j <= 20; j++) {int x = (j-1) * 28;int y = i * 28;cv::Mat part = dst(cv::Rect(x, y, 28, 28));std::string str = std::to_string(j);if (j < 10)str = "0000" + str;elsestr = "000" + str;str = std::to_string(i) + "_" + str + ".jpg";std::string input_image = images_path + str;cv::Mat src = cv::imread(input_image, 0);if (src.empty()) {fprintf(stderr, "read image error: %s\n", input_image.c_str());return -1;}src.copyTo(part);}}std::string output_image = images_path + "result.png";cv::imwrite(output_image, dst);return 0;
}
结果如下图:相关文章:
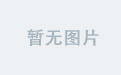
PostgreSQL学习笔记(1)
安装psql brew install postgresql 启动服务 brew services start postgresql 使用psql进入控制台,报错: psql: FATAL: database "<user>" does not exist 看来是没有给我的当前用户创建数据库,使用下面命令进入名为templat…

怎样使一个Android应用不被杀死?(整理)
2019独角兽企业重金招聘Python工程师标准>>> 方法 : 对于一个service,可以首先把它设为在前台运行: public void MyService.onCreate() { super.onCreate(); Notification notification new Notification(android.R.drawable.my_…
Ubuntu 14.04 64位机上用Caffe+MNIST训练Lenet网络操作步骤
