Swift 代码调试-善用XCode工具(UI调试,五种断点,预览UIImage...)
原创Blog,转载请注明出处
http://blog.csdn.net/hello_hwc?viewmode=list
我的stackoverflow
工欲善其事,必先利其器,强烈建议新手同学好好研究下XCode这个工具。比如Build Settings,Build Info Rules,Build Parse, Edit Scheme…
前言:这个Swift调试系列分为四篇
- 图形化界面调试
- LLDB常用命令
- LLDB进阶使用
- Zombie等其他调试
2015.12月和2016.1月主要更新iOS开发的设计模式和Instruments优化技巧,穿插着写一些别的。
断点
断点是调试中经常用到的,让代码停止在错误出现的地方,看看变量以及上下文实际的变化,往往就能够找到问题所在。
点击左侧部分就可以添加断点,再单机可以禁用单个断点
导航栏中的断点列表
可以右键来禁用,编辑,删除断点。
断点上下文
让我们来看看图中的四个区域
- 这里可以看到CPU,内存,磁盘以及网络情况。注意,只有在实际设备上才是有意义的
- 线程信息,可以看到当前停在main Queue上,app运行的时候也启动了其他几个队列。注意,调试的时候看看代码运行的线程是否正确很有必要
- 这里可以看到Local变量,Swift有个好处是按照Module来划分了变量,简单粗暴
- 这个区域是LLDB调试区域,可以用LLDB命令执行任何动态的代码
我们着重来看下3,4区域,新手往往只会看变量,其实这里有很多可以利用的信息
其中
A. 禁用,启用所有断点
B. 继续执行
C. 跳过这一行
D. step in(例如进入到函数实现内部)
E. step out (退出step in)
F. 打开UI调试 (后问会详细阐述)
G.模拟位置
H.选择线程
I. 查看线程调用堆栈
其中I的截图如下
条件断点
举个例子,我想停在第888次执行?总不能一次次的continue吧!
右键断点,选择edit breakpoint
然后填写条件
condition就是代码触发的条件
ignore就是在断点触发前忽略几次
action是断点触发后,执行的LLDB动作,这里很简单就是打印当前的sum
options,执行完action后是否继续执行
可以看到运行的截图
准备工作
接下来讲的几种断点添加方式都是,在断点导航底部,如图添加
Swift Error 断点
这个在Swift开发中很常用
添加一个Swift Error断点
定义一个方法,来抛出Swift Error
然后,这样调用
