SpringBoot conditional注解和自定义conditional注解使用
conditional注解是Springboot starter的基石,自动装配的时候会根据条件确定是否需要注入这个类。
含义:基于条件的注解。
作用:根据是否满足某个特定条件来决定是否创建某个特定的Bean。
意义:Springboot实现自动配置的关键基础能力。
@Conditional相关注解
@ConditionalOnBean:仅仅在当前上下文中存在某个对象时,才会实例化一个Bean
@ConditionalOnClass:某个class位于类路径上才会实例化一个Bean
@ConditionalOnExpression:当表达式为true的时候,才会实例化一个Bean
@ConditionalOnMissingBean:仅仅在当前上下文中不存在某个对象时,才会实例化一个Bean
@ConditionalOnMissingClass:某个class不位于类路径上才会实例化一个Bean
@ConditionalOnNotWebApplication:不是web应用
配置文件有这个配置
spring.data.mongodb.uri=mongodb://paopaoedu:paopaoedu@localhost:27017/paopaoedu
判断有这个配置才注入这个类
package com.paopaoedu.springboot.condition;import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.stereotype.Component;@Component
@ConditionalOnProperty("spring.data.mongodb.uri")
public class ConditionalTest {
}
测试文件
package com.paopaoedu.springboot;import com.paopaoedu.springboot.condition.ConditionalTest;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.BeansException;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.test.context.junit4.SpringRunner;@RunWith(SpringRunner.class)
@SpringBootTest(classes = {SpringBootLearningApplication.class})
//不用类似new ClassPathXmlApplicationContext()的方式,从已有的spring上下文取得已实例化的bean。通过ApplicationContextAware接口进行实现。
public class SpringBootLearningApplicationTests implements ApplicationContextAware {private ApplicationContext applicationContext;@Overridepublic void setApplicationContext(ApplicationContext context) throws BeansException {applicationContext = context;}@Testpublic void testA() {System.out.println("testA>>>"+applicationContext.getBean(ConditionalTest.class));}}
