C++中const指针用法汇总
这里以int类型为例,进行说明,在C++中const是类型修饰符:
int a; 定义一个普通的int类型变量a,可对此变量的值进行修改。
const int a = 3;与 int const a = 3; 这两条语句都是有效的code,并且是等价的,说明a是一个常量,不能对此常量的值进行修改。
const int* p =&a; 与 int const* p = &a; 这两条语句都是有效的code,但是它们不是等价的。其中const int* p = &a; 是平时经常使用的格式,声明一个指针,此指针指向的数据不能通过此指针被改变;int* const p = ∫ 声明一个指针,此指针不能被改变以指向别的东西。
const int *a; 这里const修饰的是int,而int定义的是一个整值,因此*a所指向的对象值不能通过*a来修改,但是可以重新给a来赋值,使其指向不同的对象;
int *const a; 这里const修饰的是a,a代表的是一个指针地址,因此不能赋给a其他的地址值,但可以修改a指向的值;
int const *a;和const int *a;的意义是相同的,它们两个的作用等价;
rules:
(1). A non-const pointer can be redirected to point to other addresses.
(2). A const pointer always points to the same address, and this address can not be changed.
(3). A pointer to a non-const value can change the value it is pointing to. These can not point to a const value.
(4). A pointer to a const value treats the value as const (even if it is not), and thus can not change the value it is pointing to.
以下是一些test code,详细信息可见相关的reference:
#include <iostream>
#include "const_pointer.hpp"int test_const_pointer_1()
{
{ // reference: https://stackoverflow.com/questions/3247285/const-int-int-constint a = 5;int *p1 = &a; //non-const pointer, non-const dataconst int *p2 = &a; //non-const pointer, const data, value pointed to by p2 can’t changeint * const p3 = &a; //const pointer, non-const data, p3 cannot point to a different locationconst int * const p4 = &a; //const pointer, const data, both the pointer and the value pointed to cannot changeint const * const p5 = &a; // 与 const int * const p5等价