会发现,在Swift Error发生的时候,断点触发
当然,Swift Error断点也支持编辑来捕获指定类型的Error
为了方便读者阅读,图片我没加水印,转发者请注明转自 Leo的CSDN博客(http://blog.csdn.net/hello_hwc?viewmode=list)
Exception断点
在抛出异常的时候触发
这个在iOS开发中很常用
有过iOS开发的都知道,Cocoa在错误的时候会抛出异常,而实用这个断点,会帮助我们捕获异常。
例如
随便performSelector,会抛出异常
在添加了All Exception后,会停在这里
Symbol断点
停在不方便直接加断点的地方
例如,停在
如图
然后,这样调用
会发现断点触发
Test Failure断点
这个就是使用XCTest框架来测试的时候,当Test Case的Assert失败的时候触发的断点。这里不截图了
变量图片预览
红圈中左边是预览,右边是打印Description
UI调试
如何打开UI调试
图中的红圈部分,点击后,整个调试区域如图
其中
- 用来查看View的层次结构,树状图
- View的可视区域,可以详细的看到View的叠加关系
- 选中某一个View后的属性
查看AutoLayout约束
右键某一个View
然后选择Show Constraints,
注意,第一张图右上角的地址
这里先记着,这个地址对LLDB的调试很有用,下一篇我会讲到
相关文章:
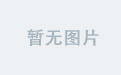
Linux下getopt_long函数的使用
getopt_long为解析命令行参数函数,它是Linux C库函数。使用此函数需要包含系统头文件getopt.h。 getopt_long函数声明如下: int getopt_long(int argc, char * const argv[], const char *optstring, const struct option *longopts, int *longindex);…
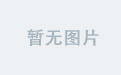
Expect自动化控制简单介绍
telnet,ftp,Passwd,fsck,rlogin,tip,ssh等等。该工具利用Unix伪终端包装其子进程,允许任意程序通过终端接入进行自动化控制;也可利用Tk工具,将交互程序包装在X11的图形用…
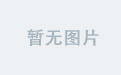
C++中标准模板库std::vector的实现
