C++/C++11中std::queue的使用
std::queue: 模板类queue定义在<queue>头文件中。队列(Queue)是一个容器适配器(Container adaptor)类型,被特别设计用来运行于FIFO(First-in first-out)场景,在该场景中,只能从容器一端添加(Insert)元素,而在另一端提取(Extract)元素。只能从容器”后面”压进(Push)元素,从容器”前面”提取(Pop)元素。用来实现队列的底层容器必须满足顺序容器的所有必要条件。
queue模板类需要两个模板参数,一个是元素类型,一个容器类型,元素类型是必要的,容器类型是可选的,默认为deque类型。
std::queue: FIFO queue, queues are a type of container adaptor, specifically designed to operate in a FIFO context (first-in first-out), where elements are inserted into one end of the container and extracted from the other. queues are implemented as containers adaptors, which are classes that use an encapsulated object of a specific container class as its underlying container, providing a specific set of member functions to access its elements. Elements are pushed into the "back"of the specific container and popped from its "front".
The std::queue class is a container adapter that gives the programmer the functionality of a queue - specifically, a FIFO (first-in, first-out) data structure. The class template acts as a wrapper to the underlying container - only a specific set of functions is provided. The queue pushes the elements on the back of the underlying container and pops them from the front. Queue does not allow iteration through its elements.
下面是从其他文章中copy的测试代码,详细内容介绍可以参考对应的reference:
#include "queue.hpp"
#include <iostream>
#include <queue> // std::queue
#include <vector>
#include <string>
#include <list>//
// reference: http://www.cplusplus.com/reference/queue/queue/
int test_queue_1()
{
{ // std::queue::back: Access last element, Returns a reference to the last element in the queue.// queue::front: access the first element, queue::back: access the last elementstd::queue<int> myqueue;myqueue.push(12);myqueue.push(20);myqueue.push(75); // this is now the backfprintf(stderr, "myqueue.back: %d, myqueue.front: %d\n", myqueue.back(), myqueue.front());myqueue.back() -= myqueue.front(); // 75 -= 12std::cout << "myqueue.back() is now " << myqueue.back() << '\n'; // myqueue.back() is now 63
}{ // std::queue::front: Access next element, Returns a reference to the next element in the queue.// The next element is the "oldest" element in the queue and// the same element that is popped out from the queue when queue::pop is called.std::queue<int> myqueue;myqueue.push(77);myqueue.push(20);myqueue.push(16);fprintf(stderr, "myqueue.back: %d, myqueue.front: %d\n", myqueue.back(), myqueue.front());myqueue.front() -= myqueue.back(); // 77-16=61std::cout << "myqueue.front() is now " << myqueue.front() << '\n';
