iOS 跑马灯封装(带点击事件)
1.WAdvertScrollView.h
#import <UIKit/UIKit.h>
@class WAdvertScrollView;
typedef enum : NSUInteger {
/// 一行文字滚动样式
WAdvertScrollViewStyleNormal,
/// 二行文字滚动样式
WAdvertScrollViewStyleMore,
} WAdvertScrollViewStyle;
@protocol WAdvertScrollViewDelegate <NSObject>
/// delegate 方法
- (void)advertScrollView:(WAdvertScrollView *)advertScrollView didSelectedItemAtIndex:(NSInteger)index;
@end
@interface WAdvertScrollView : UIView
#pragma mark - - - 公共 API
/** delegate */
@property (nonatomic, weak) id<WAdvertScrollViewDelegate> delegate;
/** 默认 SGAdvertScrollViewStyleNormal 样式 */
@property (nonatomic, assign) WAdvertScrollViewStyle advertScrollViewStyle;
/** 滚动时间间隔,默认为3s */
@property (nonatomic, assign) CFTimeInterval scrollTimeInterval;
/** 标题字体字号,默认为13号字体 */
@property (nonatomic, strong) UIFont *titleFont;
#pragma mark - - - WAdvertScrollViewStyleNormal 样式下的 API
/** 左边标志图片数组 */
@property (nonatomic, strong) NSArray *signImages;
/** 标题数组 */
@property (nonatomic, strong) NSArray *titles;
/** 标题字体颜色,默认为黑色 */
@property (nonatomic, strong) UIColor *titleColor;
/** 标题文字位置,默认为 NSTextAlignmentLeft,仅仅针对标题起作用 */
@property (nonatomic, assign) NSTextAlignment textAlignment;
#pragma mark - - - WAdvertScrollViewStyleMore 样式下的 API
/** 顶部左边标志图片数组 */
@property (nonatomic, strong) NSArray *topSignImages;
/** 顶部标题数组 */
@property (nonatomic, strong) NSArray *topTitles;
/** 底部左边标志图片数组 */
@property (nonatomic, strong) NSArray *bottomSignImages;
/** 底部标题数组 */
@property (nonatomic, strong) NSArray *bottomTitles;
/** 顶部标题字体颜色,默认为黑色 */
@property (nonatomic, strong) UIColor *topTitleColor;
/** 底部标题字体颜色,默认为黑色 */
@property (nonatomic, strong) UIColor *bottomTitleColor;
@end
2.WAdvertScrollView.m
#import "WAdvertScrollView.h"
#import "UIImageView+WebCache.h"
static NSInteger const advertScrollViewTitleFont = 13;
#pragma mark - - - WAdvertScrollViewStyleNormal 样式下的 cell
@interface WAdvertScrollViewNormalCell : UICollectionViewCell
@property (nonatomic, strong) UIImageView *signImageView;
@property (nonatomic, strong) UILabel *titleLabel;
@end
@implementation WAdvertScrollViewNormalCell
- (instancetype)initWithFrame:(CGRect)frame {
if (self = [super initWithFrame:frame]) {
self.backgroundColor = [UIColor clearColor];
[self.contentView addSubview:self.signImageView];
[self.contentView addSubview:self.titleLabel];
}
return self;
}
- (void)layoutSubviews {
[super layoutSubviews];
CGFloat spacing = 5;
CGFloat signImageViewW = self.signImageView.image.size.width;
CGFloat signImageViewH = self.signImageView.image.size.height;
CGFloat signImageViewX = 0;
CGFloat signImageViewY = 0;
self.signImageView.frame = CGRectMake(signImageViewX, signImageViewY, signImageViewW, signImageViewH);
CGFloat labelX = 0;
if (self.signImageView.image == nil) {
labelX = 0;
} else {
labelX = CGRectGetMaxX(self.signImageView.frame) + 0.5 * spacing;
}
CGFloat labelY = 0;
CGFloat labelW = self.frame.size.width - labelX;
CGFloat labelH = self.frame.size.height;
self.titleLabel.frame = CGRectMake(labelX, labelY, labelW, labelH);
CGPoint topPoint = self.signImageView.center;
topPoint.y = _titleLabel.center.y;
