【C++】C++11 STL算法(四):二分查找法(Binary search operations)、合并操作
目录
- 一、lower_bound
- 1、原型:
- 2、说明:
- 3、官方demo
- 二、upper_bound
- 1、原型:
- 2、说明:
- 3、官方demo
- 三、binary_search
- 1、原型:
- 2、说明:
- 3、官方demo
- 四、equal_range
- 1、原型:
- 2、说明:
- 3、官方demo
- 五、merge
- 1、原型:
- 2、说明:
- 3、官方demo
- 六、inplace_merge
- 1、原型:
- 2、说明:
- 2、官方demo
头文件:#include <algorithm>
一、lower_bound
1、原型:
template< class ForwardIt, class T >
ForwardIt lower_bound( ForwardIt first, ForwardIt last, const T& value );template< class ForwardIt, class T, class Compare >
ForwardIt lower_bound( ForwardIt first, ForwardIt last, const T& value, Compare comp );
2、说明:
返回不小于给定值的第一个元素的位置(迭代器)。
3、官方demo
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>template<class ForwardIt, class T, class Compare=std::less<>>
ForwardIt binary_find(ForwardIt first, ForwardIt last, const T& value, Compare comp={})
{// Note: BOTH type T and the type after ForwardIt is dereferenced must be implicitly convertible to BOTH Type1 and Type2, used in Compare. // This is stricter than lower_bound requirement (see above)first = std::lower_bound(first, last, value, comp);return first != last && !comp(value, *first) ? first : last;
}int main()
{std::vector<int> data = { 1, 1, 2, 3, 3, 3, 3, 4, 4, 4, 5, 5, 6 };auto lower = std::lower_bound(data.begin(), data.end(), 4);auto upper = std::upper_bound(data.begin(), data.end(), 4);std::copy(lower, upper, std::ostream_iterator<int>(std::cout, " "));std::cout << '\n';// :经典的二分查找,只有在存在值时才返回值data = { 1, 2, 4, 6, 9, 10 }; auto it = binary_find(data.cbegin(), data.cend(), 4); //使用 '5' 将返回 end()if(it != data.cend())std::cout << *it << " found at index "<< std::distance(data.cbegin(), it);return 0;
}
Output:
4 4 4
4 found at index 2
二、upper_bound
1、原型:
template< class ForwardIt, class T >
ForwardIt upper_bound( ForwardIt first, ForwardIt last, const T& value );template< class ForwardIt, class T, class Compare >
ForwardIt upper_bound( ForwardIt first, ForwardIt last, const T& value, Compare comp );
2、说明:
返回大于给定值的第一个元素的位置(迭代器),如果没有返回last
3、官方demo
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>int main()
{std::vector<int> data = { 1, 1, 2, 3, 3, 3, 3, 4, 4, 4, 5, 5, 6 };auto lower = std::lower_bound(data.begin(), data.end(), 4);auto upper = std::upper_bound(data.begin(), data.end(), 4);std::copy(lower, upper, std::ostream_iterator<int>(std::cout, " "));
}
Output:
4 4 4
三、binary_search
1、原型:
template< class ForwardIt, class T >
bool binary_search( ForwardIt first, ForwardIt last, const T& value );template< class ForwardIt, class T, class Compare >
bool binary_search( ForwardIt first, ForwardIt last, const T& value, Compare comp );
2、说明:
确定某个元素是否存于[first, last)范围中。
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>int main()
{std::vector<int> haystack {1, 3, 4, 5, 9};std::vector<int> needles {1, 2, 3};for (auto needle : needles) {std::cout << "Searching for " << needle << '\n';if (std::binary_search(haystack.begin(), haystack.end(), needle)) {std::cout << "Found " << needle << '\n';} else {std::cout << "no dice!\n";}}
}
Output:
Searching for 1
Found 1
Searching for 2
no dice!