}{ // std::queue::push: Inserts a new element at the end of the queue, after its current last element// std::queue::pop: Remove next element, Removes the next element in the queue, effectively reducing its size by one.// std::queue::empty: Test whether container is empty, Returns whether the queue is empty: i.e. whether its size is zero.std::queue<int> myqueue;int myint;std::cout << "Please enter some integers (enter 0 to end):\n";std::vector<int> vec{ 1, 2, 3, 4, 5 };for (const auto& value : vec) {myqueue.push(value);}fprintf(stderr, "myqueue.back: %d, myqueue.front: %d\n", myqueue.back(), myqueue.front());std::cout << "myqueue contains: ";while (!myqueue.empty()) {std::cout << ' ' << myqueue.front();myqueue.pop();}std::cout << '\n';
}{ // std::queue::emplace: C++11, Construct and insert element.// Adds a new element at the end of the queue, after its current last element.// The element is constructed in-place, i.e. no copy or move operations are performed.// queue::push: inserts element at the end , queue::emplace: constructs element in-place at the end// queue::pop: removes the first element std::queue<std::string> myqueue;myqueue.emplace("First sentence");myqueue.emplace("Second sentence");std::cout << "myqueue contains:\n";while (!myqueue.empty()) {std::cout << myqueue.front() << '\n';myqueue.pop();}
}{ // std::queue::size: Returns the number of elements in the queuestd::queue<int> myints;std::cout << "0. size: " << myints.size() << '\n';for (int i = 0; i < 5; i++) myints.push(i);std::cout << "1. size: " << myints.size() << '\n';myints.pop();std::cout << "2. size: " << myints.size() << '\n';
}{ // std::queue::swap: C++11, Exchanges the contents of the container adaptor// std::swap (queue): Exchanges the contents of x and y.std::queue<int> foo, bar;foo.push(10); foo.push(20); foo.push(30);bar.push(111); bar.push(222);foo.swap(bar);// std::swap(foo, bar);std::cout << "size of foo: " << foo.size() << '\n';std::cout << "size of bar: " << bar.size() << '\n';std::cout << "foo contains: ";while (!foo.empty()) {std::cout << ' ' << foo.front();foo.pop();}std::cout << '\n';std::cout << "bar contains: ";while (!bar.empty()) {std::cout << ' ' << bar.front();bar.pop();}std::cout << '\n';