_signImageView.center = topPoint;
}
- (UIImageView *)signImageView {
if (!_signImageView) {
_signImageView = [[UIImageView alloc] init];
}
return _signImageView;
}
- (UILabel *)titleLabel {
if (!_titleLabel) {
_titleLabel = [[UILabel alloc] init];
_titleLabel.textColor = [UIColor blackColor];
_titleLabel.font = [UIFont systemFontOfSize:advertScrollViewTitleFont];
}
return _titleLabel;
}
@end
#pragma mark - - - WAdvertScrollViewStyleMore 样式下的 cell
@interface WAdvertScrollViewMoreCell : UICollectionViewCell
@property (nonatomic, strong) UIImageView *topSignImageView;
@property (nonatomic, strong) UILabel *topLabel;
@property (nonatomic, strong) UIImageView *bottomSignImageView;
@property (nonatomic, strong) UILabel *bottomLabel;
@end
@implementation WAdvertScrollViewMoreCell
- (instancetype)initWithFrame:(CGRect)frame {
if (self = [super initWithFrame:frame]) {
self.backgroundColor = [UIColor clearColor];
[self.contentView addSubview:self.topSignImageView];
[self.contentView addSubview:self.topLabel];
[self.contentView addSubview:self.bottomSignImageView];
[self.contentView addSubview:self.bottomLabel];
}
return self;
}
- (void)layoutSubviews {
[super layoutSubviews];
CGFloat spacing = 5;
CGFloat topSignImageViewW = self.topSignImageView.image.size.width;
CGFloat topSignImageViewH = self.topSignImageView.image.size.height;
CGFloat topSignImageViewX = 0;
CGFloat topSignImageViewY = spacing;
self.topSignImageView.frame = CGRectMake(topSignImageViewX, topSignImageViewY, topSignImageViewW, topSignImageViewH);
CGFloat topLabelX = 0;
if (self.topSignImageView.image == nil) {
topLabelX = 0;
} else {
topLabelX = CGRectGetMaxX(self.topSignImageView.frame) + 0.5 * spacing;
}
CGFloat topLabelY = topSignImageViewY;
CGFloat topLabelW = self.frame.size.width - topLabelX;
CGFloat topLabelH = 0.5 * (self.frame.size.height - 2 * topLabelY);
self.topLabel.frame = CGRectMake(topLabelX, topLabelY, topLabelW, topLabelH);
CGPoint topPoint = self.topSignImageView.center;
topPoint.y = _topLabel.center.y;
_topSignImageView.center = topPoint;
CGFloat bottomSignImageViewW = self.bottomSignImageView.image.size.width;
CGFloat bottomSignImageViewH = self.bottomSignImageView.image.size.height;
CGFloat bottomSignImageViewX = 0;
CGFloat bottomSignImageViewY = CGRectGetMaxY(self.topLabel.frame);
self.bottomSignImageView.frame = CGRectMake(bottomSignImageViewX, bottomSignImageViewY, bottomSignImageViewW, bottomSignImageViewH);
CGFloat bottomLabelX = 0;
if (self.bottomSignImageView.image == nil) {
bottomLabelX = 0;
} else {
bottomLabelX = CGRectGetMaxX(self.bottomSignImageView.frame) + 0.5 * spacing;
}
CGFloat bottomLabelY = CGRectGetMaxY(self.topLabel.frame);
CGFloat bottomLabelW = self.frame.size.width - bottomLabelX;
CGFloat bottomLabelH = topLabelH;
self.bottomLabel.frame = CGRectMake(bottomLabelX, bottomLabelY, bottomLabelW, bottomLabelH);
CGPoint bottomPoint = self.bottomSignImageView.center;
bottomPoint.y = _bottomLabel.center.y;
_bottomSignImageView.center = bottomPoint;
}
- (UIImageView *)topSignImageView {
if (!_topSignImageView) {
_topSignImageView = [[UIImageView alloc] init];