关于ApplicationContextAware使用理解参考https://www.jianshu.com/p/4c0723615a52
可以看出这个ConditionalTest被注入了:
如果你去掉这个配置测试用例就会报错找不到这个bean:
自定义conditional注解实现
自定义注解引入condition接口实现类
@Target({ ElementType.TYPE, ElementType.METHOD })
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Conditional(MyCondition.class)
public @interface MyConditionAnnotation {String[] value() default {};
}
实现一个自定义注解
实现Condition接口重写matches方法,符合条件返回true
public class MyCondition implements Condition {@Overridepublic boolean matches(ConditionContext context, AnnotatedTypeMetadata metadata) {String[] properties = (String[])metadata.getAnnotationAttributes("com.paopaoedu.springboot.condition.MyConditionAnnotation").get("value");for (String property : properties) {if (StringUtils.isEmpty(context.getEnvironment().getProperty(property))) {return false;}}return true;}
}
引入conditional注解
@Component
@MyConditionAnnotation({"spring.redis.master.host", "spring.redis.follow.host"})
public class ConditionalTest2 {
}
配置文件
# Redis服务器地址
spring.redis.master.host=r-xxx1.redis.rds.aliyuncs.com
# Redis服务器连接端口
spring.redis.master.port=6379
# Redis服务器连接密码(默认为空)
spring.redis.master.password=# Redis服务器地址
spring.redis.follow.host=r-xxx2.redis.rds.aliyuncs.com
# Redis服务器连接端口
spring.redis.follow.port=6379
# Redis服务器连接密码(默认为空)
spring.redis.follow.password=
修改测试用例
@RunWith(SpringRunner.class)
@SpringBootTest(classes = {SpringBootLearningApplication.class})