}return 0;
}// reference: http://stackoverflow.com/questions/709146/how-do-i-clear-the-stdqueue-efficiently
static void clear(std::queue<int> &q)
{std::queue<int> empty;std::swap(q, empty);
}int test_queue_2()
{// clear queue, avoid to pop in a loop// A common idiom for clearing standard containers is swapping with an empty version of the containerstd::queue<int> foo;foo.push(10); foo.push(20); foo.push(30);fprintf(stderr, "foo size: %d\n", foo.size());clear(foo);fprintf(stderr, "foo size: %d\n", foo.size());return 0;
}//
// reference: https://msdn.microsoft.com/en-us/library/s23s3de6.aspx
int test_queue_3()
{
{ // queue::back: Returns a reference to the last and most recently added element at the back of the queue.// The last element of the queue. If the queue is empty, the return value is undefined.using namespace std;queue <int> q1;q1.push(10);q1.push(11);int& i = q1.back();const int& ii = q1.front();cout << "The integer at the back of queue q1 is " << i << "." << endl;cout << "The integer at the front of queue q1 is " << ii << "." << endl;
}{ // queue::empty: true if the queue is empty; false if the queue is nonempty.using namespace std;// Declares queues with default deque base containerqueue <int> q1, q2;q1.push(1);if (q1.empty()) cout << "The queue q1 is empty." << endl;else cout << "The queue q1 is not empty." << endl;if (q2.empty()) cout << "The queue q2 is empty." << endl;else cout << "The queue q2 is not empty." << endl;
}{ // queue::front: Returns a reference to the first element at the front of the queue// queue::size_type: An unsigned integer type that can represent the number of elements in a queue.using namespace std;queue <int> q1;q1.push(10);q1.push(20);q1.push(30);queue <int>::size_type i;i = q1.size();cout << "The queue length is " << i << "." << endl;int& ii = q1.back();int& iii = q1.front();cout << "The integer at the back of queue q1 is " << ii << "." << endl;cout << "The integer at the front of queue q1 is " << iii << "." << endl;
}{ // queue::pop: Removes an element from the front of the queueusing namespace std;queue <int> q1, s2;q1.push(10);q1.push(20);q1.push(30);queue <int>::size_type i;i = q1.size();cout << "The queue length is " << i << "." << endl;i = q1.front();cout << "The element at the front of the queue is " << i << "." << endl;q1.pop();i = q1.size();cout << "After a pop the queue length is " << i << "." << endl;i = q1.front();cout << "After a pop, the element at the front of the queue is " << i << "." << endl;
