C++/C++11中头文件numeric的使用
<numeric>是C++标准程序库中的一个头文件,定义了C++ STL标准中的基础性的数值算法(均为函数模板):
(1)、accumulate: 以init为初值,对迭代器给出的值序列做累加,返回累加结果值,值类型必须支持”+”算符。它还有一个重载形式。
(2)、adjacent_difference:对输入序列,计算相邻两项的差值,写入到输出序列中。它还有一个重载形式。
(3)、inner_product:计算两个输入序列的内积。它还有一个重载形式。
(4)、partial_sum:计算输入序列从开始位置到当前位置的累加值,写入输出序列中。它还有一个重载形式。
(5)、iota: 向序列中写入以val为初值的连续值序列。
The algorithms are similar to the Standard Template Library (STL) algorithms and are fully compatible with the STL but are part of the C++ Standard Library rather than the STL. Like the STL algorithms, they are generic because they can operate on a variety of data structures. The data structures that they can operate on include STL container classes, such as vector and list, and program-defined data structures and arrays of elements that satisfy the requirements of a particular algorithm. The algorithms achieve this level of generality by accessing and traversing the elements of a container indirectly through iterators. The algorithms process iterator ranges that are typically specified by their beginning or ending positions. The ranges referred to must be valid in the sense that all pointers in the ranges must be dereferenceable and within the sequences of each range, the last position must be reachable from the first by means of incrementation.
下面是从其它文章中copy的<numeric>测试代码,详细内容介绍可以参考对应的reference:
#include "numeric.hpp"
#include <iostream>
#include <functional>
#include <numeric>// reference: http://www.cplusplus.com/reference/numeric/namespace numeric_ {/*
std::accumulate: The behavior of this function template is equivalent to :
template <class InputIterator, class T>
T accumulate (InputIterator first, InputIterator last, T init)