}{ // reference: https://stackoverflow.com/questions/162480/const-int-vs-int-const-as-function-parameter-in-c-and-c// read the declaration backwards (right-to-left):const int a1 = 1; // read as "a1 is an integer which is constant"int const a2 = 1; // read as "a2 is a constant integer"// a1 = 2; // Can't do because a1 is constant// a2 = 2; // Can't do because a2 is constantchar a = 'a';const char *s = &a; // read as "s is a pointer to a char that is constant"char const *y = &a; // 与 const char *y 等价char c;char *const t = &c; // read as "t is a constant pointer to a char"// *s = 'A'; // Can't do because the char is constants++; // Can do because the pointer isn't constant*t = 'A'; // Can do because the char isn't constant// t++; // Can't do because the pointer is constant// *y = 'A';y++;
}{ // reference: http://www.geeksforgeeks.org/const-qualifier-in-c/int i = 10;int j = 20;int *ptr = &i; /* pointer to integer */printf("*ptr: %d\n", *ptr);/* pointer is pointing to another variable */ptr = &j;printf("*ptr: %d\n", *ptr);/* we can change value stored by pointer */*ptr = 100;printf("*ptr: %d\n", *ptr);
}{ // const int *ptr <==> int const *ptrint i = 10;int j = 20;const int *ptr = &i; /* ptr is pointer to constant */printf("ptr: %d\n", *ptr);// *ptr = 100; /* error: object pointed cannot be modified using the pointer ptr */ptr = &j; /* valid */printf("ptr: %d\n", *ptr);
}{ // int * const ptrint i = 10;int j = 20;int *const ptr = &i; /* constant pointer to integer */printf("ptr: %d\n", *ptr);*ptr = 100; /* valid */printf("ptr: %d\n", *ptr);// ptr = &j; /* error */
}{ // const int *const ptr;int i = 10;int j = 20;const int *const ptr = &i; /* constant pointer to constant integer */printf("ptr: %d\n", *ptr);// ptr = &j; /* error */// *ptr = 100; /* error */
}{ // reference: http://www.learncpp.com/cpp-tutorial/610-pointers-and-const/int value = 5;int *ptr = &value;*ptr = 6; // change value to 6const int value2 = 5; // value is const// int *ptr2 = &value2; // compile error: cannot convert const int* to int*const int *ptr3 = &value2; // this