【C++】C++11 STL算法(五):设置操作(Set operations)、堆操作(Heap operations)
目录
- 设置操作(Set operations)
- 一、includes
- 1、原型:
- 2、说明:
- 3、官方demo
- 二、set_difference
- 1、原型:
- 2、说明:
- 3、官方demo
- 三、set_intersection
- 1、原型:
- 2、说明:
- 3、官方demo
- 四、set_symmetric_difference
- 1、原型:
- 2、说明:
- 3、官方demo
- 五、set_union
- 1、原型:
- 2、说明:
- 3、官方demo
- 堆操作(Heap operations)
- 一、is_heap
- 1、原型:
- 2、说明:
- 3、官方demo
- 二、is_heap_until
- 1、原型:
- 2、说明:
- 3、官方demo
- 三、make_heap
- 1、原型:
- 2、说明:
- 3、官方demo
- 四、push_heap
- 1、原型:
- 2、说明:
- 3、官方demo
- 五、pop_heap
- 1、原型:
- 2、说明:
- 3、官方demo
- 六、sort_heap
- 1、原型:
- 2、说明:
- 3、官方demo
头文件:#include <algorithm>
设置操作(Set operations)
一、includes
1、原型:
template< class InputIt1, class InputIt2 >
bool includes( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2 );template< class InputIt1, class InputIt2, class Compare >
bool includes( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2, Compare comp );
2、说明:
如果一个集合是另一个集合的子集,则返回true
3、官方demo
#include <iostream>
#include <algorithm>
#include <cctype>
#include <vector>int main()
{std::vector<char> v1 {'a', 'b', 'c', 'f', 'h', 'x'};std::vector<char> v2 {'a', 'b', 'c'};std::vector<char> v3 {'a', 'c'};std::vector<char> v4 {'g'};std::vector<char> v5 {'a', 'c', 'g'};for (auto i : v1) std::cout << i << ' ';std::cout << "\nincludes:\n" << std::boolalpha;for (auto i : v2) std::cout << i << ' ';std::cout << ": " << std::includes(v1.begin(), v1.end(), v2.begin(), v2.end()) << '\n';for (auto i : v3) std::cout << i << ' ';std::cout << ": " << std::includes(v1.begin(), v1.end(), v3.begin(), v3.end()) << '\n';for (auto i : v4) std::cout << i << ' ';std::cout << ": " << std::includes(v1.begin(), v1.end(), v4.begin(), v4.end()) << '\n';for (auto i : v5) std::cout << i << ' ';std::cout << ": " << std::includes(v1.begin(), v1.end(), v5.begin(), v5.end()) << '\n';auto cmp_nocase = [](char a, char b) {return std::tolower(a) < std::tolower(b);};std::vector<char> v6 {'A', 'B', 'C'};for (auto i : v6) std::cout << i << ' ';std::cout << ": (case-insensitive) "<< std::includes(v1.begin(), v1.end(), v6.begin(), v6.end(), cmp_nocase)<< '\n';
}
Output:
a b c f h x
includes:
a b c : true
a c : true
g : false
a c g : false
A B C : (case-insensitive) true
二、set_difference
1、原型:
template< class InputIt1, class InputIt2, class OutputIt >
OutputIt set_difference( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first );template< class InputIt1, class InputIt2, class OutputIt, class Compare >
OutputIt set_difference( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first, Compare comp );
2、说明:
计算两个集合的差:将[first1,last1)中剔除[first1,last2)的元素,输出到d_first中
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>
#include <iterator>int main() {std::vector<int> v1 {1, 2, 5, 5, 5, 9};std::vector<int> v2 {2, 5, 7};std::vector<int> diff;std::set_difference(v1.begin(), v1.end(), v2.begin(), v2.end(), std::inserter(diff, diff.begin()));for (auto i : v1) std::cout << i << ' ';std::cout << "minus ";for (auto i : v2) std::cout << i << ' ';std::cout << "is: ";for (auto i : diff) std::cout << i << ' ';std::cout << '\n';
