C++中标准模板库std::vector的实现
以下实现了C++标准模板库std::vector的部分实现,参考了 cplusplus.
关于C++中标准模板库std::vector的介绍和用法可以参考 https://blog.csdn.net/fengbingchun/article/details/51510916
实现代码vector.hpp内容如下:
#ifndef FBC_STL_VECTOR_HPP_
#define FBC_STL_VECTOR_HPP_#include <stdlib.h>namespace fbcstd {template<class T>
class vector {
public:typedef size_t size_type;typedef T value_type;typedef T* iterator;typedef const T* const_iterator;vector();vector(size_type n, const value_type& val = value_type());vector(const vector& x);~vector();void reserve(size_type n);size_type capacity() const;size_type size() const;bool empty() const;value_type* data();const value_type* data() const;value_type& at(size_type n);const value_type& at(size_type n) const;value_type& operator [] (size_type n);const value_type& operator [] (size_type n) const;vector& operator = (const vector& x);void clear();value_type& back();const value_type& back() const;value_type& front();const value_type& front() const;void push_back(const value_type& val);void pop_back();void resize(size_type n, value_type val = value_type());iterator begin();const_iterator begin() const;iterator end();const_iterator end() const;private:size_type size_ = 0;size_type capacity_ = 0; // 2^nvalue_type* buffer_ = nullptr;}; // class vectortemplate<class T> inline
vector<T>::vector() : size_(0), capacity_(0), buffer_(nullptr)
{
}template<class T> inline
vector<T>::vector(size_type n, const value_type& val)
{reserve(n);size_ = n;for (size_t i = 0; i < n; ++i)buffer_[i] = val;
}template<class T> inline
vector<T>::vector(const vector& x)
{reserve(x.size_);size_ = x.size_;for (size_t i = 0; i < x.size_; ++i)buffer_[i] = x.buffer_[i];
}template<class T> inline
vector<T>::~vector()
{if (buffer_)delete [] buffer_;
}template<class T> inline
void vector<T>::reserve(size_type n)
{if (capacity_ < n) {delete [] buffer_;capacity_ = 1;if (capacity_ < n) {capacity_ = 2;while (capacity_ < n) { capacity_ *= 2;}}buffer_ = new value_type[capacity_];}
}template<class T> inline
size_t vector<T>::capacity() const
{return capacity_;
}template<class T> inline
size_t vector<T>::size() const
{return size_;
}template<class T> inline
bool vector<T>::empty() const
{return size_ == 0 ? true : false;
}template<class T> inline
T* vector<T>::data()
{return buffer_;
}template<class T> inline
const T* vector<T>::data() const
{return buffer_;
}template<class T> inline
T& vector<T>::at(size_type n)
{if (size_ <= n) abort();return buffer_[n];
}template<class T> inline
const T& vector<T>::at(size_type n) const
{if (size_ <= n) abort();return buffer_[n];
}template<class T> inline
T& vector<T>::operator [] (size_type n)
{if (size_ <= n) abort();return buffer_[n];
}template<class T> inline
const T& vector<T>::operator [] (size_type n) const
{if (size_ <= n) abort();return buffer_[n];
}template<class T> inline
vector<T>& vector<T>::operator = (const vector& x)
{reserve(x.size_);size_ = x.size_;for (size_t i = 0; i < size_; ++i)buffer_[i] = x.buffer_[i];return *this;
}template<class T> inline
void vector<T>::clear()
{size_ = 0;
}template<class T> inline
T& vector<T>::back()
{if (size_ == 0) abort();return buffer_[size_-1];
}template<class T> inline
const T& vector<T>::back() const
{if (size_ == 0) abort();return buffer_[size_-1];
}template<class T> inline
T& vector<T>::front()
{if (size_ == 0) abort();return buffer_[0];
}template<class T> inline
const T& vector<T>::front() const
{if (size_ == 0) abort();return buffer_[0];
