C++11中Lambda表达式的使用
Lambda表达式语法:[capture ] ( params ) mutable exception attribute -> return-type { body }
其中capture为定义外部变量是否可见(捕获),若为空,则表示不捕获所有外部变量,即所有外部变量均不可访问,= 表示所有外部变量均以值的形式捕获,在body中访问外部变量时,访问的是外部变量的一个副本,类似函数的值传递,因此在body中对外部变量的修改均不影响外部变量原来的值。& 表示以引用的形式捕获,后面加上需要捕获的变量名,没有变量名,则表示以引用形式捕获所有变量,类似函数的引用传递,body操作的是外部变量的引用,因此body中修改外部变量的值会影响原来的值。params就是函数的形参,和普通函数类似,不过若没有形参,这个部分可以省略。mutalbe表示运行body修改通过拷贝捕获的参数,exception声明可能抛出的异常,attribute修饰符,return-type表示返回类型,如果能够根据返回语句自动推导,则可以省略,body即函数体。除了capture和body是必需的,其他均可以省略。
Lambda表达式是用于创建匿名函数的。Lambda 表达式使用一对方括号作为开始的标识,类似于声明一个函数,只不过这个函数没有名字,也就是一个匿名函数。Lambda 表达式的返回值类型是语言自动推断的。如果不想让Lambda表达式自动推断类型,或者是Lambda表达式的内容很复杂,不能自动推断,则这时必须显示指定Lambda表达式返回值类型,通过”->”。
引入Lambda表达式的前导符是一对方括号,称为Lambda引入符(lambda-introducer):
(1)、[] // 不捕获任何外部变量
(2)、[=] //以值的形式捕获所有外部变量
(3)、[&] //以引用形式捕获所有外部变量
(4)、[x, &y] // x 以传值形式捕获,y 以引用形式捕获
(5)、[=, &z] // z 以引用形式捕获,其余变量以传值形式捕获
(6)、[&,x] // x 以值的形式捕获,其余变量以引用形式捕获
(7)、对于[=]或[&]的形式,lambda 表达式可以直接使用 this 指针。但是,对于[]的形式,如果要使用 this 指针,必须显式传入,如:[this]() { this->someFunc(); }();
Lambda functions: Constructs a closure, an unnamed function object capable of capturing variables in scope.
In C++11, a lambda expression----often called a lambda----is a convenient way of defining an anonymous function object right at the location where it is invoked or passed as an argument to a function. Typically lambdas are used to encapsulate a few lines of code that are passed to algorithms or asynchronous methods.
A lambda function is a function that you can write inline in your source code (usually to pass in to another function, similar to the idea of a functor or function pointer). With lambda, creating quick functions has become much easier, and this means that not only can you start using lambda when you'd previously have needed to write a separate named function, but you can start writing more code that relies on the ability to create quick-and-easy functions.
下面是从其他文章中copy的测试代码,详细内容介绍可以参考对应的reference:
#include "Lambda.hpp"
#include <iostream>
#include <algorithm>
#include <functional>
#include <vector>
#include <string>///
// reference: http://en.cppreference.com/w/cpp/language/lambda