以下实现了C标准模板库std::vector的部分实现,参考了 cplusplus. 关于C中标准模板库std::vector的介绍和用法可以参考 https://blog.csdn.net/fengbingchun/article/details/51510916 实现代码vector.hpp内容如下: #ifndef FBC_STL_VECTOR_HPP_ #defi…
Swift学习 OOP三大特性:继承、多态、封装
先看个例子 从上面的例子可以总结那么一句话:”学生是人”。也就是Student类继承People类。简而言之,学生是人,这句话是说得通的,但是”人是学生”这句话是说不通的,不是学生就不是人了嘛? 从代码中,我们可以看出S…
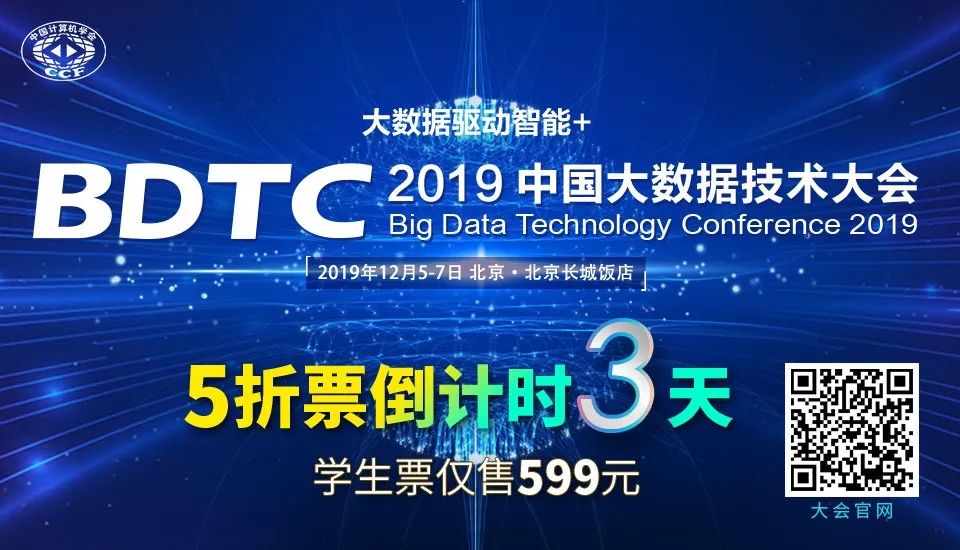
5折票倒计时3天 | 超干货议程首度曝光!2019 中国大数据技术大会邀您共赴
(大会官网https://t.csdnimg.cn/U1wA)2019年,大数据与人工智能的热度已经蔓延到了各个领域,智能交通、AIoT、智慧城市,智慧物流、AI中台、工业制造等各种黑科技成为热搜名词。而在今年的乌镇互联网大会上,大…
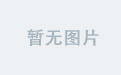
mysql select * f
mysql> select * from tb;-------------| id | name |-------------| 1 | tbone || 3 | 2d2 || 5 | 55 || 6 | 66 |-------------4 rows in set (0.00 sec)转载于:https://www.cnblogs.com/bashala/p/3974088.html
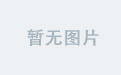
C++/C++11中用于定义类型别名的两种方法:typedef和using
类型别名(type alias)是一个名字,它是某种类型的同义词。使用类型别名有很多好处,它让复杂的类型名字变得简单明了、易于理解和使用,还有助于程序员清楚地知道使用该类型的真实目的。在C中,任何有效类型都可以有别名。 有两种方法…
iOS学习笔记--01swift实现提示框第三方库:MBProgressHUD
本文使用swift语言使用MBProgressHUD。 开源项目MBProgressHUD可以实现多种形式的提示框。使用简单,方便。GitHud的下载地址是:https://github.com/jdg/MBProgressHUD/ 下载完成后,将MBProgressHUD.h和MBProgressHUD.m拖入已经新建好的Swift项…