}template<class T> inline
void vector<T>::push_back(const value_type& val)
{if (size_ >= capacity_) {T* tmp = new T[size_];for (size_t i = 0; i < size_; ++i)tmp[i] = buffer_[i];reserve(size_+1);for (size_t i = 0; i < size_; ++i)buffer_[i] = tmp[i];delete [] tmp;}buffer_[size_] = val;size_ += 1;
}template<class T> inline
void vector<T>::pop_back()
{if (size_ == 0) abort();--size_;
}template<class T> inline
void vector<T>::resize(size_type n, value_type val)
{if (size_ < n) {if (n > capacity_) {T* tmp = new T[size_];for (size_t i = 0; i < size_; ++i)tmp[i] = buffer_[i];reserve(n);for (size_t i = 0; i < size_; ++i)buffer_[i] = tmp[i];delete [] tmp;}for (size_t i = size_; i < n; ++i)buffer_[i] = val;}size_ = n;
}template<class T> inline
T* vector<T>::begin()
{return buffer_;
}template<class T> inline
const T* vector<T>::begin() const
{return buffer_;
}template<class T> inline
T* vector<T>::end()
{return buffer_ + size_;
}template<class T> inline
const T* vector<T>::end() const
{return buffer_ + size_;
}template<class T> static inline
bool operator == (const vector<T>& lhs, const vector<T>& rhs)
{if (lhs.size() != rhs.size())return false;for (size_t i = 0; i < lhs.size(); ++i) {if (lhs[i] != rhs[i])return false;}return true;
}template<class T> static inline
bool operator != (const vector<T>& lhs, const vector<T>& rhs)
{return !(lhs == rhs);
}template<class T> static inline
bool operator < (const vector<T>& lhs, const vector<T>& rhs)
{bool flag = true;if (lhs.size() == rhs.size()) {if (lhs == rhs) {flag = false;} else {for (size_t i = 0; i < lhs.size(); ++i) {if (lhs[i] > rhs[i]) {flag = false;break;}}}} else if (lhs.size() < rhs.size()) {vector<T> vec(lhs.size());for (size_t i = 0; i < lhs.size(); ++i)vec[i] = rhs[i];if (lhs == vec) {flag = true;} else {for (size_t i = 0; i < lhs.size(); ++i) {if (lhs[i] > rhs[i]) {flag = false;break;}}}} else {vector<T> vec(rhs.size());for (size_t i = 0; i < rhs.size(); ++i)vec[i] = lhs[i];if (vec == rhs) {flag = false;} else {for (size_t i = 0; i < rhs.size(); ++i) {if (lhs[i] > rhs[i]) {flag = false;break;}}}}return flag;
}template<class T> static inline
bool operator <= (const vector<T>& lhs, const vector<T>& rhs)
{return !(rhs < lhs);
}template<class T> static inline
bool operator > (const vector<T>& lhs, const vector<T>& rhs)
{return (rhs < lhs);
}template<class T> static inline
bool operator >= (const vector<T>& lhs, const vector<T>& rhs)
{return !(lhs < rhs);
}} // namespace fbcstd#endif // FBC_STL_VECTOR_HPP_
测试代码test_vector.cpp内容如下:
#include <iostream>
#include "vector.hpp"
#include "string.hpp"#define std fbcstdint main()
{{ // constructor, capacity, size, empty, datastd::vector<int> vec1;fprintf(stdout, "vec1 size: %d, capacity: %d, empty: %d\n", vec1.size(), vec1.capacity(), vec1.empty());std::vector<int> vec2(1);fprintf(stdout, "vec2 size: %d, capacity: %d, empty: %d\n", vec2.size(), vec2.capacity(), vec2.empty());fprintf(stdout, "vec2 data: %d\n", *(vec2.data()));std::vector<std::string> vec3(5, "test vector");fprintf(stdout, "vec3 size: %d, capacity: %d, empty: %d\n", vec3.size(), vec3.capacity(), vec3.empty());const std::string* p1 = vec3.data();fprintf(stdout, "vec3 data: \n");for (size_t i = 0; i < vec3.size(); ++i) {fprintf(stdout, " %s,", (*p1).c_str());p1++;}std::vector<std::string> vec4(vec3);fprintf(stdout, "\nvec4 size: %d, capacity: %d, empty: %d\n", vec4.size(), vec4.capacity(), vec4.empty());std::string* p2 = vec4.data();fprintf(stdout, "vec4 data: \n");for (size_t i = 0; i < vec4.size(); ++i)*p2++ = "TEST VECTOR";const std::string* p3 = vec4.data();for (size_t i = 0; i < vec4.size(); ++i) {fprintf(stdout, " %s,", (*p3).c_str());p3++;}fprintf(stdout, "\n");
