C++11中rvalue references的使用
Rvalue references are a feature of C++ that was added with the C++11 standard. The syntax of an rvalue reference is to add && after a type.
为了支持移动操作,C++11引入了一种新的引用类型----右值引用(rvalue reference)。所谓右值引用就是必须绑定到右值的引用。通过&&而不是&来获得右值引用。右值引用有一个重要的性质----只能绑定到一个将要销毁的对象。因此,可以自由地将一个右值引用的资源”移动”到另一个对象中。
一般而言,一个左值表达式表示的是一个对象的身份,而一个右值表达式表示的是对象的值。
类似任何引用,一个右值引用也不过是某个对象的另一个名字而已。对于常规引用(为了与右值引用区分开来,可以称之为左值引用(lvalue reference)),不能将其绑定到要求转换的表达式、字面常量或是返回右值的表达式。右值引用有着完全相反的绑定特性:可以将一个右值引用绑定到这类表达式上,但不能将一个右值引用直接绑定到一个左值上。
返回左值引用的函数,连同赋值、下标、解引用和前置递增/递减运算符,都是返回左值得表达式的例子。可以将一个左值引用绑定到这类表达式的结果上。
返回非引用类型的函数,连同算术、关系、位以及后置递增/递减运算符,都生成右值。不能将一个左值引用绑定到这类表达式上,但可以将一个const的左值引用或者一个右值引用绑定到这类表达式上。
左值持久,右值短暂:左值有持久的状态,而右值要么是字面常量,要么是在表达式求值过程中创建的临时对象。
由于右值引用只能绑定到临时对象,可知:所引用的对象将要被销毁;该对象没有其它用户。这两个特性意味着,使用右值引用的代码可以自由地接管所引用的对象的资源。
变量是左值:变量可以看作只有一个运算对象而没有运算符的表达式。
变量是左值,因此不能将一个右值引用直接绑定到一个变量上,即使这个变量是右值引用类型也不行。
虽然不能就将一个右值引用直接绑定到一个左值上,但可以显示地将一个左值转换为对应的右值引用类型。还可以通过调用一个名为move的新标准库函数来获得绑定到左值上的右值引用,此函数定义在头文件utility中。move调用告诉编译器:有一个左值,但希望像一个右值一样处理它。在调用move之后,我们不能对移后源对象的值做任何假设。
我们可以销毁一个移后源对象,也可以赋予它新值,但不能使用一个移后源对象的值。
In C++, there are rvalues and lvalues. An lvalue is an expression whose address can be taken,a locator value--essentially, an lvalue provides a (semi)permanent piece of memory. rvalues are not lvalues. An expression is an rvalue if it results in a temporary object.
Every C++ expression is either an lvalue or an rvalue. An lvalue refers to an object that persists beyond a single expression. You can think of an lvalue as an object that has a name. All variables, including nonmodifiable (const) variables, are lvalues. An rvalue is a temporary value that does not persist beyond the expression that uses it.
an "rvalue reference", that will let you bind a mutable reference to an rvalue, but not an lvalue. In other words, rvalue references are perfect for detecting if a value is temporary object or not. Rvalue references use the && syntax instead of just &, and can be const and non-const, just like lvalue references, although you'll rarely see a const rvalue reference.
Rvalue references solve at least two problems: Implementing move semantics; Perfect forwarding.
The original definition of lvalues and rvalues from the earliest days of C is as follows: An lvalue is an expression e that may appear on the left or on the right hand side of an assignment, whereas an rvalue is an expression that can only appear on the right hand side of an assignment.
If X is any type, then X&& is called an rvalue reference to X. For better distinction, the ordinary reference X& is now also called an lvalue reference.
rvalue references enable us to distinguish an lvalue from an rvalue.
In C++11,however, the rvalue reference lets us bind a mutable reference to an rvalue,but not an lvalue. In other words, rvalue references are perfect for detecting whether a value is a temporary object or not.
Important rvalue reference properties:
(1)、For overload resolution, lvalues prefer binding to lvalue references and rvalues prefer binding to rvalue references. Hence why temporaries prefer invoking a move constructor / move assignment operator over a copy constructor / assignment operator.