{while (first!=last) {init = init + *first; // or: init=binary_op(init,*first) for the binary_op version++first;}return init;
}
*/static int myfunction(int x, int y) { return x + 2 * y; }struct myclass {int operator()(int x, int y) { return x + 3 * y; }
} myobject;int test_numeric_accumulate()
{int init = 100;int numbers[] = { 10, 20, 30 };std::cout << "using default accumulate: ";std::cout << std::accumulate(numbers, numbers + 3, init); // 160std::cout << '\n';std::cout << "using functional's minus: ";std::cout << std::accumulate(numbers, numbers + 3, init, std::minus<int>()); // 40 std::minus => x - ystd::cout << '\n';std::cout << "using custom function: ";std::cout << std::accumulate(numbers, numbers + 3, init, myfunction); // 220std::cout << '\n';std::cout << "using custom object: ";std::cout << std::accumulate(numbers, numbers + 3, init, myobject); // 280std::cout << '\n';return 0;
}///
/*
std::adjacent_difference: The behavior of this function template is equivalent to
template <class InputIterator, class OutputIterator>
OutputIterator adjacent_difference (InputIterator first, InputIterator last, OutputIterator result)
{if (first!=last) {typename iterator_traits<InputIterator>::value_type val,prev;*result = prev = *first;while (++first!=last) {val = *first;*++result = val - prev; // or: *++result = binary_op(val,prev)prev = val;}++result;}return result;
}
*/static int myop(int x, int y) { return x + y; }int test_numeric_adjacent_difference()