}{ // at, [], =, clearstd::vector<std::string> vec1(2);vec1.at(0) = "test";vec1.at(1) = "vector";fprintf(stdout, "vec1: %s, %s\n", vec1.at(0).c_str(), vec1.at(1).c_str());vec1[0] = "TEST";vec1[1] = "VECTOR";fprintf(stdout, "vec1: %s, %s\n", vec1[0].c_str(), vec1[1].c_str());std::vector<std::string> vec2;vec2 = vec1;fprintf(stdout, "vec2 size: %d, %s, %s\n", vec2.size(), vec2[0].c_str(), vec2[1].c_str());vec2.clear();fprintf(stdout, "vec2 size: %d\n", vec2.size());
}{ // back, frontstd::vector<int> vec(2, 1000);fprintf(stdout, "value: %d\n", vec.back());vec.back() = -1000;fprintf(stdout, "value: %d\n", vec.back());fprintf(stdout, "value: %d\n", vec.front());vec.front() = -1000;fprintf(stdout, "value: %d\n", vec.front());
}{ // push_back, pop_backstd::vector<int> vec;for (int i = 0; i < 10; ++i)vec.push_back((i+1)*10);fprintf(stdout, "vec: size: %d, capacity: %d\nvalue: ", vec.size(), vec.capacity());for (int i = 0; i < vec.size(); ++i)fprintf(stdout, " %d,", vec[i]);for (int i = 0; i < 5; ++i)vec.pop_back();fprintf(stdout, "\nvec: size: %d, capacity: %d\nvalue: ", vec.size(), vec.capacity());for (int i = 0; i < vec.size(); ++i)fprintf(stdout, " %d,", vec[i]);fprintf(stdout, "\n");
}{ // resizestd::vector<int> myvector;for (int i = 1; i < 10; ++i)myvector.push_back(i);myvector.resize(5);myvector.resize(8,100);myvector.resize(12);fprintf(stdout, "myvector contains:");for (size_t i = 0; i < myvector.size(); ++i)fprintf(stdout, " %d,", myvector[i]);fprintf(stdout, "\n");
}{ // begin, endstd::vector<int> myvector;for (int i = 1; i <= 5; ++i)myvector.push_back(i);fprintf(stdout, "myvector contains:");for (std::vector<int>::iterator it = myvector.begin() ; it != myvector.end(); ++it)fprintf(stdout, " %d,", *it);fprintf(stdout, "\n");for (std::vector<int>::const_iterator it = myvector.begin() ; it != myvector.end(); ++it)fprintf(stdout, " %d,", *it);fprintf(stdout, "\n");
}{ // compare operatorstd::vector<int> foo (3,100);std::vector<int> bar (2,200);if (foo == bar) fprintf(stdout, "foo and bar are equal\n");if (foo != bar) fprintf(stdout, "foo and bar are not equal\n");if (foo < bar) fprintf(stdout, "foo is less than bar\n");if (foo > bar) fprintf(stdout, "foo is greater than bar\n");if (foo <= bar) fprintf(stdout, "foo is less than or equal to bar\n");if (foo >=bar) fprintf(stdout, "foo is greater than or equal to bar\n");
}return 0;
}
CMakeLists.txt内容如下:
PROJECT(Samples_STL_Implementation)
CMAKE_MINIMUM_REQUIRED(VERSION 3.0)SET(CMAKE_C_FLAGS "${CMAKE_C_FLAGS} -g -Wall -O2 -std=c11")
SET(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -g -Wall -O2 -std=c++11")INCLUDE_DIRECTORIES(${PROJECT_SOURCE_DIR}/src)
MESSAGE(STATUS "project source dir: ${PROJECT_SOURCE_DIR}")FILE(GLOB tests ${PROJECT_SOURCE_DIR}/test/*.cpp)FOREACH (test ${tests})STRING(REGEX MATCH "[^/]+$" test_file ${test})STRING(REPLACE ".cpp" "" test_basename ${test_file})ADD_EXECUTABLE(${test_basename} ${test})TARGET_LINK_LIBRARIES(${test_basename} pthread)
ENDFOREACH()
build.sh脚本内容如下:
#! /bin/bashreal_path=$(realpath $0)
dir_name=`dirname "${real_path}"`
echo "real_path: ${real_path}, dir_name: ${dir_name}"new_dir_name=${dir_name}/build
mkdir -p ${new_dir_name}
cd ${new_dir_name}
cmake ..