(2)、rvalue references will implicitly bind to rvalues and to temporaries that are the result of an implicit conversion. i.e. float f = 0f; int&& i = f; is well formed because float is implicitly convertible to int; the reference would be to a temporary that is the result of the conversion.
(3)、Named rvalue references are lvalues. Unnamed rvalue references are rvalues. This is important to understand why the std::move call is necessary in: foo&& r= foo(); foo f = std::move(r).
Rvalue references enable you to distinguish an lvalue from an rvalue. Lvalue references and rvalue references are syntactically and semantically similar,but they follow somewhat different rules.
右值引用是C++11中最重要的新特性之一,它解决了C++中大量的历史遗留问题,使C++标准库的实现在多种场景下消除了不必要的额外开销(如std::vector, std::string),也使得另外一些标准库(如std::unique_ptr, std::function)成为可能。即使你并不直接使用右值引用,也可以通过标准库,间接从这一新特性中受益。
右值引用的意义通常解释为两大作用:移动语义和完美转发。
右值引用可以使我们区分表达式的左值和右值。
右值引用它实现了移动语义(Move Sementics)和完美转发(Perfect Forwarding)。它的主要目的有两个方面:(1)、消除两个对象交互时不必要的对象拷贝,节省运算存储资源,提高效率;(2)、能够更简洁明确地定义泛型函数。
右值引用主要就是解决一个拷贝效率低下的问题,因为针对于右值,或者打算更改的左值,我们可以采用类似与auto_ptr的move(移动)操作,大大的提高性能(move semantics)。另外,C++的模板推断机制为参数T&&做了一个例外规则,让左值和右值的识别和转向(forward)非常简单,帮助我们写出高效并且简捷的泛型代码(perfect forwarding)。
左值的声明符号为”&”, 为了和左值区分,右值的声明符号为”&&”。
下面是从其他文章中copy的测试代码,详细内容介绍可以参考对应的reference:
#include "rvalue_references.hpp"
#include <iostream>
#include <string>
#include <utility>//
// reference: http://en.cppreference.com/w/cpp/language/reference
void double_string(std::string& s)
{s += s; // 's' is the same object as main()'s 'str'
}char& char_number(std::string& s, std::size_t n)
{return s.at(n); // string::at() returns a reference to char
}int test_lvalue_references1()
{// 1. Lvalue references can be used to alias an existing object (optionally with different cv-qualification):std::string s = "Ex";std::string& r1 = s;const std::string& r2 = s;r1 += "ample"; // modifies s// r2 += "!"; // error: cannot modify through reference to conststd::cout << r2 << '\n'; // prints s, which now holds "Example"// 2. They can also be used to implement pass-by-reference semantics in function calls:std::string str = "Test";double_string(str);std::cout << str << '\n';// 3. When a function's return type is lvalue reference, the function call expression becomes an lvalue expressionstd::string str_ = "Test";char_number(str_, 1) = 'a'; // the function call is lvalue, can be assigned tostd::cout << str_ << '\n';return 0;
}//
// reference: http://en.cppreference.com/w/cpp/language/reference
static void f(int& x)
{std::cout << "lvalue reference overload f(" << x << ")\n";
}static void f(const int& x)
{std::cout << "lvalue reference to const overload f(" << x << ")\n";
}static void f(int&& x)
{std::cout << "rvalue reference overload f(" << x << ")\n";
}int test_rvalue_references1()
{// 1. Rvalue references can be used to extend the lifetimes of temporary objects// (note, lvalue references to const can extend the lifetimes of temporary objects too, but they are not modifiable through them):std::string s1 = "Test";// std::string&& r1 = s1; // error: can't bind to lvalueconst std::string& r2 = s1 + s1; // okay: lvalue reference to const extends lifetime// r2 += "Test"; // error: can't modify through reference to conststd::string&& r3 = s1 + s1; // okay: rvalue reference extends lifetimer3 += "Test"; // okay: can modify through reference to non-conststd::cout << r3 << '\n';// 2. More importantly, when a function has both rvalue reference and lvalue reference overloads,// the rvalue reference overload binds to rvalues (including both prvalues and xvalues),// while the lvalue reference overload binds to lvalues:int i = 1;const int ci = 2;f(i); // calls f(int&)f(ci); // calls f(const int&)f(3); // calls f(int&&)// would call f(const int&) if f(int&&) overload wasn't providedf(std::move(i)); // calls f(int&&)// This allows move constructors, move assignment operators, and other move-aware functions// (e.g. vector::push_back() to be automatically selected when suitable.return 0;
}/
// reference: http://www.bogotobogo.com/cplusplus/C11/5_C11_Move_Semantics_Rvalue_Reference.php
static void printReference(int& value)
{std::cout << "lvalue: value = " << value << std::endl;
}static void printReference(int&& value)
{std::cout << "rvalue: value = " << value << std::endl;
}static int getValue()
{int temp_ii = 99;return temp_ii;
}int test_rvalue_references2()
{int ii = 11;printReference(ii);printReference(getValue()); // printReference(99);return 0;
}// references: https://msdn.microsoft.com/en-us/library/dd293668.aspx
template<typename T> struct S;// The following structures specialize S by
// lvalue reference (T&), const lvalue reference (const T&),
// rvalue reference (T&&), and const rvalue reference (const T&&).