}{ // queue::push: Adds an element to the back of the queue.using namespace std;queue <int> q1;q1.push(10);q1.push(20);q1.push(30);queue <int>::size_type i;i = q1.size();cout << "The queue length is " << i << "." << endl;i = q1.front();cout << "The element at the front of the queue is " << i << "." << endl;
}{ // queue::queue: Constructs a queue that is empty or that is a copy of a base container object.using namespace std;// Declares queue with default deque base containerqueue <char> q1;// Explicitly declares a queue with deque base containerqueue <char, deque<char> > q2;// These lines don't cause an error, even though they// declares a queue with a vector base containerqueue <int, vector<int> > q3;q3.push(10);// but the following would cause an error because vector has// no pop_front member function// q3.pop( );// Declares a queue with list base containerqueue <int, list<int> > q4;// The second member function copies elements from a containerlist<int> li1;li1.push_back(1);li1.push_back(2);queue <int, list<int> > q5(li1);cout << "The element at the front of queue q5 is " << q5.front() << "." << endl;cout << "The element at the back of queue q5 is " << q5.back() << "." << endl;
}{ // queue::size: Returns the number of elements in the queueusing namespace std;queue <int> q1, q2;queue <int>::size_type i;q1.push(1);i = q1.size();cout << "The queue length is " << i << "." << endl;q1.push(2);i = q1.size();cout << "The queue length is now " << i << "." << endl;
}{ // queue::value_type: A type that represents the type of object stored as an element in a queue.using namespace std;// Declares queues with default deque base containerqueue<int>::value_type AnInt;AnInt = 69;cout << "The value_type is AnInt = " << AnInt << endl;queue<int> q1;q1.push(AnInt);cout << "The element at the front of the queue is " << q1.front() << "." << endl;
}return 0;
}
GitHub: https://github.com/fengbingchun/Messy_Test
相关文章:
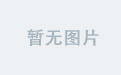
常见的http状态码(Http Status Code)
常见的http状态码:(收藏学习) 2**开头 (请求成功)表示成功处理了请求的状态代码。 200 (成功) 服务器已成功处理了请求。 通常,这表示服务器提供了请求的网页。201 (已创…
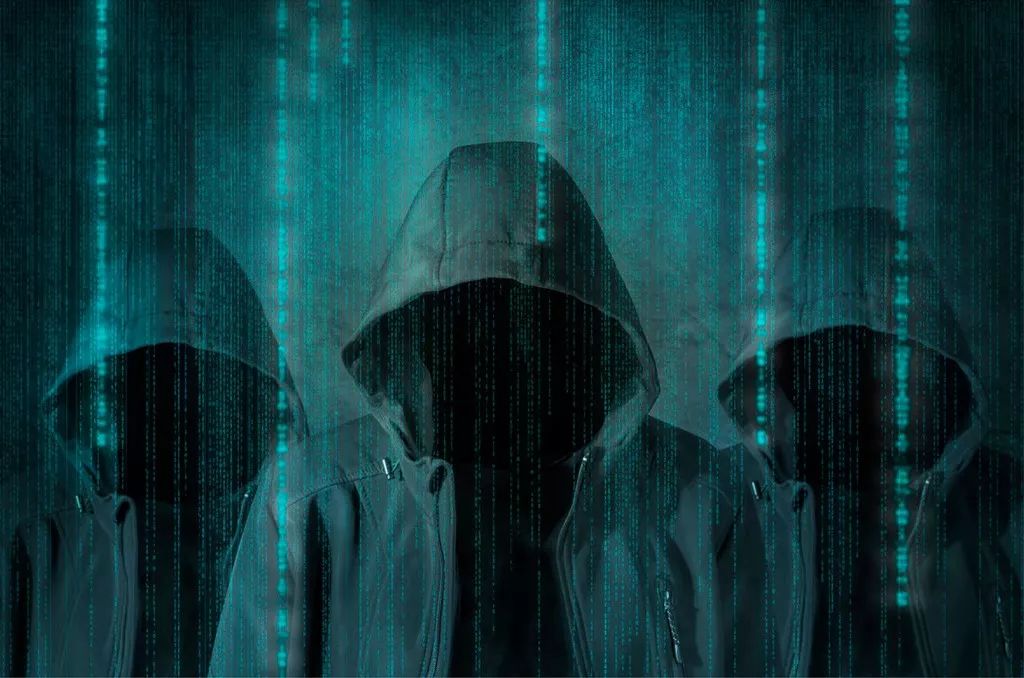
“不给钱就删库”的勒索病毒, 程序员该如何防护?