Searching for 3
Found 3
四、equal_range
1、原型:
template< class ForwardIt, class T >
std::pair<ForwardIt,ForwardIt>equal_range( ForwardIt first, ForwardIt last, const T& value );template< class ForwardIt, class T, class Compare >
std::pair<ForwardIt,ForwardIt>equal_range( ForwardIt first, ForwardIt last, const T& value, Compare comp );
2、说明:
返回[first, last)范围内等于value的子范围
3、官方demo
#include <algorithm>
#include <vector>
#include <iostream>struct S
{int number;char name;// note: name is ignored by this comparison operatorbool operator< ( const S& s ) const { return number < s.number; }
};int main()
{// note: not ordered, only partitioned w.r.t. S defined belowstd::vector<S> vec = { {1,'A'}, {2,'B'}, {2,'C'}, {2,'D'}, {4,'G'}, {3,'F'} };S value = {2, '?'};auto p = std::equal_range(vec.begin(), vec.end(), value);for ( auto i = p.first; i != p.second; ++i )std::cout << i->name << ' ';// heterogeneous comparison:struct Comp {bool operator() ( const S& s, int i ) const { return s.number < i; }bool operator() ( int i, const S& s ) const { return i < s.number; }};auto p2 = std::equal_range(vec.begin(),vec.end(), 2, Comp{});for ( auto i = p2.first; i != p2.second; ++i )std::cout << i->name << ' ';
}
Output:
B C D B C D
五、merge
1、原型:
template< class InputIt1, class InputIt2, class OutputIt >
OutputIt merge( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first );template< class InputIt1, class InputIt2, class OutputIt, class Compare>
OutputIt merge( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first, Compare comp );
2、说明:
合并两个已经排序好的序列
3、官方demo
#include <iostream>
#include <iterator>
#include <algorithm>
#include <vector>
#include <random>