// Each structure provides a print method that prints the type of
// the structure and its parameter.
template<typename T> struct S<T&> {static void print(T& t){std::cout << "print<T&>: " << t << std::endl;}
};template<typename T> struct S<const T&> {static void print(const T& t){std::cout << "print<const T&>: " << t << std::endl;}
};template<typename T> struct S<T&&> {static void print(T&& t){std::cout << "print<T&&>: " << t << std::endl;}
};template<typename T> struct S<const T&&> {static void print(const T&& t){std::cout << "print<const T&&>: " << t << std::endl;}
};// This function forwards its parameter to a specialized
// version of the S type.
template <typename T> void print_type_and_value(T&& t)
{S<T&&>::print(std::forward<T>(t));
}// This function returns the constant string "fourth".
const std::string fourth() { return std::string("fourth"); }int test_rvalue_references3()
{// The following call resolves to:// print_type_and_value<string&>(string& && t)// Which collapses to:// print_type_and_value<string&>(string& t)std::string s1("first");print_type_and_value(s1);// The following call resolves to:// print_type_and_value<const string&>(const string& && t)// Which collapses to:// print_type_and_value<const string&>(const string& t)const std::string s2("second");print_type_and_value(s2);// The following call resolves to:// print_type_and_value<string&&>(string&& t)print_type_and_value(std::string("third"));// The following call resolves to:// print_type_and_value<const string&&>(const string&& t)print_type_and_value(fourth());return 0;
}
GitHub: https://github.com/fengbingchun/Messy_Test
相关文章:
AIの幕后人:探秘“硬核英雄”的超级武器
作者 | 云计算的阿晶 出品 | AI科技大本营(ID:rgznai100) 掐指一算八年之前,那时正是国内互联网卯足劲头起飞的一年,各行各业表现都很突出,尤其是与人们生活密切相关的手机,正大踏步地从功能机向智能手机转…
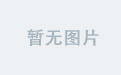
PAT乙级1003
1003 我要通过! (20 point(s))“答案正确”是自动判题系统给出的最令人欢喜的回复。本题属于 PAT 的“答案正确”大派送 —— 只要读入的字符串满足下列条件,系统就输出“答案正确”,否则输出“答案错误”。 得到“答案…
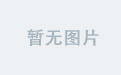
史上最简洁的UITableView Sections 展示包含NSDicionary 的NSArray
这个最典型的就是电话本,然后根据A-Z分组, 当然很多例子,不过现在发现一个很简洁易懂的: 1. 准备数据,定义一个dictionary来显示所有的内容,这个dictionary对应的value全是数组 也就是: A &…
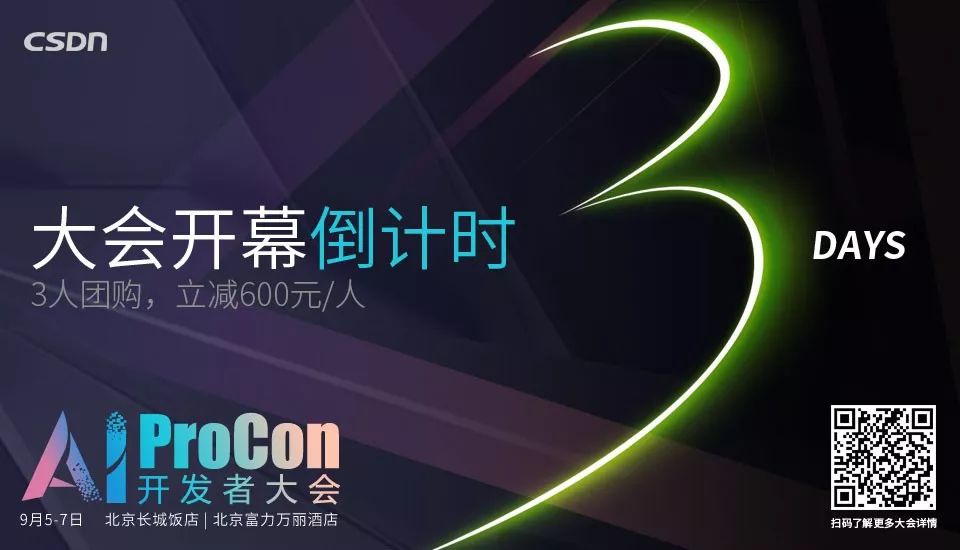
微软麻将AI Suphx或引入“凤凰房”,与其他AI对打
作者 | 夕颜出品 | AI科技大本营(ID:rgznai100)【导读】在刚刚结束的上海2019世界人工智能大会上,微软宣布了其在人工智能领域的最新研究突破——由微软亚洲研究院研发的麻将 AI 系统 Suphx 在国际知名的专业麻将平台“天凤”上荣升十段&…
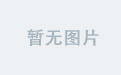
C++11中std::function的使用
类模版std::function是一种通用、多态的函数封装。std::function的实例可以对任何可以调用的目标实体进行存储、复制、和调用操作,这些目标实体包括普通函数、Lambda表达式、函数指针、以及其它函数对象等。 通过std::function对C中各种可调用实体(普通函数、Lambd…
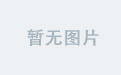
django模板的导入