{int val[] = { 1, 2, 3, 5, 9, 11, 12 };int result[7];std::adjacent_difference(val, val + 7, result);std::cout << "using default adjacent_difference: ";for (int i = 0; i<7; i++) std::cout << result[i] << ' '; // 1 1 1 2 4 2 1std::cout << '\n';std::adjacent_difference(val, val + 7, result, std::multiplies<int>()); // x * ystd::cout << "using functional operation multiplies: ";for (int i = 0; i<7; i++) std::cout << result[i] << ' '; // 1 2 6 15 45 99 132std::cout << '\n';std::adjacent_difference(val, val + 7, result, myop); // 1 3 5 8 14 20 23std::cout << "using custom function: ";for (int i = 0; i<7; i++) std::cout << result[i] << ' ';std::cout << '\n';return 0;
}/*
std::inner_product: The behavior of this function template is equivalent to:
template <class InputIterator1, class InputIterator2, class T>
T inner_product (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, T init)
{while (first1!=last1) {init = init + (*first1)*(*first2);// or: init = binary_op1 (init, binary_op2(*first1,*first2));++first1; ++first2;}return init;
}
*/static int myaccumulator(int x, int y) { return x - y; }
static int myproduct(int x, int y) { return x + y; }int test_numeric_inner_product()
{int init = 100;int series1[] = { 10, 20, 30 };int series2[] = { 1, 2, 3 };std::cout << "using default inner_product: ";std::cout << std::inner_product(series1, series1 + 3, series2, init); // 240std::cout << '\n';std::cout << "using functional operations: ";std::cout << std::inner_product(series1, series1 + 3, series2, init, std::minus<int>(), std::divides<int>()); // 70std::cout << '\n';std::cout << "using custom functions: ";std::cout << std::inner_product(series1, series1 + 3, series2, init,myaccumulator, myproduct); // 34std::cout << '\n';return 0;
}//
/*
std::partial_sum: The behavior of this function template is equivalent to:
template <class InputIterator, class OutputIterator>