#include <functional>int main()
{// 用随机数填充向量std::random_device rd;std::mt19937 mt(rd());std::uniform_int_distribution<> dis(0, 9);std::vector<int> v1(10), v2(10);std::generate(v1.begin(), v1.end(), std::bind(dis, std::ref(mt)));std::generate(v2.begin(), v2.end(), std::bind(dis, std::ref(mt)));// 排序std::sort(v1.begin(), v1.end());std::sort(v2.begin(), v2.end());// output v1std::cout << "v1 : ";std::copy(v1.begin(), v1.end(), std::ostream_iterator<int>(std::cout, " "));std::cout << '\n';// output v2std::cout << "v2 : ";std::copy(v2.begin(), v2.end(), std::ostream_iterator<int>(std::cout, " "));std::cout << '\n';// 合并std::vector<int> dst;std::merge(v1.begin(), v1.end(), v2.begin(), v2.end(), std::back_inserter(dst));// outputstd::cout << "dst: ";std::copy(dst.begin(), dst.end(), std::ostream_iterator<int>(std::cout, " "));std::cout << '\n';
}
Possible output:
v1 : 0 1 3 4 4 5 5 8 8 9
v2 : 0 2 2 3 6 6 8 8 8 9
dst: 0 0 1 2 2 3 3 4 4 5 5 6 6 8 8 8 8 8 9 9
六、inplace_merge
1、原型:
template< class BidirIt >
void inplace_merge( BidirIt first, BidirIt middle, BidirIt last );
2、说明:
将已经排序好的两段序列[first, middle)、[middle,last)合并并且配序成[first, last)
2、官方demo
#include <vector>
#include <iostream>
#include <algorithm>template<class Iter>
void merge_sort(Iter first, Iter last) //归并排序,参见https://www.runoob.com/w3cnote/merge-sort.html,有动图演示
{if (last - first > 1) {Iter middle = first + (last - first) / 2;merge_sort(first, middle);merge_sort(middle, last);std::inplace_merge(first, middle, last);}
}int main()
{std::vector<int> v{8, 2, -2, 0, 11, 11, 1, 7, 3};merge_sort(v.begin(), v.end());for(auto n : v) {std::cout << n << ' ';}std::cout << '\n';
}