}
return _topSignImageView;
}
- (UILabel *)topLabel {
if (!_topLabel) {
_topLabel = [[UILabel alloc] init];
_topLabel.textColor = [UIColor blackColor];
_topLabel.font = [UIFont systemFontOfSize:advertScrollViewTitleFont];
}
return _topLabel;
}
- (UIImageView *)bottomSignImageView {
if (!_bottomSignImageView) {
_bottomSignImageView = [[UIImageView alloc] init];
}
return _bottomSignImageView;
}
- (UILabel *)bottomLabel {
if (!_bottomLabel) {
_bottomLabel = [[UILabel alloc] init];
_bottomLabel.textColor = [UIColor blackColor];
_bottomLabel.font = [UIFont systemFontOfSize:advertScrollViewTitleFont];
}
return _bottomLabel;
}
@end
#pragma mark - - - WAdvertScrollView
@interface WAdvertScrollView () <UICollectionViewDelegate, UICollectionViewDataSource>
@property (nonatomic, strong) UICollectionViewFlowLayout *flowLayout;
@property (nonatomic, strong) UICollectionView *collectionView;
@property (nonatomic, strong) NSTimer *timer;
@property (nonatomic, strong) NSArray *titleArr;
@property (nonatomic, strong) NSArray *imageArr;
@property (nonatomic, strong) NSArray *bottomImageArr;
@property (nonatomic, strong) NSArray *bottomTitleArr;
@end
@implementation WAdvertScrollView
static NSInteger const advertScrollViewMaxSections = 100;
static NSString *const advertScrollViewNormalCell = @"advertScrollViewNormalCell";
static NSString *const advertScrollViewMoreCell = @"advertScrollViewMoreCell";
- (void)awakeFromNib {
[super awakeFromNib];
[self initialization];
[self setupSubviews];
}
- (instancetype)initWithFrame:(CGRect)frame {
if (self = [super initWithFrame:frame]) {
self.backgroundColor = [UIColor whiteColor];
[self initialization];
[self setupSubviews];
}
return self;
}
- (void)willMoveToSuperview:(UIView *)newSuperview {
if (!newSuperview) {
[self removeTimer];
}
}
- (void)dealloc {
_collectionView.delegate = nil;
_collectionView.dataSource = nil;
}
- (void)initialization {
_scrollTimeInterval = 3.0;
[self addTimer];
_advertScrollViewStyle = WAdvertScrollViewStyleNormal;
}
- (void)setupSubviews {
UIView *tempView = [[UIView alloc] initWithFrame:CGRectZero];
[self addSubview:tempView];
[self addSubview:self.collectionView];
}
- (UICollectionView *)collectionView {
if (!_collectionView) {
_flowLayout = [[UICollectionViewFlowLayout alloc] init];
_flowLayout.minimumLineSpacing = 0;
_collectionView = [[UICollectionView alloc] initWithFrame:self.bounds collectionViewLayout:_flowLayout];
_collectionView.delegate = self;
_collectionView.dataSource = self;
_collectionView.scrollsToTop = NO;
_collectionView.scrollEnabled = NO;
_collectionView.pagingEnabled = YES;
_collectionView.showsVerticalScrollIndicator = NO;
_collectionView.backgroundColor = [UIColor clearColor];
[_collectionView registerClass:[WAdvertScrollViewNormalCell class] forCellWithReuseIdentifier:advertScrollViewNormalCell];
}
return _collectionView;
}
- (void)layoutSubviews {
[super layoutSubviews];
_flowLayout.itemSize = CGSizeMake(self.frame.size.width, self.frame.size.height);
_collectionView.frame = self.bounds;
if (self.titleArr.count > 1) {
[self defaultSelectedScetion];
}
}
