高德地图关键字搜索oc版
.h文件
// MapSearchViewController.h
// JMT
//
// Created by walker on 16/10/11.
// Copyright © 2016年 BOOTCAMP. All rights reserved.
//
#import <UIKit/UIKit.h>
#import <AMapNaviKit/MaMapKit.h>
#import <AMapSearchKit/AMapSearchKit.h>
typedef void(^moveBlock)(AMapPOI *location);
@interface MapSearchViewController : UIViewController
@property (nonatomic,retain) MAUserLocation *currentLocation;//当前位置
@property (nonatomic,retain) NSString *currentCity;//当前参数
@property (nonatomic,copy) moveBlock moveBlock;
@property (nonatomic,retain) NSString *searchStr;//搜索的内容
@property (nonatomic,strong) NSString *searchName;//点击cell时,cell的内容,利用代理传值,传回上一界面
@end
.m
//常量
#define kWidth [UIScreen mainScreen].bounds.size.width
#define kHeight [UIScreen mainScreen].bounds.size.height
#import "MapSearchViewController.h"
#import <AMapSearchKit/AMapSearchKit.h>
//#import "MapViewController.h"
@interface MapSearchViewController ()<UISearchBarDelegate,UISearchResultsUpdating,UITableViewDataSource,UITableViewDelegate,AMapSearchDelegate,UITextFieldDelegate>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (nonatomic, strong) UISearchController *searchController;
@property (nonatomic,retain) NSMutableArray *dataList;
@property (strong,nonatomic) NSMutableArray *searchList;
@property (nonatomic,retain) AMapSearchAPI *search;
@property (weak, nonatomic) IBOutlet UITextField *searchTextField;//搜索的文本输入框
@property (nonatomic,assign) BOOL isSelected;//是否点击了搜索,点击之前都是只能匹配
@end
@implementation MapSearchViewController
- (NSMutableArray *)searchList
{
if (!_searchList) {
_searchList = [NSMutableArray array];
}
return _searchList;
}
- (NSMutableArray *)dataList
{
if (!_dataList) {
_dataList = [NSMutableArray array];
}
return _dataList;
}
- (void)viewDidLoad {
[super viewDidLoad];
_search = [[AMapSearchAPI alloc] init];
_search.delegate = self;
//添加到顶部
_searchController = [[UISearchController alloc] initWithSearchResultsController:nil];
_searchController.searchResultsUpdater = self;
_searchController.searchBar.delegate = self;
// _searchController.delegate = self;
_searchController.dimsBackgroundDuringPresentation = NO;
_searchController.hidesNavigationBarDuringPresentation = NO;
_searchController.searchBar.frame = CGRectMake(kWidth/2 - 100, 20, 200, 44.0);
self.navigationItem.titleView = self.searchController.searchBar;
self.searchController.searchBar.text = _searchStr;
[_searchController.searchBar becomeFirstResponder];
self.navigationItem.leftBarButtonItem = [[UIBarButtonItem alloc]initWithTitle:@"返回" style:UIBarButtonItemStylePlain target:self action:@selector(backToMapVC)];
[self.tableView registerClass:[UITableViewCell class] forCellReuseIdentifier:@"CELL"];
_currentCity = @"河南省";
NSLog(@"搜索界面%@,%@",_currentLocation,_currentCity);
}
- (void)viewWillAppear:(BOOL)animated
{
[super viewWillAppear:animated];
[_searchController.searchBar becomeFirstResponder];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return _searchList.count;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
//设置区域的行数(重点),这个就是使用委托之后需要需要判断是一下是否是需要使用Search之后的视图:
if (!_isSelected) {
return [self.searchList count];
}else{
return [self.dataList count];
}
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *flag=@"CELL";
UITableViewCell *cell=[tableView dequeueReusableCellWithIdentifier:flag];
if (cell==nil) {
cell=[[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:flag];
}
//如果搜索框激活
if (!_isSelected) {
AMapPOI *poi = _searchList[indexPath.row];
[cell.textLabel setText:poi.name];
}
else{
AMapPOI *poi = _dataList[indexPath.row];
[cell.textLabel setText:poi.name];
}
return cell;
}
//点击cell
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[self.searchController.searchBar resignFirstResponder];
AMapPOI *poi = [[AMapPOI alloc]init];
//如果搜索框激活
if (!_isSelected) {
poi = _searchList[indexPath.row];
}
else{
poi = _dataList[indexPath.row];
}
NSLog(@"%@,%f,%f",poi.name,poi.location.latitude,poi.location.longitude);
_searchName = poi.name;
NSLog(@"_searchName%@",_searchName);
NSLog(@"poi.name%@",poi.name);
// self.moveBlock(poi);
//向上以页面传值并返回..