Output:
-2 0 1 2 3 7 8 11 11
相关文章:
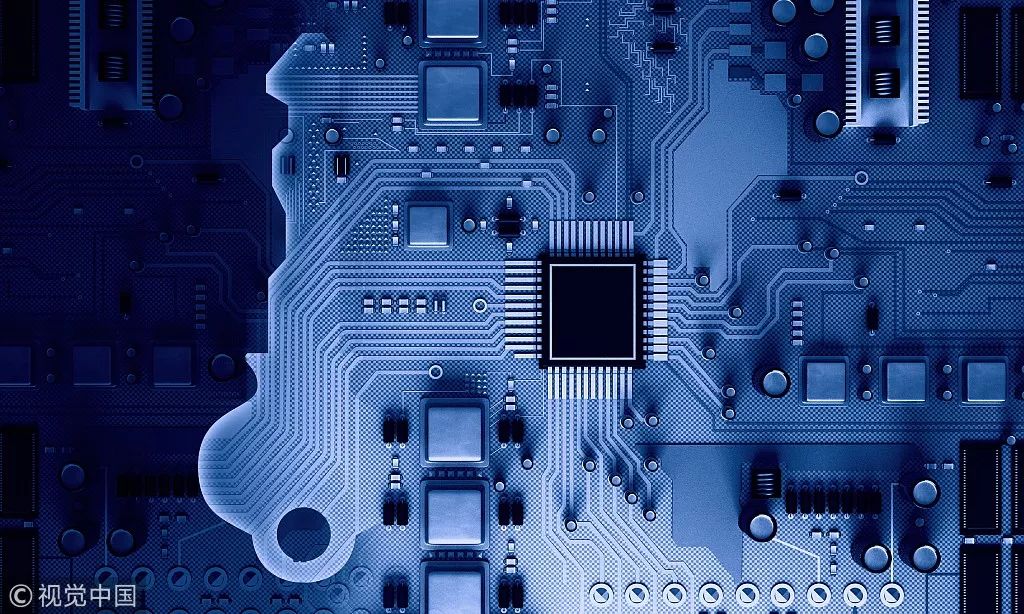
腾讯开源分布式NoSQL存储系统DCache | 技术头条
作者 | 山宝银,腾讯后台高级工程师,专注于分布式 NoSQL 存储领域的技术研发工作,参与腾讯多个自研存储系统的开发,在分布式系统、高可用与高性能服务等领域有较丰富的经验。来源 | 腾讯技术博客当你在电商平台秒杀商品或者在社交网…
老司机带你学爬虫——Python爬虫技术分享
什么是“爬虫”? 简单来说,写一个从web上获取需要数据并按规定格式存储的程序就叫爬虫; 爬虫理论上步骤很简单,第一步获取html源码,第二步分析html并拿到数据。但实际操作,老麻烦了~ 用Python写“爬虫”有哪…
[转载]分享WCF聊天程序--WCFChat
http://www.cnblogs.com/gaoweipeng/archive/2009/09/04/1560260.html 无意中在一个国外的站点下到了一个利用WCF实现聊天的程序,作者是:Nikola Paljetak。研究了一下,自己做了测试和部分修改,感觉还不错,分享给大家。…
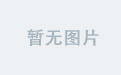
【C++】C++11 STL算法(五):设置操作(Set operations)、堆操作(Heap operations)
目录设置操作(Set operations)一、includes1、原型:2、说明:3、官方demo二、set_difference1、原型:2、说明:3、官方demo三、set_intersection1、原型:2、说明:3、官方demo四、set_symmetric_difference1、…
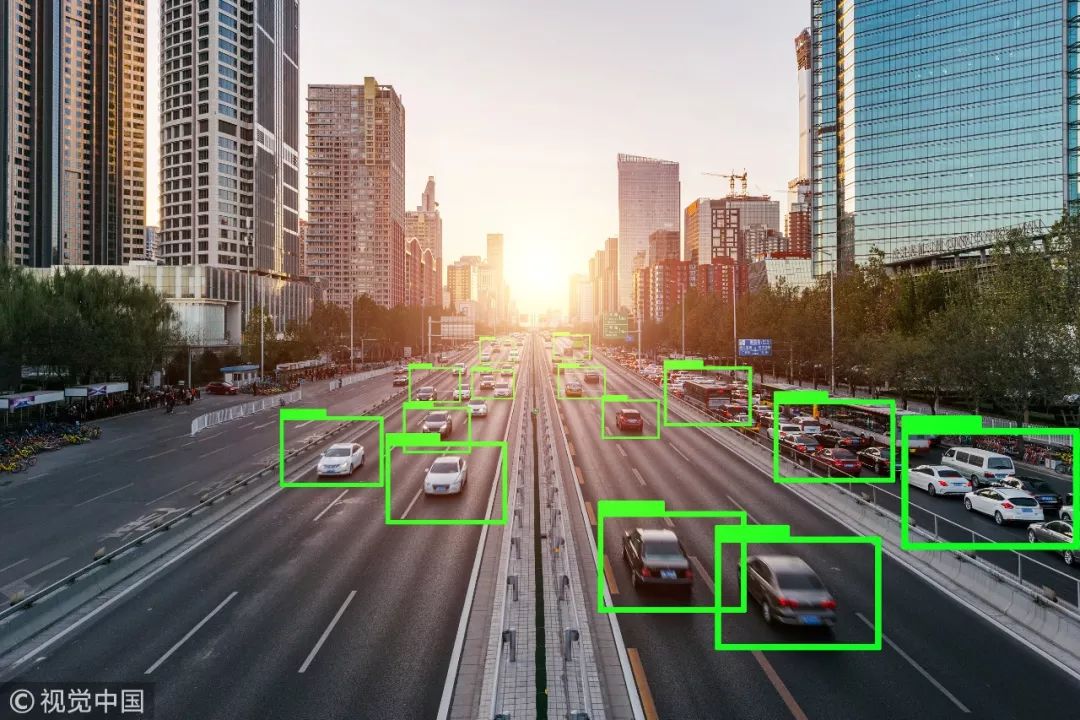
63万张!旷视发布最大物体检测数据集Objects365 | 技术头条
编辑 | 琥珀来源 | AI科技大本营(id:rgznai100)昨日,在旷视科技联合北京智源人工智能研究院举办的发布会上,旷视研究院发布了物体检测数据集 Objects365,包含 63 万张图像数量,365 个类别数量&a…
(一)Android Studio 安装部署 华丽躲坑
叨叨两句先 小宇之前一直做前后端开发,只是略懂JS,未接触过Java和Android 近期工作任务也是兴趣使然,开始琢磨DJI二次开发 DJI是我最服气的无人机厂商,无人机稳定性极强,性价比狂高,还给了极度丰富的二次开…
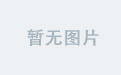
linux 环境配置 安装jdk
一. 下载jdk5.0 for linux 到sun的主页 http://java.sun.com/j2se/1.5.0/download.jsp 下载jdk安装文件jdk-1_5_0_05-linux-i586.bin 二. 解压安装jdk 在shell终端下进入jdk-1_5_0_05-linux-i586.bin文件所在目录,执行命令 ./jdk-1_5_0_05-linux-i586.bin 这时会出现…
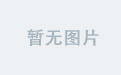
【C++】C++11 STL算法(六):最小/最大操作(Minimum/maximum operations)、比较运算(Comparison operations)
目录最小/最大操作(Minimum/maximum operations)一、max1、原型:2、说明:3、官方demo二、max_element1、原型:2、说明:3、官方demo三、min1、原型:2、说明:3、官方demo四、min_element1、原型:2…