OutputIterator partial_sum (InputIterator first, InputIterator last, OutputIterator result)
{if (first!=last) {typename iterator_traits<InputIterator>::value_type val = *first;*result = val;while (++first!=last) {val = val + *first; // or: val = binary_op(val,*first)*++result = val;}++result;}return result;
}
*/static int myop_(int x, int y) { return x + y + 1; }int test_numeric_partial_sum()
{int val[] = { 1, 2, 3, 4, 5 };int result[5];std::partial_sum(val, val + 5, result);std::cout << "using default partial_sum: ";for (int i = 0; i<5; i++) std::cout << result[i] << ' '; // 1 3 6 10 15std::cout << '\n';std::partial_sum(val, val + 5, result, std::multiplies<int>());std::cout << "using functional operation multiplies: ";for (int i = 0; i<5; i++) std::cout << result[i] << ' '; // 1 2 6 24 120std::cout << '\n';std::partial_sum(val, val + 5, result, myop_);std::cout << "using custom function: ";for (int i = 0; i<5; i++) std::cout << result[i] << ' '; // 1 4 8 13 19std::cout << '\n';return 0;
}///
/*
std::iota: The behavior of this function template is equivalent to:
template <class ForwardIterator, class T>
void iota (ForwardIterator first, ForwardIterator last, T val)
{while (first!=last) {*first = val;++first;++val;}
}
*/int test_numeric_iota()
{int numbers[10];std::iota(numbers, numbers + 10, 100);std::cout << "numbers:";for (int& i : numbers) std::cout << ' ' << i; // 100 101 102 103 104 105 106 107 108 109std::cout << '\n';return 0;
}} // namespace numeric_
GitHub: https://github.com/fengbingchun/Messy_Test
相关文章:
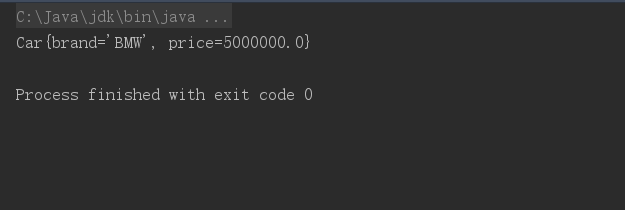
Spring基础16——使用FactoryBean来创建
1.配置bean的方式 配置bean有三种方式:通过全类名(class反射)、通过工厂方法(静态工厂&实例工厂)、通过FactoryBean。前面我们已经一起学习过全类名方式和工厂方法方式,下面通过这篇文章来学习一下Fact…

查看进程 端口