makecd -
编译及执行操作步骤:将终端定位到Samples_STL_Implementation目录下,执行:$ ./build.sh , 然后再执行$ ./build/test_vector即可。
GitHub: https://github.com/fengbingchun/Linux_Code_Test
相关文章:
Swift学习 OOP三大特性:继承、多态、封装
先看个例子 从上面的例子可以总结那么一句话:”学生是人”。也就是Student类继承People类。简而言之,学生是人,这句话是说得通的,但是”人是学生”这句话是说不通的,不是学生就不是人了嘛? 从代码中,我们可以看出S…
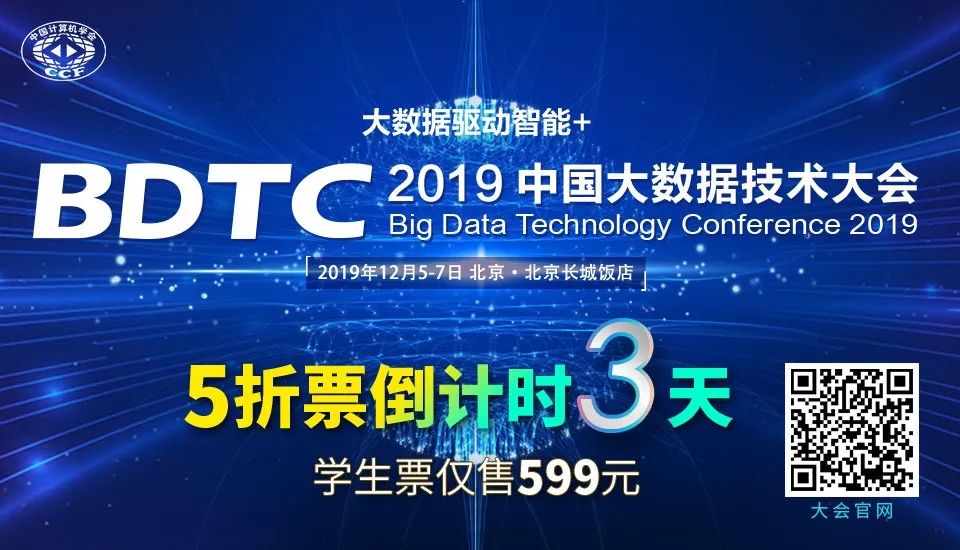
5折票倒计时3天 | 超干货议程首度曝光!2019 中国大数据技术大会邀您共赴
(大会官网https://t.csdnimg.cn/U1wA)2019年,大数据与人工智能的热度已经蔓延到了各个领域,智能交通、AIoT、智慧城市,智慧物流、AI中台、工业制造等各种黑科技成为热搜名词。而在今年的乌镇互联网大会上,大…
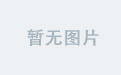
mysql select * f
mysql> select * from tb;-------------| id | name |-------------| 1 | tbone || 3 | 2d2 || 5 | 55 || 6 | 66 |-------------4 rows in set (0.00 sec)转载于:https://www.cnblogs.com/bashala/p/3974088.html
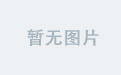
C++/C++11中用于定义类型别名的两种方法:typedef和using
类型别名(type alias)是一个名字,它是某种类型的同义词。使用类型别名有很多好处,它让复杂的类型名字变得简单明了、易于理解和使用,还有助于程序员清楚地知道使用该类型的真实目的。在C中,任何有效类型都可以有别名。 有两种方法…