//不用类似new ClassPathXmlApplicationContext()的方式,从已有的spring上下文取得已实例化的bean。通过ApplicationContextAware接口进行实现。
public class SpringBootLearningApplicationTests implements ApplicationContextAware {private ApplicationContext applicationContext;@Overridepublic void setApplicationContext(ApplicationContext context) throws BeansException {applicationContext = context;}@Testpublic void testA() {System.out.println("testA>>>"+applicationContext.getBean(ConditionalTest.class));System.out.println("testA>>>"+applicationContext.getBean(ConditionalTest2.class));}}
输出
重要的调试断点ClassPathScanningCandidateComponentProvider.scanCandidateComponents()
private Set<BeanDefinition> scanCandidateComponents(String basePackage) {Set<BeanDefinition> candidates = new LinkedHashSet<>();try {String packageSearchPath = ResourcePatternResolver.CLASSPATH_ALL_URL_PREFIX +resolveBasePackage(basePackage) + '/' + this.resourcePattern;Resource[] resources = getResourcePatternResolver().getResources(packageSearchPath);boolean traceEnabled = logger.isTraceEnabled();boolean debugEnabled = logger.isDebugEnabled();for (Resource resource : resources) {if (traceEnabled) {logger.trace("Scanning " + resource);}if (resource.isReadable()) {try {MetadataReader metadataReader = getMetadataReaderFactory().getMetadataReader(resource);if (isCandidateComponent(metadataReader)) {ScannedGenericBeanDefinition sbd = new ScannedGenericBeanDefinition(metadataReader);sbd.setResource(resource);sbd.setSource(resource);if (isCandidateComponent(sbd)) {if (debugEnabled) {logger.debug("Identified candidate component class: " + resource);}candidates.add(sbd);}else {if (debugEnabled) {logger.debug("Ignored because not a concrete top-level class: " + resource);}}}else {if (traceEnabled) {logger.trace("Ignored because not matching any filter: " + resource);}}}catch (Throwable ex) {throw new BeanDefinitionStoreException("Failed to read candidate component class: " + resource, ex);}}else {if (traceEnabled) {logger.trace("Ignored because not readable: " + resource);}}}}catch (IOException ex) {throw new BeanDefinitionStoreException("I/O failure during classpath scanning", ex);}return candidates;}
相关文章:
TOPSIS算法及代码
TOPSIS的全称是“逼近于理想值的排序方法” 根据多项指标、对多个方案进行比较选择的分析方法,这种方法的中心思想在于首先确定各项指标的正理想值和负理想值,所谓正理想值是一设想的最好值(方案),它的的各个属性值都…
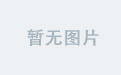
django框架的基础知识点《贰》
状态保持-----session作用:状态保持与cookie区别: cookie保存在浏览器中 session:保存在服务器中,即python代码运行的那台电脑 支持配置,可以指定保存的位置在django中保存方案: 关系型数据库 内存 关系型数…
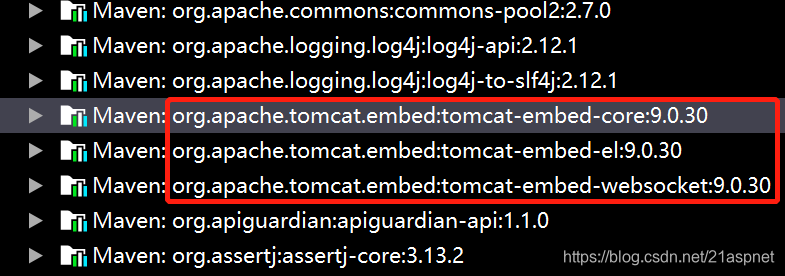
Springboot源码分析之内嵌tomcat源码分析
Springboot源码是内嵌tomcat的,这个和完整的tomcat还是不同。 内嵌tomcat的源码在tomcat-embed-core等3个jar包里 展开tomcat-embed-core的catalina目录 再对照下载的apache-tomcat-9.0.31源码 打开bin目录,看到很多库文件比如catalina.jar 再展开看看类…
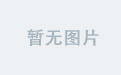
spring amqp rabbitmq fanout配置
基于spring amqp rabbitmq fanout配置如下: 发布端 <rabbit:connection-factory id"rabbitConnectionFactory" username"guest" password"guest" host"localhost" port"5672"/> <rabbit:template id&qu…