模板导入 前提:多个页面有一个相同的页面版块(多个有样式标签的集合体) 如何运用:可以将多个样式标签的集合进行封装对外提供版块的名字(接口),在有该版块的页面中直接导入即可 语法:{% include 版块页面的路径 %} 四inclusion_tag自定义标签 -- 模板导入 前提:多个页面有一个相…

[UML]UML系列——包图Package
系列文章 [UML]UML系列——用例图Use Case [UML]UML系列——用例图中的各种关系(include、extend) [UML]UML系列——类图Class [UML]UML系列——类图class的关联关系(聚合、组合) [UML]UML系列——类图class的依赖关系 [UML]UML系…
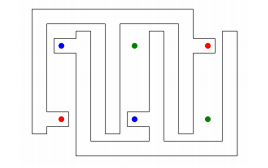
2017-2018 ACM-ICPC German Collegiate Programming Contest (GCPC 2017)
A Drawing Borders 很多构造方法,下图可能是最简单的了 代码: #include<bits/stdc.h> using namespace std; const int maxn1e610; struct Point{ int x,y; }; Point a[maxn]; int numa0; Point b[maxn]; int numb0; vector<pair<double,d…
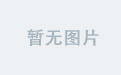
C++11中std::bind的使用
std::bind函数是用来绑定函数调用的某些参数的。std::bind它可以预先把指定可调用实体的某些参数绑定到已有的变量,产生一个新的可调用实体。它绑定的参数的个数不受限制,绑定的具体哪些参数也不受限制,由用户指定。 std::bind:(…
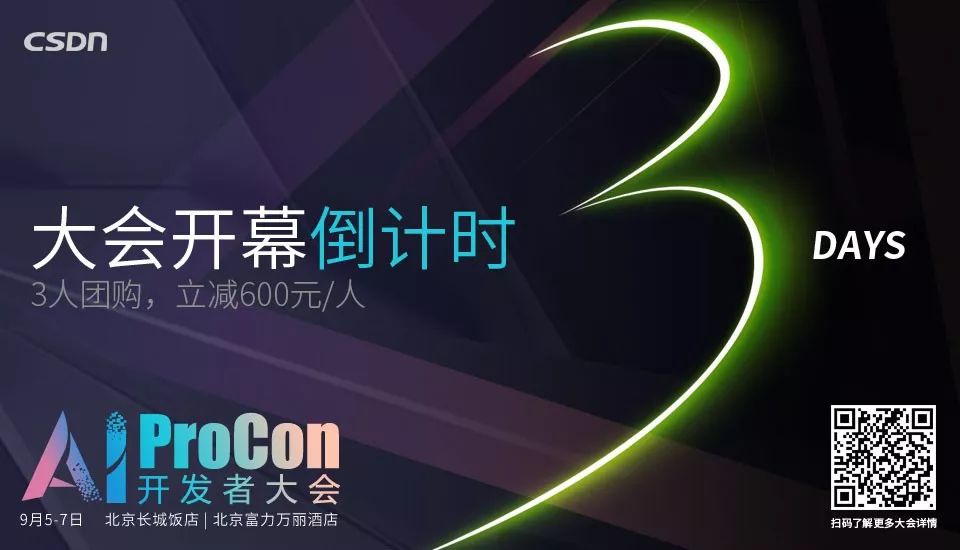
在图数据上做机器学习,应该从哪个点切入?
作者 | David Mack编译 | ronghuaiyang来源 | AI公园(ID:AI_Paradise)【导读】很多公司和机构都在使用图数据,想在图上做机器学习但不知从哪里开始做,希望这篇文章给大家一点启发。自从我们在伦敦互联数据中心(Connected Data Lon…
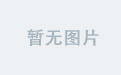
C++11中Lambda表达式的使用
Lambda表达式语法:[capture ] ( params ) mutable exception attribute -> return-type { body } 其中capture为定义外部变量是否可见(捕获),若为空,则表示不捕获所有外部变量,即所有外部变量均不可访问, 表示所有…
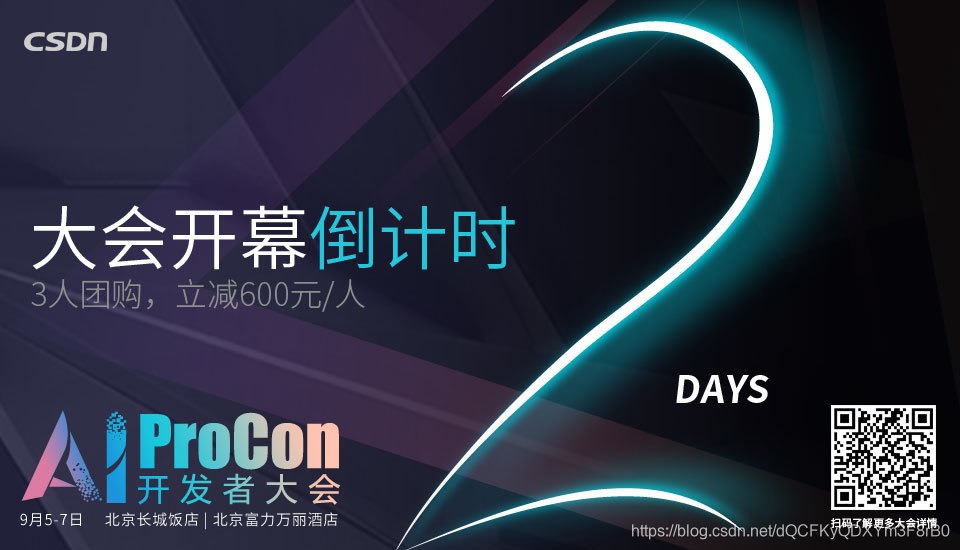
倒计时2天 | 专属技术人的盛会,为你而来!