1. 将终端定位到Caffe根目录; 2. 下载MNIST数据库并解压缩:$ ./data/mnist/get_mnist.sh 3. 将其转换成Lmdb数据库格式:$ ./examples/mnist/create_mnist.sh 执行完此shell脚本后,会在./examples/mnist下增加两个新…
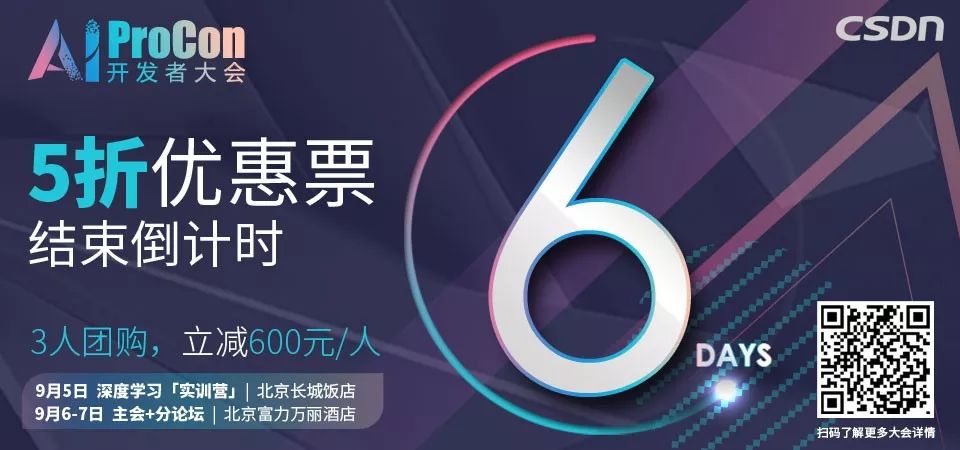
IJCAI 2019:中国团队录取论文超三成,北大、南大榜上有名
作者 | 神经小姐姐来源 | HyperAI超神经( ID: HyperAI )【导读】AI 顶会 IJCAI 2019 已于 8 月 16 日圆满落幕。在连续 7 天的技术盛会中,与会者在工作坊了解了 AI 技术在各个领域的应用场景,聆听了 AI 界前辈的主题演讲,还有机会…
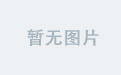
适合小小白的完整建设流程
时常有中小企业建站的客户问到我要自己建网站,应该怎么开始?建站有一定的技术门槛,首先要明白建站要做的哪些事情,里面有哪些坑,把流程弄清楚了才能避免入坑,半途而废!下面总结了建站的流程还有…

ios项目文件结构 目录的整理
2019独角兽企业重金招聘Python工程师标准>>> /<ProjectName>/Shared/Application # App delegate and related files/Controllers # Base view controllers/Models # Models, Core Data schema etc/Views # Shared views/Libr…
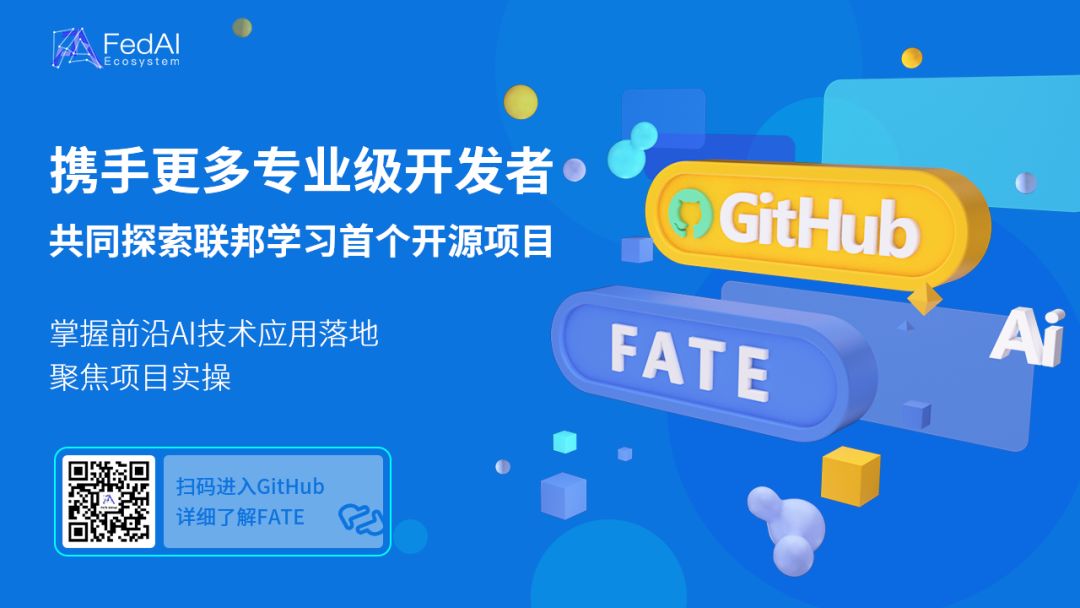
重磅!全球首个可视化联邦学习产品与联邦pipeline生产服务上线
【导读】作为全球首个联邦学习工业级技术框架,FATE支持联邦学习架构体系与各种机器学习算法的安全计算,实现了基于同态加密和多方计算(MPC)的安全计算协议,能够帮助多个组织机构在符合数据安全和政府法规前提下&#x…
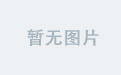
SpringBoot之集成swagger2
maven配置 <dependency><groupId>io.springfox</groupId><artifactId>springfox-swagger2</artifactId><version>2.5.0</version> </dependency> <dependency><groupId>io.springfox</groupId><artifact…