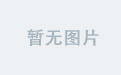
【MATLAB】数组运算
(这里这列举笔者不熟悉的,容易忘的数组运算) 1、数组的转置 >> a[1 2 3 4 5 6 7]a 1 2 3 4 5 6 7>> bab 1234567 2、对数组的赋值 >> a([1 4])[0 0]a 0 2 3 0 5 6 73、注…

RLCenter云平台配置中心
榕力RLCenter云平台配置中心以图形界面的方式实现对云桌面系统的统一管理,包括用户管理、服务器管理、虚拟机管理、策略管理。可配置U盘类设备的读写权限,避免企业敏感信息泄密。实行数据集中存储,支持用户数据进行备份和恢复。 (1)云桌面性能…

SSL/TLS原理详解
本文大部分整理自网络,相关文章请见文后参考。 关于证书授权中心CA以及数字证书等概念,请移步 OpenSSL 与 SSL 数字证书概念贴 ,如果你想快速自建CA然后签发数字证书,请移步 基于OpenSSL自建CA和颁发SSL证书 。 SSL/TLS作为一种互…
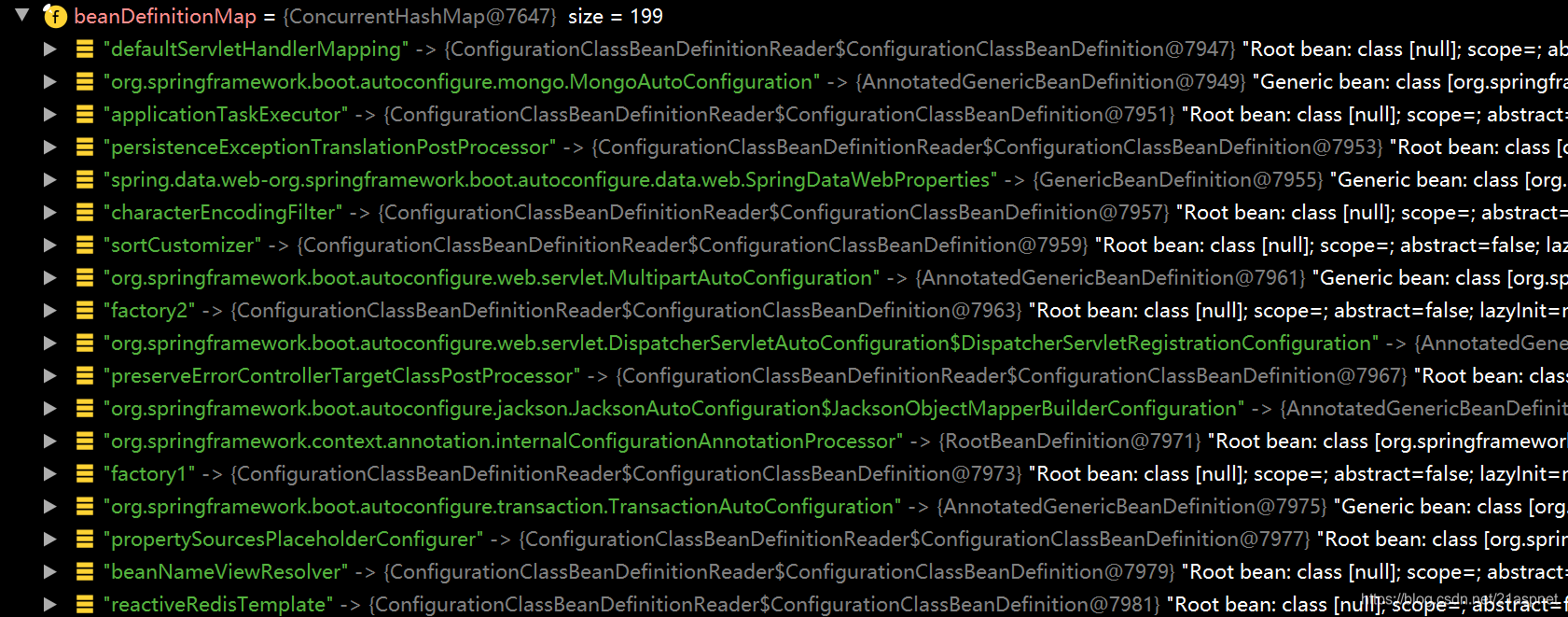
SpringBoot源码分析之@Scheduled
Springboot写上注解Scheduled就可以实现定时任务, 这里对其源码做一点分析 Service public class MyScheduled {Scheduled(cron"${time.cron}")void paoapaoScheduled() {System.out.println("Execute at " System.currentTimeMillis());} }…
【MATLAB】矩阵分析之向量和矩阵的范数运算
本片借鉴于 https://blog.csdn.net/u013534498/article/details/52674008 https://blog.csdn.net/left_la/article/details/9159949 向量范数当p1时,即为各个向量的元素绝对值之和 >> norm(x,1)ans 21>> xx 1 2 3 4 5 6>> no…
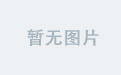
如何打一个FatJar(uber-jar)
如何打一个FatJar(uber-jar) FatJar也就叫做UberJar,是一种可执行的Jar包(Executable Jar)。FatJar和普通的jar不同在于它包含了依赖的jar包。 1. maven-jar-plugin 例子 <build><finalName>demo</finalName><plugins&g…
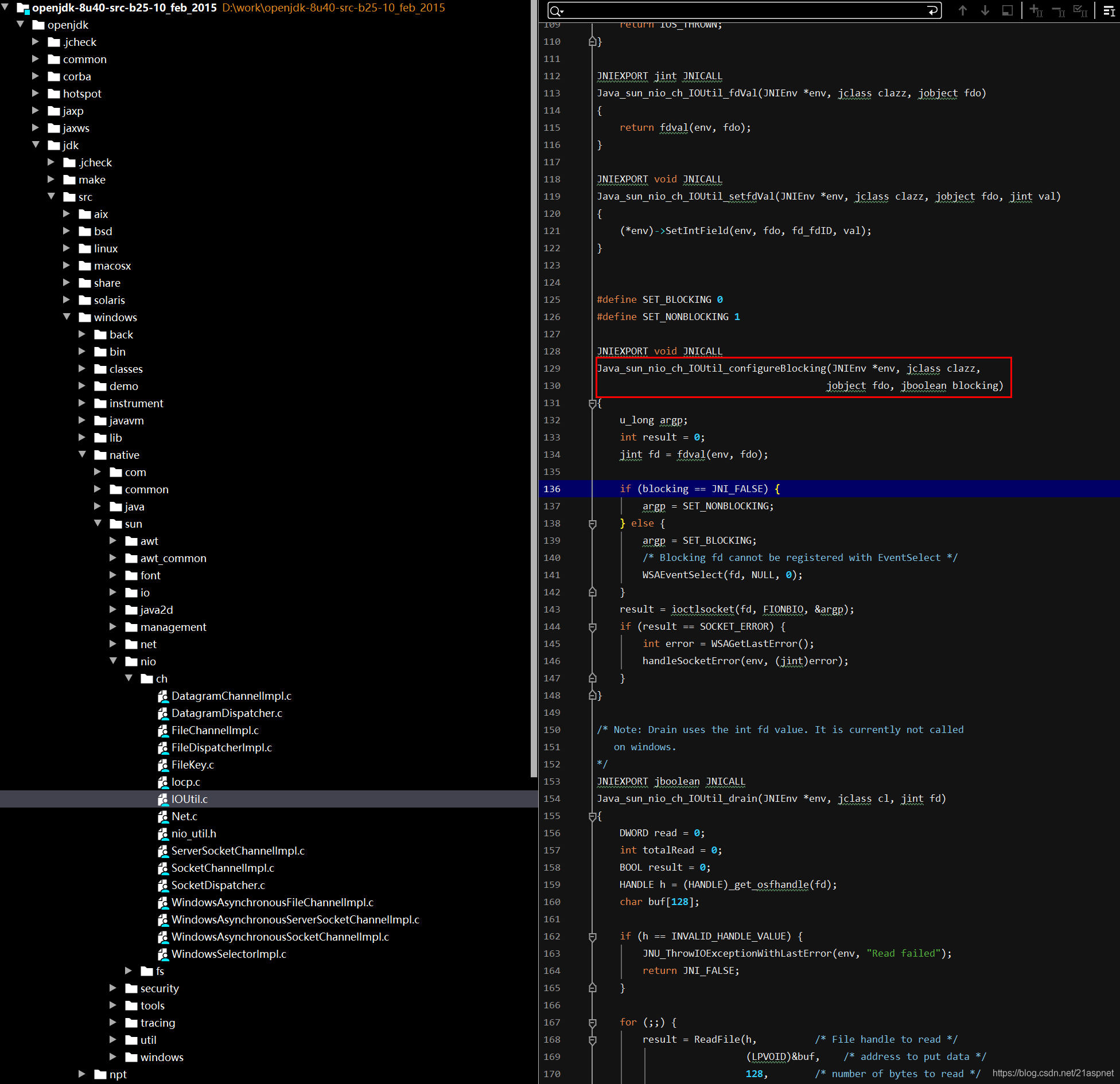
JDK源码分析 NIO实现
总列表:http://hg.openjdk.java.net/ 小版本:http://hg.openjdk.java.net/jdk8u jdk:http://hg.openjdk.java.net/jdk8u/jdk8u60/file/d8f4022fe0cd hotspot:http://hg.openjdk.java.net/jdk8u/jdk8u60/hotspot/file/37240c1019fd 调用本地native方法…
Linux进程ID号--Linux进程的管理与调度(三)