int test_lambda1()
{/*[] Capture nothing (or, a scorched earth strategy?)[&] Capture any referenced variable by reference[=] Capture any referenced variable by making a copy[=, &foo] Capture any referenced variable by making a copy, but capture variable foo by reference[bar] Capture bar by making a copy; don't copy anything else[this] Capture the this pointer of the enclosing class*/int a = 1, b = 1, c = 1;auto m1 = [a, &b, &c]() mutable {auto m2 = [a, b, &c]() mutable {std::cout << a << b << c << '\n';a = 4; b = 4; c = 4;};a = 3; b = 3; c = 3;m2();};a = 2; b = 2; c = 2;m1(); // calls m2() and prints 123std::cout << a << b << c << '\n'; // prints 234return 0;
}///
// reference: http://en.cppreference.com/w/cpp/language/lambda
int test_lambda2()
{std::vector<int> c = { 1, 2, 3, 4, 5, 6, 7 };int x = 5;c.erase(std::remove_if(c.begin(), c.end(), [x](int n) { return n < x; }), c.end());std::cout << "c: ";std::for_each(c.begin(), c.end(), [](int i){ std::cout << i << ' '; });std::cout << '\n';// the type of a closure cannot be named, but can be inferred with autoauto func1 = [](int i) { return i + 4; };std::cout << "func1: " << func1(6) << '\n';// like all callable objects, closures can be captured in std::function// (this may incur unnecessary overhead)std::function<int(int)> func2 = [](int i) { return i + 4; };std::cout << "func2: " << func2(6) << '\n';return 0;
}///
// reference: https://msdn.microsoft.com/zh-cn/library/dd293608.aspx
int test_lambda3()
{// The following example contains a lambda expression that explicitly captures the variable n by value// and implicitly captures the variable m by reference:int m = 0;int n = 0;[&, n](int a) mutable { m = ++n + a; }(4);// Because the variable n is captured by value, its value remains 0 after the call to the lambda expression.// The mutable specification allows n to be modified within the lambda.std::cout << m << std::endl << n << std::endl;return 0;
}//
// reference: https://msdn.microsoft.com/zh-cn/library/dd293608.aspx
template <typename C>
void print(const std::string& s, const C& c)