作者 | 阿木,王洪鹏,运营有个人公众号新新生活志。目前任职网易云计算技术部高级工程师,近3年云计算从业经验,爱读书、爱写作、爱技术。责编 | 郭芮来源 | CSDN(ID:CSDNnews)近期一家名为ProPub…
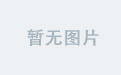
ruby实时查看日志
(文章是从我的个人主页上粘贴过来的, 大家也可以访问我的主页 www.iwangzheng.com) 在调试代码的时候,把日志文件打开,边操作边调试能很快帮助我们发现系统中存在的问题。 $tail rails_2014_03_03.log -f转载于:https://www.cnblogs.com/iw…
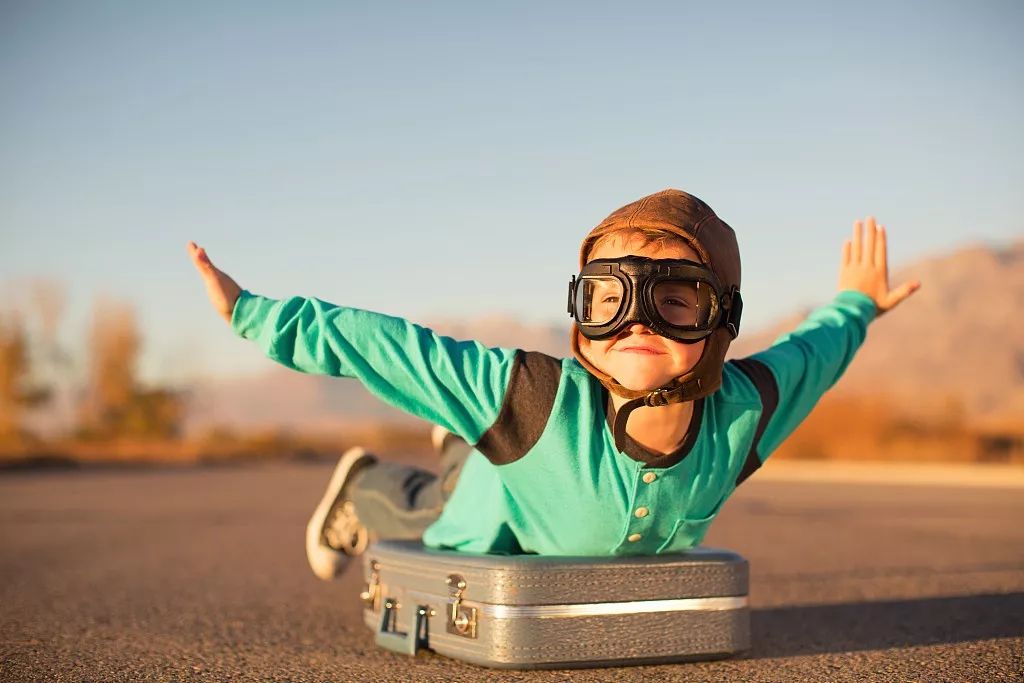
干货 | OpenCV看这篇就够了,9段代码详解图像变换基本操作
作者 | 王天庆,长期从事分布式系统、数据科学与工程、人工智能等方面的研究与开发,在人脸识别方面有丰富的实践经验。现就职某世界100强企业的数据实验室,从事数据科学相关技术领域的预研工作。来源 | 大数据(ID:hzdas…
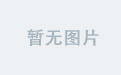
C++/C++11中std::priority_queue的使用
std::priority_queue:在优先队列中,优先级高的元素先出队列,并非按照先进先出的要求,类似一个堆(heap)。其模板声明带有三个参数,priority_queue<Type, Container, Functional>, 其中Type为数据类型,Container为…
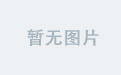
left join 和 left outer join 的区别
老是混淆,做个笔记,转自:https://www.cnblogs.com/xieqian111/p/5735977.html left join 和 left outer join 的区别 通俗的讲: A left join B 的连接的记录数与A表的记录数同 A right join B 的连接的记录数与…
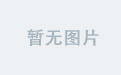
php减少损耗的方法之一 缓存对象
即把实例后的对象缓存起来(存入变量),当需要再次实例化时,先去缓存里查看是否存在。存在则返回。否则实例化。转载于:https://www.cnblogs.com/zuoxiaobing/p/3581139.html
windows10 vs2013控制台工程中添加并编译cuda8.0文件操作步骤
一般有两种方法可以在vs2013上添加运行cuda8.0程序:一、直接新建一个基于CUDA8.0的项目:如下图所示,点击确定后即可生成test_cuda项目;默认会自动生成一个kernel.cu文件;默认已经配置好Debug/Release, Win32/x64环境&a…
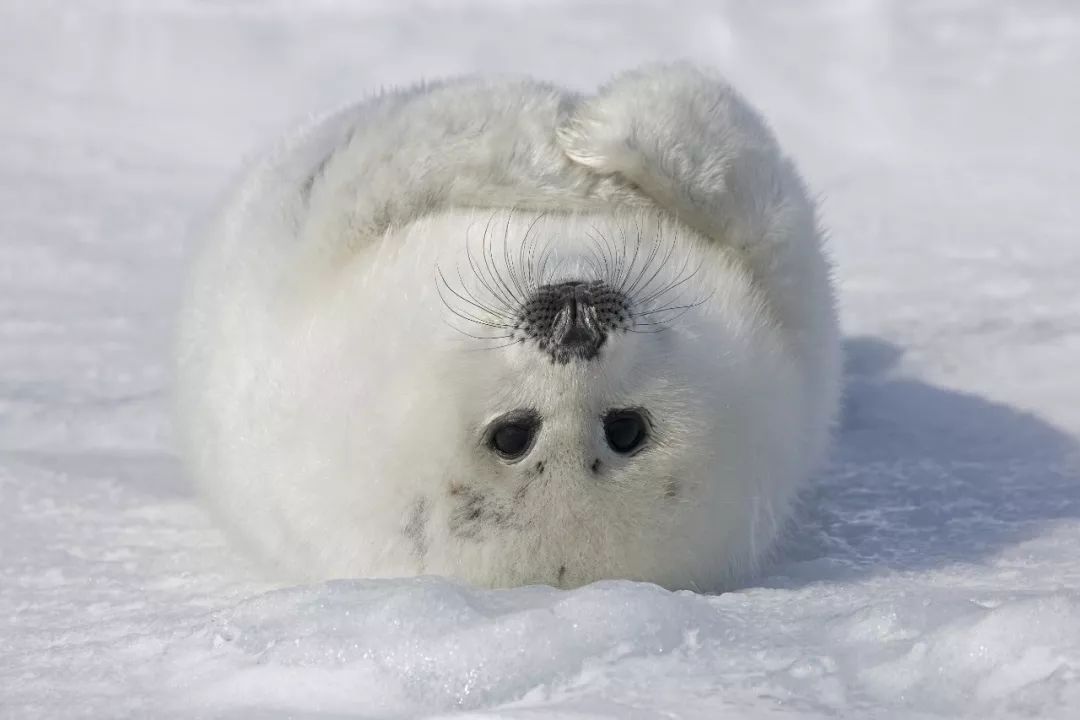
算法人必懂的进阶SQL知识,4道面试常考题
(图片付费下载自视觉中国)作者 | 石晓文来源|小小挖掘机(ID:wAlsjwj)近期在不同群里有小伙伴们提出了一些在面试和笔试中遇到的Hive SQL问题,Hive作为算法工程师的一项必备技能,在面…
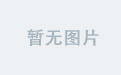
007-迅雷定时重启AutoHotkey脚本-20190411
;; 定时重启迅雷.ahk,;;~ 2019年04月11日;#SingleInstance,forceSetWorkingDir,%A_ScriptDir%DetectHiddenWindows,OnSetTitleMatchMode,2#Persistent ;让脚本持久运行(即直到用户关闭或遇到 ExitApp)。#NoEnv;~ #NoTrayIcon Hotkey,^F10,ExitThisApp lo…

关于ExtJS在使用下拉列表框的二级联动获取数据
2019独角兽企业重金招聘Python工程师标准>>> 使用下拉列表框的二级联动获取数据,如果第一个下拉列表框有默认值时,需要设置fireEvent执行select事件 示例: var combo Ext.getCmp("modifyBuildCom"); combo.setValue(re…
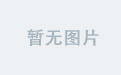
C++中std::sort/std::stable_sort/std::partial_sort的区别及使用
某些算法会重排容器中元素的顺序,如std::sort。调用sort会重排输入序列中的元素,使之有序,它默认是利用元素类型的<运算符来实现排序的。也可以重载sort的默认排序,即通过sort的第三个参数,此参数是一个谓词(predic…
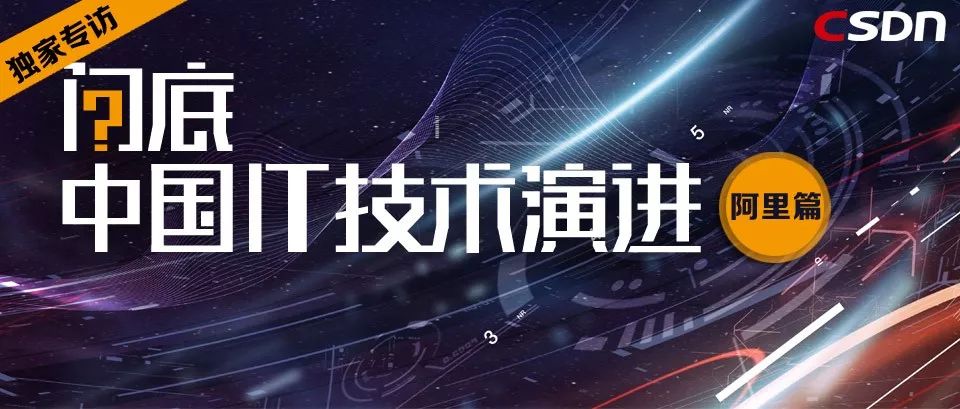
阿里云智能 AIoT 首席科学家丁险峰:阿里全面进军IoT这一年 | 问底中国IT技术演进...
作者 | 屠敏受访者 | 丁险峰来源 | CSDN(ID:CSDNnews)「忽如一夜春风来,千树万树梨花开。」从概念的流行、至科技巨头的相继入局、再到诸多应用的落地,IoT 的发展终于在万事俱备只欠东风的条件下真正地迎来了属于自己的…

eBCC性能分析最佳实践(1) - 线上lstat, vfs_fstatat 开销高情景分析...