进程ID概述 进程ID类型 要想了解内核如何来组织和管理进程ID,先要知道进程ID的类型: 内核中进程ID的类型用pid_type来描述,它被定义在include/linux/pid.h中 enum pid_type {PIDTYPE_PID,PIDTYPE_PGID,PIDTYPE_SID,PIDTYPE_MAX };12345671234567PID 内核…
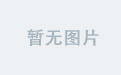
【MATLAB】矩阵运算之矩阵分解
矩阵分解:把一个矩阵分解成为矩阵连乘的形式。矩阵的分解函数cholCholesky分解cholinc稀疏矩阵的不完全Cholesky分解lu矩阵LU分解luinc稀疏矩阵的不完全LU分解qr正交三角分解svd奇异值分解gsvd一般奇异值分解schur舒尔分解 在MATLAB中线性方程组的求解主要基于四种基…
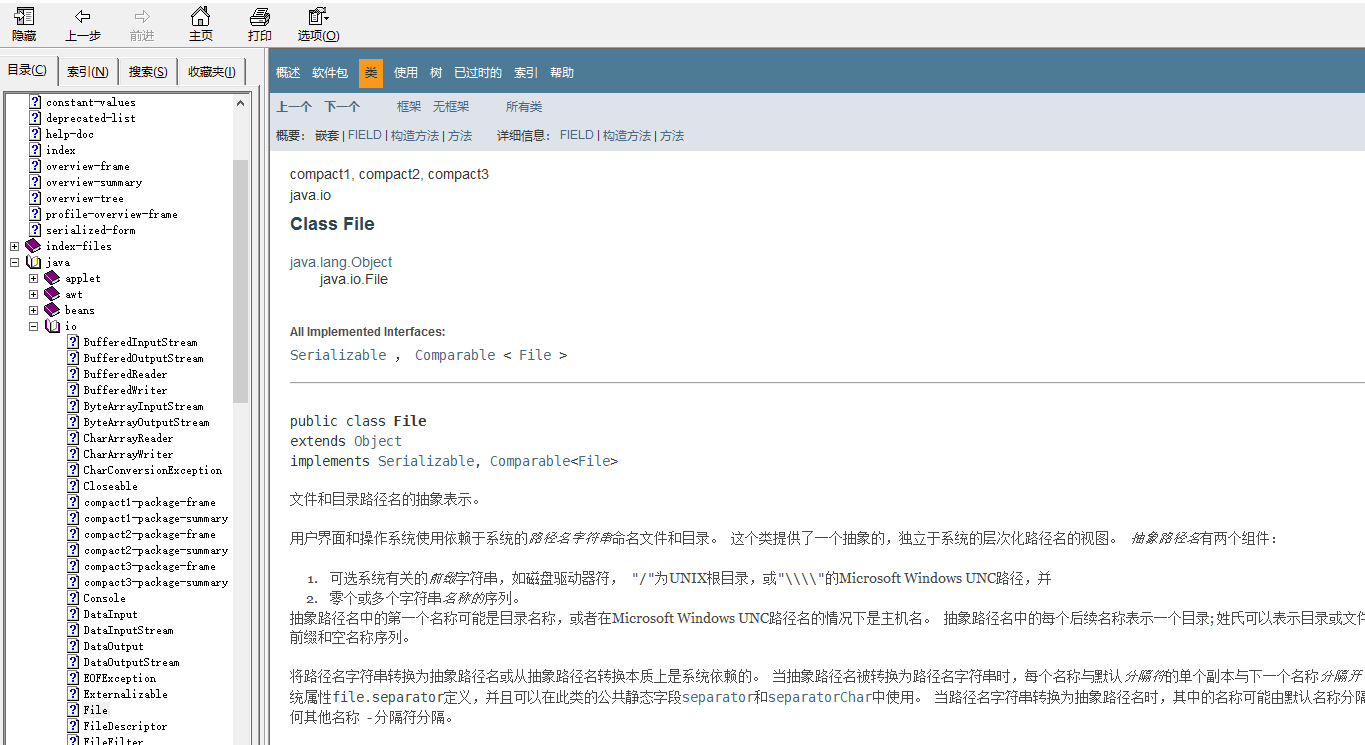
Java入门—输入输出流
File类的使用 文件是:文件可认为是相关记录或放在一起的数据的集合。 Java中,使用java.io.File类对文件进行操作 public class FileDemo {public static void main(String[] args) {String path "E:\\pdd";File f new File(path);//判断是文…
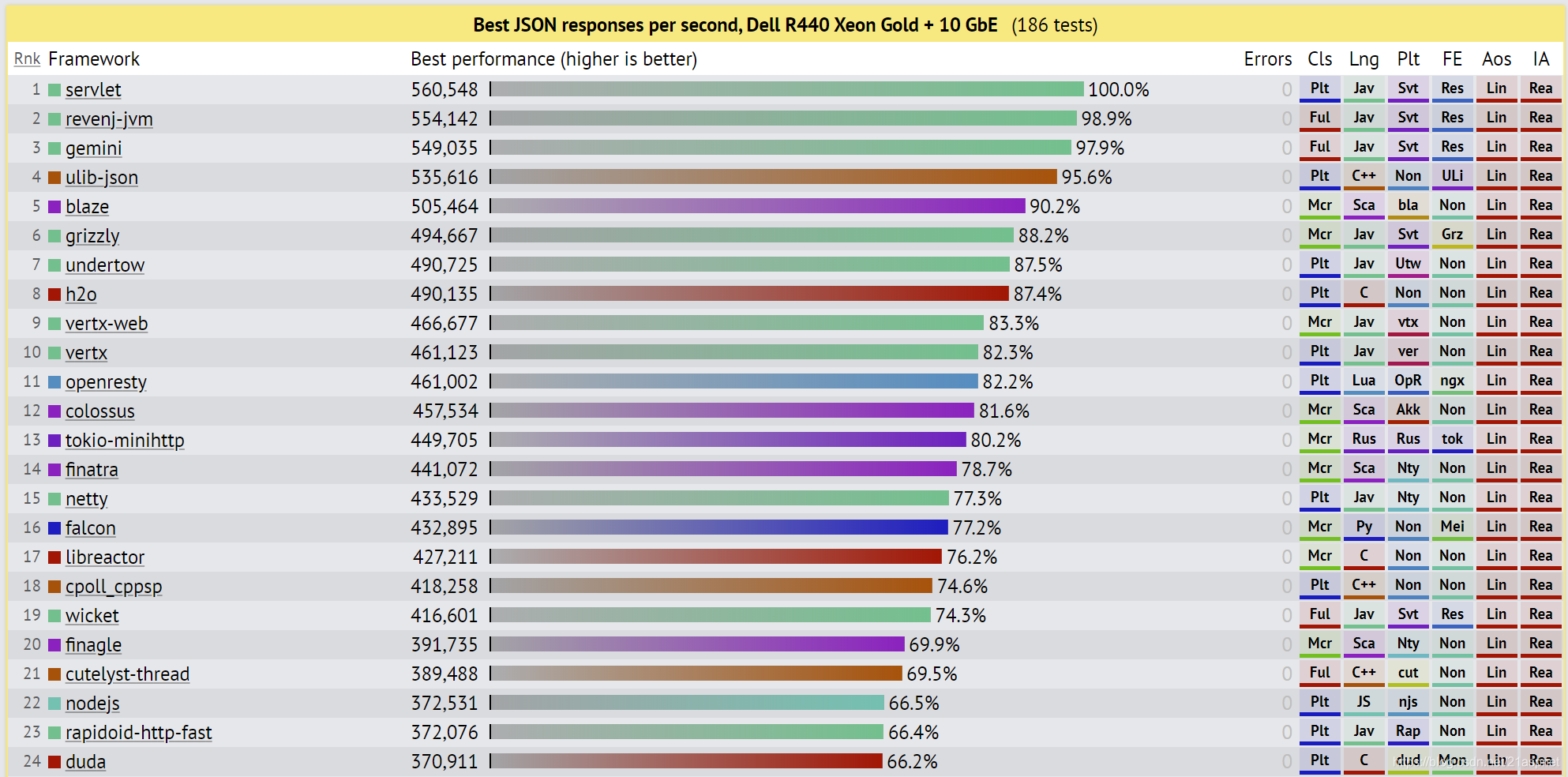
Web框架基准测试
Web Framework Benchmarks 这是许多执行基本任务(例如JSON序列化,数据库访问和服务器端模板组成)的Web应用程序框架的性能比较。每个框架都在实际的生产配置中运行。结果在云实例和物理硬件上捕获。测试实现主要是由社区贡献的,所…
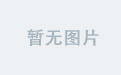
vsftpd用户配置 No.2
在配置ftp虚拟用户的过程中,还有一种配置方式。yum -y install 安装vsftpdcp /etc/vsftpd/vsftpd.conf /etc/vsftpd/vsftpd.conf.bak编辑vsftpd.conf开启下列选项:anonymous_enableNOlocal_enableYESwrite_enableYESlocal_umask022anon_mkdir_write_enab…
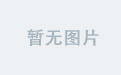
【MATLAB】稀疏矩阵(含有大量0元素的矩阵)
1、稀疏矩阵的储存方式 对于稀疏矩阵,MATLAB仅储存矩阵所有非零元素的值及其位置(行号和列号)。 2、稀疏矩阵的生成 1)利用sparse函数从满矩阵转换得到稀疏矩阵函数名称表示意义sparse(A)由非零元素和下标建立稀疏矩阵A。如果A已是…
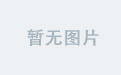
httpTomcat
Tomcat是web应用服务器的一种 转载于:https://juejin.im/post/5beaf7e451882517165d91d1

memcached(二)事件模型源码分析
在memcachedd中,作者为了专注于缓存的设计,使用了libevent来开发事件模型。memcachedd的时间模型同nginx的类似,拥有一个主进行(master)以及多个工作者线程(woker)。 流程图 在memcached中&…