{std::cout << s;for (const auto& e : c) {std::cout << e << " ";}std::cout << std::endl;
}void fillVector(std::vector<int>& v)
{// A local static variable.static int nextValue = 1;// The lambda expression that appears in the following call to// the generate function modifies and uses the local static // variable nextValue.generate(v.begin(), v.end(), [] { return nextValue++; });//WARNING: this is not thread-safe and is shown for illustration only
}int test_lambda4()
{// The number of elements in the vector.const int elementCount = 9;// Create a vector object with each element set to 1.std::vector<int> v(elementCount, 1);// These variables hold the previous two elements of the vector.int x = 1;int y = 1;// Sets each element in the vector to the sum of the // previous two elements.generate_n(v.begin() + 2,elementCount - 2,[=]() mutable throw() -> int { // lambda is the 3rd parameter// Generate current value.int n = x + y;// Update previous two values.x = y;y = n;return n;});print("vector v after call to generate_n() with lambda: ", v);// Print the local variables x and y.// The values of x and y hold their initial values because // they are captured by value.std::cout << "x: " << x << " y: " << y << std::endl;// Fill the vector with a sequence of numbersfillVector(v);print("vector v after 1st call to fillVector(): ", v);// Fill the vector with the next sequence of numbersfillVector(v);print("vector v after 2nd call to fillVector(): ", v);return 0;
}/
// reference: http://blogorama.nerdworks.in/somenotesonc11lambdafunctions/template<typename T>
std::function<T()> makeAccumulator(T& val, T by) {return [=, &val]() {return (val += by);};
}int test_lambda5()
{int val = 10;auto add5 = makeAccumulator(val, 5);std::cout << add5() << std::endl;std::cout << add5() << std::endl;std::cout << add5() << std::endl;std::cout << std::endl;val = 100;auto add10 = makeAccumulator(val, 10);std::cout << add10() << std::endl;std::cout << add10() << std::endl;std::cout << add10() << std::endl;return 0;
}// reference: http://blogorama.nerdworks.in/somenotesonc11lambdafunctions/
class Foo_lambda {