- (void)defaultSelectedScetion {
[self.collectionView scrollToItemAtIndexPath:[NSIndexPath indexPathForItem:0 inSection:0.5 * advertScrollViewMaxSections] atScrollPosition:UICollectionViewScrollPositionBottom animated:NO];
}
#pragma mark - - - UICollectionView 的 dataSource、delegate方法
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView {
return advertScrollViewMaxSections;
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return self.titleArr.count;
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
if (self.advertScrollViewStyle == WAdvertScrollViewStyleMore) {
WAdvertScrollViewMoreCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:advertScrollViewMoreCell forIndexPath:indexPath];
NSInteger topImagesCount = self.imageArr.count;
if (topImagesCount > 0) {
NSString *topImagePath = self.imageArr[indexPath.item];
if (topImagePath == nil || [topImagePath isEqualToString:@""]) { // 解决 iOS 11 无图片,控制台打印问题
if ([topImagePath hasPrefix:@"http"]) {
[cell.topSignImageView sd_setImageWithURL:[NSURL URLWithString:@"www.kingsic22.com"]];
} else {
cell.topSignImageView.image = [UIImage imageNamed:@"kingsic"];
}
} else {
if ([topImagePath hasPrefix:@"http"]) {
[cell.topSignImageView sd_setImageWithURL:[NSURL URLWithString:topImagePath]];
} else {
cell.topSignImageView.image = [UIImage imageNamed:topImagePath];
}
}
}
cell.topLabel.text = self.titleArr[indexPath.item];
NSInteger bottomImagesCount = self.bottomImageArr.count;
if (bottomImagesCount > 0) {
NSString *bottomImagePath = self.bottomImageArr[indexPath.item];
if (bottomImagePath == nil || [bottomImagePath isEqualToString:@""]) { // 解决 iOS 11 无图片,控制台打印问题
if ([bottomImagePath hasPrefix:@"http"]) {
[cell.bottomSignImageView sd_setImageWithURL:[NSURL URLWithString:@"www.kingsic22.com"]];
} else {
cell.bottomSignImageView.image = [UIImage imageNamed:@"kingsic"];
}
} else {
if ([bottomImagePath hasPrefix:@"http"]) {
[cell.bottomSignImageView sd_setImageWithURL:[NSURL URLWithString:bottomImagePath]];
} else {
cell.bottomSignImageView.image = [UIImage imageNamed:bottomImagePath];
}
}
}
cell.bottomLabel.text = self.bottomTitleArr[indexPath.item];
if (self.titleFont) {
cell.topLabel.font = self.titleFont;
cell.bottomLabel.font = self.titleFont;
}
if (self.topTitleColor) {
cell.topLabel.textColor = self.topTitleColor;
}
if (self.bottomTitleColor) {
cell.bottomLabel.textColor = self.bottomTitleColor;
}
return cell;
} else {
WAdvertScrollViewNormalCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:advertScrollViewNormalCell forIndexPath:indexPath];
NSInteger imagesCount = self.imageArr.count;
if (imagesCount > 0) {
NSString *imagePath = self.imageArr[indexPath.item];
if (imagePath == nil || [imagePath isEqualToString:@""]) { // 解决 iOS 11 无图片,控制台打印问题
if ([imagePath hasPrefix:@"http"]) {
[cell.signImageView sd_setImageWithURL:[NSURL URLWithString:@"www.kingsic22.com"]];
} else {
cell.signImageView.image = [UIImage imageNamed:@"kingsic"];
}
} else {
if ([imagePath hasPrefix:@"http"]) {
[cell.signImageView sd_setImageWithURL:[NSURL URLWithString:imagePath]];
} else {
cell.signImageView.image = [UIImage imageNamed:imagePath];