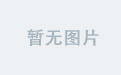
【MATLAB】MATLAB的控制流
1、if-else-end if expressioncommands1 elseif expression2commands2 ... else commandsn end 2、switch-case switch valuecase1 test1%如果value等于test1,执行command1,并结束此结构command1case2 test2command2...case3 testkcommandk otherw…
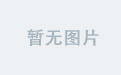
Linux查看本机端口
查看指定的端口 # lsof -i:port 查看所有端口 # netstat -aptn 安装telnet #yum install -y telnet.x86_64 #telnet ip 端口
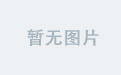
Node.js安装
通过nvm安装 下载nvm并执行wget -qO- https://raw.github.com/creationix/nvm/v0.33.11/install.sh | sh将命令输出到终端命令中~/.bashrcexport NVM_DIR"$HOME/.nvm"更新文件source .bashrc通过nvm安装node.jsnvm install 10.13安装的版本是10.13的版本 通过命令查看…
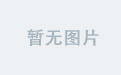
mongodb常用语句以及SpringBoot中使用mongodb
普通查询 某个字段匹配数组内的元素数量的,假如region只有一个元素的 db.getCollection(map).find({region:{$size:1}}) 假如region只有0个元素的 db.getCollection(map).find({region:{$size:0}}) db.getCollection(map).find({region:{$size:1}}).count() db.get…
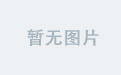
2002高教社杯---A车灯线光源的优化设计
A题 车灯线光源的优化设计 安装在汽车头部的车灯的形状为一旋转抛物面,车灯的对称轴水平地指向正前方, 其开口半径36毫米,深度21.6毫米。经过车灯的焦点,在与对称轴相垂直的水平方向,对称地放置一定长度的均匀分布的线光源。要求…
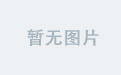
从Date类型转为中文字符串
//主方法public static String DateToCh(Date date) {Calendar cal Calendar.getInstance();cal.setTime(date);int year cal.get(Calendar.YEAR);int month cal.get(Calendar.MONTH) 1;int day cal.get(Calendar.DAY_OF_MONTH);return getYear(year) getTenString(month…
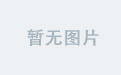
第十四课 如何在DAPP应用实现自带钱包转账功能?