5G 元年,人工智能 60 年,全球AI市场正发生着巨大的变化,顶尖科技企业和创新力量不断地进行着技术的更迭和应用的推进,专属于 AI 开发者的技术盛宴——2019 AI开发者大会(AI ProCon)将于 2 天后(…
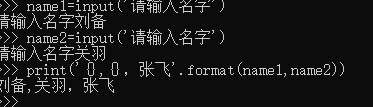
了解大数据的特点、来源与数据呈现方式
作业来源:https://edu.cnblogs.com/campus/gzcc/GZCC-16SE1/homework/2639 浏览2019春节各种大数据分析报告,例如: 这世间,再无第二个国家有能力承载如此庞大的人流量。http://www.sohu.com/a/290025769_313993春节人口迁徙大数据…
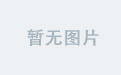
Mysql使用大全 从基础到存储过程
平常习惯了phpmyadmin等其他工具的的朋友有的根本就不会命令,如果让你笔试去面试我看你怎么办,所以,学习一下还是非常有用的,也可以知道你通过GUI工具的时候工具到底做了什么。Mysql用处很广,是php最佳拍档,…
GDAL库简介以及在Windows下编译过程
GDAL(Geospatial Data Abstraction Library,地理空间数据抽象库)是一个在X/MIT许可协议下的开源栅格空间数据转换库。官网http://www.gdal.org/index.html,也可参考GitHub https://github.com/OSGeo/gdal,最新release版本为2.1.1. GDAL是一个…
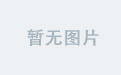
Hexo博客NexT主题美化之评论系统
前言 更多效果展示,请访问我的 个人博客。 效果图: Valine 诞生于2017年8月7日,是一款基于Leancloud的快速、简洁且高效的无后端评论系统。 教程: 登录 Leancloud 官网,注册之后创建一个应用,选择【设置】-…
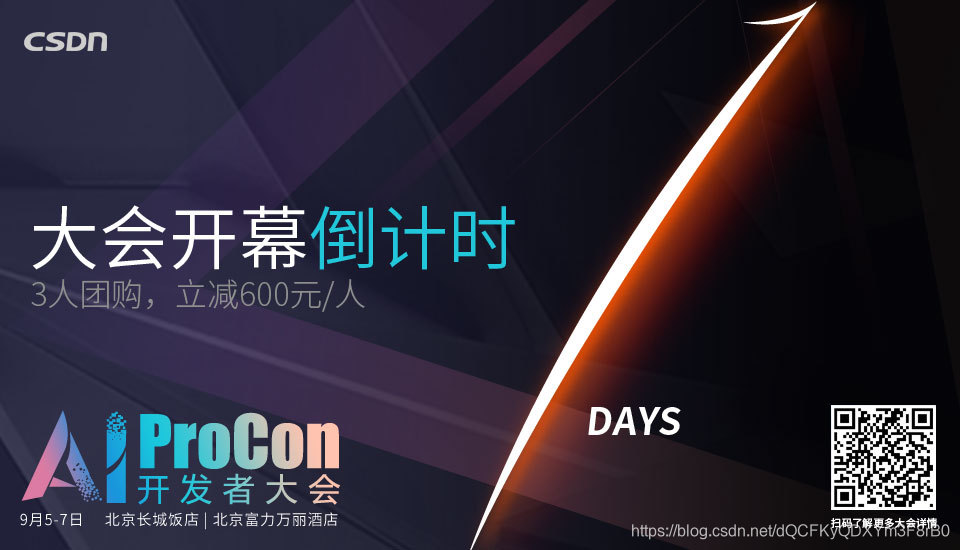
倒计时1天 | 专属技术人的盛会,为你而来!