iOS学习笔记--01swift实现提示框第三方库:MBProgressHUD
本文使用swift语言使用MBProgressHUD。 开源项目MBProgressHUD可以实现多种形式的提示框。使用简单,方便。GitHud的下载地址是:https://github.com/jdg/MBProgressHUD/ 下载完成后,将MBProgressHUD.h和MBProgressHUD.m拖入已经新建好的Swift项…
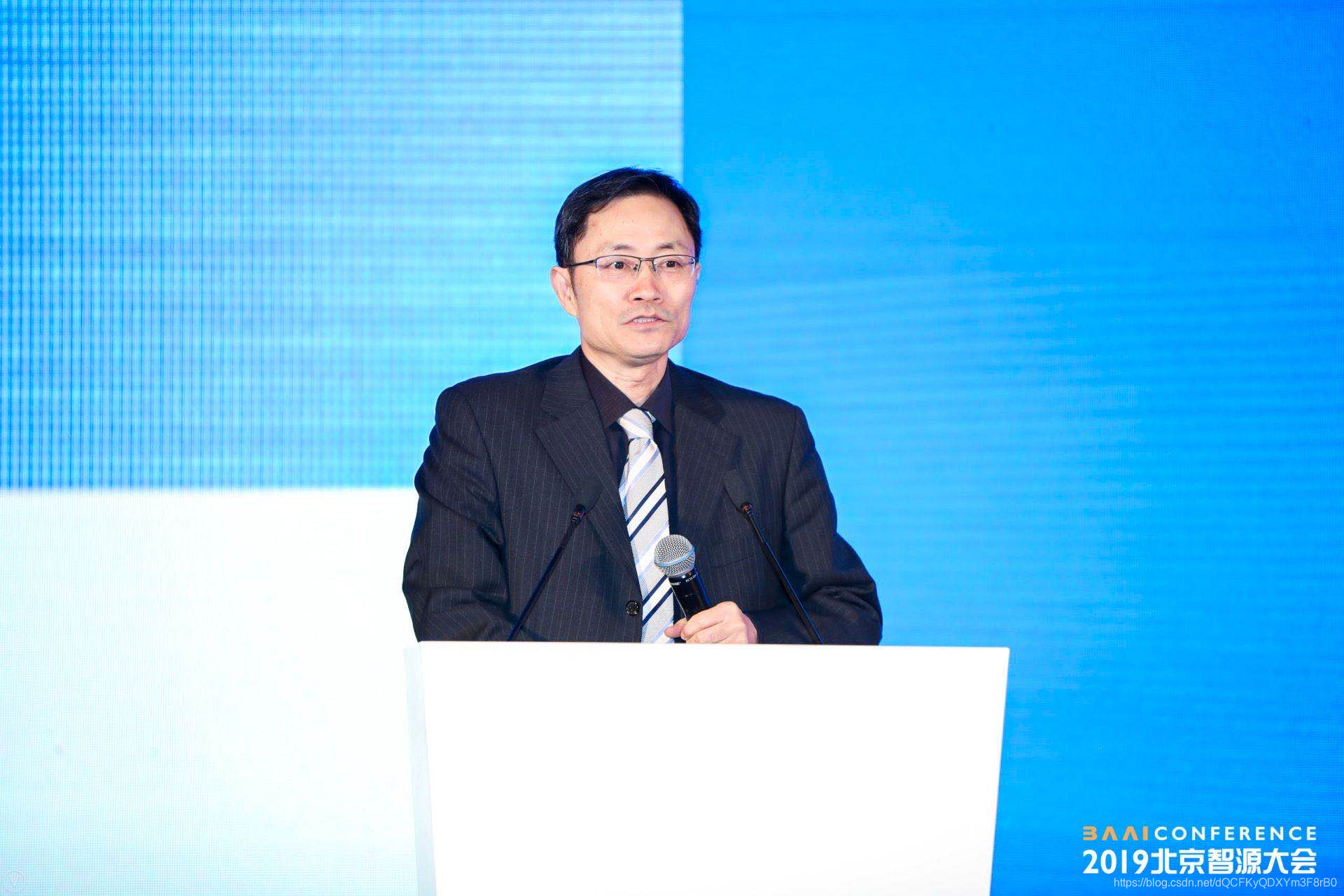
2019北京智源大会在京开幕, 中外学术大咖共话人工智能研究前沿
10月31日,由北京智源人工智能研究院主办的2019北京智源大会在国家会议中心开幕,会期两天。智源大会是北京创建全球人工智能学术和创新最优生态的标志性学术活动,定位于“内行的AI盛会”,以国际性、权威性、专业性和前瞻性为特色&a…
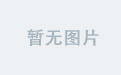
linux中登录类型及配置文件
linux中登录shell的类型1.交互式登录:直接通过终端输入用户信息登录1)login:2)在shell中 su - usernamesu -l username2.非交互式登录1)su username2)图形界面的终端3)执行脚本的过程用户配置文…

Swift项目引入第三方库的方法
分类:iOS(55) 目录(?)[] Swift项目引入第三方库的方法 转自 http://blog.shiqichan.com/How-To-Import-3rd-Lib-Into-Swift-Project/ 以下,将创建一个Swift项目,然后引入3个库: Snappy 简化autolayout代码…
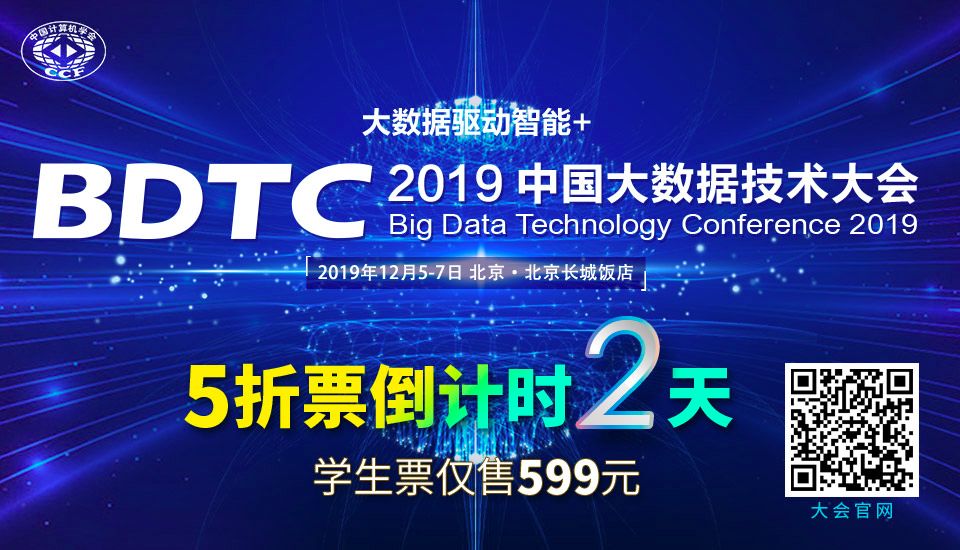
最新NLP架构的直观解释:多任务学习– ERNIE 2.0(附链接)| CSDN博文精选
作者 | Michael Ye翻译 | 陈雨琳,校对 | 吴金笛来源 | 数据派THU(ID:DatapiTHU)百度于今年早些时候发布了其最新的NLP架构ERNIE 2.0,在GLUE基准测试中的所有任务上得分均远高于XLNet和BERT。NLP的这一重大突破利用了一…