2019独角兽企业重金招聘Python工程师标准>>> 一 进程 ps -ef 1.UID 用户ID2.PID 进程ID3.PPID 父进程ID4.C CPU占用率5.STIME 开始时间6.TTY 开始此进程的TTY7.TIME 此进程运行的总时间8.CMD 命令名 二端口 netstat Linux下如果我…
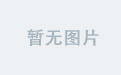
深度学习中的欠拟合和过拟合简介
通常情况下,当我们训练机器学习模型时,我们可以使用某个训练集,在训练集上计算一些被称为训练误差(training error)的度量误差,目标是降低训练误差。机器学习和优化不同的地方在于,我们也希望泛化误差(generalization …
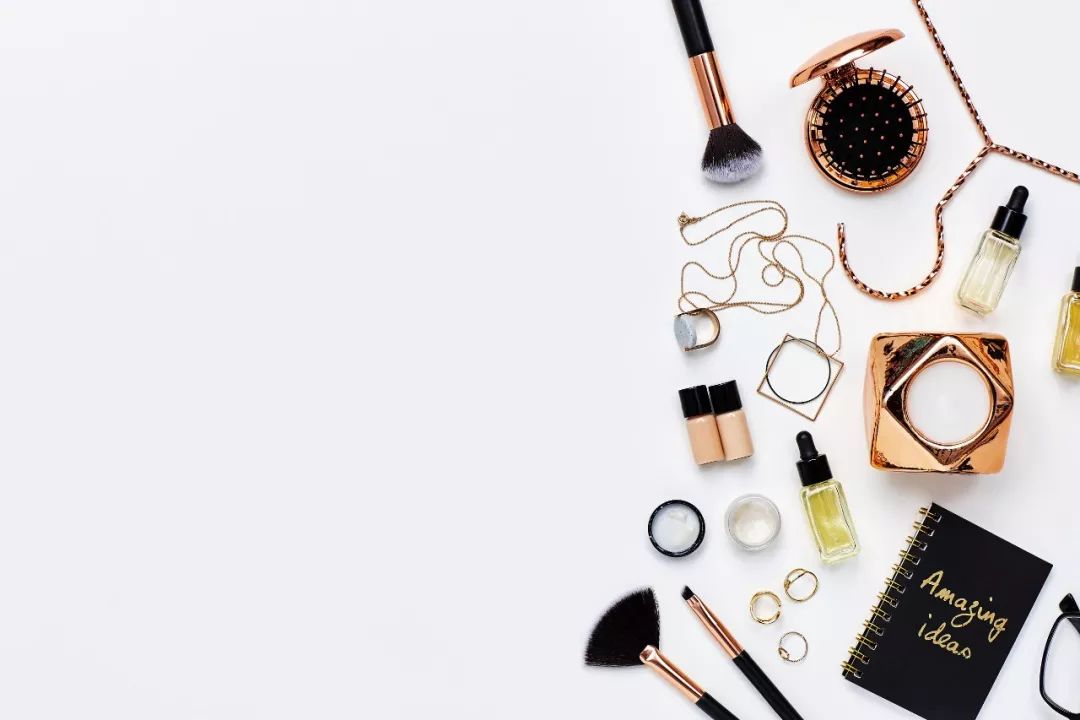
今日头条首次改进DQN网络,解决推荐中的在线广告投放问题
(图片付费下载自视觉中国)作者 | 深度传送门来源 | 深度传送门(ID:gh_5faae7b50fc5)【导读】本文主要介绍今日头条推出的强化学习应用在推荐的最新论文[1],首次改进DQN网络解决推荐中的在线广告投放问题。背景介绍随着…
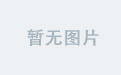
RPA实施过程中可能会遇到的14个坑
RPA的实施过程并非如我们所想的那样,总是一帆风顺。碰坑,在所难免。但也不必为此过于惊慌,因为,我们已经帮你把RPA实施之路上的坑找了出来。RPA实施过程中,将会遇到哪些坑? 【不看全文大纲版】●组织层面&a…

Android问题汇总
2019独角兽企业重金招聘Python工程师标准>>> 1. Only the original thread that created a view hierarchy can touch its views 在初始化activity是需要下载图片,所以重新开启了一个线程,下载图片更新ui,此时就出现了上面的错误。…
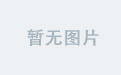
深度学习中的验证集和超参数简介
大多数机器学习算法都有超参数,可以设置来控制算法行为。超参数的值不是通过学习算法本身学习出来的(尽管我们可以设计一个嵌套的学习过程,一个学习算法为另一个学习算法学出最优超参数)。在多项式回归示例中,有一个超参数:多项式…
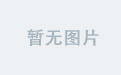
自定义View合辑(8)-跳跃的小球(贝塞尔曲线)
为了加强对自定义 View 的认知以及开发能力,我计划这段时间陆续来完成几个难度从易到难的自定义 View,并简单的写几篇博客来进行介绍,所有的代码也都会开源,也希望读者能给个 star 哈 GitHub 地址:github.com/leavesC/…
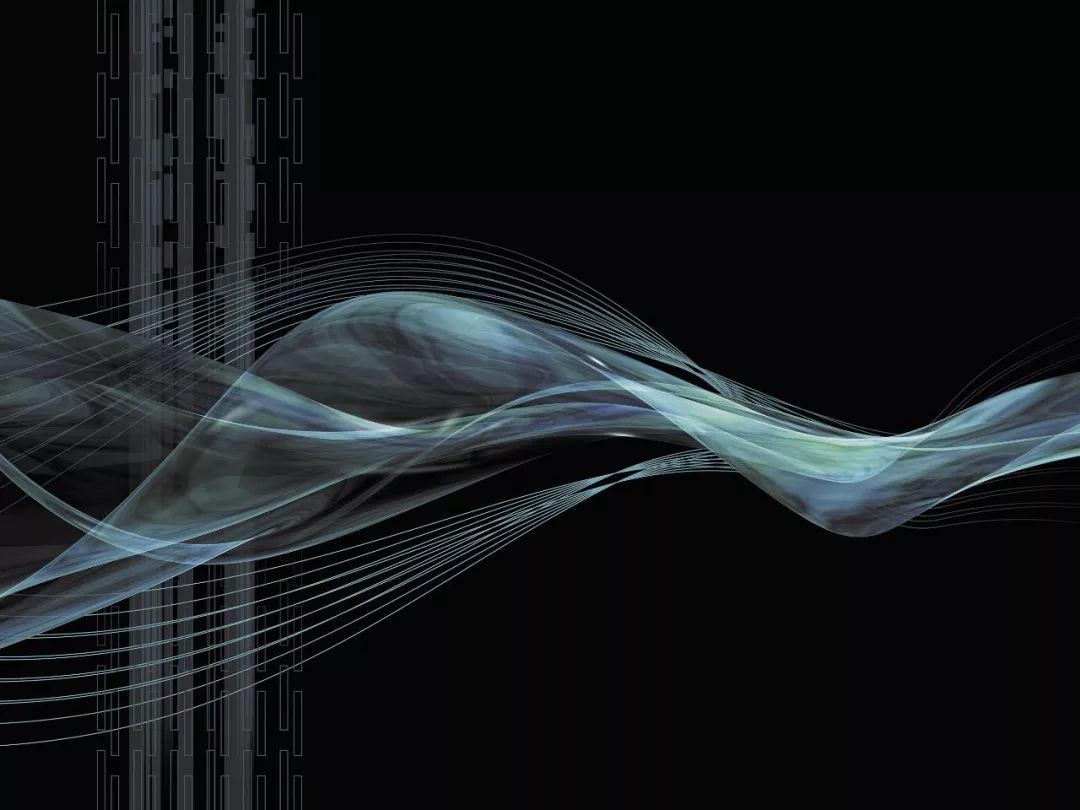
分析Booking的150种机器学习模型,我总结了六条成功经验
(图片付费下载自视觉中国)作者 | Adrian Colyer译者 | Monanfei出品 | AI科技大本营(ID:rgznai100)本文是一篇有趣的论文(150 successful machine learning models: 6 lessons learned at Booking.com Bernadi et al.,…

Android官方提供的支持不同屏幕大小的全部方法
2019独角兽企业重金招聘Python工程师标准>>> 本文将告诉你如何让你的应用程序支持各种不同屏幕大小,主要通过以下几种办法: 让你的布局能充分的自适应屏幕根据屏幕的配置来加载合适的UI布局确保正确的布局应用在正确的设备屏幕上提供可以根据…
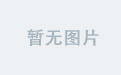
C++/C++11中头文件iterator的使用