is okay, ptr3 is pointing to a "const int"// *ptr3 = 6; // not allowed, we can't change a const valueconst int *ptr4 = &value; // ptr4 points to a "const int"fprintf(stderr, "*ptr4: %d\n", *ptr4);value = 7; // the value is non-const when accessed through a non-const identifierfprintf(stderr, "*ptr4: %d\n", *ptr4);
}return 0;
}//
// reference: https://en.wikipedia.org/wiki/Const_(computer_programming)
class C {int i;
public:int Get() const { // Note the "const" tagreturn i;}void Set(int j) { // Note the lack of "const"i = j;}
};static void Foo_1(C& nonConstC, const C& constC)
{int y = nonConstC.Get(); // Okint x = constC.Get(); // Ok: Get() is constnonConstC.Set(10); // Ok: nonConstC is modifiable// constC.Set(10); // Error! Set() is a non-const method and constC is a const-qualified object
}class MyArray {int data[100];
public:int & Get(int i) { return data[i]; }int const & Get(int i) const { return data[i]; }
};static void Foo_2(MyArray & array, MyArray const & constArray) {// Get a reference to an array element// and modify its referenced value.array.Get(5) = 42; // OK! (Calls: int & MyArray::Get(int))// constArray.Get(5) = 42; // Error! (Calls: int const & MyArray::Get(int) const)
}typedef struct S_ {int val;int *ptr;
} S;void Foo_3(const S & s)
{int i = 42;// s.val = i; // Error: s is const, so val is a const int// s.ptr = &i; // Error: s is const, so ptr is a const pointer to int// *s.ptr = i; // OK: the data pointed to by ptr is always mutable,// even though this is sometimes not desirable
}int test_const_pointer_2()
{C a, b;Foo_1(a, b);MyArray x, y;Foo_2(x, y);S s;Foo_3(s);return 0;
}
GitHub: https://github.com/fengbingchun/Messy_Test
相关文章:
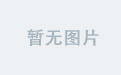
mongodb基础应用
一些概念 一个mongod服务可以有建立多个数据库,每个数据库可以有多张表,这里的表名叫collection,每个collection可以存放多个文档(document),每个文档都以BSON(binary json)的形式存…