相关文章:
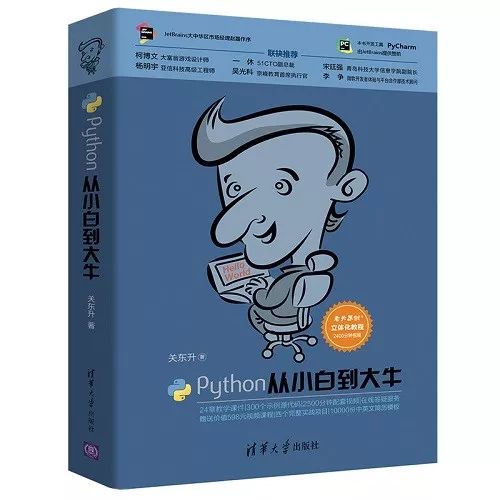
同一个内容,对比Java、C、PHP、Python的代码量,结局意外了
为什么都说Python容易上手!是真的吗?都说Python通俗易懂,容易上手,甚至不少网友表示「完成同一个任务,C 语言要写 1000 行代码,Java 只需要写 100 行,而 Python 可能只要 20 行」到底是真的还是…
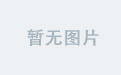
图片存储思考:
http://blog.csdn.net/liuruhong/article/details/4072386
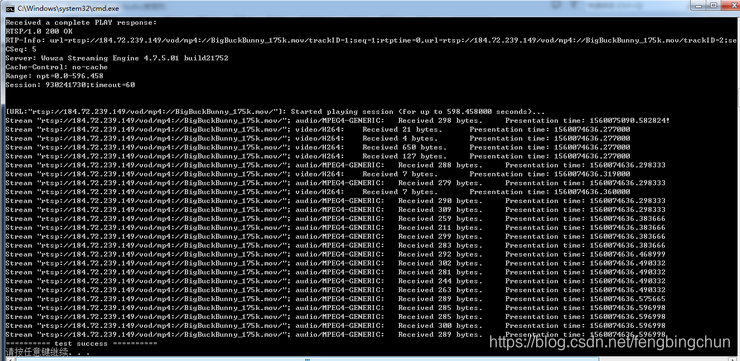
LIVE555中RTSP客户端接收媒体流分析及测试代码
LIVE555中testProgs目录下的testRTSPClient.cpp代码用于测试接收RTSP URL指定的媒体流,向服务器端发送的命令包括:DESCRIBE、SETUP、PLAY、TERADOWN。 1. 设置使用环境:new一个BasicTaskScheduler对象;new一个BasicUsageEnvironm…
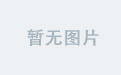
swift代理传值
比如我们这个场景,B要给A传值,那B就拥有代理属性, A就是B的代理,很简单吧!有代理那就离不开协议,所以第一步就是声明协议。在那里声明了?谁拥有代理属性就在那里声明,所以代码就是这…