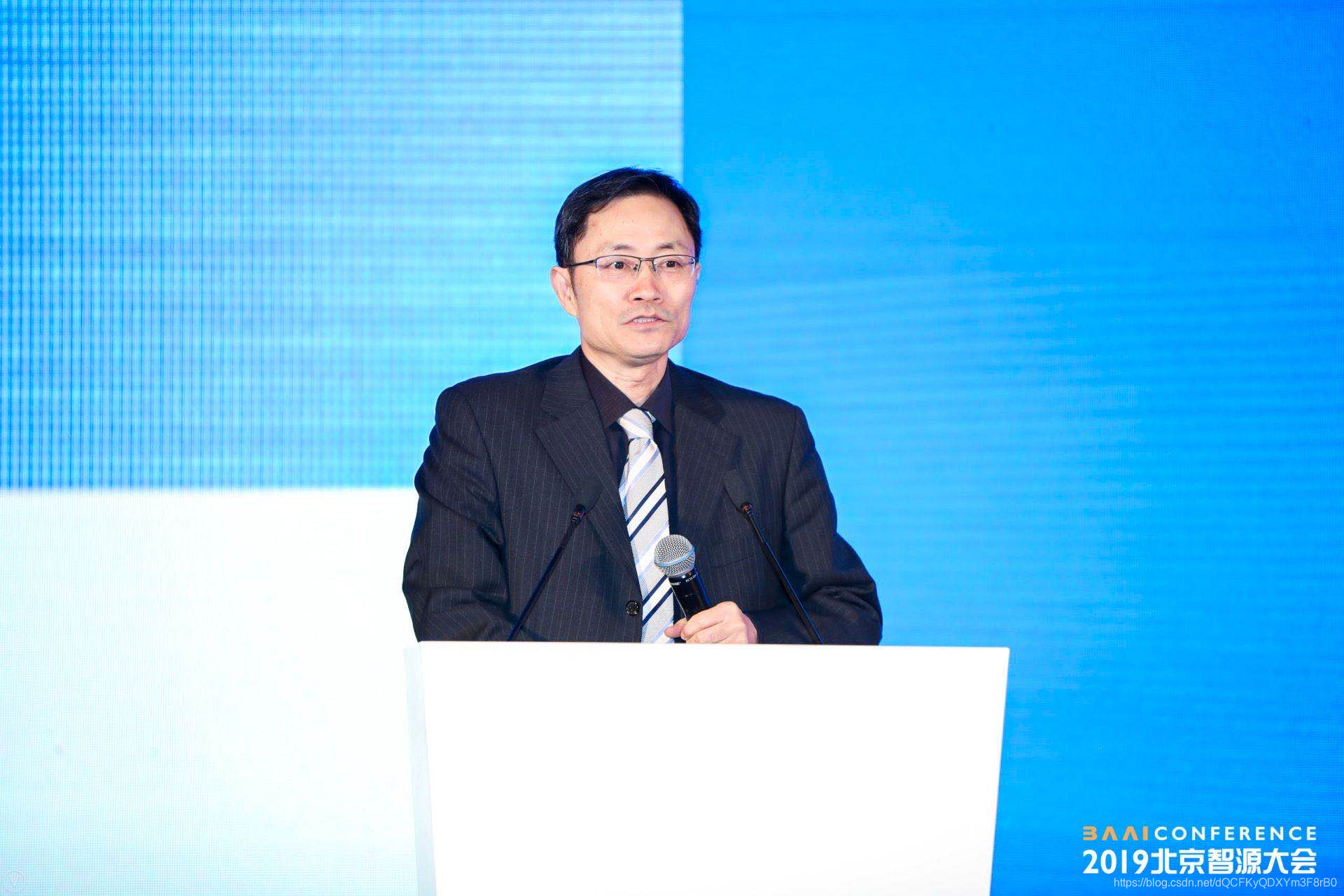
2019北京智源大会在京开幕, 中外学术大咖共话人工智能研究前沿
10月31日,由北京智源人工智能研究院主办的2019北京智源大会在国家会议中心开幕,会期两天。智源大会是北京创建全球人工智能学术和创新最优生态的标志性学术活动,定位于“内行的AI盛会”,以国际性、权威性、专业性和前瞻性为特色&a…
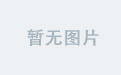
linux中登录类型及配置文件
linux中登录shell的类型1.交互式登录:直接通过终端输入用户信息登录1)login:2)在shell中 su - usernamesu -l username2.非交互式登录1)su username2)图形界面的终端3)执行脚本的过程用户配置文…

Swift项目引入第三方库的方法
分类:iOS(55) 目录(?)[] Swift项目引入第三方库的方法 转自 http://blog.shiqichan.com/How-To-Import-3rd-Lib-Into-Swift-Project/ 以下,将创建一个Swift项目,然后引入3个库: Snappy 简化autolayout代码…
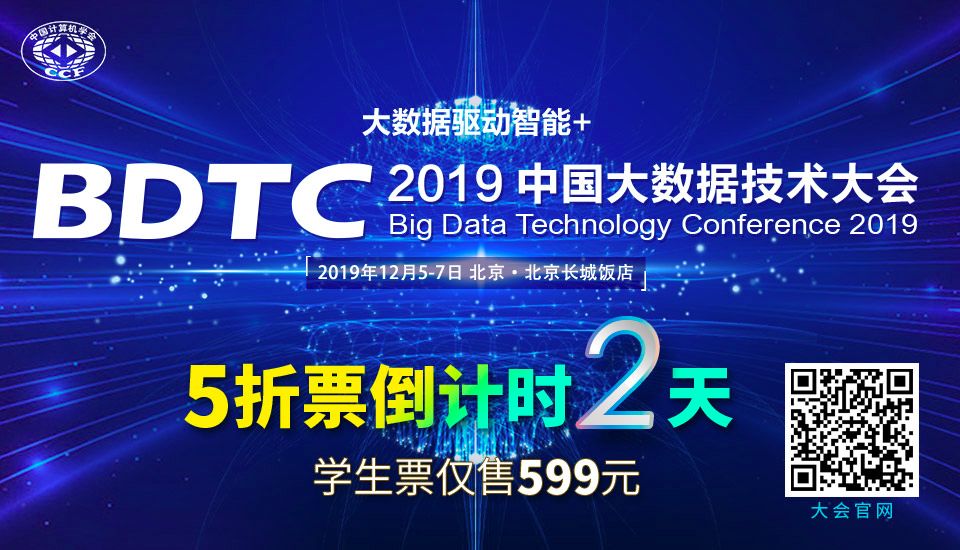
最新NLP架构的直观解释:多任务学习– ERNIE 2.0(附链接)| CSDN博文精选
作者 | Michael Ye翻译 | 陈雨琳,校对 | 吴金笛来源 | 数据派THU(ID:DatapiTHU)百度于今年早些时候发布了其最新的NLP架构ERNIE 2.0,在GLUE基准测试中的所有任务上得分均远高于XLNet和BERT。NLP的这一重大突破利用了一…
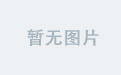
C++中的内存对齐介绍
网上有很多介绍字节对齐或数据对齐或内存对齐的文章,虽然名字不一样,但是介绍的内容大致都是相同的。这里以内存对齐相称。注:以下内容主要来自网络。 内存对齐,通常也称为数据对齐,是计算机对数据类型合法地址做出了…
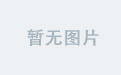
__cplusplus的用处
经常在/usr/include目录下看到这种字句: #ifdef __cplusplus extern "C" { #endif ... #ifdef __cplusplus } #endif 不太明白是怎么用的。今天阅读autobook,在第53页看到了作者的解释:C/C编译器对函数和变量名的命名方法不一样…