<iterator>是C标准程序库中的一个头文件,定义了C STL标准中的一些迭代器模板类,这些类都是以std::iterator为基类派生出来的。迭代器提供对集合(容器)元素的操作能力。迭代器提供的基本操作就是访问和遍历。迭代器模拟了C中的指针,可以…
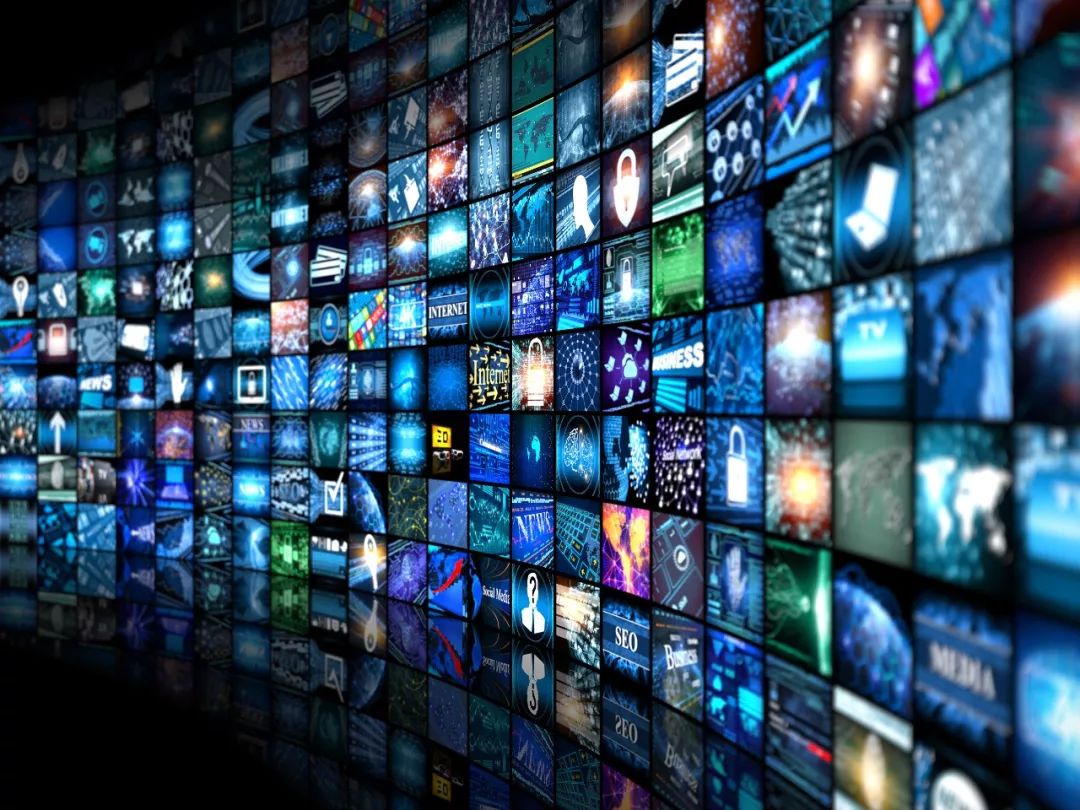
从多媒体技术演进看AI技术
(图片付费下载自视觉中国)文 / LiveVideoStack主编 包研在8月的LiveVideoStackCon2019北京开场致辞中,我分享了一组数据——把2019年和2017年两场LiveVideoStackCon上的AI相关的话题做了统计,这是数字从9.3%增长到31%,…
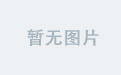
五. python的日历模块
一 .日历 import calendar# 日历模块# 使用# 返回指定某年某月的日历 print(calendar.month(2017,7))# July 2017 # Mo Tu We Th Fr Sa Su # 1 2 # 3 4 5 6 7 8 9 # 10 11 12 13 14 15 16 # 17 18 19 20 21 22 23 # 24 25 26 27 28 29 30 # 31# 返…

Linux下的Shell工作原理
为什么80%的码农都做不了架构师?>>> Linux系统提供给用户的最重要的系统程序是Shell命令语言解释程序。它不 属于内核部分,而是在核心之外,以用户态方式运行。其基本功能是解释并 执行用户打入的各种命令,实现用户与L…
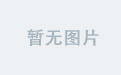
C++/C++11中头文件functional的使用
<functional>是C标准库中的一个头文件,定义了C标准中多个用于表示函数对象(function object)的类模板,包括算法操作、比较操作、逻辑操作;以及用于绑定函数对象的实参值的绑定器(binder)。这些类模板的实例是具有函数调用运算符(functi…
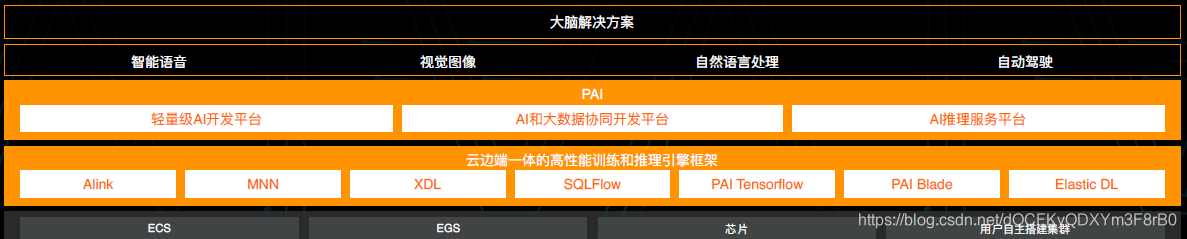
飞天AI平台到底哪里与众不同?听听它的架构者怎么说
采访嘉宾 | 林伟 整理 | 夕颜 出品 | AI科技大本营(ID:rgznai100) 天下没有不散的宴席。 9 月 25 日,云栖大会在云栖小镇开始,历经三天的技术盛宴,于 9 月 27 日的傍晚结束。 三天、全球6.7万人现场参会、超1250万人…
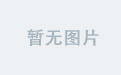
浅谈 sessionStorage、localStorage、cookie 的区别以及使用
1、sessionStorage、localStorage、cookie 之间的区别 相同点 cookie 和 webStorage 都是用来存储客户端的一些信息不同点 localStorage localStorage 的生命周期是 永久的。也就是说 只要不是 手动的去清除。localStorage 会一直存储 sessionStorage 相反 sessionStorage 的生…

任务栏窗口和状态图标的闪动 z
Demo程序: 实现任务栏窗体和图标的闪动: 整个程序是基于Windows Forms的,对于任务栏右下角状态图标的闪动,创建了一个类型:NotifyIconAnimator,基本上是包装了Windows Forms中的NotifyIcon类型,…
深度学习中的最大似然估计简介
统计领域为我们提供了很多工具来实现机器学习目标,不仅可以解决训练集上的任务,还可以泛化。例如参数估计、偏差和方差,对于正式地刻画泛化、欠拟合和过拟合都非常有帮助。点估计:点估计试图为一些感兴趣的量提供单个”最优”预测…
简单粗暴上手TensorFlow 2.0,北大学霸力作,必须人手一册!