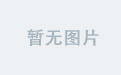
【leetcode】1030. Matrix Cells in Distance Order
题目如下: We are given a matrix with R rows and C columns has cells with integer coordinates (r, c), where 0 < r < R and 0 < c < C. Additionally, we are given a cell in that matrix with coordinates (r0, c0). Return the coordinates of…
深度学习面临天花板,亟需更可信、可靠、安全的第三代AI技术|AI ProCon 2019
整理 | 夕颜 出品 | AI科技大本营(ID:rgznai100) 在人工智能领域中,深度学习掀起了最近一次浪潮,但在实践和应用中也面临着诸多挑战,特别是关系到人的生命,如医疗、自动驾驶等领域场景时,黑盒…

java robot类自动截屏
直接上代码:package robot;import java.awt.Rectangle;import java.awt.Robot;import java.awt.event.InputEvent;import java.awt.p_w_picpath.BufferedImage;import java.io.File;import java.io.IOException;import javax.p_w_picpathio.ImageIO;import com.sun.glass.event…
激活函数之softmax介绍及C++实现
下溢(underflow):当接近零的数被四舍五入为零时发生下溢。许多函数在其参数为零而不是一个很小的正数时才会表现出质的不同。例如,我们通常要避免被零除或避免取零的对数。上溢(overflow):当大量级的数被近似为∞或-∞时发生上溢。进一步的运…
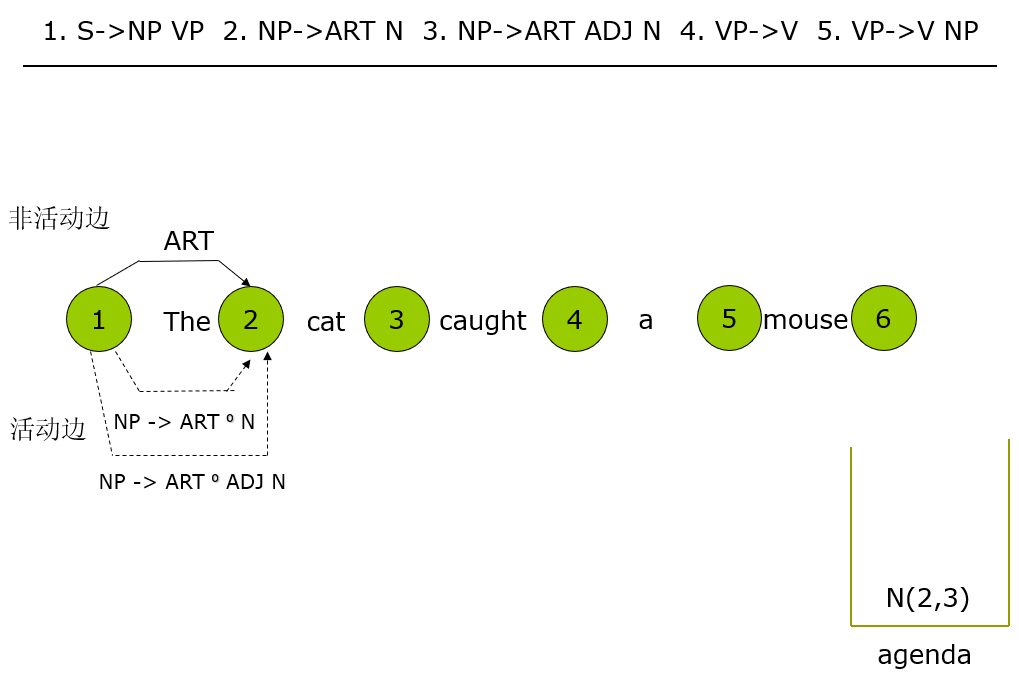
parsing:NLP之chart parser句法分析器
已迁移到我新博客,阅读体验更佳parsing:NLP之chart parser句法分析器 完整代码实现放在我的github上:click me 一、任务要求 实现一个基于简单英语语法的chart句法分析器。二、技术路线 采用自底向上的句法分析方法,简单的自底向上句法分析效率不高,常常…
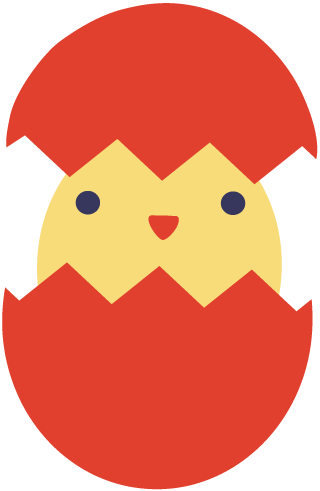
图解Python算法
普通程序员,不学算法,也可以成为大神吗?对不起,这个,绝对不可以。可是算法好难啊~~看两页书就想睡觉……所以就不学了吗?就一直当普通程序员吗?如果有一本算法书,看着很轻松……又有…
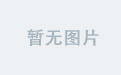
详解SSH框架的原理和优点
Struts的原理和优点. Struts工作原理 MVC即Model-View-Controller的缩写,是一种常用的设计模式。MVC 减弱了业务逻辑接口和数据接口之间的耦合,以及让视图层更富于变化。MVC的工作原理,如下图1所示:Struts 是MVC的一种实现࿰…

Numpy and Matplotlib
Numpy介绍 编辑 一个用python实现的科学计算,包括:1、一个强大的N维数组对象Array;2、比较成熟的(广播)函数库;3、用于整合C/C和Fortran代码的工具包;4、实用的线性代数、傅里叶变换和随机数生成…
梯度下降法简介
条件数表征函数相对于输入的微小变化而变化的快慢程度。输入被轻微扰动而迅速改变的函数对于科学计算来说可能是有问题的,因为输入中的舍入误差可能导致输出的巨大变化。大多数深度学习算法都涉及某种形式的优化。优化指的是改变x以最小化或最大化某个函数f(x)的任务…
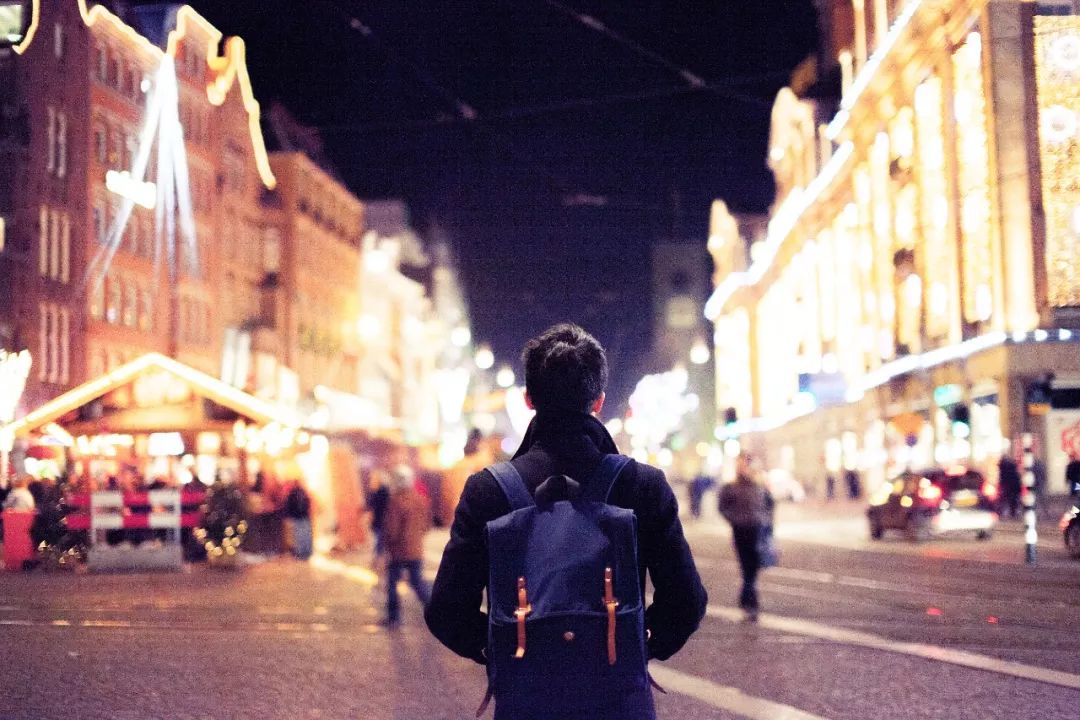
微软亚研院CV大佬代季峰跳槽商汤为哪般?