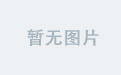
springboot之定时任务
定时线程 说到定时任务,通常会想到JDK自带的定时线程来执行,定时任务。 回顾一下定时线程池。 public static ScheduledExecutorService newScheduledThreadPool(int var0) {return new ScheduledThreadPoolExecutor(var0);}public static ScheduledExec…
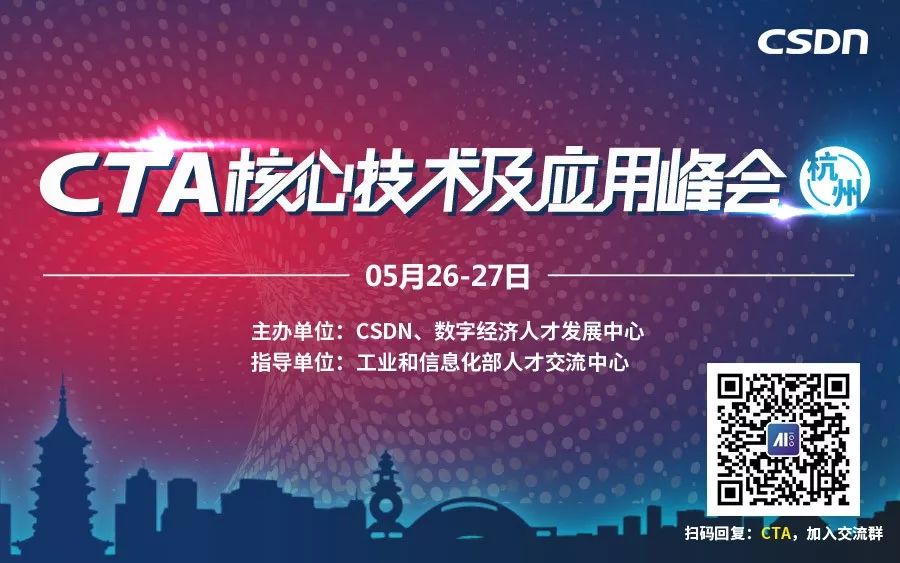
10只机器狗拉卡车!井然有序,毫不费力 | 极客头条
整理 | 琥珀出品 | AI科技大本营(ID:rgznai100)看来,这家娱乐网友多年的机器人公司终于要开始实现商用化了!最先备受期待的是它的网红机器狗 SpotMini。今日凌晨,据多家外媒报道,波士顿动力 (Boston Dynami…
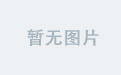
linux下查看nginx,apache,mysql,php的编译参数
有时候nginx,apache,mysql,php编译完了想看看编译参数可以用以下方法 nginx编译参数: #/usr/local/nginx/sbin/nginx -V nginx version: nginx/0.6.32 built by gcc 4.1.2 20071124 (Red Hat 4.1.2-42) configure arguments: --us…
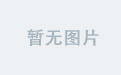
【C++】C++11 STL算法(七):排列操作(Permutation operations)、数值操作(Numeric operations)
排列操作(Permutation operations) 一、is_permutation 1、原型: template< class ForwardIt1, class ForwardIt2 > bool is_permutation( ForwardIt1 first1, ForwardIt1 last1, ForwardIt2 first2 );template< class ForwardIt…
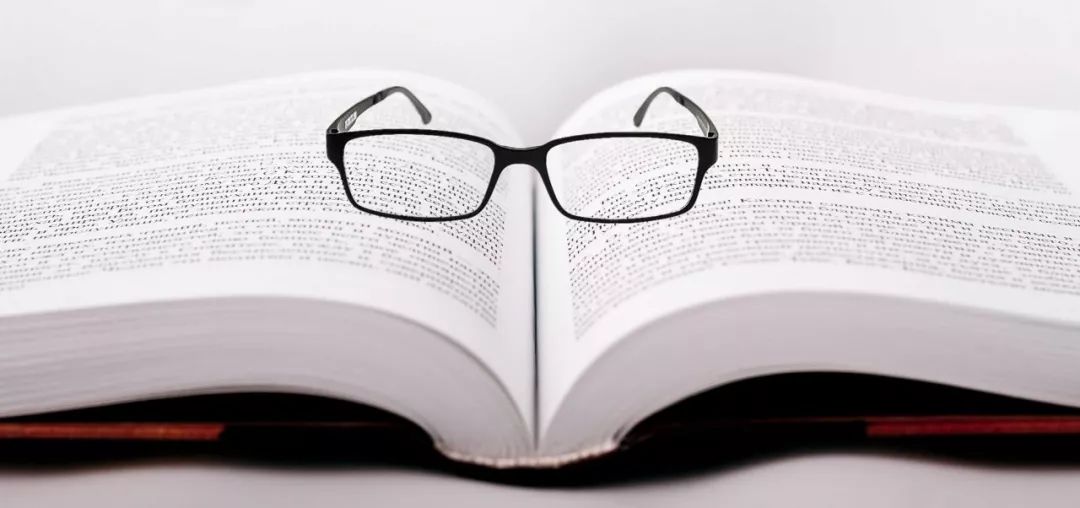
码书:入门中文NLP必备干货:5分钟看懂“结巴”分词(Jieba)
导读:近年来,随着NLP技术的日益成熟,开源实现的分词工具越来越多,如Ansj、盘古分词等。在本文中,我们选取了Jieba进行介绍和案例展示,主要基于以下考虑:社区活跃。截止本文发布前,Ji…
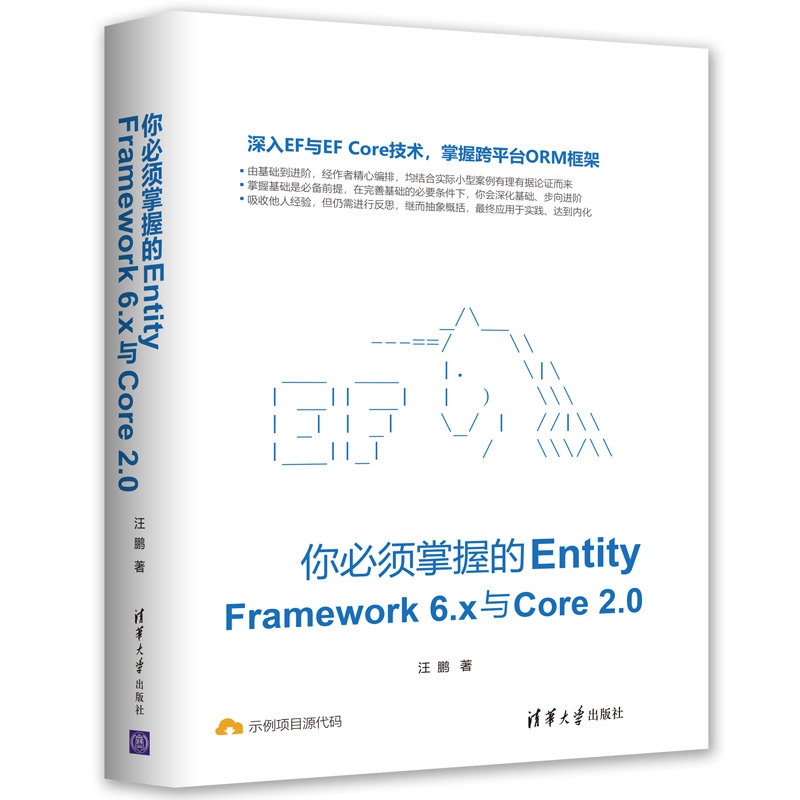
《你必须掌握的Entity Framework 6.x与Core 2.0》正式出版感想
前言 借书正式出版之际,完整回顾下从写博客到写书整个历程,也算是对自己近三年在技术上的一个总结,整个历程可通过三个万万没想到来概括,请耐心阅读。 写博、写书完整历程回顾 从2013年12月注册博客园账号,注册博客园账…
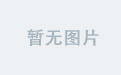
JSF实现“Hello World!”
我们编写一个在页面上显示是“Hello World! ”,我们至少需要编写一个Page对象和一个对应模板文件(tml)。 第一步,Page对象编写 在Tapestry5中Page是与一个页面对应的POJO对象,它不需要继承Tapestry框架的任何基类或实现…
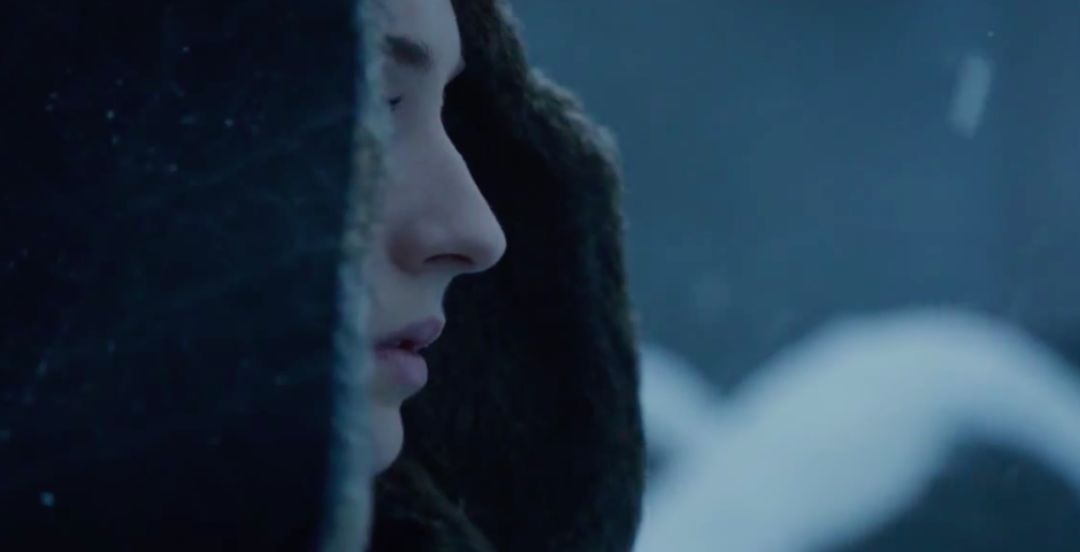
《权力的游戏》最终季上线!谁是你最喜爱的演员?这里有一份Python教程 | 附源码...