}
}
}
cell.titleLabel.text = self.titleArr[indexPath.item];
if (self.textAlignment) {
cell.titleLabel.textAlignment = self.textAlignment;
}
if (self.titleFont) {
cell.titleLabel.font = self.titleFont;
}
if (self.titleColor) {
cell.titleLabel.textColor = self.titleColor;
}
return cell;
}
}
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath {
if (self.delegate && [self.delegate respondsToSelector:@selector(advertScrollView:didSelectedItemAtIndex:)]) {
[self.delegate advertScrollView:self didSelectedItemAtIndex:indexPath.item];
}
}
#pragma mark - - - NSTimer
- (void)addTimer {
[self removeTimer];
self.timer = [NSTimer timerWithTimeInterval:self.scrollTimeInterval target:self selector:@selector(beginUpdateUI) userInfo:nil repeats:YES];
[[NSRunLoop mainRunLoop] addTimer:_timer forMode:NSRunLoopCommonModes];
}
- (void)removeTimer {
[_timer invalidate];
_timer = nil;
}
- (void)beginUpdateUI {
if (self.titleArr.count == 0) return;
// 1、当前正在展示的位置
NSIndexPath *currentIndexPath = [[self.collectionView indexPathsForVisibleItems] lastObject];
// 马上显示回最中间那组的数据
NSIndexPath *resetCurrentIndexPath = [NSIndexPath indexPathForItem:currentIndexPath.item inSection:0.5 * advertScrollViewMaxSections];
[self.collectionView scrollToItemAtIndexPath:resetCurrentIndexPath atScrollPosition:UICollectionViewScrollPositionBottom animated:NO];
// 2、计算出下一个需要展示的位置
NSInteger nextItem = resetCurrentIndexPath.item + 1;
NSInteger nextSection = resetCurrentIndexPath.section;
if (nextItem == self.titleArr.count) {
nextItem = 0;
nextSection++;
}
NSIndexPath *nextIndexPath = [NSIndexPath indexPathForItem:nextItem inSection:nextSection];
// 3、通过动画滚动到下一个位置
[self.collectionView scrollToItemAtIndexPath:nextIndexPath atScrollPosition:UICollectionViewScrollPositionBottom animated:YES];
}
#pragma mark - - - setting
- (void)setAdvertScrollViewStyle:(WAdvertScrollViewStyle)advertScrollViewStyle {
_advertScrollViewStyle = advertScrollViewStyle;
if (advertScrollViewStyle == WAdvertScrollViewStyleMore) {
_advertScrollViewStyle = WAdvertScrollViewStyleMore;
[_collectionView registerClass:[WAdvertScrollViewMoreCell class] forCellWithReuseIdentifier:advertScrollViewMoreCell];
}
}
- (void)setSignImages:(NSArray *)signImages {
_signImages = signImages;
if (signImages) {
self.imageArr = [NSArray arrayWithArray:signImages];
}
}
- (void)setTitles:(NSArray *)titles {
_titles = titles;
if (titles.count > 1) {
[self addTimer];
} else {
[self removeTimer];
}
self.titleArr = [NSArray arrayWithArray:titles];
[self.collectionView reloadData];
}
- (void)setTitleFont:(UIFont *)titleFont {
_titleFont = titleFont;
}
- (void)setTextAlignment:(NSTextAlignment)textAlignment {
_textAlignment = textAlignment;
}
- (void)setTopSignImages:(NSArray *)topSignImages {
_topSignImages = topSignImages;
if (topSignImages) {
self.imageArr = [NSArray arrayWithArray:topSignImages];
}
}
- (void)setTopTitles:(NSArray *)topTitles {
_topTitles = topTitles;
if (topTitles.count > 1) {
[self addTimer];
} else {
[self removeTimer];
}
self.titleArr = [NSArray arrayWithArray:topTitles];
[self.collectionView reloadData];
}