整理 | 夕颜出品 | AI科技大本营(ID:rgznai100)近日,知乎上一篇离开关于MSRA(微软亚洲研究院)和MSRA CV未来发展的帖子讨论热度颇高,这个帖子以MSRA CV执行研究主任代季峰离职加入商汤为引子,引…

iOS Block实现探究
2019独角兽企业重金招聘Python工程师标准>>> 使用clang的rewrite-objc filename 可以将有block的c代码转换成cpp代码。从中可以看到block的实现。 #include <stdio.h> int main() {void (^blk)(void) ^{printf("Block\n");};blk();return 0; } 使…
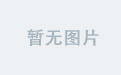
CUDA Samples: Long Vector Add
以下CUDA sample是分别用C和CUDA实现的两个非常大的向量相加操作,并对其中使用到的CUDA函数进行了解说,各个文件内容如下:common.hpp:#ifndef FBC_CUDA_TEST_COMMON_HPP_ #define FBC_CUDA_TEST_COMMON_HPP_#include<random>template&l…
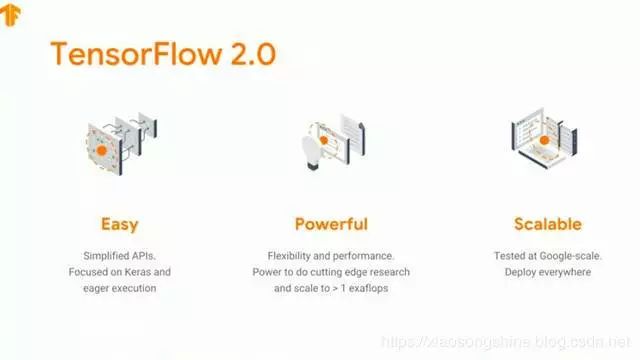
TensorFlow2.0正式版发布,极简安装TF2.0(CPUGPU)教程
作者 | 小宋是呢转载自CSDN博客【导读】TensorFlow 2.0,昨天凌晨,正式放出了2.0版本。不少网友表示,TensorFlow 2.0比PyTorch更好用,已经准备全面转向这个新升级的深度学习框架了。本篇文章就带领大家用最简单地方式安装TF2.0正式…
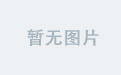
javascript全栈开发实践-准备
目标: 我们将会通过一些列教程,在只使用JavaScript开发的情况下,实现一个手写笔记应用。该应用具有以下特点: 全平台,有手机客户端(Android/iOS),Windows,macOSÿ…