5G 元年,人工智能 60 年,全球AI市场正发生着巨大的变化,顶尖科技企业和创新力量不断地进行着技术的更迭和应用的推进,专属于 AI 开发者的技术盛宴——2019 AI开发者大会(AI ProCon)将于 明天(9 …

Selenium 2 WebDriver 多线程 并发
我用的是Selenium2,至于它的背景和历史就不赘述了。Selenium2也叫WebDriver。下面讲个例子,用WebDriverjava来写个自动化测试的程序。(如果能用firefox去测试的话,我就直接用Selenium IDE录脚本了。。。)有个前提&…
GDAL2.1.1库在Ubuntu14.04下编译时遇到的问题处理方法
不用作任何调整,直接在Linux下编译GDAL2.1.1源码的步骤是:$ ./configure $ make $ make install非常简单, 这样也能正常生成gdal动态库、静态库,如果想将生成的文件放到指定的目录,则需改第一条命令为:$ ./…
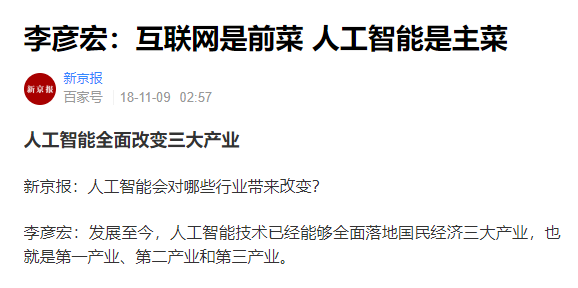
刷爆了!这项技术BAT力捧!程序员:我彻底慌了...
人工智能离我们还遥远吗?近日,海底捞斥资1.5亿打造了中国首家火锅无人餐厅;阿里酝酿了两年之久的全球首家无人酒店也正式开始运营,百度无人车彻底量产。李彦宏称,这是中国第一款能够量产的无人驾驶乘用车。而阿里的这家…
redux的compose源码,中文注释
用图片会更清楚一点,注释和代码会分的清楚源码解析参考请参考https://segmentfault.com/a/11...
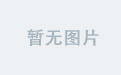
做好职业规划:做自己的船长
要想在职场上有所斩获,就必须做好职业规划。对于职场中人来说,职业规划是职业发展中最关键的向导。职业规划因人而异,不同的对象有不同的需求,因此制定的目标与计划也不尽相同,但个人为自己做职业规划的方法和流程是大…
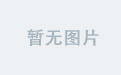
GDAL中GDALDataset::RasterIO分块读取的实现
GDALDataset类中的RasterIO函数能够对图像任意指定区域、任意波段的数据按指定数据类型、指定排列方式读入内存和写入文件中,因此可以实现对大影像的分块读、写运算操作。针对特大的影像图像,有时为了减少内存消耗,对图像进行分块读取很有必要…
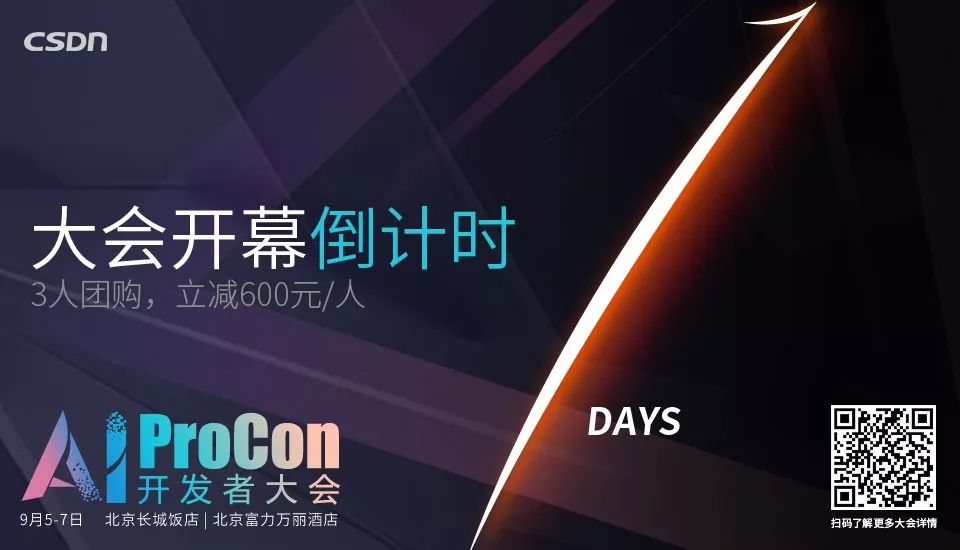
掌握深度学习,为什么要用PyTorch、TensorFlow框架?