}
Output:
1 2 5 5 5 9 minus 2 5 7 is: 1 5 5 9
三、set_intersection
1、原型:
template< class InputIt1, class InputIt2, class OutputIt >
OutputIt set_intersection( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first );template< class InputIt1, class InputIt2, class OutputIt, class Compare >
OutputIt set_intersection( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first, Compare comp );
2、说明:
计算两个集合从交集
3、官方demo
#include <iostream>
#include <vector>
#include <algorithm>
#include <iterator>
int main()
{std::vector<int> v1{1,2,3,4,5,6,7,8};std::vector<int> v2{5,7,9,10};std::sort(v1.begin(), v1.end());std::sort(v2.begin(), v2.end());std::vector<int> v_intersection;std::set_intersection(v1.begin(), v1.end(),v2.begin(), v2.end(),std::back_inserter(v_intersection));for(int n : v_intersection)std::cout << n << ' ';
}
Output:
5 7
四、set_symmetric_difference
1、原型:
template< class InputIt1, class InputIt2, class OutputIt >
OutputIt set_symmetric_difference( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first );template< class InputIt1, class InputIt2, class OutputIt, class Compare >
OutputIt set_symmetric_difference( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first, Compare comp );
2、说明:
计算两个集合之间的对称差:两个集合的并集减去它们的交集
3、官方demo
#include <iostream>
#include <vector>
#include <algorithm>
#include <iterator>
int main()
{std::vector<int> v1{1,2,3,4,5,6,7,8 };std::vector<int> v2{ 5, 7, 9,10};std::sort(v1.begin(), v1.end());std::sort(v2.begin(), v2.end());std::vector<int> v_symDifference;std::set_symmetric_difference(v1.begin(), v1.end(),v2.begin(), v2.end(),std::back_inserter(v_symDifference));for(int n : v_symDifference)std::cout << n << ' ';
}
Output:
1 2 3 4 6 8 9 10
五、set_union
1、原型:
template< class InputIt1, class InputIt2, class OutputIt >
OutputIt set_union( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first );template< class InputIt1, class InputIt2, class OutputIt, class Compare >
OutputIt set_union( InputIt1 first1, InputIt1 last1,InputIt2 first2, InputIt2 last2,OutputIt d_first, Compare comp );
2、说明:
计算两个集合的并集
3、官方demo
#include <vector>
#include <iostream>
#include <algorithm>
#include <iterator>int main()
{{std::vector<int> v1 = {1, 2, 3, 4, 5}; std::vector<int> v2 = { 3, 4, 5, 6, 7}; std::vector<int> dest1;std::set_union(v1.begin(), v1.end(),v2.begin(), v2.end(), std::back_inserter(dest1));for (const auto &i : dest1) {std::cout << i << ' ';} std::cout << '\n';}{std::vector<int> v1 = {1, 2, 3, 4, 5, 5, 5}; std::vector<int> v2 = { 3, 4, 5, 6, 7}; std::vector<int> dest1;std::set_union(v1.begin(), v1.end(),v2.begin(), v2.end(), std::back_inserter(dest1));for (const auto &i : dest1) {std::cout << i << ' ';} std::cout << '\n';}
}
Output:
1 2 3 4 5 6 7
1 2 3 4 5 5 5 6 7
堆操作(Heap operations)
一、is_heap
1、原型:
template< class RandomIt >