POJ 1017 Packets 贪心 模拟
一步一步模拟,做这种题好累 先放大的的,然后记录剩下的空位有多少,塞1*1和2*2的进去 //#pragma comment(linker, "/STACK:1024000000,1024000000") #include<cstdio> #include<cstring> #include<cstdlib> #incl…
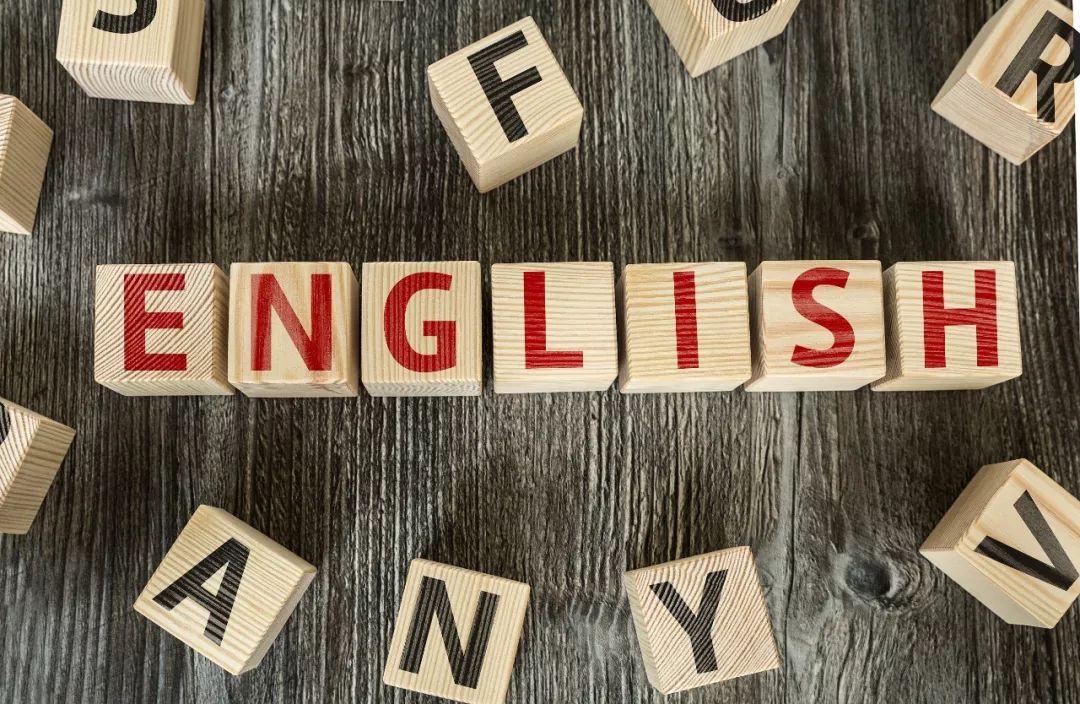
NLP被英语统治?打破成见,英语不应是「自然语言」同义词
(图片付费下载自视觉中国)作者 | Emily M. Bender译者 | 陆离责编 | 夕颜出品 | AI科技大本营(ID: rgznai100) 【导读】在NLP领域,多资源语言以英语、汉语(普通话)、阿拉伯语和法语为代表&#…
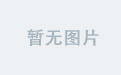
CUDA Samples: Dot Product
以下CUDA sample是分别用C和CUDA实现的两个非常大的向量实现点积操作,并对其中使用到的CUDA函数进行了解说,各个文件内容如下:common.hpp:#ifndef FBC_CUDA_TEST_COMMON_HPP_ #define FBC_CUDA_TEST_COMMON_HPP_#include<random>templa…

element ui只输入数字校验
注意:圈起来的两个地方,刚开始忘记写typenumber了,导致可以输入‘123abc’这样的,之后加上了就OK了 转载于:https://www.cnblogs.com/samsara-yx/p/10774270.html

对DeDecms之index.php页面的补充
2019独角兽企业重金招聘Python工程师标准>>> 1、301是什么? 其实就是HTTP状态表。就是当用户输入url请求时,服务器的一个反馈状态。 详细链接http://www.cnblogs.com/kunhony/archive/2006/06/16/427305.html 2、common.inc.php和arc.partvi…