Guide: eBCC性能分析最佳实践(0) - 开启性能分析新篇章eBCC性能分析最佳实践(1) - 线上lstat, vfs_fstatat 开销高情景分析eBCC性能分析最佳实践(2) - 一个简单的eBCC分析网络函数的latency敬请期待...0. I…

spring-data-mongodb必须了解的操作
http://docs.spring.io/spring-data/data-mongo/docs/1.0.0.M5/api/org/springframework/data/mongodb/core/MongoTemplate.html 在线api文档 1关键之识别 KeywordSampleLogical resultGreaterThanfindByAgeGreaterThan(int age){"age" : {"$gt" : age}}Le…
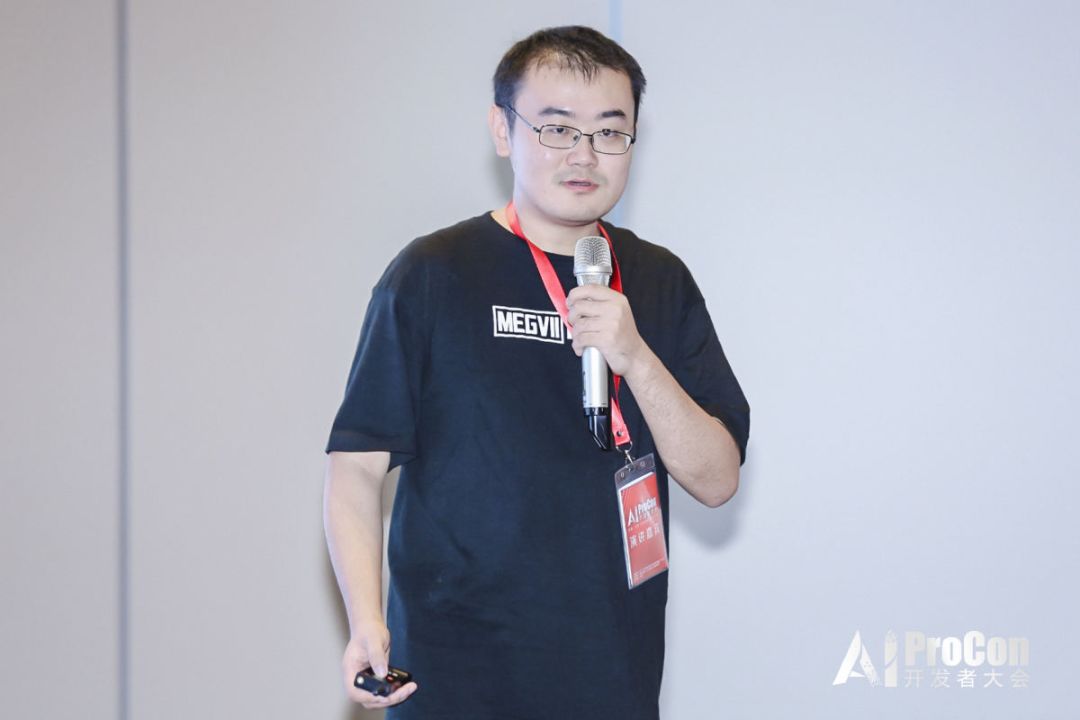
旷视张祥雨:高效轻量级深度模型的研究和实践 | AI ProCon 2019
演讲嘉宾 | 张祥雨(旷视研究院主任研究员、基础模型组负责人)编辑 | Just出品 | AI科技大本营(ID:rgznai100)基础模型是现代视觉识别系统中一个至关重要的关注点。基础模型的优劣主要从精度、速度或功耗等角度判定,如何…
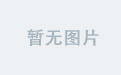
Python脱产8期 Day02
一 语言分类 机器语言,汇编语言,高级语言(编译和解释) 二 环境变量 1、配置环境变量不是必须的2、配置环境变量的目的:为终端提供执行环境 三Python代码执行的方式 1交互式:.控制台直接编写运行python代码 …
分别用Eigen和C++(OpenCV)实现图像(矩阵)转置
(1)、标量(scalar):一个标量就是一个单独的数。(2)、向量(vector):一个向量是一列数,这些数是有序排列的,通过次序中的索引,可以确定每个单独的数。(3)、矩阵(matrix):矩阵是一个二维数组,其中的…
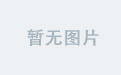
Linux基础优化
***************************************************************************************linux系统的优化有很多,我简单阐述下我经常优化的方针:记忆口诀:***********************一清、一精、一增;两优、四设、七其他。*****…
数据集cifar10到Caffe支持的lmdb/leveldb转换的实现