(图片付费下载自视觉中国) 整理 | 夕颜 出品 | AI科技大本营(ID:rgznai100) 【导读】 TensorFlow 2.0 于近期正式发布后,立即受到学术界与科研界的广泛关注与好评。此前,AI 科技大本营曾特邀专家回顾了 Te…

常见运维漏洞-Rsync-Redis
转载于:https://blog.51cto.com/10945453/2394651

zabbix笔记
(1)转载于:https://blog.51cto.com/zlong37/1406441
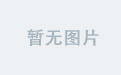
C++/C++11中头文件algorithm的使用
<algorithm>是C标准程序库中的一个头文件,定义了C STL标准中的基础性的算法(均为函数模板)。<algorithm>定义了设计用于元素范围的函数集合。任何对象序列的范围可以通过迭代器或指针访问。 std::adjacent_find:在序列中查找第一对相邻且值…
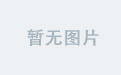
js filter 用法
filter filter函数可以看成是一个过滤函数,返回符合条件的元素的数组 filter需要在循环的时候判断一下是true还是false,是true才会返回这个元素; filter()接收的回调函数,其实可以有多个参数。通常我们仅使用第一个参数ÿ…
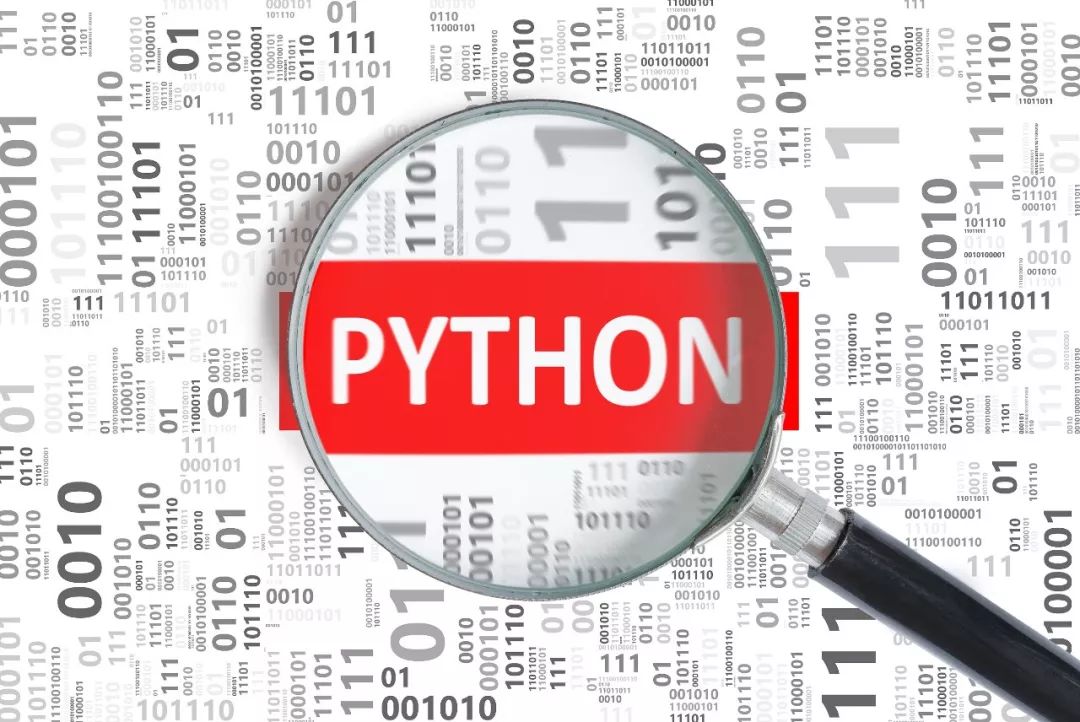
每30秒学会一个Python小技巧,GitHub星数4600+
(图片付费下载自视觉中国)作者 | xiaoyu,数据爱好者来源 | Python数据科学(ID:PyDataScience)很多学习Python的朋友在项目实战中会遇到不少功能实现上的问题,有些问题并不是很难的问题,或者已经…
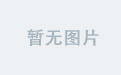