public:Foo_lambda() {std::cout << "Foo_lambda::Foo_lambda()" << std::endl;}Foo_lambda(const Foo_lambda& f) {std::cout << "Foo_lambda::Foo_lambda(const Foo_lambda&)" << std::endl;}~Foo_lambda() {std::cout << "Foo_lambda~Foo_lambda()" << std::endl;}
};int test_lambda6()
{Foo_lambda f;auto fn = [f]() { std::cout << "lambda" << std::endl; };std::cout << "Quitting." << std::endl;return 0;
}
GitHub:https://github.com/fengbingchun/Messy_Test
相关文章:
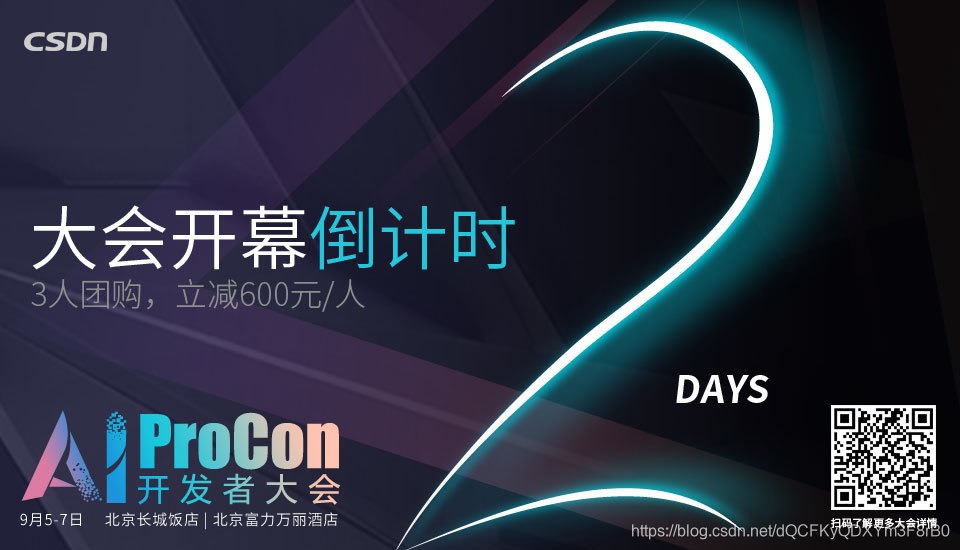
倒计时2天 | 专属技术人的盛会,为你而来!
5G 元年,人工智能 60 年,全球AI市场正发生着巨大的变化,顶尖科技企业和创新力量不断地进行着技术的更迭和应用的推进,专属于 AI 开发者的技术盛宴——2019 AI开发者大会(AI ProCon)将于 2 天后(…
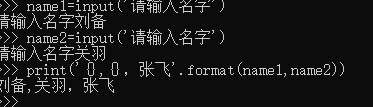
了解大数据的特点、来源与数据呈现方式
作业来源:https://edu.cnblogs.com/campus/gzcc/GZCC-16SE1/homework/2639 浏览2019春节各种大数据分析报告,例如: 这世间,再无第二个国家有能力承载如此庞大的人流量。http://www.sohu.com/a/290025769_313993春节人口迁徙大数据…
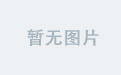
Mysql使用大全 从基础到存储过程
平常习惯了phpmyadmin等其他工具的的朋友有的根本就不会命令,如果让你笔试去面试我看你怎么办,所以,学习一下还是非常有用的,也可以知道你通过GUI工具的时候工具到底做了什么。Mysql用处很广,是php最佳拍档,…
GDAL库简介以及在Windows下编译过程
GDAL(Geospatial Data Abstraction Library,地理空间数据抽象库)是一个在X/MIT许可协议下的开源栅格空间数据转换库。官网http://www.gdal.org/index.html,也可参考GitHub https://github.com/OSGeo/gdal,最新release版本为2.1.1. GDAL是一个…
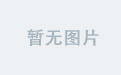
Hexo博客NexT主题美化之评论系统
前言 更多效果展示,请访问我的 个人博客。 效果图: Valine 诞生于2017年8月7日,是一款基于Leancloud的快速、简洁且高效的无后端评论系统。 教程: 登录 Leancloud 官网,注册之后创建一个应用,选择【设置】-…
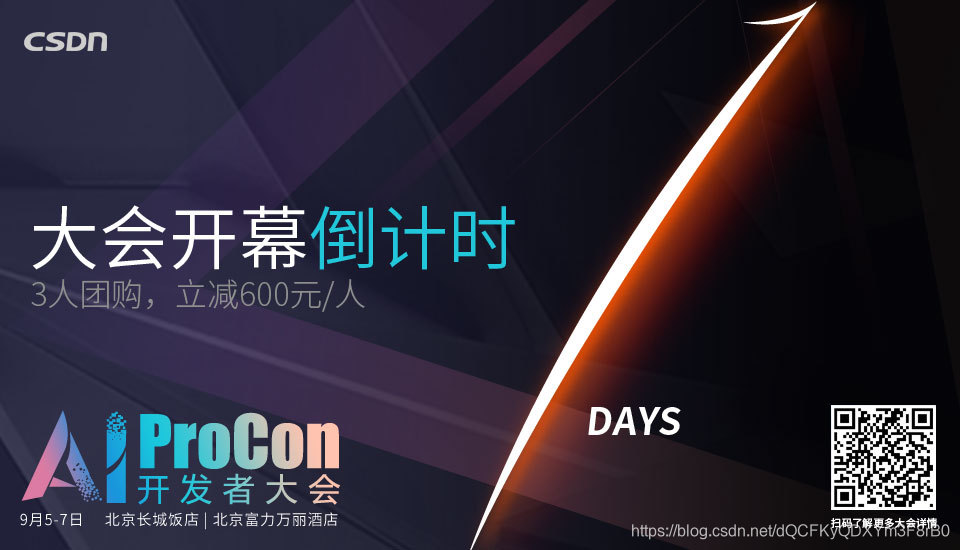
倒计时1天 | 专属技术人的盛会,为你而来!
5G 元年,人工智能 60 年,全球AI市场正发生着巨大的变化,顶尖科技企业和创新力量不断地进行着技术的更迭和应用的推进,专属于 AI 开发者的技术盛宴——2019 AI开发者大会(AI ProCon)将于 明天(9 …

Selenium 2 WebDriver 多线程 并发
我用的是Selenium2,至于它的背景和历史就不赘述了。Selenium2也叫WebDriver。下面讲个例子,用WebDriverjava来写个自动化测试的程序。(如果能用firefox去测试的话,我就直接用Selenium IDE录脚本了。。。)有个前提&…
GDAL2.1.1库在Ubuntu14.04下编译时遇到的问题处理方法
不用作任何调整,直接在Linux下编译GDAL2.1.1源码的步骤是:$ ./configure $ make $ make install非常简单, 这样也能正常生成gdal动态库、静态库,如果想将生成的文件放到指定的目录,则需改第一条命令为:$ ./…
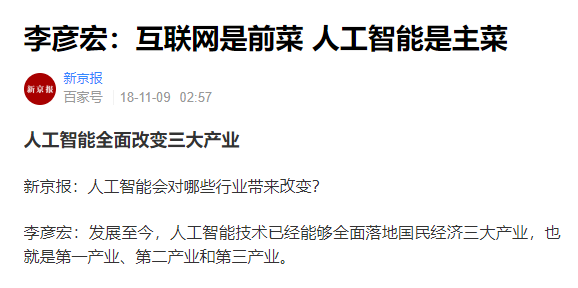
刷爆了!这项技术BAT力捧!程序员:我彻底慌了...
人工智能离我们还遥远吗?近日,海底捞斥资1.5亿打造了中国首家火锅无人餐厅;阿里酝酿了两年之久的全球首家无人酒店也正式开始运营,百度无人车彻底量产。李彦宏称,这是中国第一款能够量产的无人驾驶乘用车。而阿里的这家…
redux的compose源码,中文注释
用图片会更清楚一点,注释和代码会分的清楚源码解析参考请参考https://segmentfault.com/a/11...