1,为什么DAPP生态需要自带钱包功能? 区块链是一个伟大的发明,它改变了生产关系。很多生态,有了区块链技术,可以由全公司员工的"全员合伙人"变成了全平台的”全体合伙人”了,是真正的共享经济模式…
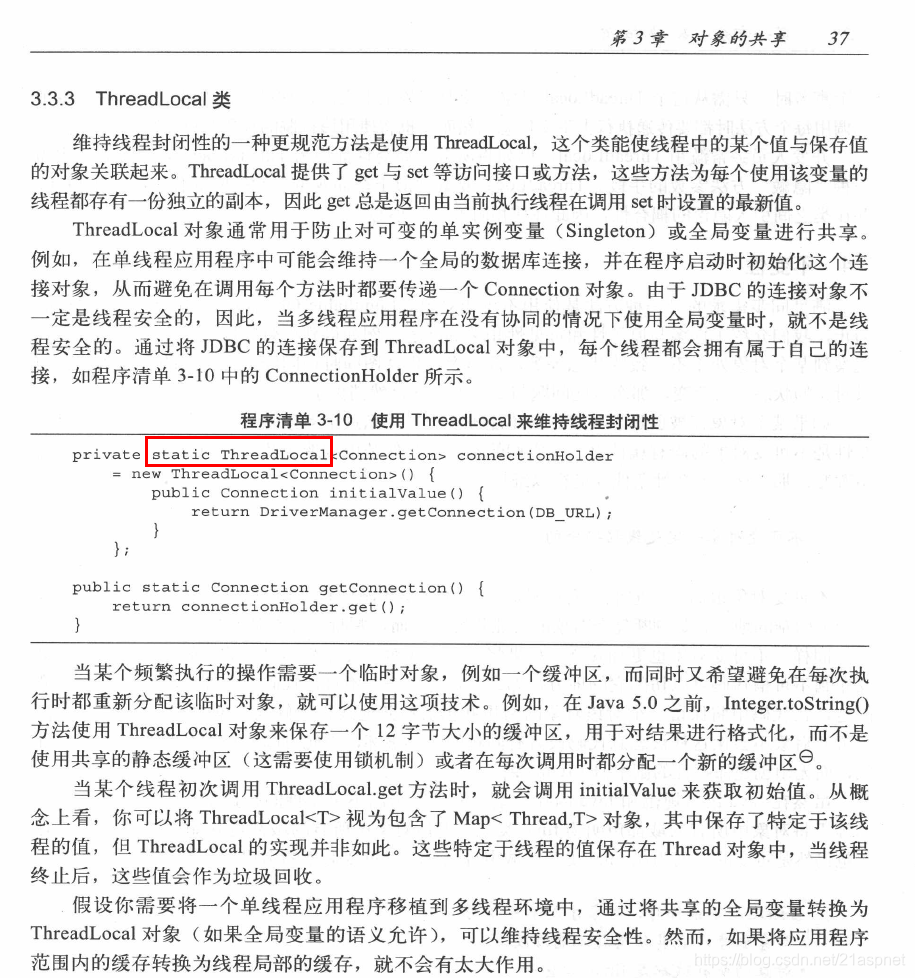
为什么jdk源码推荐ThreadLocal使用static
ThreadLocal是线程私有变量,本身是解决多线程环境线程安全,可以说单线程实际上没必要使用。 既然多线程环境本身不使用static,那么又怎么会线程不安全。所以这个问题本身并不是问题,只是有人没有理解ThreadLocal的真正使用场景&a…

C与C++之间相互调用
1、导出C函数以用于C或C的项目 如果使用C语言编写的DLL,希望从中导出函数给C或C的模块访问,则应使用 __cplusplus 预处理器宏确定正在编译的语言。如果是从C语言模块使用,则用C链接声明这些函数。如果使用此技术并为DLL提供头文件,…
【MATLAB】三维图形的绘制mesh
步骤如下: (1)确定自变量x和y的取值范围和取值间隔 x x1 :dx :x2 , y y1 : dy : y2 (2)构成xoy平面上的自变量采样“格点”矩阵 ①利用格点矩阵的原理生成矩阵。 xx1:dx:x2; yy1:dy:y2; Xones(size(y))*x; Yy*o…
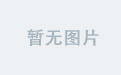
ORA-01919: role 'PLUSTRACE' does not exist
环境:Oracle 10g,11g.现象:在一次迁移测试中,发现有这样的角色赋权会报错不存在: SYSorcl> grant PLUSTRACE to jingyu; grant PLUSTRACE to jingyu* ERROR at line 1: ORA-01919: role PLUSTRACE does not exist 查询发现这个…