bool is_heap( RandomIt first, RandomIt last );template< class RandomIt, class Compare >
bool is_heap( RandomIt first, RandomIt last, Compare comp );
2、说明:
判断是否为最大堆:父结点的键值总是大于或等于任何一个子结点的键值时为最大堆
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>int main()
{std::vector<int> v { 3, 1, 4, 1, 5, 9 };std::cout << "initially, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';if (!std::is_heap(v.begin(), v.end())) {std::cout << "making heap...\n";std::make_heap(v.begin(), v.end());}std::cout << "after make_heap, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';
}
Output:
initially, v: 3 1 4 1 5 9
making heap...
after make_heap, v: 9 5 4 1 1 3
二、is_heap_until
1、原型:
template< class RandomIt >
RandomIt is_heap_until( RandomIt first, RandomIt last );template< class RandomIt, class Compare >
RandomIt is_heap_until( RandomIt first, RandomIt last, Compare comp );
2、说明:
找到从first开始最大范围的最大堆
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>int main()
{std::vector<int> v { 3, 1, 4, 1, 5, 9 };std::make_heap(v.begin(), v.end());// 可能把堆搞乱了v.push_back(2);v.push_back(6);auto heap_end = std::is_heap_until(v.begin(), v.end());std::cout << "all of v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';std::cout << "only heap: ";for (auto i = v.begin(); i != heap_end; ++i) std::cout << *i << ' ';std::cout << '\n';
}
Output:
all of v: 9 5 4 1 1 3 2 6
only heap: 9 5 4 1 1 3 2
三、make_heap
1、原型:
template< class RandomIt >
void make_heap( RandomIt first, RandomIt last );
2、说明:
生成堆
3、官方demo
#include <iostream>
#include <algorithm>
#include <functional>
#include <vector>int main()
{std::cout << "Max heap:\n";std::vector<int> v { 3, 2, 4, 1, 5, 9 }; // 最初序列std::cout << "initially, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';std::make_heap(v.begin(), v.end()); // 产生最大堆std::cout << "after make_heap, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';std::pop_heap(v.begin(), v.end()); // 将最大元素移至尾部(待删除)std::cout << "after pop_heap, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';auto top = v.back();v.pop_back(); // 删除尾部元素std::cout << "former top element: " << top << '\n';std::cout << "after removing the former top element, v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n' << '\n';std::cout << "Min heap:\n";std::vector<int> v1 { 3, 2, 4, 1, 5, 9 };std::cout << "initially, v1: ";for (auto i : v1) std::cout << i << ' ';std::cout << '\n';std::make_heap(v1.begin(), v1.end(), std::greater<>{}); // 产生最小堆std::cout << "after make_heap, v1: ";for (auto i : v1) std::cout << i << ' ';std::cout << '\n';std::pop_heap(v1.begin(), v1.end(), std::greater<>{}); // 将最小元素移至尾部(待删除)std::cout << "after pop_heap, v1: ";for (auto i : v1) std::cout << i << ' ';std::cout << '\n';auto top1 = v1.back();v1.pop_back(); // 删除尾部数据std::cout << "former top element: " << top1 << '\n';std::cout << "after removing the former top element, v1: ";for (auto i : v1) std::cout << i << ' ';std::cout << '\n';
}
Output:
Max heap:
initially, v: 3 2 4 1 5 9
after make_heap, v: 9 5 4 1 2 3
after pop_heap, v: 5 3 4 1 2 9
former top element: 9
after removing the former top element, v: 5 3 4 1 2 Min heap:
initially, v1: 3 2 4 1 5 9
after make_heap, v1: 1 2 4 3 5 9
after pop_heap, v1: 2 3 4 9 5 1
former top element: 1
after removing the former top element, v1: 2 3 4 9 5
四、push_heap
1、原型:
template< class RandomIt >
void push_heap( RandomIt first, RandomIt last );template< class RandomIt, class Compare >
void push_heap( RandomIt first, RandomIt last, Compare comp );
2、说明:
将位于last-1的元素插入到[first, last-1)中,并保持最大堆。在此之前需要执行push_back将新元素添加到尾部。
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>int main()
{std::vector<int> v { 3, 1, 4, 1, 5, 9 };std::make_heap(v.begin(), v.end());std::cout << "v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';v.push_back(6);std::cout << "before push_heap: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';std::push_heap(v.begin(), v.end());std::cout << "after push_heap: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';
}
Output:
v: 9 5 4 1 1 3
before push_heap: 9 5 4 1 1 3 6
after push_heap: 9 5 6 1 1 3 4
五、pop_heap
1、原型:
template< class RandomIt >
void pop_heap( RandomIt first, RandomIt last );template< class RandomIt, class Compare >
void pop_heap( RandomIt first, RandomIt last, Compare comp );
2、说明:
交换位于first和last-1的值,然后使子范围[first, last-1)成为堆。
等于把最大堆中第一个元素即最大的值“删除”,这里的删除是加引号,因为没有真正删除。紧接着使用pop_back才能真正删除最大的元素。
3、官方demo
#include <iostream>
#include <algorithm>
#include <vector>int main()
{std::vector<int> v { 3, 1, 4, 1, 5, 9 };std::make_heap(v.begin(), v.end());std::cout << "v: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';std::pop_heap(v.begin(), v.end()); // 把最大的元素移到最后,此时还没有删除std::cout << "after pop_heap: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';int largest = v.back();v.pop_back(); // 在这里会真正删除最大元素。std::cout << "largest element: " << largest << '\n';std::cout << "heap without largest: ";for (auto i : v) std::cout << i << ' ';std::cout << '\n';
}
Output:
v: 9 5 4 1 1 3
after pop_heap: 5 3 4 1 1 9
largest element: 9
heap without largest: 5 3 4 1 1
六、sort_heap
1、原型:
template< class RandomIt >
void sort_heap( RandomIt first, RandomIt last );template< class RandomIt, class Compare >
void sort_heap( RandomIt first, RandomIt last, Compare comp );
2、说明:
将最大堆转换成升序排列
3、官方demo
#include <algorithm>
#include <vector>
#include <iostream>int main()
{std::vector<int> v = {3, 1, 4, 1, 5, 9}; std::make_heap(v.begin(), v.end());std::cout << "heap:\t";for (const auto &i : v) {std::cout << i << ' ';} std::sort_heap(v.begin(), v.end());std::cout << "\nsorted:\t";for (const auto &i : v) { std::cout << i << ' ';} std::cout << '\n';
}
Output:
heap: 9 4 5 1 1 3
sorted: 1 1 3 4 5 9
相关文章:
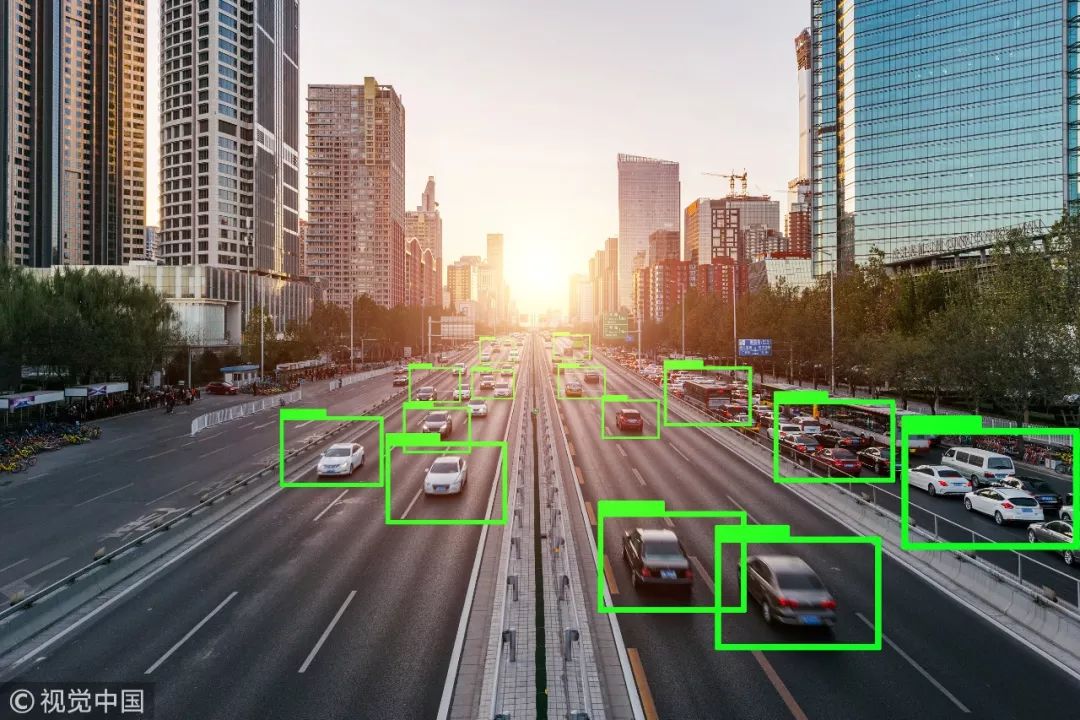
63万张!旷视发布最大物体检测数据集Objects365 | 技术头条
编辑 | 琥珀来源 | AI科技大本营(id:rgznai100)昨日,在旷视科技联合北京智源人工智能研究院举办的发布会上,旷视研究院发布了物体检测数据集 Objects365,包含 63 万张图像数量,365 个类别数量&a…