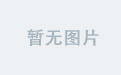
Windows Caffe中MNIST数据格式转换实现
Caffe源码中src/caffe/caffe/examples/mnist/convert_mnist_data.cpp提供的实现代码并不能直接在Windows下运行,这里在源码的基础上进行了改写,使其可以直接在Windows 64位上直接运行,改写代码如下:#include "stdafx.h"…

关于less在DW中高亮显示问题
首先, 找到DW 安装目录。 Adobe Dreamweaver CS5.5\configuration\DocumentTypes 中的,MMDocumentTypes.xml 这个文件,然后用记事本打开,查找CSS 把 CSS 后边加上,less 然后到。C:\Users\Administrator\AppData\Roamin…
Windows7 64bit VS2013 Caffe train MNIST操作步骤
1. 使用http://blog.csdn.net/fengbingchun/article/details/47905907中生成的Caffe静态库; 2. 使用http://blog.csdn.net/fengbingchun/article/details/49794453中生成的LMDB数据库文件; 3. 新建一个train_mnist控制台工程&#…
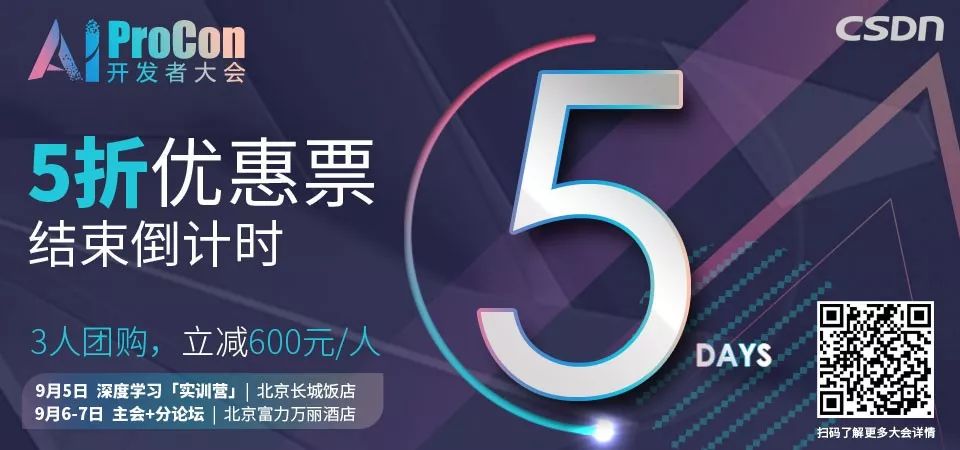
NLP机器翻译深度学习实战课程基础 | 深度应用
作者 | 小宋是呢来源 | CSDN博客0.前言深度学习用的有一年多了,最近开始 NLP 自然处理方面的研发。刚好趁着这个机会写一系列 NLP 机器翻译深度学习实战课程。本系列课程将从原理讲解与数据处理深入到如何动手实践与应用部署,将包括以下内容:…
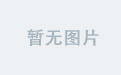
个人站点渲染和跳转过滤功能
核心逻辑:在url里加入正则,匹配分类、标签、年月日和其后面的参数,在视图函数接收这些参数,然后进行过滤。 urls.py # 个人站点的跳转 re_path(r^(?P<username>\w)/(?P<condition>tag|category|archive)/(?P<pa…