在 http://blog.csdn.net/fengbingchun/article/details/53560637 对数据集cifar10进行过介绍,它是一个普通的物体识别数据集。为了使用Caffe对cifar10数据集进行train,下面实现了将cifar10到lmdb/leveldb的转换实现:#include "funset.h…
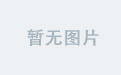
计算两个时间的间隔时间是多少
/*** 计算两个时间间隔* param startTime 开始时间* param endTime 结束时间* param type 类型(1:相隔小时 2:)* return*/public static int compareTime(String startTime, String endTime, int type) {if (endTime nul…
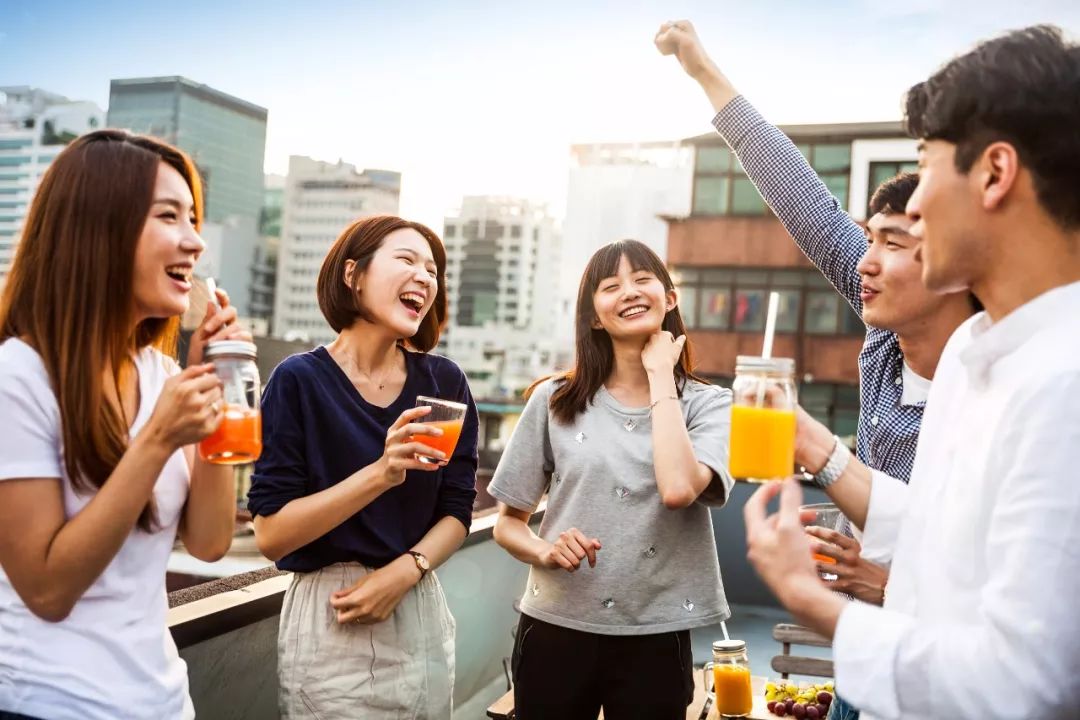
作为西二旗程序员,我是这样学习的.........
作为一名合格的程序员,需要时刻保持对新技术的敏感度,并且要定期更新自己的技能储备,是每个技术人的日常必修课。但要做到这一点,知乎上的网友说最高效的办法竟然是直接跟 BAT 等一线大厂取经。讲真的,BAT大厂的平台是…

2月国内搜索市场:360继续上升 百度下降0.62%
IDC评述网(idcps.com)03月06日报道:根据CNZZ数据显示,在国内搜索引擎市场中,百度在2014年2月份所占的份额继续被蚕食,环比1月份,下降了0.62%,为60.50%。与此相反,360搜索…
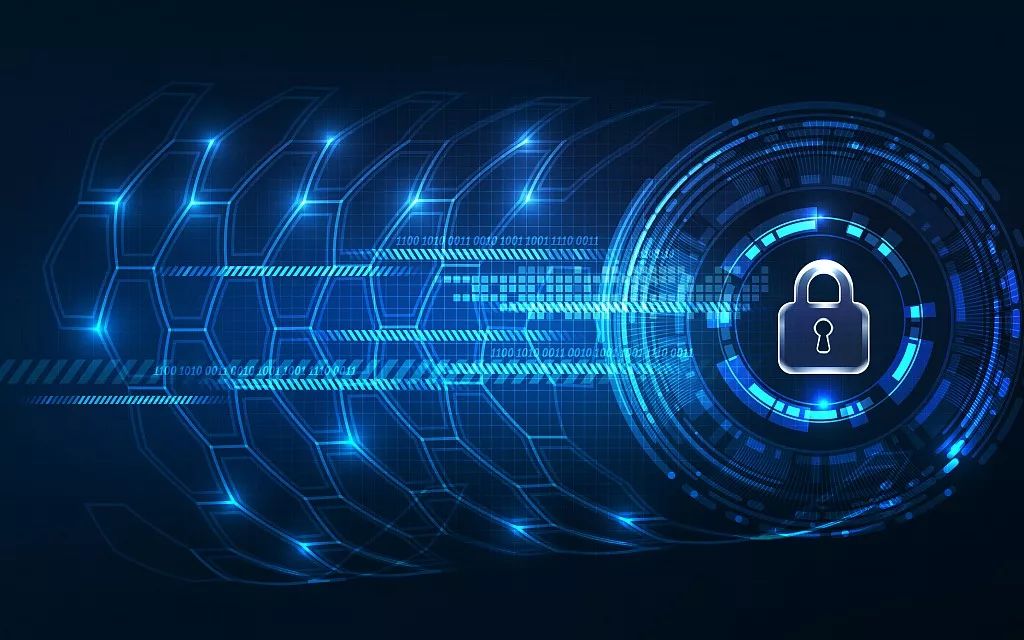
不止于刷榜,三大CV赛事夺冠算法技术的“研”与“用”
(由AI科技大本营付费下载自视觉中国)整理 | Jane出品 | AI科技大本营(ID:rgznai100)在 5 个月时间里(5月-9月),创新工场旗下人工智能企业创新奇智连续在世界顶级人脸检测竞赛 WIDER …
Ubuntu14.04上编译指定版本的protobuf源码操作步骤