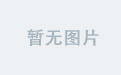
做好职业规划:做自己的船长
要想在职场上有所斩获,就必须做好职业规划。对于职场中人来说,职业规划是职业发展中最关键的向导。职业规划因人而异,不同的对象有不同的需求,因此制定的目标与计划也不尽相同,但个人为自己做职业规划的方法和流程是大…
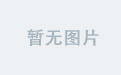
GDAL中GDALDataset::RasterIO分块读取的实现
GDALDataset类中的RasterIO函数能够对图像任意指定区域、任意波段的数据按指定数据类型、指定排列方式读入内存和写入文件中,因此可以实现对大影像的分块读、写运算操作。针对特大的影像图像,有时为了减少内存消耗,对图像进行分块读取很有必要…
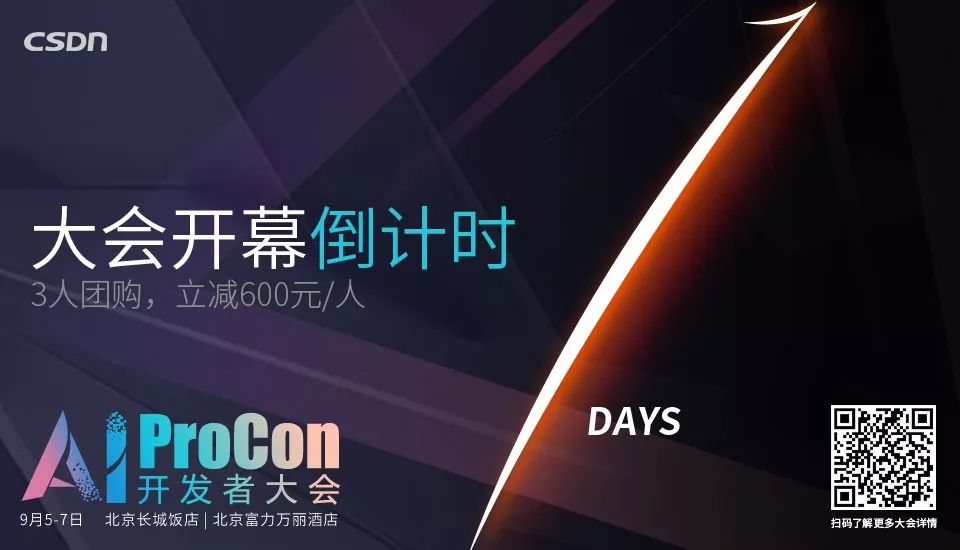
掌握深度学习,为什么要用PyTorch、TensorFlow框架?
作者 | Martin Heller译者 | 弯月责编 | 屠敏来源 | CSDN(ID:CSDNnews)【导读】如果你需要深度学习模型,那么 PyTorch 和 TensorFlow 都是不错的选择。并非每个回归或分类问题都需要通过深度学习来解决。甚至可以说,并…
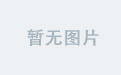
ICANN敦促业界使用DNSSEC,应对DNS劫持攻击
HTTPS加密 可以有效帮助服务器应对DNS欺骗、DNS劫持、ARP攻击等安全威胁。DNS是什么?DNS如何被利用?HTTPS如何防止DNS欺骗? DNS如何工作? 如果您想访问www.example.com,您的浏览器需要找到该特定Web服务器的IP地址。它…

Lucene.net: the main concepts
2019独角兽企业重金招聘Python工程师标准>>> In the previous post you learnt how to get a copy of Lucene.net and where to go in order to look for more information. As you noticed the documentation is far from being complete and easy to read. So in …
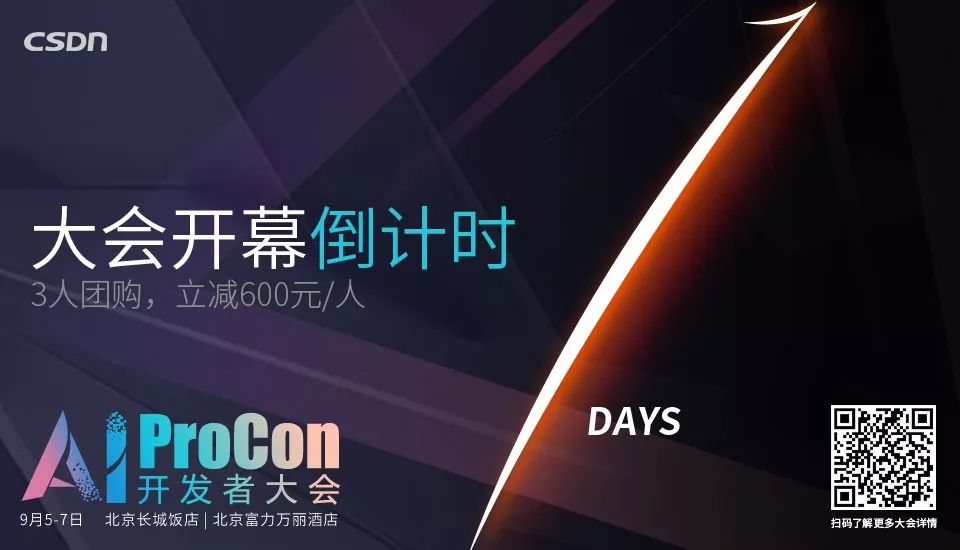
einsum,一个函数走天下