三步10分钟搞定数据库版本的降迁 (将后台数据库SQL2008R2降为SQL2005版本)
三步10分钟搞定数据库版本的降迁 (将SQL2008R2降为SQL2005版本)转载原文,并注明出处!虽无多少技术含量,毕竟是作者心血原创,希望理解。转自 http://blog.csdn.net/claro/article/details/6449824前思后想仍…

jdbc链接数据库
JDBC简介 JDBC全称为:Java Data Base Connectivity (java数据库连接),可以为多种数据库提供填统一的访问。JDBC是sun开发的一套数据库访问编程接口,是一种SQL级的API。它是由java语言编写完成,所以具有很好的跨平台特性…
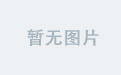
Google Protocol Buffers介绍
Google Protocol Buffers(简称Protobuf),是Google的一个开源项目,它是一种结构化数据存储格式,是Google公司内部的混合语言数据标准,是一个用来序列化(将对象的状态信息转换为可以存储或传输的形式的过程)结…
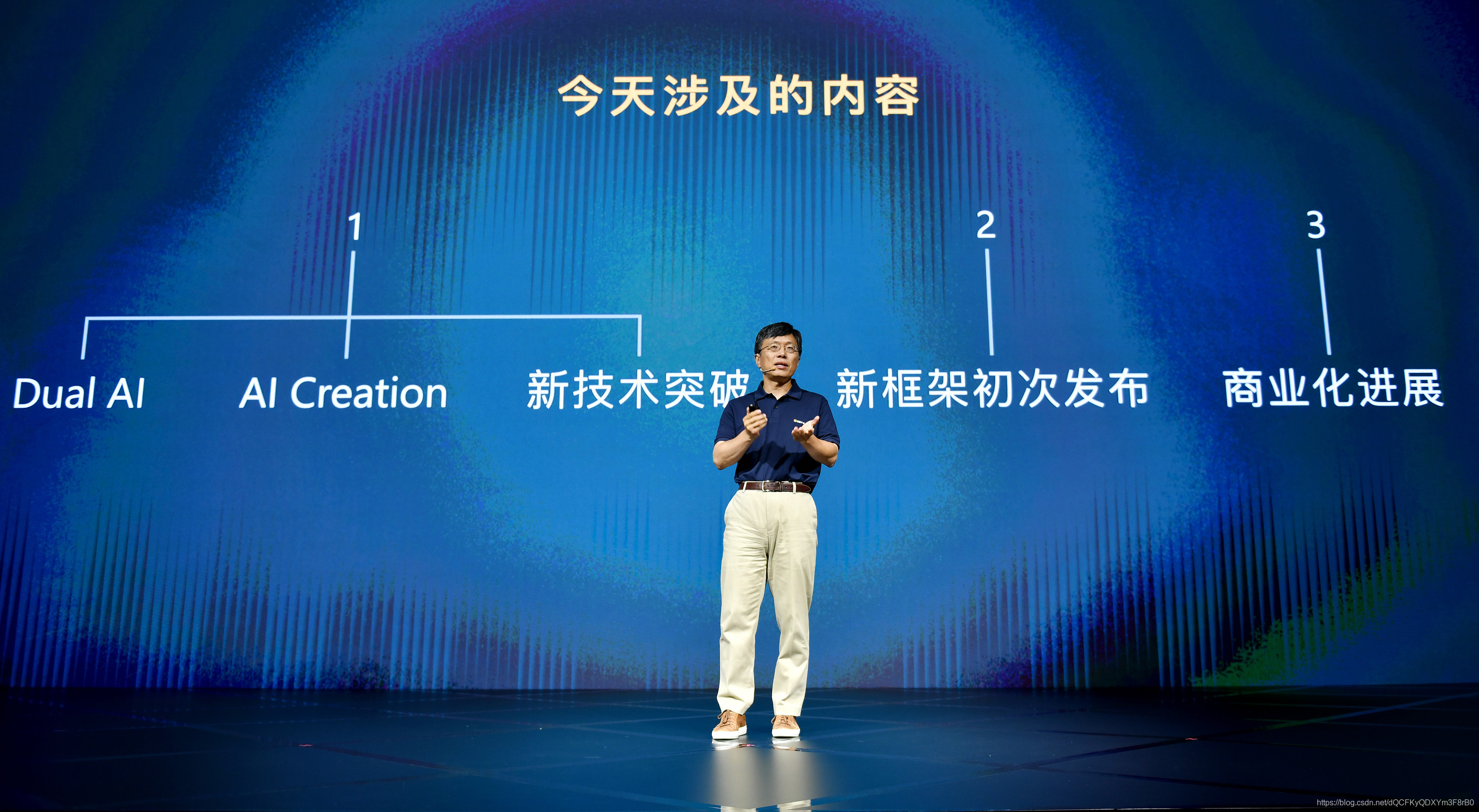
打造 AI Beings,和微信合作…第七代微软小冰的成长之路
8月15日, “第七代微软小冰”年度发布会在北京举行。本次发布会上,微软(亚洲)互联网工程院带来了微软小冰在 Dual AI 领域的新进展,全新升级的部分核心技术,最新的人工智能创造成果,以及更多的合作与产品落地。其中&am…
感知机介绍及实现
感知机(perceptron)由Rosenblatt于1957年提出,是神经网络与支持向量机的基础。感知机是最早被设计并被实现的人工神经网络。感知机是一种非常特殊的神经网络,它在人工神经网络的发展史上有着非常重要的地位,尽管它的能力非常有限,…
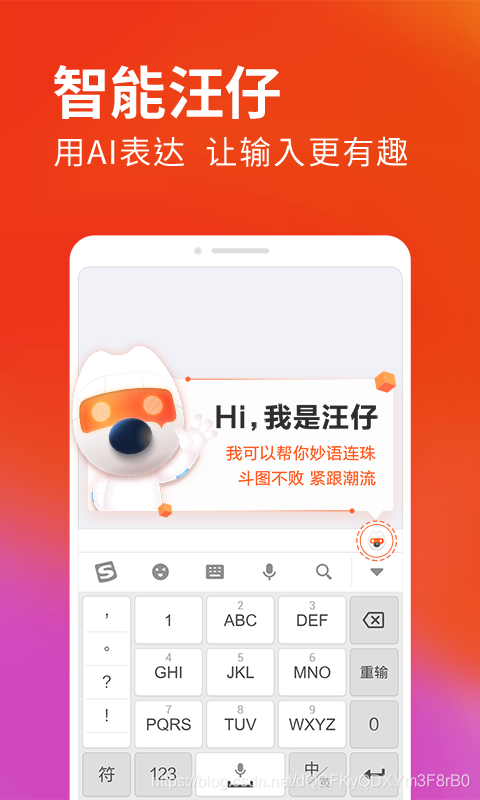
不甘心只做输入工具,搜狗输入法上线AI助手,提供智能服务
8月19日搜狗输入法上线了新功能——智能汪仔,在输入法中引入了AI助手,这是搜狗输入法继今年5月推出“语音变声功能”后又一个AI落地产品。 有了智能汪仔AI助手的加持后,搜狗输入法能够在不同的聊天场景,提供丰富多样的表达方式从…