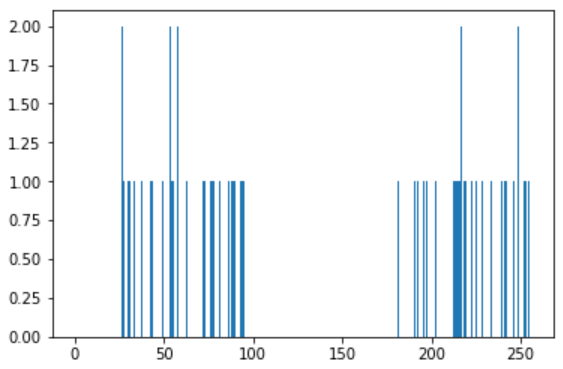
OpenCV-Python:K值聚类
关于K聚类,我曾经在一篇博客中提到过,这里简单的做个回顾。 KMeans的步骤以及其他的聚类算法 K-均值是因为它可以发现k个不同的簇,且每个簇的中心采用簇中所含值的均值计算 其他聚类算法:二分K-均值 讲解一下步骤,其实…
CUDA Samples: Julia
以下CUDA sample是分别用C和CUDA实现的绘制Julia集曲线,并对其中使用到的CUDA函数进行了解说,code参考了《GPU高性能编程CUDA实战》一书的第四章,各个文件内容如下:funset.cpp:#include "funset.hpp" #include <rand…
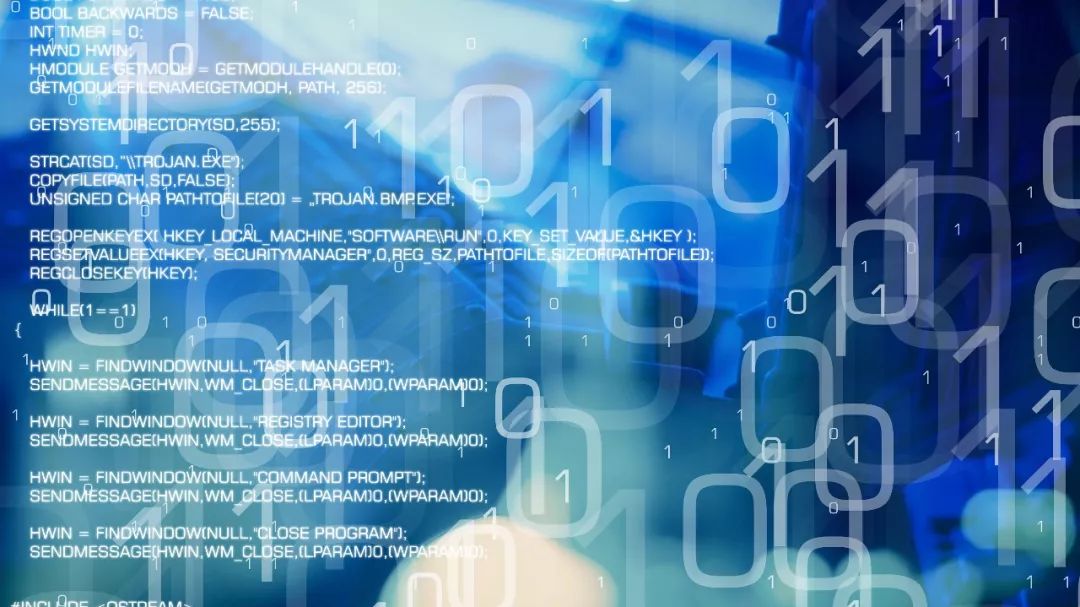
给初学者的深度学习入门指南
从无人驾驶汽车到AlphaGo战胜人类,机器学习成为了当下最热门的技术。而机器学习中一种重要的方法就是深度学习。作为一个有理想的程序员,若是不懂人工智能(AI)领域中深度学习(DL)这个超热的技术,…

epoll/select
为什么80%的码农都做不了架构师?>>> epoll相对select优点主要有三: 1. select的句柄数目受限,在linux/posix_types.h头文件有这样的声明:#define __FD_SETSIZE 1024 表示select最多同时监听1024个fd。而epoll没…
CUDA Samples: ripple
以下CUDA sample是分别用C和CUDA实现的生成的波纹图像,并对其中使用到的CUDA函数进行了解说,code参考了《GPU高性能编程CUDA实战》一书的第五章,各个文件内容如下:funset.cpp:#include "funset.hpp" #includ…
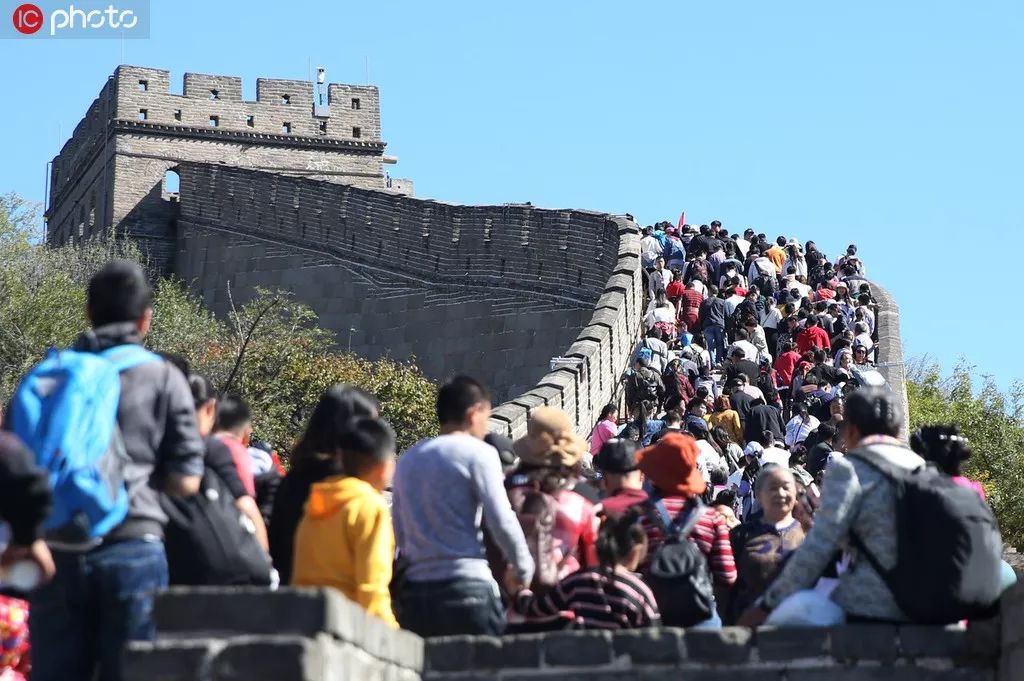