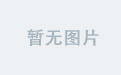
Linux下的内存对齐函数
在Linux下内存对齐的函数包括posix_memalign, aligned_alloc, memalign, valloc, pvalloc,其各个函数的声明如下: int posix_memalign(void **memptr, size_t alignment, size_t size); void *memalign(size_t alignment, size_t size); void *aligned_…
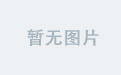
Swift2.0系列]Error Handling(项目应用篇)
1.FileManager中的应用 倘若你只是想看FileManager中的 Error Handling是如何实现的,请找到3.删除文件以及4.获取文件信息。我分别为你提供了do-catch以及try?的使用方法。 打开Xcode,选中Single View Application,输入项目名称例如FileManagerDemo,点击…
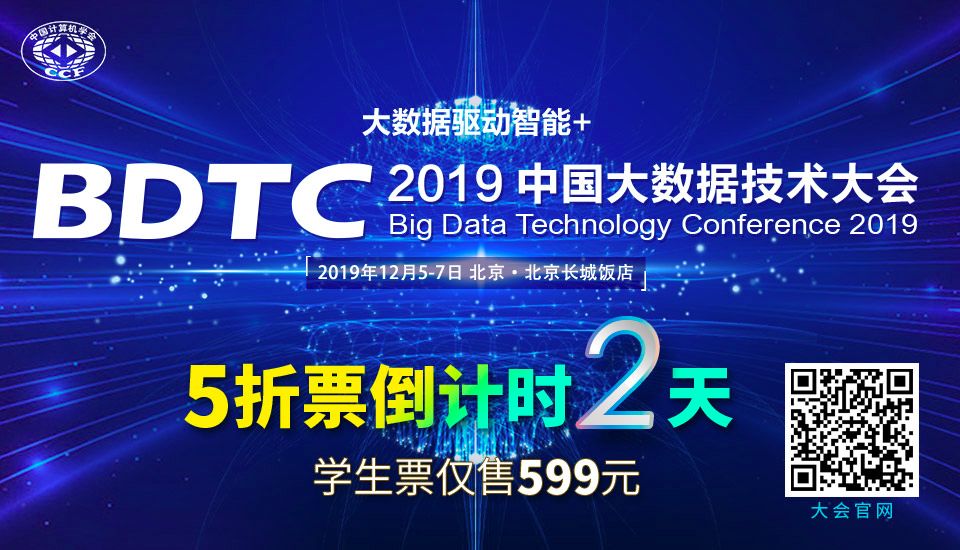
总点第一个视频产生选择偏差?Youtube用“浅塔”来纠正
作者 | Tim Elfrink译者 | Tianyu出品 | AI科技大本营(ID:rgznai100)【导读】本文来自于谷歌研究人员最近发表的一篇论文,介绍了视频平台 Youtube 的视频推荐方法,并在 RecSys 2019 大会上做了分享。本文总结归纳了一些论文中的重…

HTML样式offset[Direction] 和 style.[direction]的区别
为什么80%的码农都做不了架构师?>>> 以offsetLeft与style.left为例: offsetLeft使用的值是字符串,如“100px", style.left则使用数值,如 100 offsetLeft只可以读,因此用无法通过Js改变这个值实现样…
Ubuntu 14.04上安装pip3/numpy/matplotlib/scipy操作步骤
Ubuntu 14.04 64位上默认安装了两个版本的python,一个是python2.7.6,另外一个是python3.4.0,如下图所示: 安装完pip3的结果如下图所示: 升级完pip3的结果如下图所示: 安装完numpy的结果如下图所示: 通过sudo pip3 install matplot…