可构造样式表 - 通过javascript来生成css的新方式
可构造样式表是一种使用Shadow DOM进行创建和分发可重用样式的新方法。 使用Javascript来创建样式表是可能的。然而,这个过程在历史上一直是使用document.createElement(style)来创建<style>元素,然后通过访问其sheet属性来获得一个基础的CSSStyle…

模板方法模式与策略模式的区别
2019独角兽企业重金招聘Python工程师标准>>> 模板方法模式:在一个方法中定义一个算法的骨架,而将一些步骤延迟到子类中。模板方法使得子类可以在不改变算法结构的情况下,重新定义算法中的某些步骤。 策略模式:定义一个…
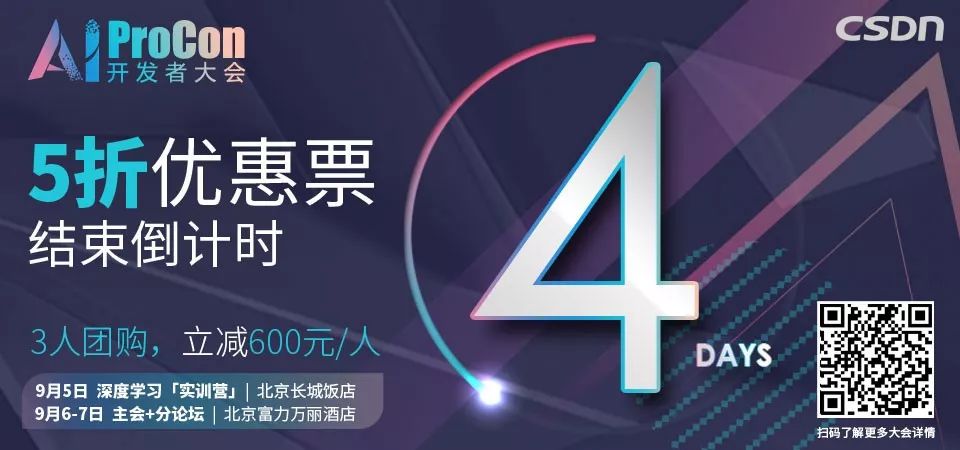
简单明了,一文入门视觉SLAM
作者 | 黄浴转载自知乎【导读】SLAM是“Simultaneous Localization And Mapping”的缩写,可译为同步定位与建图。最早,SLAM 主要用在机器人领域,是为了在没有任何先验知识的情况下,根据传感器数据实时构建周围环境地图,…
大主子表关联的性能优化方法
【摘要】主子表是数据库最常见的关联关系之一,最典型的包括合同和合同条款、订单和订单明细、保险保单和保单明细、银行账户和账户流水、电商用户和订单、电信账户和计费清单或流量详单。当主子表的数据量较大时,关联计算的性能将急剧降低,在…
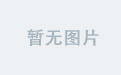
Windows7上配置Python Protobuf 操作步骤
1、 按照http://blog.csdn.net/fengbingchun/article/details/8183468 中步骤,首先安装Python 2.7.10; 2、 按照http://blog.csdn.net/fengbingchun/article/details/47905907 中步骤,配置、编译Protobuf; 3、 将(2)中生成的pr…
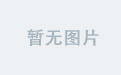
鲜为人知的静态、命令式编程语言——Nimrod
Nimrod是一个新型的静态类型、命令式编程语言,支持过程式、函数式、面向对象和泛型编程风格而保持简单和高效。Nimrod从Lisp继承来的一个特殊特性抽象语法树(AST)作为语言规范的一部分,可以用作创建领域特定语言的强大宏系统。它还…