Python告诉你这些旅游景点好玩、便宜、人又少!
(图片由CSDN付费下载自东方IC)作者 | 猪哥来源 | 裸睡的猪(ID:IT--Pig) 2019年国庆马上就要到来,今年来点新花样吧,玩肯定是要去玩的,不然怎么给祖国庆生?那去哪里玩&…
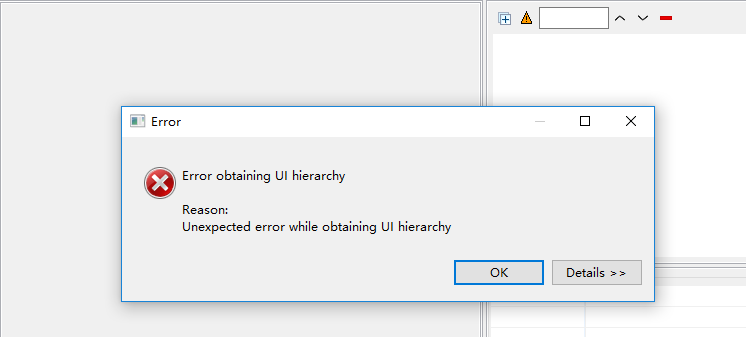
手机APP自动化之uiautomator2 +python3 UI自动化
题记: 之前一直用APPium直到用安卓9.0 发现uiautomatorviewer不支持安卓 9.0,点击截屏按钮 一直报错,百度很久解决方法都不可以,偶然间看见有人推荐:uiautomator2 就尝试使用 发现比appium要简单一些; 下面…
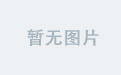
爱上MVC3系列~开发一个站点地图(俗称面包屑)
回到目录 原来早在webform控件时代就有了SiteMap这个东西,而进行MVC时代后,我们也希望有这样一个东西,它为我们提供了不少方便,如很方便的实现页面导航的内容修改,页面导航的样式换肤等. 我的MvcSiteMap主要由实体文件,XML配置文件,C#调用文件组成,当然为了前台使用方便,可以为…
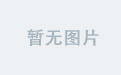
Django web框架-----Django连接现有mysql数据库
第一步:win10下载mysql5.7压缩包配置安装mysql,创建数据库或导入数据库 第二步:win10搭建django2.1.7开发环境,创建项目为mytestsite,创建应用app为quicktool 第三步:编辑与项目同名的文件夹的配置文件&…
CUDA Samples: green ball
以下CUDA sample是分别用C和CUDA实现的生成的绿色的球图像,并对其中使用到的CUDA函数进行了解说,code参考了《GPU高性能编程CUDA实战》一书的第五章,各个文件内容如下:funset.cpp:#include "funset.hpp" #include <r…