Nginx自定义模块编写:根据post参数路由到不同服务器
Nginx可以轻松实现根据不同的url 或者 get参数来转发到不同的服务器,然而当我们需要根据http包体来进行请求路由时,Nginx默认的配置规则就捉襟见肘了,但是没关系,Nginx提供了强大的自定义模块功能,我们只要进行需要的扩…
深度学习中的贝叶斯统计简介
贝叶斯用概率反映知识状态的确定性程度。数据集能够被直接观测到,因此不是随机的。另一方面,真实参数θ是未知或不确定的,因此可以表示成随机变量。在观察到数据前,我们将θ的已知知识表示成先验概率分布(prior probability distr…
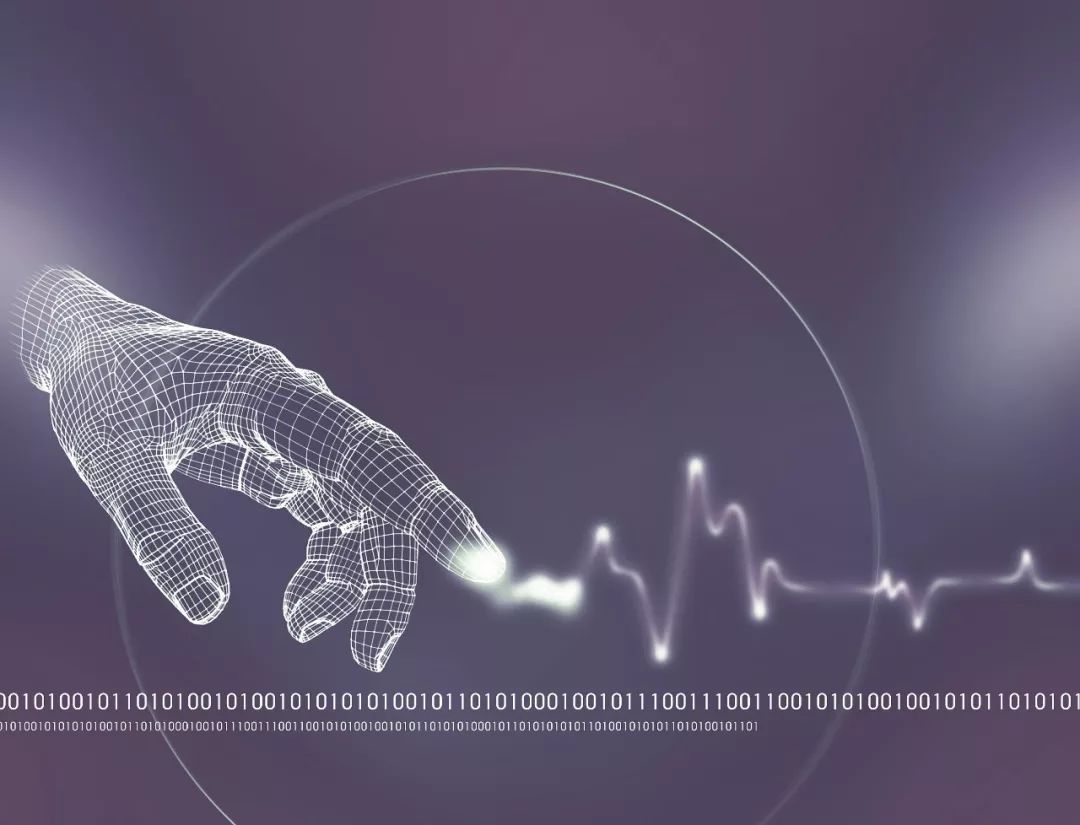
少走弯路:强烈推荐的TensorFlow快速入门资料(可下载)
(图片付费下载自视觉中国)作者 | 黄海广来源 | 机器学习初学者(ID: ai-start-com)知识更新非常快,需要一直学习才能跟上时代进步,举个例子:吴恩达老师在深度学习课上讲的TensorFlow使用…
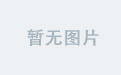
有状态bean与无状态bean
在学习bean的作用域的时候,了解了这个问题。 bean5种作用域:分别是:singleton、prototype、request、session、gloabal session 接下来就讲一下有状态bean与无状态bean: 有状态会话bean :每个用户有自己特有的一个实例…

从Developer Removed From Sale 回到可下载状态的方法
2019独角兽企业重金招聘Python工程师标准>>> 如果你不小心点了”Remove“ 按钮,App的状态会变成"Developer Removed From Sale ",这时,即使更新应用也无法改变这个状态。想要让App恢复可下载状态,你需要尝试…