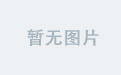
NSHelper.showAlertTitle的两种用法 swift
var model : CarCity CarCity() if (NSString.isNullOrEmpty(locationLabel.text)) { NSHelper.showAlertTitle(nil, message: "暂无法定位,请检查网络。", cancel: "确定") return } if (NSString.isNullOrEmpty(plateTextFild.text)) { NSHe…
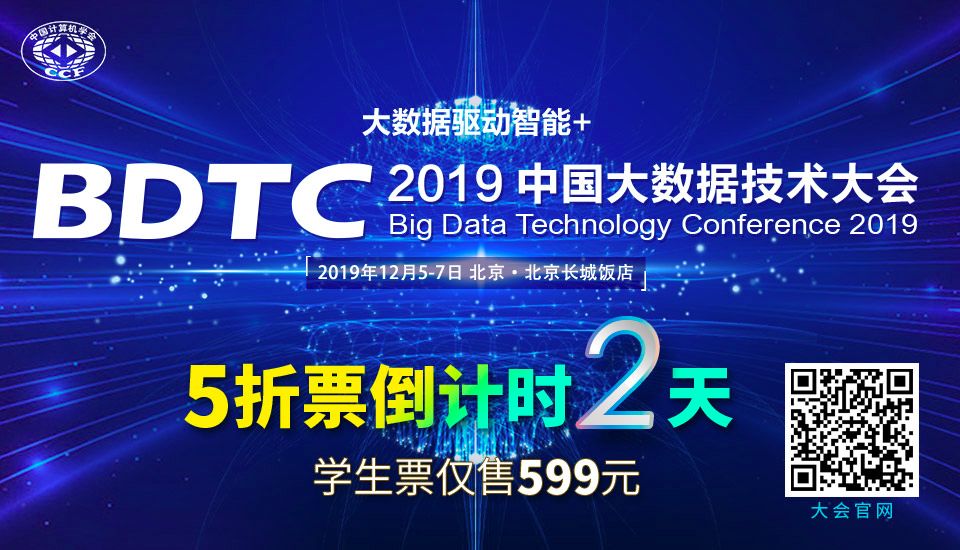
通俗易懂:图卷积神经网络入门详解
作者 | 蝈蝈来源 | 转载自知乎用户蝈蝈【导读】GCN问世已经有几年了(2016年就诞生了),但是这两年尤为火爆。本人愚钝,一直没能搞懂这个GCN为何物,最开始是看清华写的一篇三四十页的综述,读了几页就没读了&a…

Java数据结构一 —— Java Collections API中的表
1.Collection接口 位于java.util包中,以下是重要的部分。 1 public interface Collection<AnyType> extends Iterable<AnyType> 2 { 3 int size(); 4 boolean isEmpty(); 5 void clear(); 6 boolean add(AnyType x); 7 …

Swift 中的内存管理详解
这篇文章是在阅读《The Swift Programming Language》Automatic Reference Counting(ARC,自动引用计数)一章时做的一些笔记,同时参考了其他的一些资料。 在早期的 iOS 开发中,内存管理是由开发者手动来完成的。因为传统…
Ubuntu14.04 64位机上配置OpenCV3.4.2+OpenCV_Contrib3.4.2+Python3.4.3操作步骤
Ubuntu 14.04 64位上默认安装了两个版本的python,一个是python2.7.6,另外一个是python3.4.3。这里使用OpenCV最新的稳定版本3.4.2在Ubuntu上安装python3.4.3支持OpenCV的操作步骤如下: 1. 更新包,执行: sudo apt-get update sud…
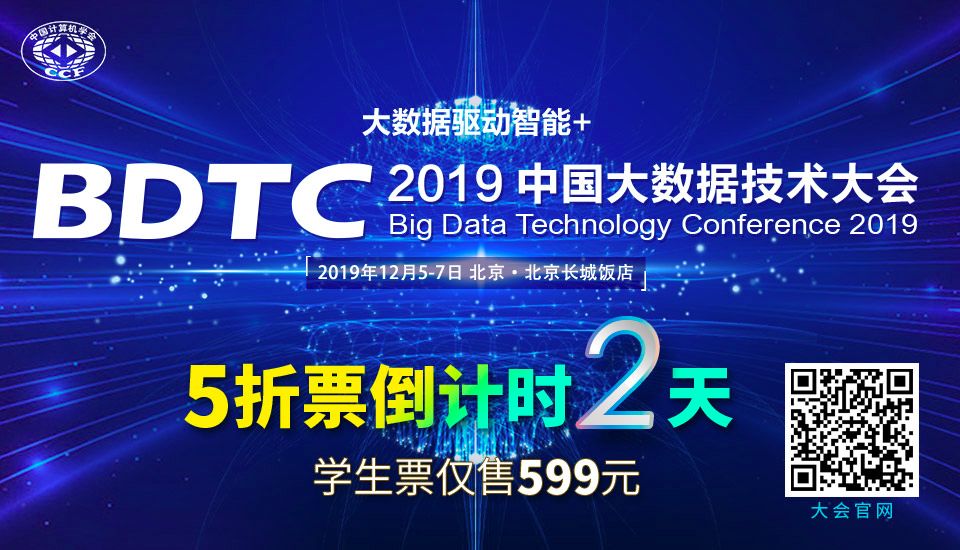
“Python之父”从Dropbox退休