(一)Android Studio 安装部署 华丽躲坑
叨叨两句先 小宇之前一直做前后端开发,只是略懂JS,未接触过Java和Android 近期工作任务也是兴趣使然,开始琢磨DJI二次开发 DJI是我最服气的无人机厂商,无人机稳定性极强,性价比狂高,还给了极度丰富的二次开…
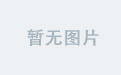
linux 环境配置 安装jdk
一. 下载jdk5.0 for linux 到sun的主页 http://java.sun.com/j2se/1.5.0/download.jsp 下载jdk安装文件jdk-1_5_0_05-linux-i586.bin 二. 解压安装jdk 在shell终端下进入jdk-1_5_0_05-linux-i586.bin文件所在目录,执行命令 ./jdk-1_5_0_05-linux-i586.bin 这时会出现…
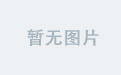
【C++】C++11 STL算法(六):最小/最大操作(Minimum/maximum operations)、比较运算(Comparison operations)
目录最小/最大操作(Minimum/maximum operations)一、max1、原型:2、说明:3、官方demo二、max_element1、原型:2、说明:3、官方demo三、min1、原型:2、说明:3、官方demo四、min_element1、原型:2…
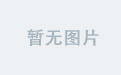
springboot之定时任务
定时线程 说到定时任务,通常会想到JDK自带的定时线程来执行,定时任务。 回顾一下定时线程池。 public static ScheduledExecutorService newScheduledThreadPool(int var0) {return new ScheduledThreadPoolExecutor(var0);}public static ScheduledExec…
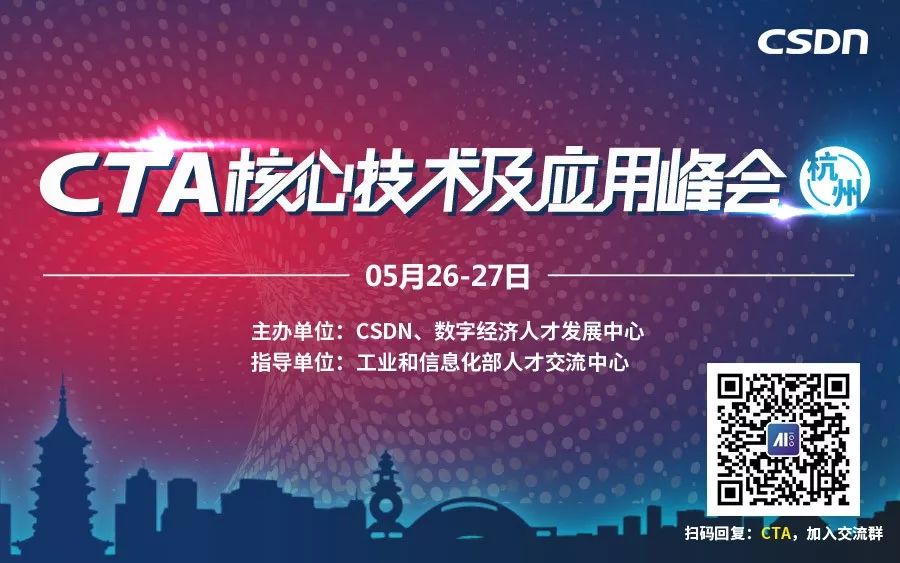
10只机器狗拉卡车!井然有序,毫不费力 | 极客头条
整理 | 琥珀出品 | AI科技大本营(ID:rgznai100)看来,这家娱乐网友多年的机器人公司终于要开始实现商用化了!最先备受期待的是它的网红机器狗 SpotMini。今日凌晨,据多家外媒报道,波士顿动力 (Boston Dynami…
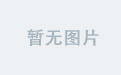
linux下查看nginx,apache,mysql,php的编译参数
有时候nginx,apache,mysql,php编译完了想看看编译参数可以用以下方法 nginx编译参数: #/usr/local/nginx/sbin/nginx -V nginx version: nginx/0.6.32 built by gcc 4.1.2 20071124 (Red Hat 4.1.2-42) configure arguments: --us…
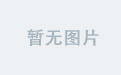
【C++】C++11 STL算法(七):排列操作(Permutation operations)、数值操作(Numeric operations)
排列操作(Permutation operations) 一、is_permutation 1、原型: template< class ForwardIt1, class ForwardIt2 > bool is_permutation( ForwardIt1 first1, ForwardIt1 last1, ForwardIt2 first2 );template< class ForwardIt…
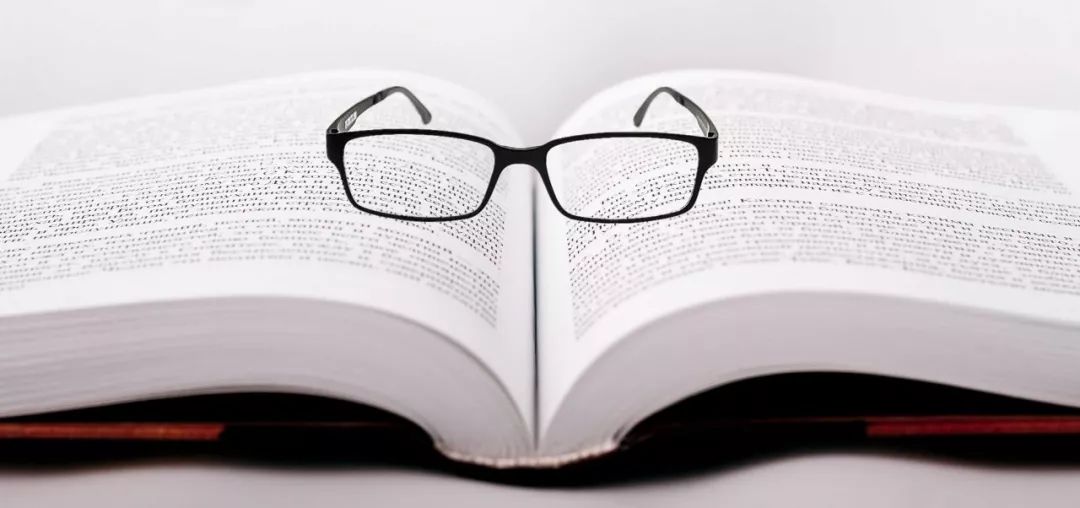
码书:入门中文NLP必备干货:5分钟看懂“结巴”分词(Jieba)
导读:近年来,随着NLP技术的日益成熟,开源实现的分词工具越来越多,如Ansj、盘古分词等。在本文中,我们选取了Jieba进行介绍和案例展示,主要基于以下考虑:社区活跃。截止本文发布前,Ji…
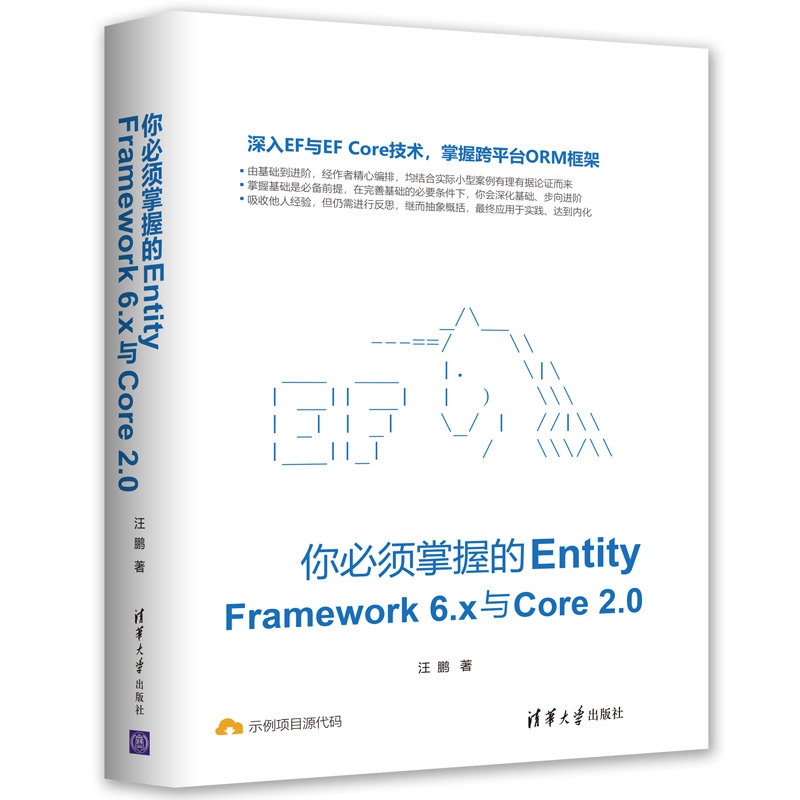
《你必须掌握的Entity Framework 6.x与Core 2.0》正式出版感想
前言 借书正式出版之际,完整回顾下从写博客到写书整个历程,也算是对自己近三年在技术上的一个总结,整个历程可通过三个万万没想到来概括,请耐心阅读。 写博、写书完整历程回顾 从2013年12月注册博客园账号,注册博客园账…
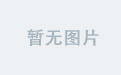
JSF实现“Hello World!”
我们编写一个在页面上显示是“Hello World! ”,我们至少需要编写一个Page对象和一个对应模板文件(tml)。 第一步,Page对象编写 在Tapestry5中Page是与一个页面对应的POJO对象,它不需要继承Tapestry框架的任何基类或实现…
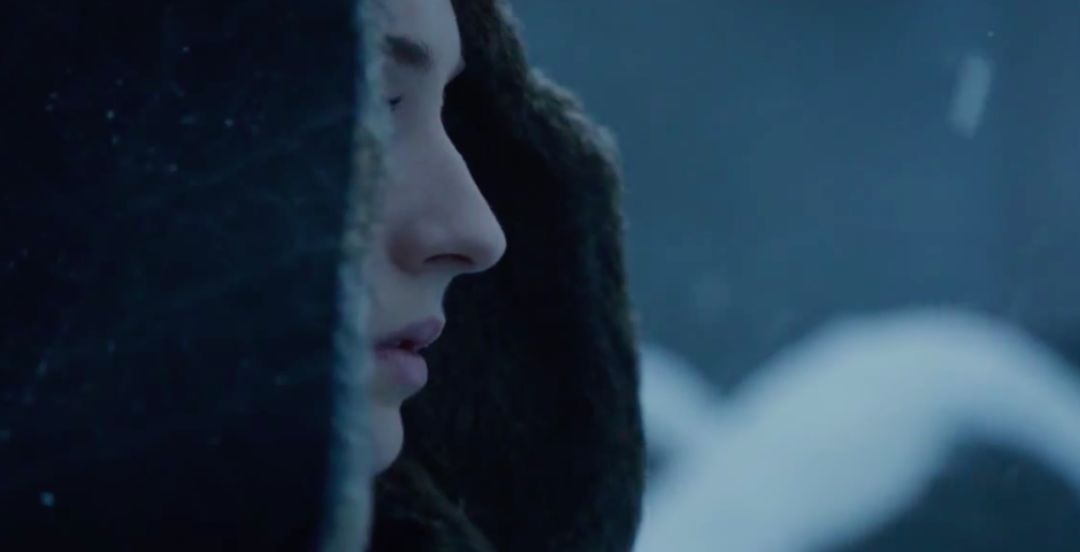
《权力的游戏》最终季上线!谁是你最喜爱的演员?这里有一份Python教程 | 附源码...
译者 | 刘畅编辑 | 琥珀出品 | AI科技大本营(id:rgznai100)《权力的游戏》最终季已于近日开播,对于全世界翘首以待的粉丝们来说,其最大的魅力就在于“无法预知的人物命运”。那些在魔幻时代的洪流中不断沉浮的人们&…
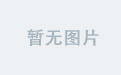
【C++】C++11 STL算法(八):对未初始化内存的操作(Operations on uninitialized memory)、C库(C library)
对未初始化内存的操作(Operations on uninitialized memory) 一、uninitialized_copy 1、原型: template< class InputIt, class ForwardIt > ForwardIt uninitialized_copy( InputIt first, InputIt last, ForwardIt d_first );2、…

OSPF高级设置实现全网互通
OSPF(开放式最短路径优先)是对链路状态路由协议的一种实现,隶属内部网关协议(IGP),故运作于自治系统内部(AS)。采用戴克斯特拉算法(Dijkstras algorithm)被用来计算最短路径树。“Cost”作为路由度量值。链…

学习PHP ?