- (void)setBottomSignImages:(NSArray *)bottomSignImages {
_bottomSignImages = bottomSignImages;
if (bottomSignImages) {
self.bottomImageArr = [NSArray arrayWithArray:bottomSignImages];
}
}
- (void)setBottomTitles:(NSArray *)bottomTitles {
_bottomTitles = bottomTitles;
if (bottomTitles) {
self.bottomTitleArr = [NSArray arrayWithArray:bottomTitles];
}
}
- (void)setScrollTimeInterval:(CFTimeInterval)scrollTimeInterval {
_scrollTimeInterval = scrollTimeInterval;
if (scrollTimeInterval) {
[self addTimer];
}
}
@end
3.调用
切记 遵循代理WAdvertScrollViewDelegate
self.titleScrollView = [[WAdvertScrollView alloc] initWithFrame:CGRectMake(imagView.frame.size.width + 10, 0, self.titleView.frame.size.width - (imagView.frame.size.width + 20), 40)];
self.titleScrollView.advertScrollViewStyle = WAdvertScrollViewStyleMore;
self.titleScrollView.titleColor = [[UIColor blackColor] colorWithAlphaComponent:0.7];
self.titleScrollView.scrollTimeInterval = 5;
self.titleScrollView.titles = @[@"", @"", @""];
self.titleScrollView.titleFont = [UIFont systemFontOfSize:14];
self.titleScrollView.delegate = self;
self.titleScrollView.layer.cornerRadius = 20;
self.titleScrollView.layer.masksToBounds = YES;
self.titleScrollView.backgroundColor = [UIColor whiteColor];
[self.titleView addSubview:self.titleScrollView];
4.代理方法
/// 代理方法
- (void)advertScrollView:(WAdvertScrollView *)advertScrollView didSelectedItemAtIndex:(NSInteger)index {
NSLog(@"点击跑马灯");
}
相关文章:
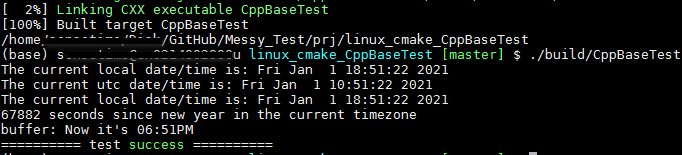
日期与unix时间戳之间的转换C++实现
之前在https://blog.csdn.net/fengbingchun/article/details/107023645 中介绍过gmtime和localtime的区别,这里介绍下日期与Unix时间戳之间转换的实现,其中也会用到这两个函数。 Unix时间戳(Unix timestamp):是一种时间表示方式,…
模型训练完才是业务的开始?说说模型监控 | CSDN博文精选
扫码参与CSDN“原力计划”作者 | A字头来源 | 数据札记倌(ID:Data_Groom)“模型训练结束后才是业务真正的开始”简述每次模型训练完成后,并不意味着项目的结束,在训练模型后,我们还需要将其稳定上线,然后部署一套相应的监控体系&a…
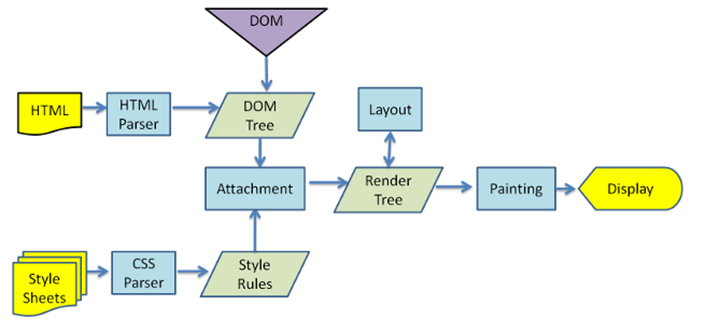
后端码农谈前端(CSS篇)第一课:CSS概述
一、从扮演浏览器开始 扮演浏览器是Head First图书中很有意义的一个环节。可作者忘记了告诉我们扮演浏览器的台本。我们从这里开始。 上图是webkit内核渲染html和css的流程图。从该图我们可以知道以下几个关键信息: HTML的解析过程和CSS的解析过程是独立完成的。HTM…
远场语音识别错误率降低30%,百度提基于复数CNN网络的新技术
【12月公开课预告】,入群直接获取报名地址12月11日晚8点直播主题:人工智能消化道病理辅助诊断平台——从方法到落地12月12日晚8点直播:利用容器技术打造AI公司技术中台12月17日晚8点直播主题:可重构计算:能效比、通用性…
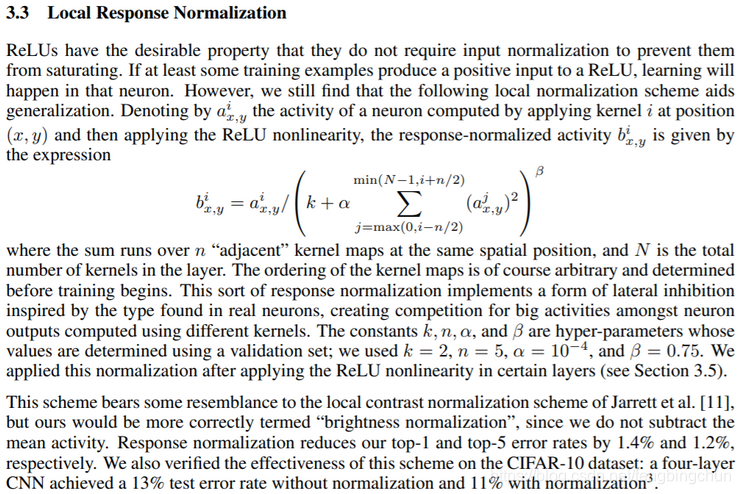
深度神经网络中的局部响应归一化LRN简介及实现
Alex、Hinton等人在2012年的NIPS论文《ImageNet Classification with Deep Convolutional Neural Networks》中将LRN应用于深度神经网络中(AlexNet)。论文见:http://www.cs.toronto.edu/~hinton/absps/imagenet.pdf ,截图如下: 公式解释&…
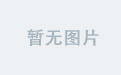
iOS 被拒解析
原因: Your app uses the "prefs:root" non-public URL scheme, which is a private entity. The use of non-public APIs is not permitted on the App Store because it can lead to a poor user experience should these APIs change.Continuing to us…
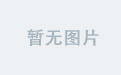
MSSQL数据库统计所有表的记录数
今天需要筛选出来库中行数不为零的表,于是动手写下了如下存储过程。 CREATE PROCEDURE TableCount AS BEGIN SET NOCOUNT ON DECLARE t1 AS TABLE(id INT IDENTITY,NAME NVARCHAR(50),RowsCount INT) DECLARE indexid AS INT DECLARE maxid AS INT DECLARE count A…
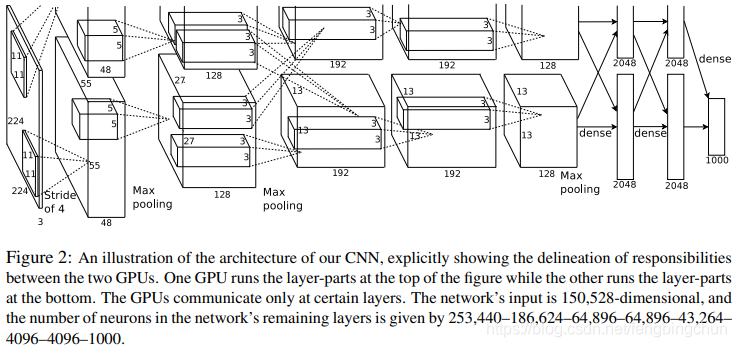
经典网络AlexNet介绍