译者 | 刘畅编辑 | 琥珀出品 | AI科技大本营(id:rgznai100)《权力的游戏》最终季已于近日开播,对于全世界翘首以待的粉丝们来说,其最大的魅力就在于“无法预知的人物命运”。那些在魔幻时代的洪流中不断沉浮的人们&…
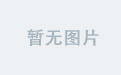
【C++】C++11 STL算法(八):对未初始化内存的操作(Operations on uninitialized memory)、C库(C library)
对未初始化内存的操作(Operations on uninitialized memory) 一、uninitialized_copy 1、原型: template< class InputIt, class ForwardIt > ForwardIt uninitialized_copy( InputIt first, InputIt last, ForwardIt d_first );2、…

OSPF高级设置实现全网互通
OSPF(开放式最短路径优先)是对链路状态路由协议的一种实现,隶属内部网关协议(IGP),故运作于自治系统内部(AS)。采用戴克斯特拉算法(Dijkstras algorithm)被用来计算最短路径树。“Cost”作为路由度量值。链…

学习PHP ?
学PHP的决定真的是好的吗? 不怕又再错一次了吗? 已经是最后的一年半上学时间了.... 真的不愿再走之前那条失败的路,不愿,真的不愿; 这年半无论如何都要把一样技术搞精了 一年半的时间,对我来讲够了....只看…
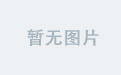
【数据库】sqlite中的限制:数据库大小、表数、列数、行数、参数个数、连接数等
目录一、参考网址二、详解1、查看、设置sqlite限制命令.limit2、SQLite中的限制汇总1)字符串或BLOB的最大长度2)最大列数3)SQL语句的最大长度4)联接中的最大表数5)表达式树的最大深度6)函数的最大参数个数7…
flutter中的生命周期
前言 和其他的视图框架比如android的Activity一样,flutter中的视图Widget也存在生命周期,生命周期的回调函数提现在了State上面。理解flutter的生命周期,对我们写出一个合理的控件至关重要。组件State的生命周期整理如下图所示: 大…
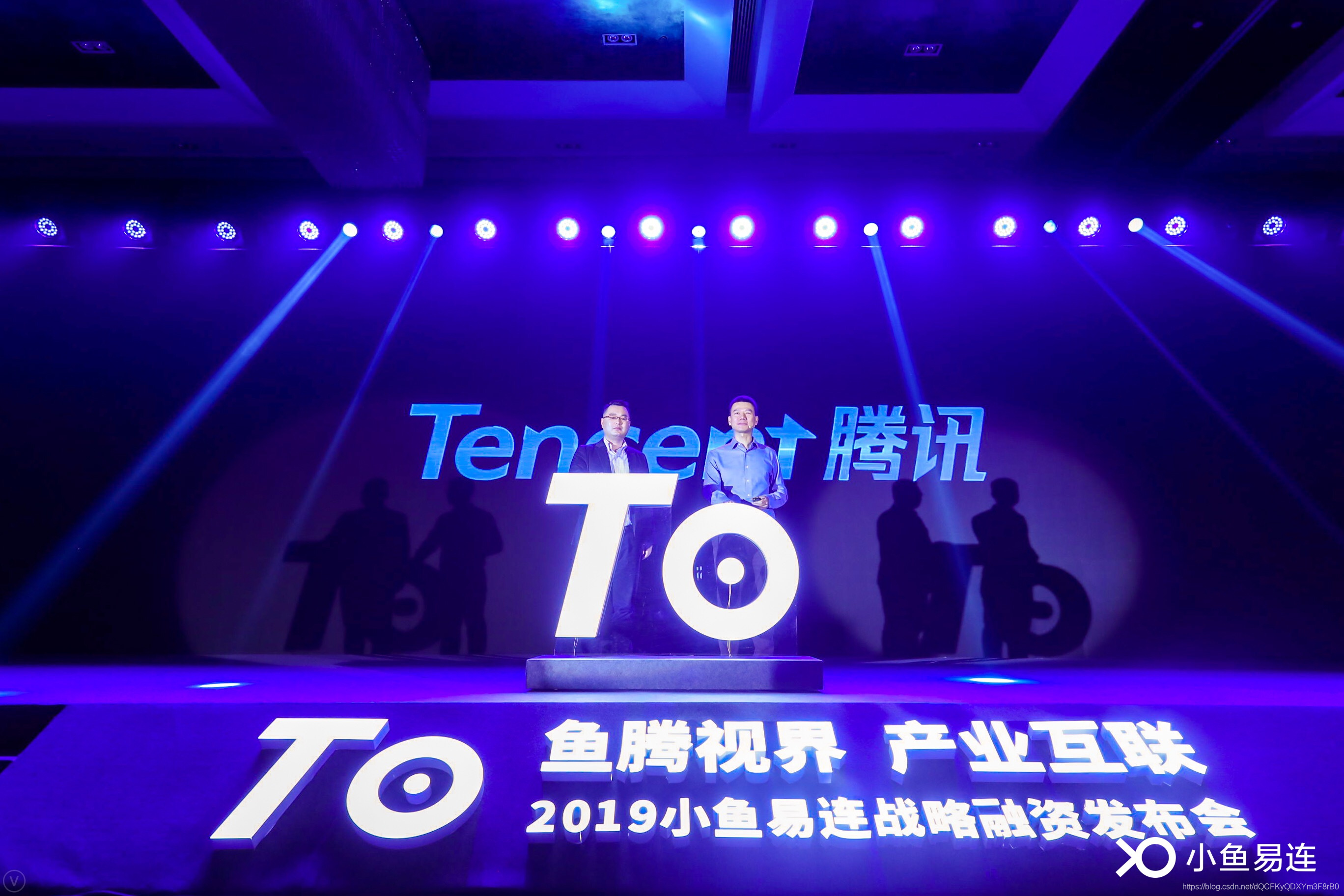
小鱼易连获腾讯数亿C轮投资,云视频布局产业互联网
4 月 18 日,小鱼易连在北京举行 “鱼腾视界 产业互联” 战略合作暨融资发布会上,正式宣布获得 C 轮融资,由腾讯领投。融得的资金将全面用于小鱼易连云视频系统在产业互联网领域的落地,打通企业、政府、个人三者之间的柔性生态全产…
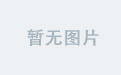
异步IO一定更好吗?