学PHP的决定真的是好的吗? 不怕又再错一次了吗? 已经是最后的一年半上学时间了.... 真的不愿再走之前那条失败的路,不愿,真的不愿; 这年半无论如何都要把一样技术搞精了 一年半的时间,对我来讲够了....只看…
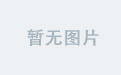
【数据库】sqlite中的限制:数据库大小、表数、列数、行数、参数个数、连接数等
目录一、参考网址二、详解1、查看、设置sqlite限制命令.limit2、SQLite中的限制汇总1)字符串或BLOB的最大长度2)最大列数3)SQL语句的最大长度4)联接中的最大表数5)表达式树的最大深度6)函数的最大参数个数7…
flutter中的生命周期
前言 和其他的视图框架比如android的Activity一样,flutter中的视图Widget也存在生命周期,生命周期的回调函数提现在了State上面。理解flutter的生命周期,对我们写出一个合理的控件至关重要。组件State的生命周期整理如下图所示: 大…
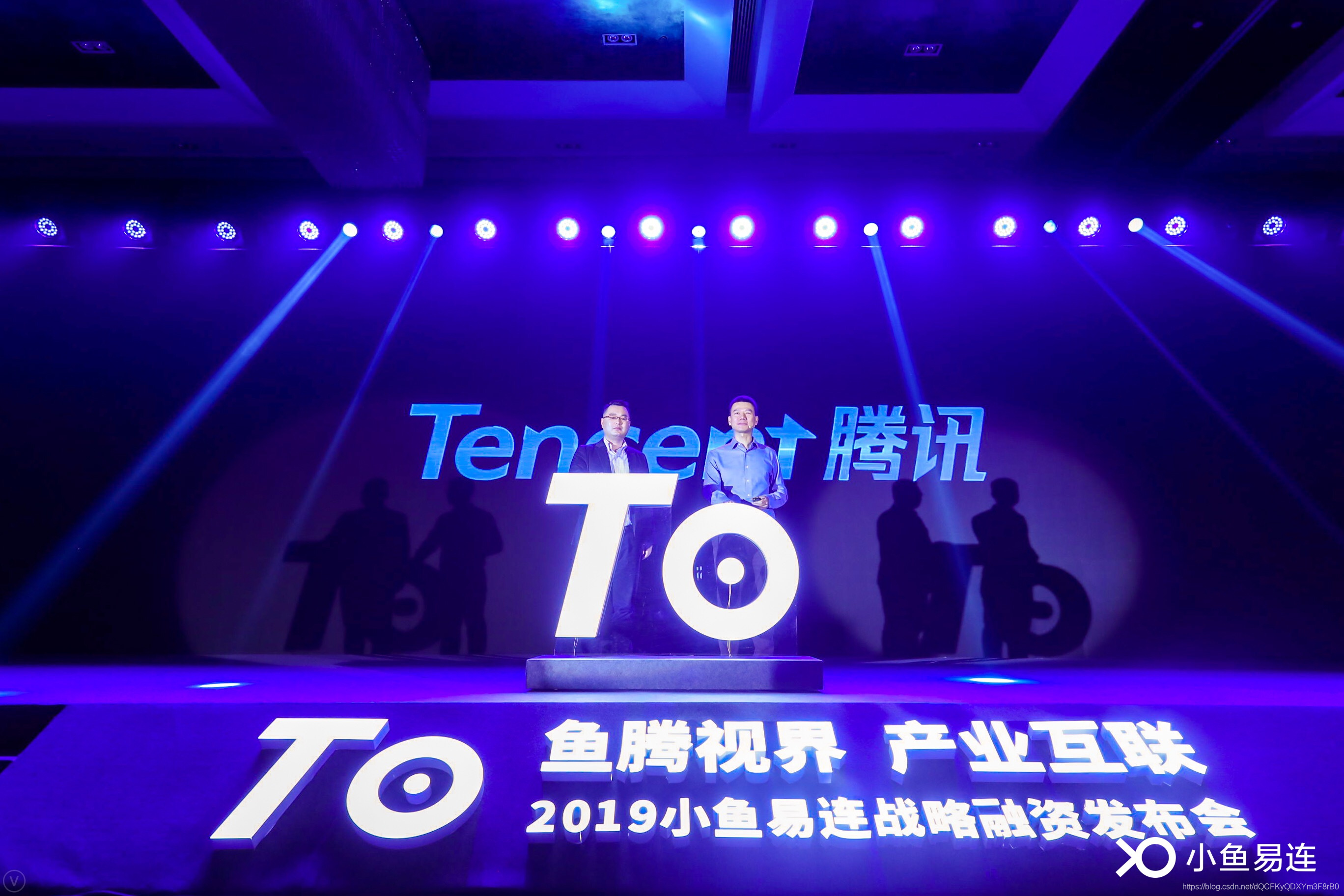
小鱼易连获腾讯数亿C轮投资,云视频布局产业互联网
4 月 18 日,小鱼易连在北京举行 “鱼腾视界 产业互联” 战略合作暨融资发布会上,正式宣布获得 C 轮融资,由腾讯领投。融得的资金将全面用于小鱼易连云视频系统在产业互联网领域的落地,打通企业、政府、个人三者之间的柔性生态全产…
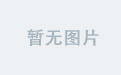
异步IO一定更好吗?