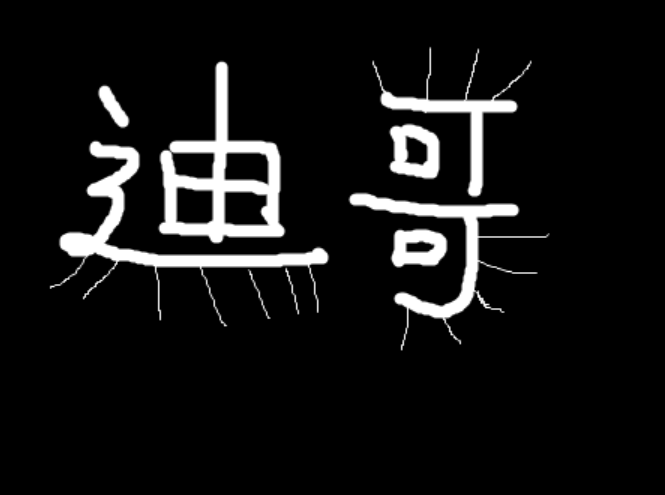
机器学习进阶-图像形态学操作-腐蚀操作 1.cv2.erode(进行腐蚀操作)
1.cv2.erode(src, kernel, iteration) 参数说明:src表示的是输入图片,kernel表示的是方框的大小,iteration表示迭代的次数 腐蚀操作原理:存在一个kernel,比如(3, 3),在图像中不断的平移,在这个9…
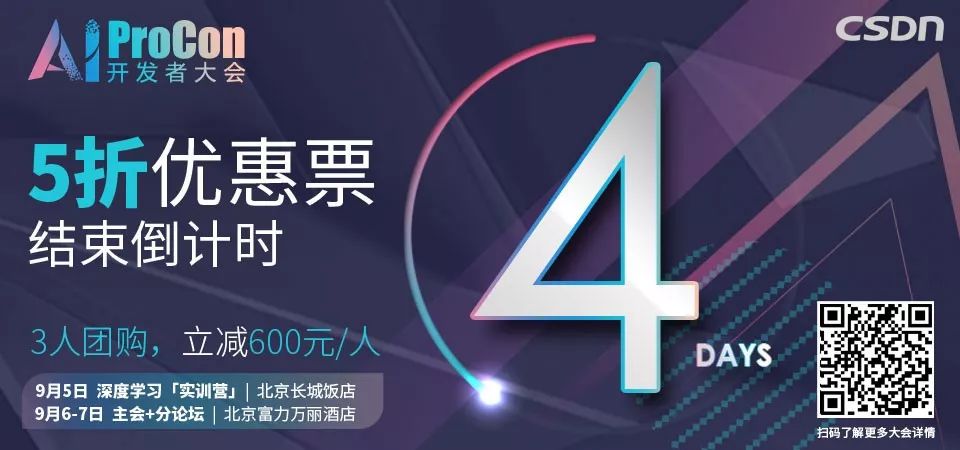
无需成对示例、无监督训练,CycleGAN生成图像简直不要太简单
作者 | Jason Brownlee译者 | Freesia,Rachel编辑 | 夕颜出品 | AI科技大本营(ID: rgznai100)【导读】图像到图像的转换技术一般需要大量的成对数据,然而要收集这些数据异常耗时耗力。因此本文主要介绍了无需成对示例便能实现图…
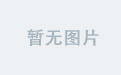
Git使用常见问题解决方法汇总
1. 在Ubuntu下使用$ git clone时出现server certificate verification failed. CAfile:/etc/ssl/certs/ca-certificates.crt CRLfile: none 解决方法:在执行$ git clone 之前,在终端输入: export GIT_SSL_NO_VERIFY1 2. 在Windows上更新了…

服务器监控常用命令
在网站性能优化中,我们经常要检查服务器的各种指标,以便快速找到害群之马。大多情况下,我们会使用cacti、nagois或者zabbix之类的监控软件,但是这类软件安装起来比较麻烦,在一个小型服务器,我们想尽快找到问…
Ubuntu下内存泄露检测工具Valgrind的使用
在VS中可以用VLD检测是否有内存泄露,可以参考http://blog.csdn.net/fengbingchun/article/details/44195959,下面介绍下Ubuntu中内存泄露检测工具Valgrind的使用。Valgrind目前最新版本是3.11.0, 可以从http://www.valgrind.org/ 通过下载源码…