作者 | 若名出品 | AI科技大本营(ID:rgznai100)10 月 30 日,Python 之父 Guido Van Rossum 宣布将从工作六年的 Dropbox 公司退休,他在 Twitter 上转发了 Dropbox 团队写的《Thank you, Guido》公开信长文。Guido 表示,…

谭浩强《C++程序设计》书后习题 第十三章-第十四章
2019独角兽企业重金招聘Python工程师标准>>> 最近要复习一下C和C的基础知识,于是计划把之前学过的谭浩强的《C程序设计》和《C程序设计》习题重新做一遍。 编译环境为:操作系统32位Win7,编译工具VC6.0 第十三章:输入输…
图像处理库(fbc_cv):源自OpenCV代码提取
在实际项目中会经常用到一些基本的图像处理操作,而且经常拿OpenCV进行结果对比,因此这里从OpenCV中提取了一些代码组织成fbc_cv库。项目fbc_cv所有的代码已放到GitHub中,地址为 https://github.com/fengbingchun/OpenCV_Test ,它…

Swift2.x编写NavigationController动态缩放titleView
这两天看到一篇文章iOS 关于navigationBar的一些..中的动态缩放比较有意思,看了一下源码,然后用Swift写了一下,使用storyboard实现. 效果图: 部分代码: 设置滑动代理 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26/**设置滑动代理- parameter scrollV…
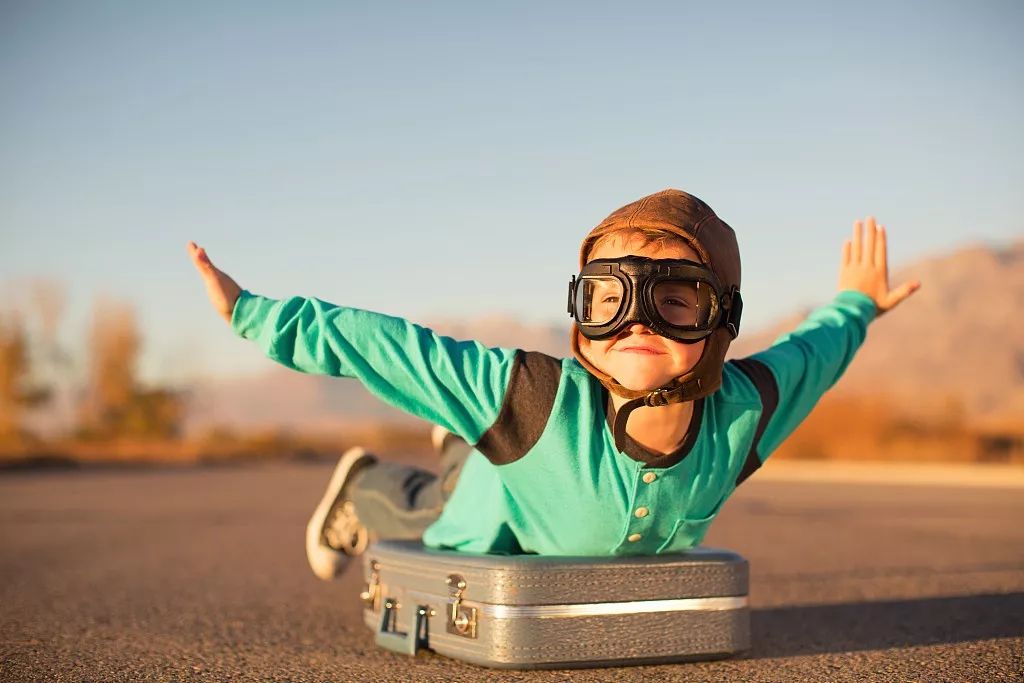
云厂商和开源厂商“鹬蚌相争”,他却看到了开发者的新机会
作者 | 夕颜出品 | AI科技大本营(ID:rgznai100)【导读】过去一年,开发者生态发生了一些或巨大、或微妙的变化,大的变化如巨头云厂商正在通过开源、收购等方式争夺开发者生态,比如微软以 75 亿美金收购 GitHubÿ…
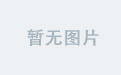
Error: could not open 'D:\Program Files\Java\jre7\lib\amd64\jvm.cfg'
重装JDK后,因为没有装在以前的目录,运行java命令后报错,环境变量的设置都没有问题。解决方法:删除c:/windows/system32/目录下的java.exe 、javaw.exe、javaws.exe,找不到的话在C:\Windows\SysWOW64下找。删除三个文件…