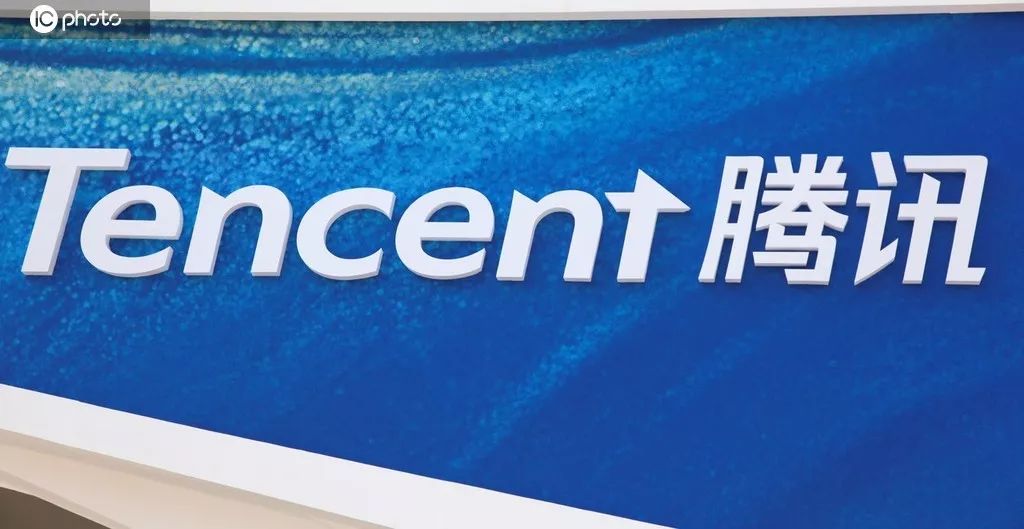
重磅:腾讯正式开源图计算框架Plato,十亿级节点图计算进入分钟级时代
整理 | 唐小引 来源 | CSDN(ID:CSDNnews)腾讯开源进化 8 年,进入爆发期。 继刚刚连续开源 TubeMQ、Tencent Kona JDK、TBase、TKEStack 四款重点开源项目后,腾讯开源再次迎来重磅项目!北京时间 11 月 14 日…

类似ngnix的多进程监听用例
2019独角兽企业重金招聘Python工程师标准>>> 多进程监听适合于短连接,且连接间无交集的应用。前两天简单写了一个,在这里保存一下。 #include <sys/types.h>#include <stdarg.h>#include <signal.h>#include <unistd.h&…
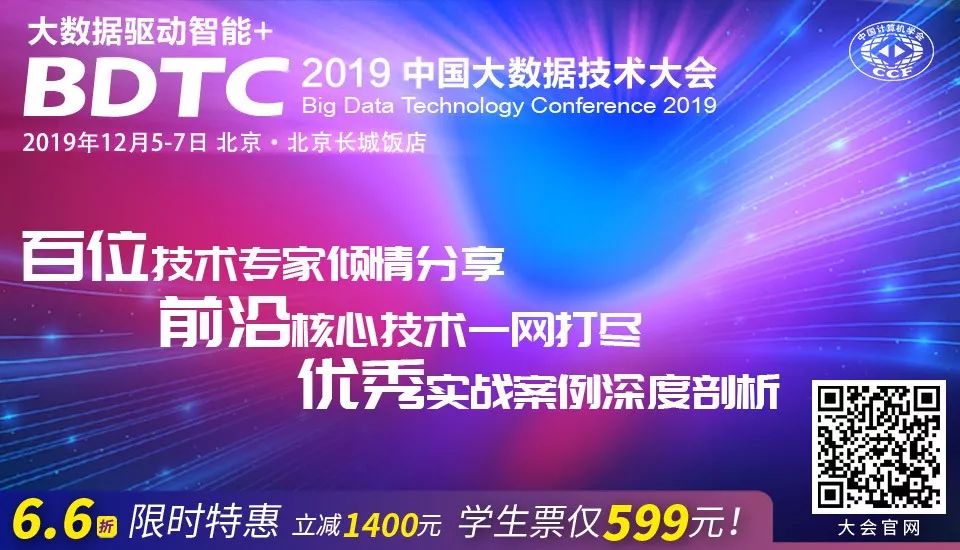
今日头条李磊等最新论文:用于文本生成的核化贝叶斯Softmax
译者 | Raku 出品 | AI科技大本营(ID:rgznai100)摘要用于文本生成的神经模型需要在解码阶段具有适当词嵌入的softmax层,大多数现有方法采用每个单词单点嵌入的方式,但是一个单词可能具有多种意义,在不同的背景下&#…
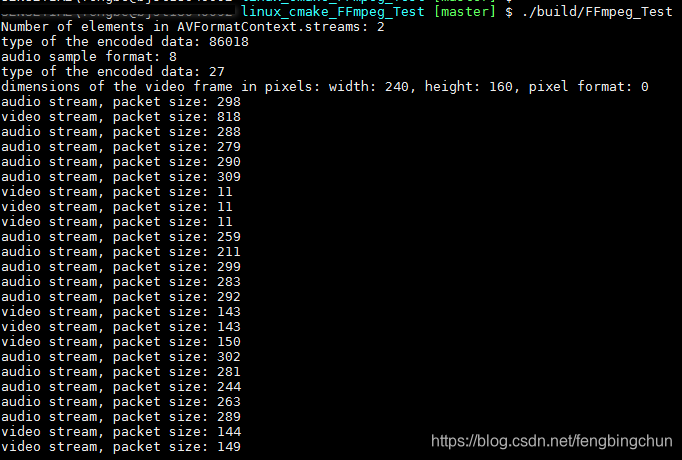
FFmpeg中RTSP客户端拉流测试代码