作者 | 永远在你身后转载自知乎【导读】einsum 全称 Einstein summation convention(爱因斯坦求和约定),又称为爱因斯坦标记法,是爱因斯坦 1916 年提出的一种标记约定,本文主要介绍了einsum 的应用。简单的说ÿ…
常用排序算法的C++实现
排序是将一组”无序”的记录序列调整为”有序”的记录序列。假定在待排序的记录序列中,存在多个具有相同的关键字的记录,若经过排序,这些记录的相对次序保持不变,即在原序列中,rirj,且ri在rj之前࿰…

4.65FTP服务4.66测试登录FTP
2019独角兽企业重金招聘Python工程师标准>>> FTP服务 测试登录FTP 4.65FTP服务 文件传输协议(FTP),可以上传和下载文件。比如我们可以把Windows上的文件shan上传到Linux,也可以把Linux上的文件下载到Windows上。 Cent…
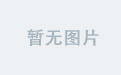
JavaScript的应用
DOM, BOM, XMLHttpRequest, Framework, Tool (Functionality) Performance (Caching, Combine, Minify, JSLint) ---------------- 人工做不了,交给程序去做,这样可以流程化。 Maintainability (Pattern) http://www.jmarshall.com/easy/http/ http://dj…
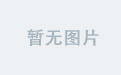
miniz库简介及使用
miniz:Google开源库,它是单一的C源文件,紧缩/膨胀压缩库,使用zlib兼容API,ZIP归档读写,PNG写方式。关于miniz的更详细介绍可以参考:https://code.google.com/archive/p/miniz/miniz.c is a loss…
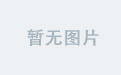
iOS之runtime详解api(三)
第一篇我们讲了关于Class和Category的api,第二篇讲了关于Method的api,这一篇来讲关于Ivar和Property。 4.objc_ivar or Ivar 首先,我们还是先找到能打印出Ivar信息的函数: const char * _Nullable ivar_getName(Ivar _Nonnull v) …
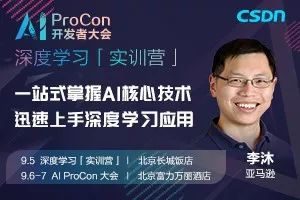
亚马逊首席科学家李沐「实训营」国内独家直播,马上报名 !