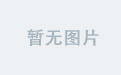
C++中的内存对齐介绍
网上有很多介绍字节对齐或数据对齐或内存对齐的文章,虽然名字不一样,但是介绍的内容大致都是相同的。这里以内存对齐相称。注:以下内容主要来自网络。 内存对齐,通常也称为数据对齐,是计算机对数据类型合法地址做出了…
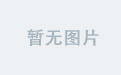
__cplusplus的用处
经常在/usr/include目录下看到这种字句: #ifdef __cplusplus extern "C" { #endif ... #ifdef __cplusplus } #endif 不太明白是怎么用的。今天阅读autobook,在第53页看到了作者的解释:C/C编译器对函数和变量名的命名方法不一样…
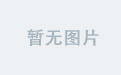
Linux下的内存对齐函数
在Linux下内存对齐的函数包括posix_memalign, aligned_alloc, memalign, valloc, pvalloc,其各个函数的声明如下: int posix_memalign(void **memptr, size_t alignment, size_t size); void *memalign(size_t alignment, size_t size); void *aligned_…
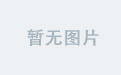
Swift2.0系列]Error Handling(项目应用篇)
1.FileManager中的应用 倘若你只是想看FileManager中的 Error Handling是如何实现的,请找到3.删除文件以及4.获取文件信息。我分别为你提供了do-catch以及try?的使用方法。 打开Xcode,选中Single View Application,输入项目名称例如FileManagerDemo,点击…
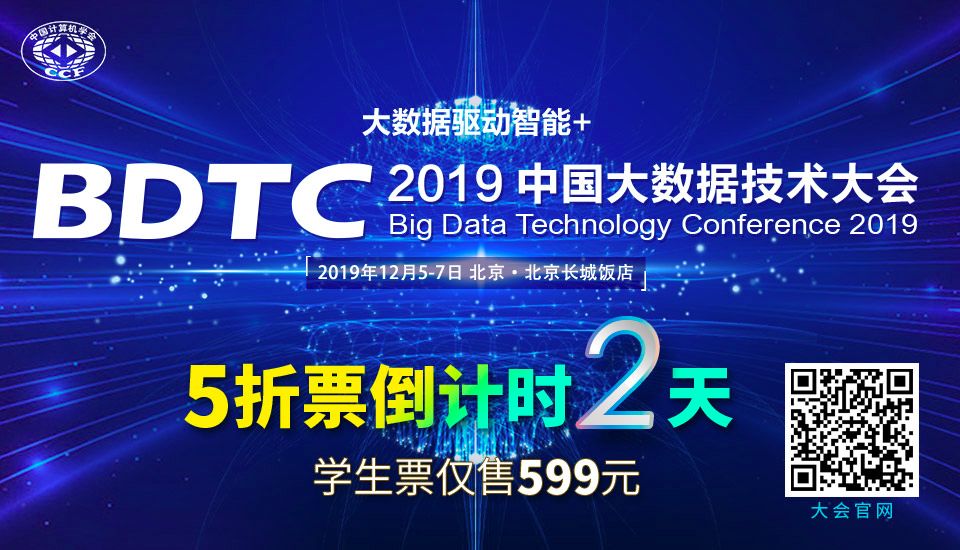