AlexNet经典网络由Alex Krizhevsky、Hinton等人在2012年提出,发表在NIPS,论文名为《ImageNet Classification with Deep Convolutional Neural Networks》,论文见:http://www.cs.toronto.edu/~hinton/absps/imagenet.pdf …
微软张若非:搜索引擎和广告系统,那些你所不知的AI落地技术
【12月公开课预告】,入群直接获取报名地址12月11日晚8点直播主题:人工智能消化道病理辅助诊断平台——从方法到落地12月12日晚8点直播:利用容器技术打造AI公司技术中台12月17日晚8点直播主题:可重构计算:能效比、通用性…
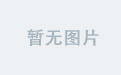
iOS 之 IQKeyboardManager 解决使用UITableView 界面上移问题
- (void)viewWillAppear:(BOOL)animated {[IQKeyboardManager sharedManager].enable NO;}- (void)viewWillDisappear:(BOOL)animated{[super viewWillDisappear:animated];[IQKeyboardManager sharedManager].enable YES; }
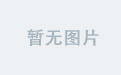
excel增加上一列的数值(日期)
TEXT(D2-1,"m月d日") 有年的话就是 TEXT(D2-1,"yyyy年m月d日") D2就是参照日期转载于:https://www.cnblogs.com/hont/p/4352877.html
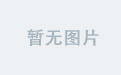
iOS 一些基础的方法
iOS button字体居中等的设置 self.replyBtn.contentHorizontalAlignment UIControlContentHorizontalAlignmentCenter; UIControlContentHorizontalAlignmentCenter 0, UIControlContentHorizontalAlignmentLeft 1, UIControlContentHorizontalAlignmentRight 2…
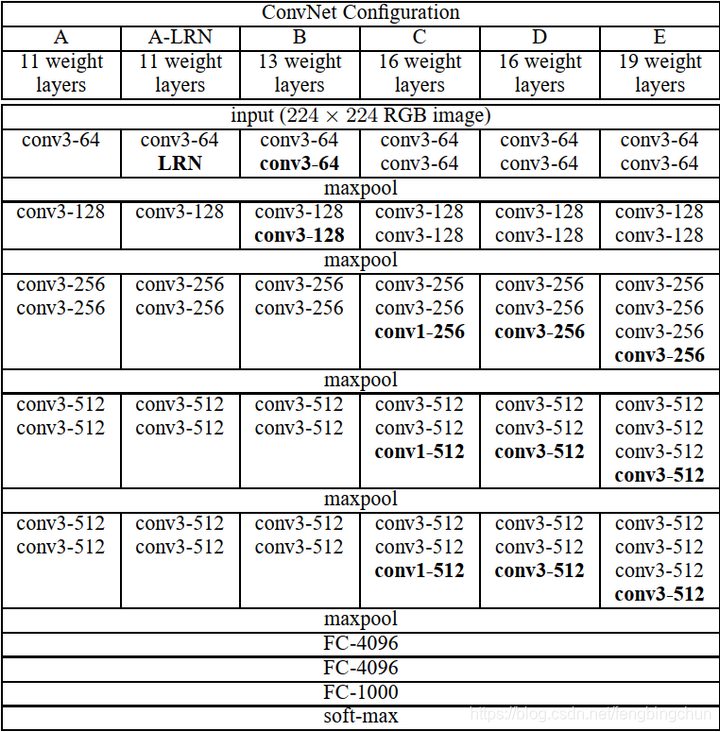
经典网络VGGNet介绍
经典网络VGGNet(其中VGG为Visual Geometry Group)由Karen Simonyan等于2014年提出,论文名为《Very Deep Convolutional Networks for Large-Scale Image Recognition》,论文见:https://arxiv.org/pdf/1409.1556.pdf,网络结构如下图…
70行Go代码打败C
【12月公开课预告】,入群直接获取报名地址12月11日晚8点直播主题:人工智能消化道病理辅助诊断平台——从方法到落地12月12日晚8点直播:利用容器技术打造AI公司技术中台12月17日晚8点直播主题:可重构计算:能效比、通用性…
Android开源框架ImageLoader的完美例子
要使用ImageLoader就要到这里下载jar包: https://github.com/nostra13/Android-Universal-Image-Loader 然后导入项目中去就行了 项目文档结构图: 从界面说起,界面本身是没什么好说的,就是如何在xml当中进行定义罢了 有以下这么多…
“掘金”金融AI落地,英特尔趟出一套通关攻略
有人说,金融业是最大的AI应用场景,但不管怎样,不可否认的事实是金融业已经从数字化走向AI化。某种程度上,AI与金融业有着天然的契合性:其一,金融业本身就是以数据为基本元素的行业,它为AI的模型…
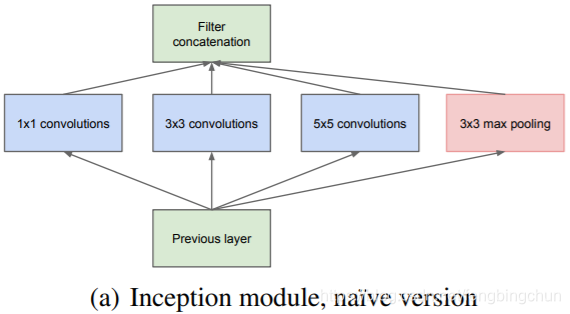
深度神经网络中的Inception模块介绍
深度神经网络(Deep Neural Networks, DNN)或深度卷积网络中的Inception模块是由Google的Christian Szegedy等人提出,包括Inception-v1、Inception-v2、Inception-v3、Inception-v4及Inception-ResNet系列。每个版本均是对其前一个版本的迭代改进。另外,依…
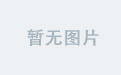
iOS隐藏导航栏的方法
- (void)viewWillAppear:(BOOL)animated { [super viewWillAppear:animated]; [self.navigationController setNavigationBarHidden:YES animated:animated]; }
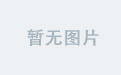
NEW关键字的三种用法
声明:本文最初是本人从他出转载到51CTO上的一篇文章,但现在记不清最初从出处了,原文作者看到还请原来,现在发表在这里只为学习,本人在51CTO的该文章的地址为:http://kestrelsaga.blog.51cto.com/3015222/75…
论文解读 | 微信看一看实时Look-alike推荐算法
作者丨gongyouliu编辑丨lily来源 | 授权转载自大数据与人工智能(ID:ai-big-data)微信看一看的精选文章推荐(见下面图1)大家应该都用过,微信团队在今年发表了一篇文章来专门介绍精选推荐的算法实现细节(Real-time Attention based Look-alike Model,简称R…

经典网络GoogLeNet介绍
经典网络GoogLeNet由Christian Szegedy等于2014年提出,论文名为《Going deeper with convolutions》,论文见:https://arxiv.org/pdf/1409.4842v1.pdf GoogLeNet网络用到了Inception-v1模块,关于Inception模块的介绍可以参考&…
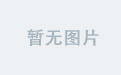
iOS webview 点击按钮返回上一页面或者返回首页
- (void)floatBtn:(UIButton *)sender { NSLog("点击"); if ([self.webView canGoBack]) { [self.webView goBack]; } else{ [self.view resignFirstResponder]; [self.navigationController popViewControllerAnimate…

centos6.6 Kickstart无人值守安装(一):原理篇
为什么80%的码农都做不了架构师?>>> #为什么要自动化无人值守安装? 偷懒……nb……zb……geekno no no 瞬间完成大规模机器部署,提高生产力,节省时间精力,为公司谋取更多利益,实现社会和谐!#怎…
拿来就能用!如何用 AI 算法提高安全运维效率?