http://cnodejs.org/blog/?p1015续:异步IO一定更好吗?我之前的一篇文章《异步IO一定更好吗?》中举了一个很变态的例子,用以说明在单碟机械式硬盘上异步IO反而可能降低性能的问题,大家的讨论很热烈。前天的NodeParty杭…
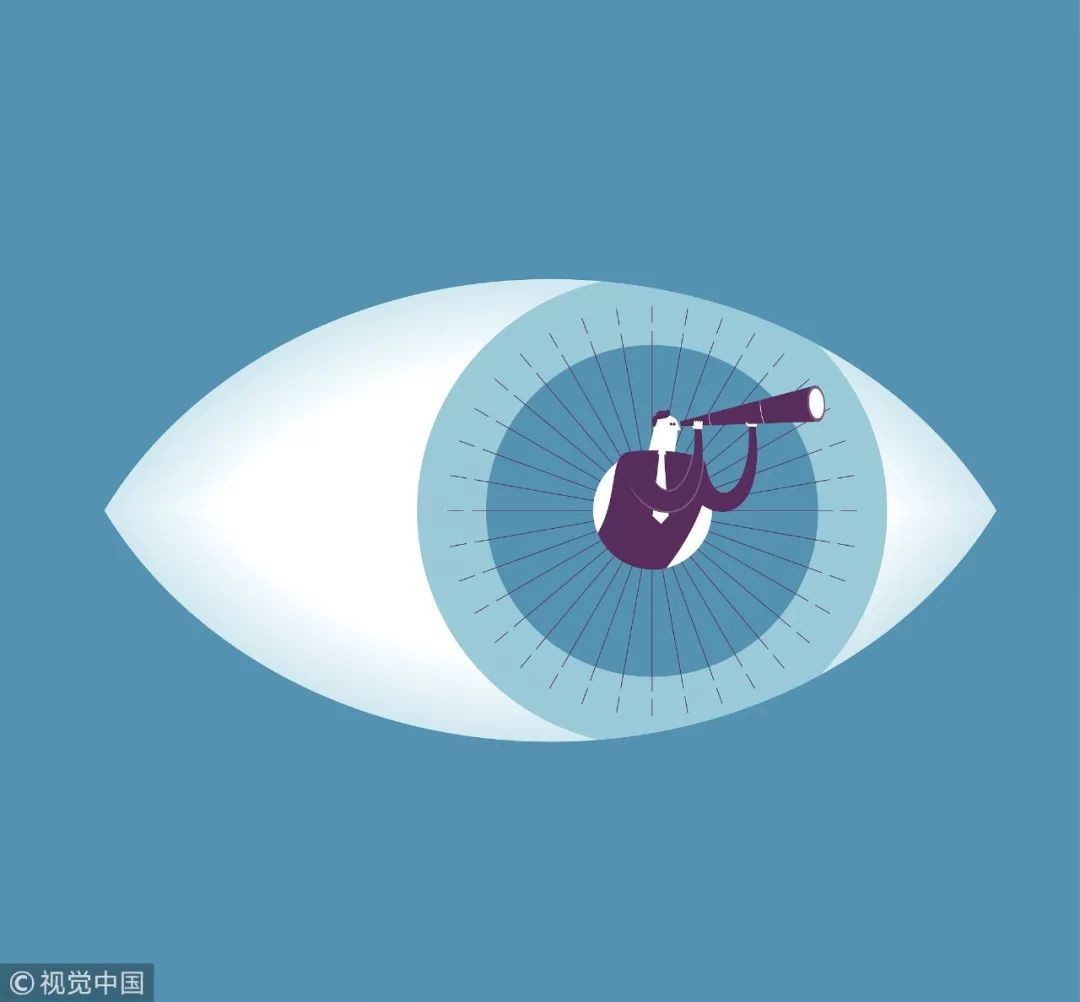
谈谈Python那些不为人知的冷知识(二)
本文转载自Python的编程时光(ID:Python-Time)小明在日常Code中遇到一些好玩,冷门的事情,通常都会记录下来。从上一篇的分享来看,仍然有不少 Pythoner 对这些冷知识存在盲区,所以今天迎来第二篇。如果上篇你…
前端每日实战:45# 视频演示如何用纯 CSS 创作一个菱形 loader 动画
效果预览 按下右侧的“点击预览”按钮可以在当前页面预览,点击链接可以全屏预览。 https://codepen.io/comehope/pen/eKzjqK 可交互视频教程 此视频是可以交互的,你可以随时暂停视频,编辑视频中的代码。 请用 chrome, safari, edge 打开观看。…
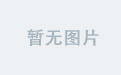
【数据库】SQLite和MySQL之间的对比和选择
目录1、各自特定2、使用场景3、选择哪个1、各自特定 SQLite :独立、简单(零配置);适用于为单个应用程序和设备提供本地数据存储。 MySQL:可伸缩、高并发性;适用于客户端/服务器模式企业数据的共享数据存储…
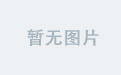
MySql中管理百万级要注意些什么东西(转载)
一、我们可以且应该优化什么? 硬件 操作系统/软件库 SQL服务器(设置和查询) 应 用编程接口(API) 应用程序 二、优化硬件 如果你需要庞大的数据库表 (>2G),你应该考虑使用64位的硬件结构,像Alpha、Sparc或即将推出的IA64。因为MySQL内部使用…
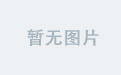
【数据库】sqlite3数据库备份、导出方法汇总
【数据库】sqlite3常用命令及SQL语句 目录1、直接拷贝数据库2、使用.backup .clone1)交互式2)脚本3、导出到csv文件中(其它格式类似)1)交互式2)脚本3)导出成其它格式汇总a> .mode asciib>…
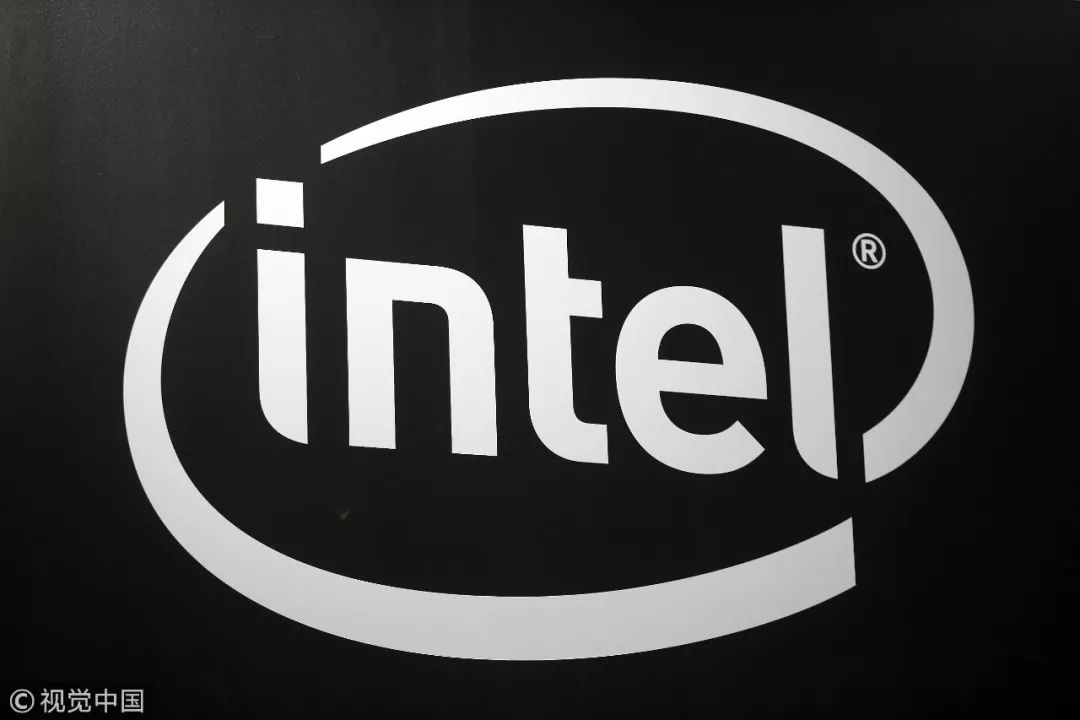