之前在https://blog.csdn.net/fengbingchun/article/details/91355410中给出了通过LIVE555实现拉流的测试代码,这里通过FFmpeg来实现,代码量远小于LIVE555,实现模块在libavformat。 在4.0及以上版本中,FFmpeg有了些变动ÿ…

虚拟机下运行linux通过nat模式与主机通信、与外网连接
首先:打开虚拟机的编辑菜单下的虚拟网络编辑器,选中VMnet8 NAT模式。通过NAT设置获取网关IP,通过DHCP获取可配置的IP区间。同时,将虚拟机的虚拟机菜单的设置选项中的网络适配器改为NAT模式。即可! 打开linux࿰…
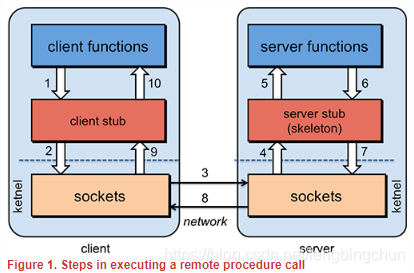
远程过程调用RPC简介
RPC(Remote Procedure Call, 远程过程调用):是一种通过网络从远程计算机程序上请求服务,而不需要了解底层网络技术的思想。 RPC是一种技术思想而非一种规范或协议,常见RPC技术和框架有: (1). 应用级的服务框架:阿里的…
iOS开发:沙盒机制以及利用沙盒存储字符串、数组、字典等数据
iOS开发:沙盒机制以及利用沙盒存储字符串、数组、字典等数据 1、初识沙盒:(1)、存储在内存中的数据,程序关闭,内存释放,数据就会丢失,这种数据是临时的。要想数据永久保存,将数据保存成文件&am…
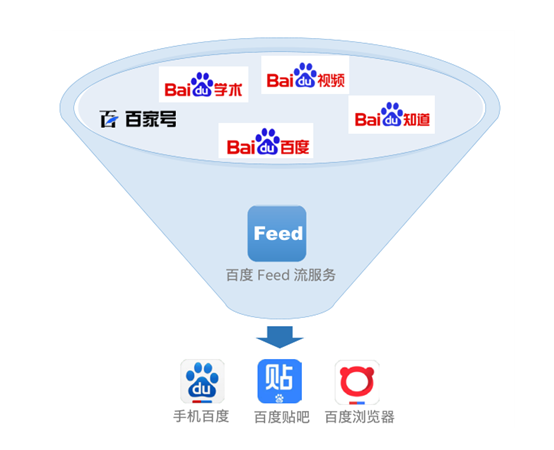
支撑亿级用户“刷手机”,百度Feed流背后的新技术装备有多牛?