作者 | Martin Heller译者 | 弯月责编 | 屠敏来源 | CSDN(ID:CSDNnews)【导读】如果你需要深度学习模型,那么 PyTorch 和 TensorFlow 都是不错的选择。并非每个回归或分类问题都需要通过深度学习来解决。甚至可以说,并…
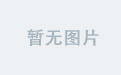
ICANN敦促业界使用DNSSEC,应对DNS劫持攻击
HTTPS加密 可以有效帮助服务器应对DNS欺骗、DNS劫持、ARP攻击等安全威胁。DNS是什么?DNS如何被利用?HTTPS如何防止DNS欺骗? DNS如何工作? 如果您想访问www.example.com,您的浏览器需要找到该特定Web服务器的IP地址。它…

Lucene.net: the main concepts
2019独角兽企业重金招聘Python工程师标准>>> In the previous post you learnt how to get a copy of Lucene.net and where to go in order to look for more information. As you noticed the documentation is far from being complete and easy to read. So in …
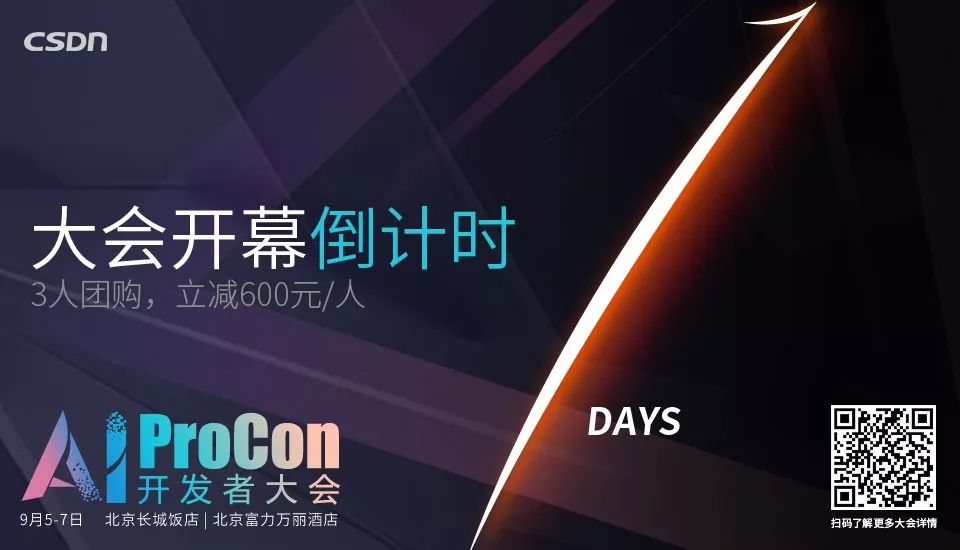
einsum,一个函数走天下
作者 | 永远在你身后转载自知乎【导读】einsum 全称 Einstein summation convention(爱因斯坦求和约定),又称为爱因斯坦标记法,是爱因斯坦 1916 年提出的一种标记约定,本文主要介绍了einsum 的应用。简单的说ÿ…
常用排序算法的C++实现
排序是将一组”无序”的记录序列调整为”有序”的记录序列。假定在待排序的记录序列中,存在多个具有相同的关键字的记录,若经过排序,这些记录的相对次序保持不变,即在原序列中,rirj,且ri在rj之前࿰…

4.65FTP服务4.66测试登录FTP
2019独角兽企业重金招聘Python工程师标准>>> FTP服务 测试登录FTP 4.65FTP服务 文件传输协议(FTP),可以上传和下载文件。比如我们可以把Windows上的文件shan上传到Linux,也可以把Linux上的文件下载到Windows上。 Cent…
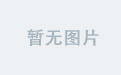
JavaScript的应用
DOM, BOM, XMLHttpRequest, Framework, Tool (Functionality) Performance (Caching, Combine, Minify, JSLint) ---------------- 人工做不了,交给程序去做,这样可以流程化。 Maintainability (Pattern) http://www.jmarshall.com/easy/http/ http://dj…