开学了,别人家的学校都开始人工智能专业的学习之旅了,你呢?近年来,国内外顶尖科技企业的 AI 人才抢夺战愈演愈烈。华为开出200万年薪吸引 AI 人才,今年又有 35 所高校新增人工智能本科专业,众多新生即将开展…
人脸检测库libfacedetection介绍
libfacedetection是于仕琪老师放到GitHub上的二进制库,没有源码,它的License是MIT,可以商用。目前只提供了windows 32和64位的release动态库,主页为https://github.com/ShiqiYu/libfacedetection,采用的算法好像是Mult…
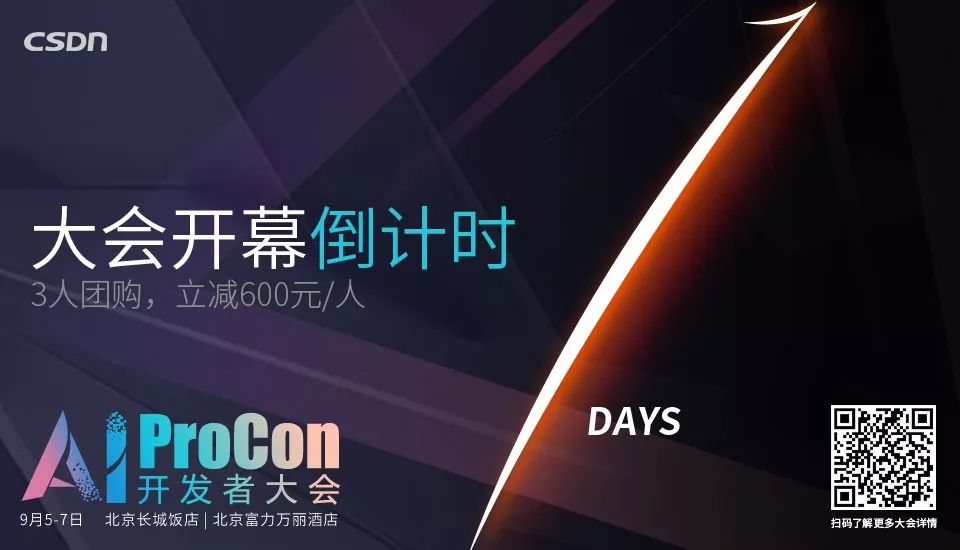
倒计时1天 | 2019 AI ProCon报名通道即将关闭(附参会指南)
2019年9月5-7日,面向AI技术人的年度盛会—— 2019 AI开发者大会 AI ProCon,震撼来袭!2018 年由 CSDN 成功举办 AI 开发者大会一年之后,全球 AI 市场正发生着巨大的变化。顶尖科技企业和创新力量不断地进行着技术的更迭和应用的推…
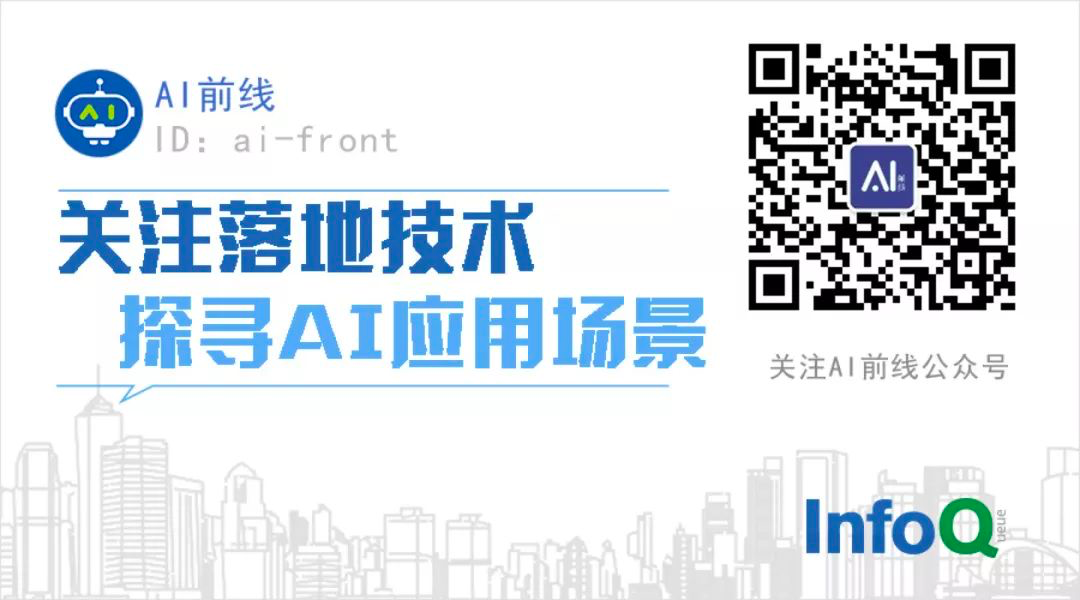
法院判决:优步无罪,无人车安全员可能面临过失杀人控诉
据路透社报道,负责优步无人车在亚利桑那州致人死亡事件调查的律师事务所发布公开信宣布,优步在事故中“不承担刑事责任”,但是当时在车上的安全员Rafaela Vasquez要接受进一步调查,可能面临车辆过失杀人罪指控。2018年3月…