Google Protobuf的介绍可以参考 http://blog.csdn.net/fengbingchun/article/details/49977903 ,这里介绍在Ubuntu14.04上编译安装指定版本的protobuf的操作步骤,这里以2.4.1为例:1. Ubuntu14.04上默认安装的是2.5.0,…
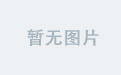
Linux下,各种解压缩命令集合
Linux下,各种解压缩命令集合tar xvfj lichuanhua.tar.bz2tar xvfz lichuanhua.tar.gztar xvfz lichuanhua.tgztar xvf lichuanhua.tarunzip lichuanhua.zip.gz解压 1:gunzip FileName.gz解压 2:gzip -d FileName.gz压缩:gzip File…
gtest使用初级指南
之前在 http://blog.csdn.net/fengbingchun/article/details/39667571 中对google的开源库gtest进行过介绍,现在看那篇博文,感觉有些没有说清楚,这里再进行总结下:Google Test是Google的开源C单元测试框架,简称gtest。…
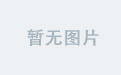
iOS视频流采集概述(AVCaptureSession)
需求:需要采集到视频帧数据从而可以进行一系列处理(如: 裁剪,旋转,美颜,特效....). 所以,必须采集到视频帧数据. 阅读前提: 使用AVFoundation框架采集音视频帧数据GitHub地址(附代码) : iOS视频流采集概述 简书地址 : iOS视频流采…
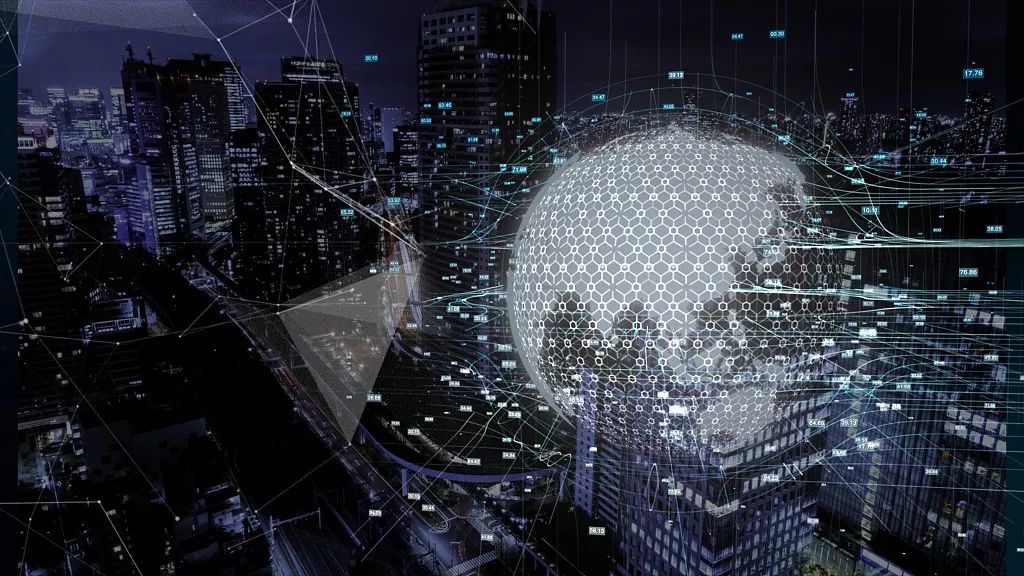
300秒搞定第一超算1万年的计算量,量子霸权时代已来?
(由AI科技大本营付费下载自视觉中国)作者 | 马超责编 | 郭芮来源 | CSDN 博客近日,美国航天局(NASA)发布了一篇名为《Quantum Supremacy Using a Programmable Superconducting Processor》的报道,称谷歌的…
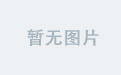
2014-3-6 星期四 [第一天执行分析]
昨日进度: [毛思想]:看测控技术量待定 --> [良]超额完成,昨天基本上把测控看了一大半啦 [汇编]:认真听课,边听边消化自学 --> [中]基本满足,还需要抽时间总结,特别是前面寻址的各种情况…