作者 | 黄龙责编 | 伍杏玲来源 | CSDN(ID:CSDNnews) 在整个安全工作中,安全运维是不可或缺的一环,其目的是保证各项安全工作持续有效地运作。除了对外的沟通和业务对接相关工作,大部分安全运维的日常工作相…
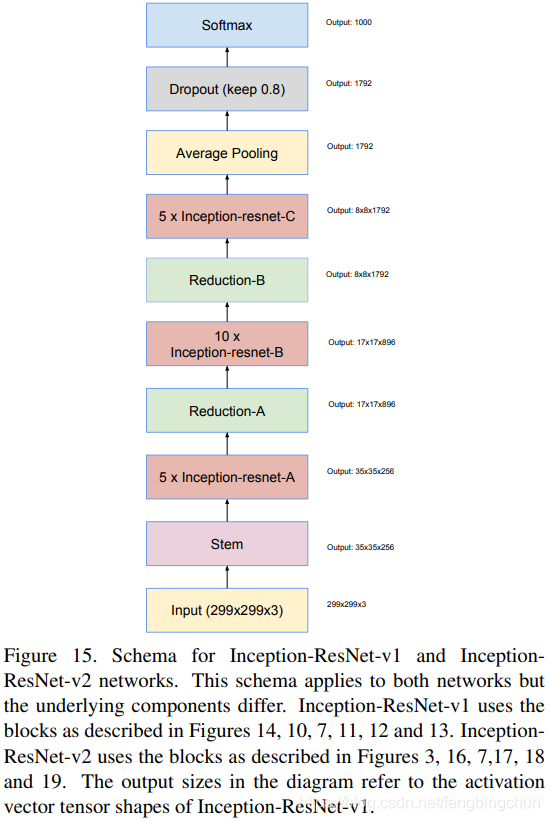
深度神经网络中Inception-ResNet模块介绍
之前在https://blog.csdn.net/fengbingchun/article/details/113482036 介绍了Inception,在https://blog.csdn.net/fengbingchun/article/details/114167581 介绍了ResNet,这里介绍下深度神经网络中的Inception-ResNet模块。 介绍Inception-ResNet的论文…
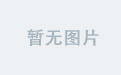
iOS 让UIView的左上角和右上角为圆角
-(UIView *)platFormBGV{ if (!_platFormBGV) { _platFormBGV [[UIView alloc] init]; _platFormBGV.backgroundColor [UIColor whiteColor]; _platFormBGV.frame CGRectMake(0, self.view.frame.size.height, APP_WIDTH, 220); // 左上和右上为圆角 UIBezierPath *cornerRa…
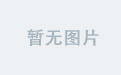
HttpUnit学习笔记
HttpUnit 能模拟浏览器的动作,如提交表单、JavaScript执行、基本HTTP认证、cookies建立以及自己主动页面重定向,通过编写代码能够处理取回来的文本、XML DOM或表单、表、链接。当与Junit等框架结合时,就能很easy地进行一个站点的功能測试了。…
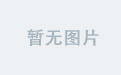
C++11中头文件type_traits介绍
C11中的头文件type_traits定义了一系列模板类,在编译期获得某一参数、某一变量、某一个类等等类型信息,主要做静态检查。 此头文件包含三部分: (1).Helper类:帮助创建编译时常量的标准模板类。介绍见以下测试代码: …
反季大清仓,最低仅需34.9元
不知不觉已经12月份了还有一个月就要过年啦很多地方已经进入了寒冬的季节有的地方已经开启了下雪模式纷纷开始买冬天的商品棉衣、羽绒服、取暖器......但是.......今天我是来搞反季清仓的快来看看今天的反季清仓有啥商品~●反季清仓商品—程序员专属定制T ●专属定制T_shirt&am…
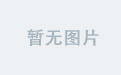
iOS 预览word pdf 文件
此类用于改变QLPreviewController 导航栏title #import <QuickLook/QuickLook.h> NS_ASSUME_NONNULL_BEGIN interface QLPreviewController (title) property (nonatomic, strong) NSString *qlpTitle; end NS_ASSUME_NONNULL_END #import "QLPreviewControllertitl…