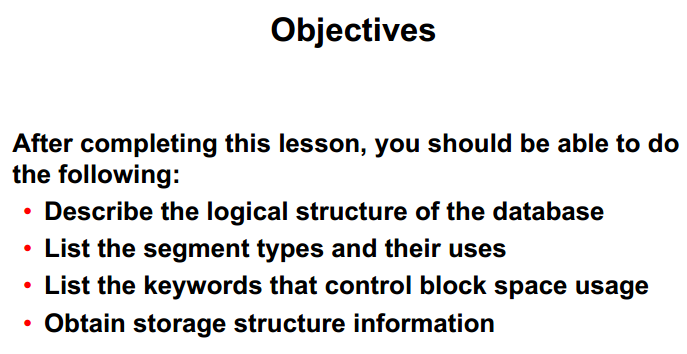
09 Storage Structure and Relationships
目标:存储结构:Segments分类:Extents介绍:Blocks介绍:转载于:https://blog.51cto.com/eread/1333894
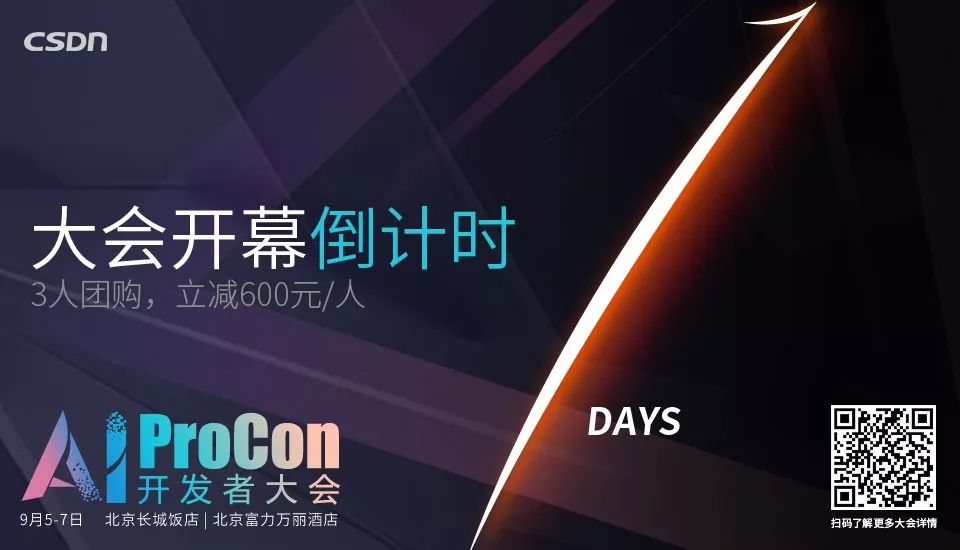
边界框的回归策略搞不懂?算法太多分不清?看这篇就够了
作者 | fivetrees来源 | https://zhuanlan.zhihu.com/p/76477248本文已由作者授权,未经允许,不得二次转载【导读】目标检测包括目标分类和目标定位 2 个任务,目标定位一般是用一个矩形的边界框来框出物体所在的位置,关于边界框的回…
人脸识别引擎SeetaFaceEngine简介及在windows7 vs2013下的编译
SeetaFaceEngine是开源的C人脸识别引擎,无需第三方库,它是由中科院计算所山世光老师团队研发。它的License是BSD-2.SeetaFaceEngine库包括三个模块:人脸检测(detection)、面部特征点定位(alignment)、人脸特征提取与比对(identification)。人…

当移动数据分析需求遇到Quick BI
我叫洞幺,是一名大型婚恋网站“我在这等你”的资深老员工,虽然在公司五六年,还在一线搬砖。“我在这等你”成立15年,目前积累注册用户高达2亿多,在我们网站成功牵手的用户达2千多万。目前我们的公司在CEO的英名带领下&…