http://cnodejs.org/blog/?p1015续:异步IO一定更好吗?我之前的一篇文章《异步IO一定更好吗?》中举了一个很变态的例子,用以说明在单碟机械式硬盘上异步IO反而可能降低性能的问题,大家的讨论很热烈。前天的NodeParty杭…
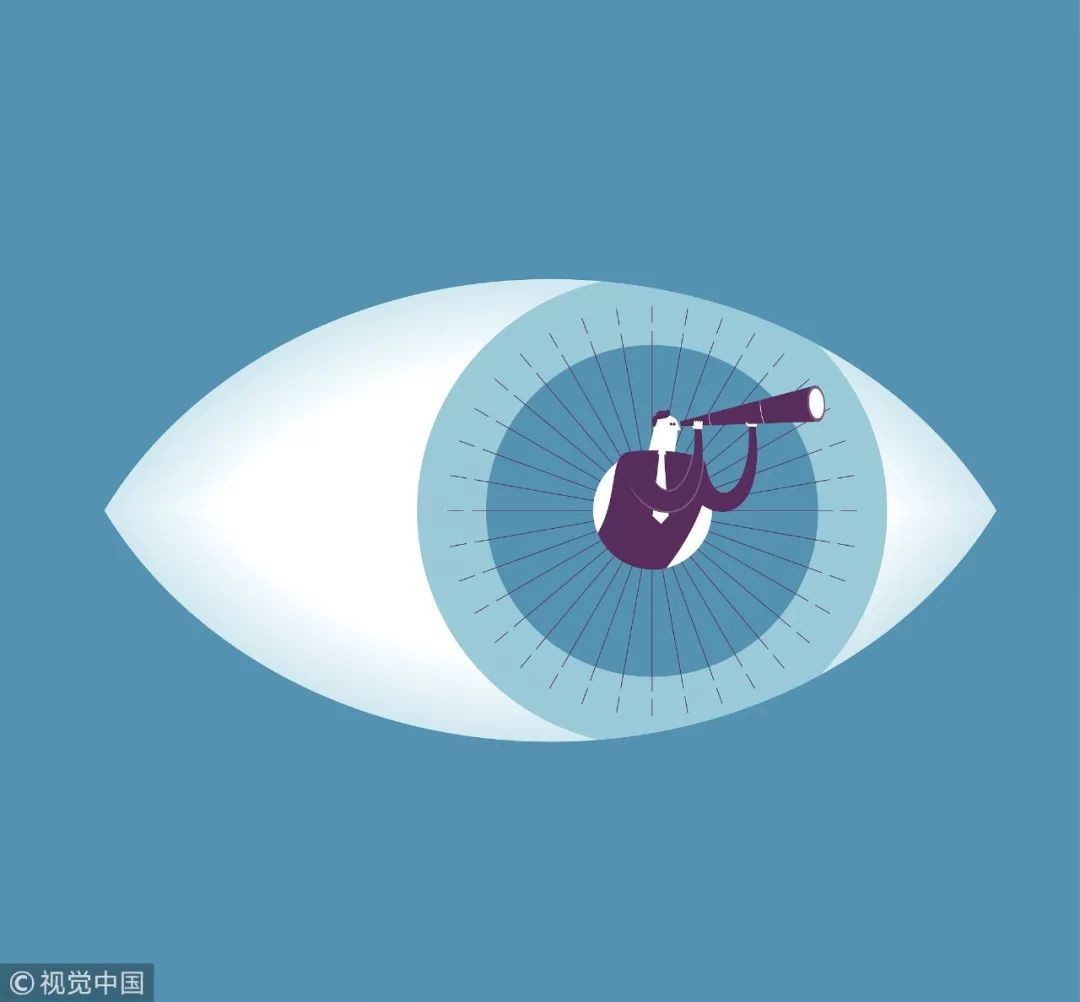
谈谈Python那些不为人知的冷知识(二)
本文转载自Python的编程时光(ID:Python-Time)小明在日常Code中遇到一些好玩,冷门的事情,通常都会记录下来。从上一篇的分享来看,仍然有不少 Pythoner 对这些冷知识存在盲区,所以今天迎来第二篇。如果上篇你…
前端每日实战:45# 视频演示如何用纯 CSS 创作一个菱形 loader 动画
效果预览 按下右侧的“点击预览”按钮可以在当前页面预览,点击链接可以全屏预览。 https://codepen.io/comehope/pen/eKzjqK 可交互视频教程 此视频是可以交互的,你可以随时暂停视频,编辑视频中的代码。 请用 chrome, safari, edge 打开观看。…
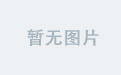
【数据库】SQLite和MySQL之间的对比和选择
目录1、各自特定2、使用场景3、选择哪个1、各自特定 SQLite :独立、简单(零配置);适用于为单个应用程序和设备提供本地数据存储。 MySQL:可伸缩、高并发性;适用于客户端/服务器模式企业数据的共享数据存储…
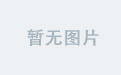