导读:截止到2018年底,我国网民使用手机上网的比例已高达98.6%,移动互联网基本全方位覆盖。智能手机的操作模式让我们更倾向于通过简单的“划屏”动作,相对于传统的文本交互方式来获取信息,用户更希望一拿起手机就能刷到…

玩转高性能超猛防火墙nf-HiPAC
中华国学,用英文讲的,稀里糊涂听了个大概,不得不佩服西方人的缜密的逻辑思维,竟然把玄之又玄的道家思想说的跟牛顿定律一般,佩服。归家,又收到了邮件,还是关于nf-hipac的,不知不觉就…

ios 沙盒 plist 数据的读取和存储
plist 只能存储基本的数据类型 和 array 字典 [objc] view plaincopy - (void)saveArray { // 1.获得沙盒根路径 NSString *home NSHomeDirectory(); // 2.document路径 NSString *docPath [home stringByAppendingPathComponent:"Document…
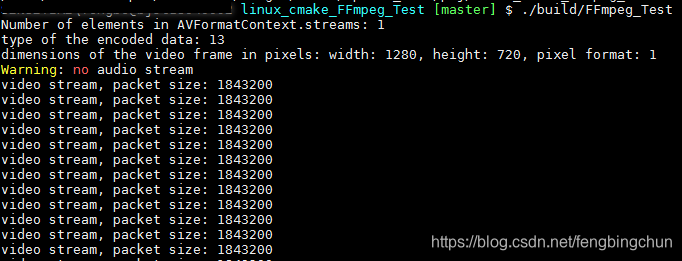
FFmpeg实现获取USB摄像头视频流测试代码
通过USB摄像头(注:windows7/10下使用内置摄像头,linux下接普通的usb摄像头(Logitech))获取视频流用到的模块包括avformat和avdevice。头文件仅include avdevice.h即可,因为avdevice.h中会include avformat.h。libavdevice库是libavformat的一…
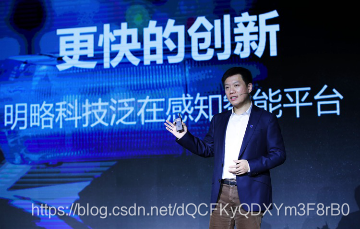
重磅!明略发布数据中台战略和三大解决方案
11月15日,明略科技在上海举办以“FASTER 聚变增长新动力”为主题的2019数据智能峰会,宣布“打造智能时代的企业中台”新战略,同时推出了两大新产品“新一代数据中台”和“营销智能平台”,以及三大行业解决方案,分别是“…
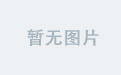
Android程序完全退出的三种方法
1. Dalvik VM的本地方法 android.os.Process.killProcess(android.os.Process.myPid()) //获取PID,目前获取自己的也只有该API,否则从/proc中自己的枚举其他进程吧,不过要说明的是,结束其他进程不一定有权限,不然就…
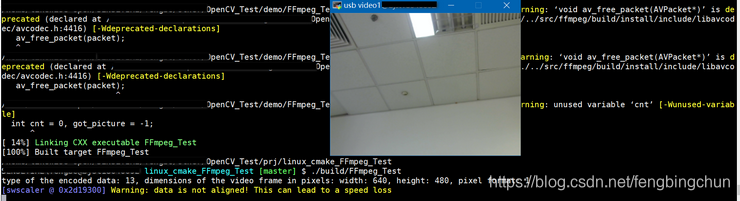
FFmpeg通过摄像头实现对视频流进行解码并显示测试代码(旧接口)
这里通过USB摄像头(注:windows7/10下使用内置摄像头,linux下接普通的usb摄像头(Logitech))获取视频流,然后解码,最后再用opencv显示。用到的模块包括avformat、avcodec和avdevice。libavdevice库是libavformat的一个补充库(comple…

IOS数据存储之文件沙盒存储
前言: 之前学习了数据存储的NSUserDefaults,归档和解档,对于项目开发中如果要存储一些文件,比如图片,音频,视频等文件的时候就需要用到文件存储了。文件沙盒存储主要存储非机密数据,大的数据。 …
剖析Focal Loss损失函数: 消除类别不平衡+挖掘难分样本 | CSDN博文精选
作者 | 图像所浩南哥来源 | CSDN博客论文名称:《 Focal Loss for Dense Object Detection 》论文下载:https://arxiv.org/pdf/1708.02002.pdf论文代码:https://github.com/facebookresearch/Detectron/tree/master/configs/12_2017_baselines…
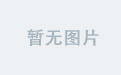
windows下mysql开启慢查询
mysql在windows系统中的配置文件一般是my.ini,我的路径是c:\mysql\my.ini,你根据自己安装mysql路径去查找[mysqld]#The TCP/IP Port the MySQL Server will listen onport3306#开启慢查询log-slow-queries E:\Program Files\MySQL\MySQL Server 5.5\mysql_slow_query.loglong_…