高通与苹果宣布“复合”,英特尔黯然退场 | 极客头条
作者 | 郭芮转载自公众号CSDN(ID:CSDNnews)为期两年的苹果高通“诉讼之争”经历了各种推波助澜愈演愈烈,俨然到了最为关键的白热化阶段,没成想,在刚刚正式进入美国司法庭审环节的两天后却被强势叫停了!4 月…
MQTT 协议 Client ID 长度不能超过23个字符
今天遇到一个MQTT的问题,MqttException: MQIsdp ClientId > 23 bytes ClientId的长度大于23时,无法链接MQTT服务器。 经过查看协议发现:客户端标识符(Client ID)是介于1和23个字符长度,客户端到服务器的唯一标识。它必须在搜有客户端连接到…
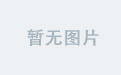
【数据库】适用于SQLite的SQL语句(一)
目录一、统计函数二、表TABLE1、创建表CREATE TABLE2、更改表ALTER TABLE3、删除表DROP TABLE三、分析表ANALYZE四、附加数据库 ATTACH DATABASE五、事务六、核心函数七、索引INDEX1、创建索引:CREATE INDEX2、查看索引:3、使用索引 INDEXED BY4、删除索…
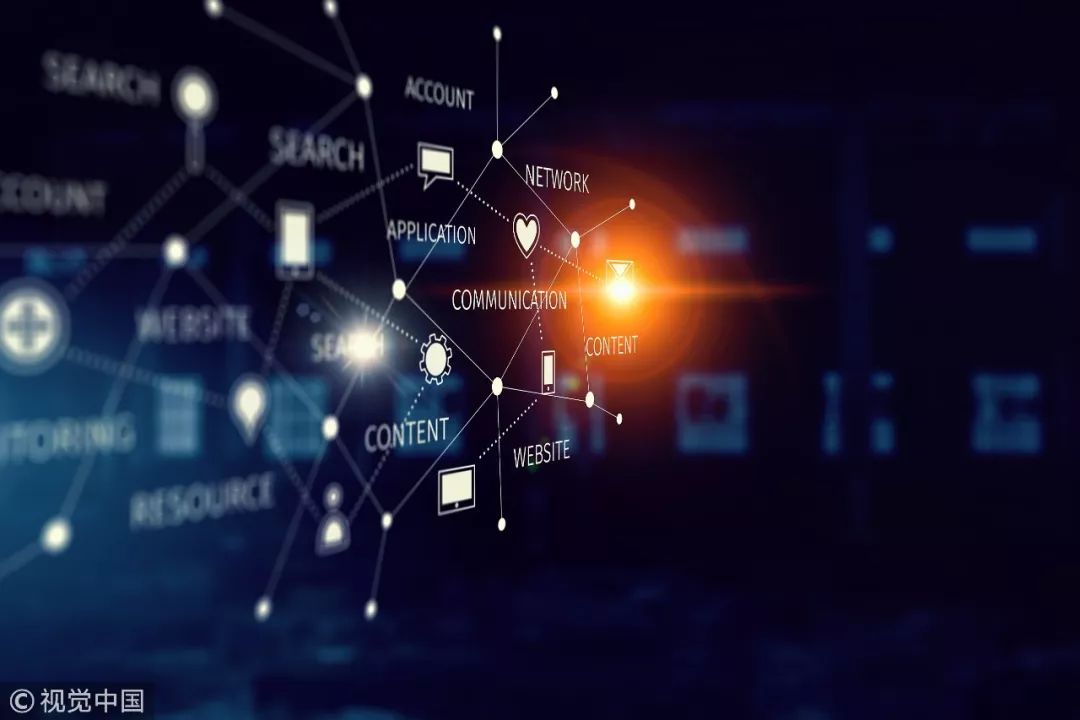
谷歌大神Jeff Dean点赞网红博士论文:改进分布式共识机制 | 技术头条
作者 | Heidi Howard编译 | 刘静本文转载自公众号图灵TOPIA(ID:turingtopia)本文作者Heidi Howard,是剑桥大学计算机科学与技术系系统研究小组的分布式系统研究员。Heidi的研究领域一直围绕分布式系统中的一致性,容错性和性能并且…
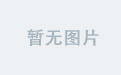
使用Nginx做前端服务器时让Apache得到真实IP的方法
一:nginx.conf proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; 其实这个proxy.conf里面默认都有,在nginx.conf使用include proxy.conf就可以 二:apa…
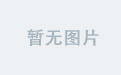
Hadoop生态圈-hive五种数据格式比较
Hadoop生态圈-hive五种数据格式比较 作者:尹正杰 版权声明:原创作品,谢绝转载!否则将追究法律责任。