MySql中管理百万级要注意些什么东西(转载)
一、我们可以且应该优化什么? 硬件 操作系统/软件库 SQL服务器(设置和查询) 应 用编程接口(API) 应用程序 二、优化硬件 如果你需要庞大的数据库表 (>2G),你应该考虑使用64位的硬件结构,像Alpha、Sparc或即将推出的IA64。因为MySQL内部使用…
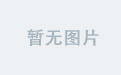
【数据库】sqlite3数据库备份、导出方法汇总
【数据库】sqlite3常用命令及SQL语句 目录1、直接拷贝数据库2、使用.backup .clone1)交互式2)脚本3、导出到csv文件中(其它格式类似)1)交互式2)脚本3)导出成其它格式汇总a> .mode asciib>…
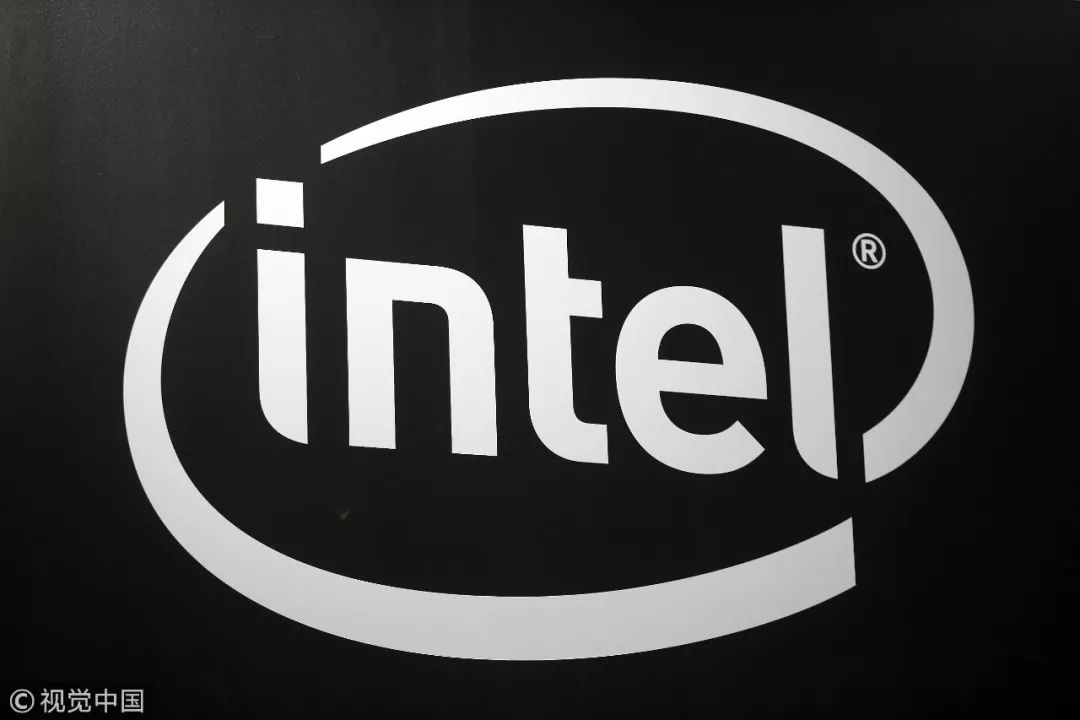
高通与苹果宣布“复合”,英特尔黯然退场 | 极客头条
作者 | 郭芮转载自公众号CSDN(ID:CSDNnews)为期两年的苹果高通“诉讼之争”经历了各种推波助澜愈演愈烈,俨然到了最为关键的白热化阶段,没成想,在刚刚正式进入美国司法庭审环节的两天后却被强势叫停了!4 月…
MQTT 协议 Client ID 长度不能超过23个字符
今天遇到一个MQTT的问题,MqttException: MQIsdp ClientId > 23 bytes ClientId的长度大于23时,无法链接MQTT服务器。 经过查看协议发现:客户端标识符(Client ID)是介于1和23个字符长度,客户端到服务器的唯一标识。它必须在搜有客户端连接到…