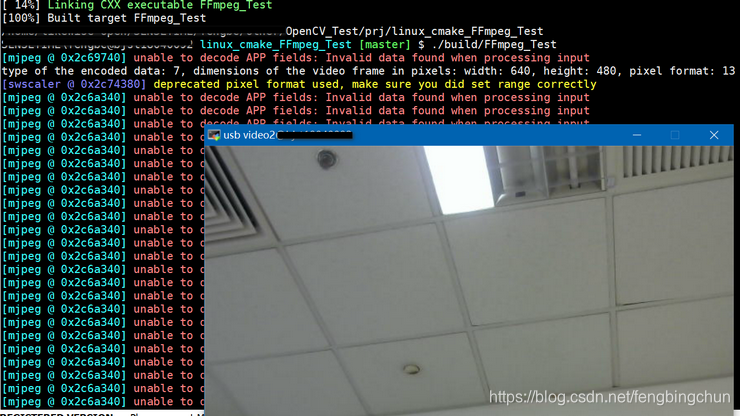
FFmpeg通过摄像头实现对视频流进行解码并显示测试代码(新接口)
在https://blog.csdn.net/fengbingchun/article/details/93975325 中给出了通过旧接口即FFmpeg中已废弃的接口实现通过摄像头获取视频流然后解码并显示的测试代码,这里通过使用FFmpeg中的新接口再次实现通过的功能,主要涉及到的接口函数包括:…
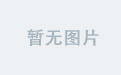
iOS经典讲解之获取沙盒文件路径写入和读取简单对象
#import "RootViewController.h" interface RootViewController () end 实现文件: implementation RootViewController - (void)viewDidLoad { [super viewDidLoad]; [self path]; [self writeFile]; [self readingFi…
Google最新论文:Youtube视频推荐如何做多目标排序
作者 | 深度传送门来源 | 深度传送门(ID:deep_deliver)导读:本文是“深度推荐系统”专栏的第十五篇文章,这个系列将介绍在深度学习的强力驱动下,给推荐系统工业界所带来的最前沿的变化。本文主要介绍下Google在RecSys …
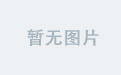
Jmeter 笔记
Apache JMeter是Apache组织开发的基于Java的压力测试工具。用于对软件做压力测试,它最初被设计用于Web应用测试但后来扩展到其他测试领域。 它可以用于测试静态和动态资源例如静态文件、Java 小服务程序、CGI 脚本、Java 对象、数据库, FTP 服务器&#…
王贻芳院士:为什么中国要探究中微子实验?
演讲嘉宾 | 王贻芳(中国科学院院士、中科院高能物理研究所所长)整理 | 德状出品 | AI科技大本营(ID:rgznai100)日前,在2019腾讯科学WE大会期间,中国科学院院士、高能物理研究所所长王贻芳分享了中微子与光电…
一个苹果证书供多台电脑开发使用——导出p12文件
摘要 在苹果开发者网站申请的证书,是授权mac设备的开发或者发布的证书,这意味着一个设备对应一个证书,但是99美元账号只允许生成3个发布证书,两个开发证书,这满足不了多mac设备的使用,使用p12文件可以解决这…
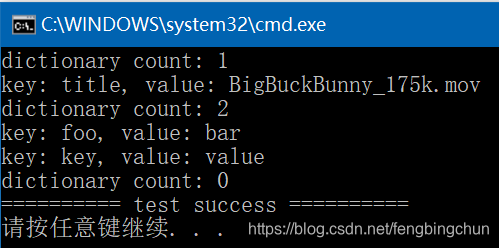
FFmpeg中AVDictionary介绍
FFmpeg中的AVDictionary是一个结构体,简单的key/value存储,经常使用AVDictionary设置或读取内部参数,声明如下,具体实现在libavutil模块中的dict.c/h,提供此结构体是为了与libav兼容,但它实现效率低下&…
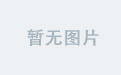
RocketMQ3.2.2生产者发送消息自动创建Topic队列数无法超过4个
问题现象RocketMQ3.2.2版本,测试时尝试发送消息时自动创建Topic,设置了队列数量为8:producer.setDefaultTopicQueueNums(8);同时设置broker服务器的配置文件broker.properties:defaultTopicQueueNums16但实际创建后从控制台及后台…
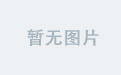
iOS各种宏定义
#ifndef MacroDefinition_h #define MacroDefinition_h //************************ 获取设备屏幕尺寸********************************************** //宽度 #define SCREEN_WIDTH [UIScreen mainScreen].bounds.size.width //高度 #define SCREENH_HEIGHT [UIScree…