总点第一个视频产生选择偏差?Youtube用“浅塔”来纠正
作者 | Tim Elfrink译者 | Tianyu出品 | AI科技大本营(ID:rgznai100)【导读】本文来自于谷歌研究人员最近发表的一篇论文,介绍了视频平台 Youtube 的视频推荐方法,并在 RecSys 2019 大会上做了分享。本文总结归纳了一些论文中的重…

HTML样式offset[Direction] 和 style.[direction]的区别
为什么80%的码农都做不了架构师?>>> 以offsetLeft与style.left为例: offsetLeft使用的值是字符串,如“100px", style.left则使用数值,如 100 offsetLeft只可以读,因此用无法通过Js改变这个值实现样…
Ubuntu 14.04上安装pip3/numpy/matplotlib/scipy操作步骤
Ubuntu 14.04 64位上默认安装了两个版本的python,一个是python2.7.6,另外一个是python3.4.0,如下图所示: 安装完pip3的结果如下图所示: 升级完pip3的结果如下图所示: 安装完numpy的结果如下图所示: 通过sudo pip3 install matplot…
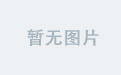
NSHelper.showAlertTitle的两种用法 swift
var model : CarCity CarCity() if (NSString.isNullOrEmpty(locationLabel.text)) { NSHelper.showAlertTitle(nil, message: "暂无法定位,请检查网络。", cancel: "确定") return } if (NSString.isNullOrEmpty(plateTextFild.text)) { NSHe…
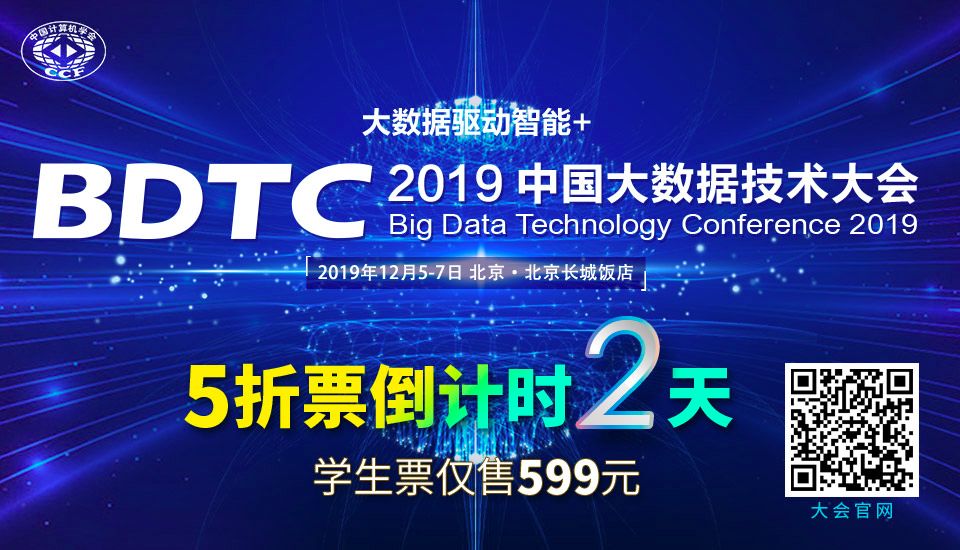
通俗易懂:图卷积神经网络入门详解
作者 | 蝈蝈来源 | 转载自知乎用户蝈蝈【导读】GCN问世已经有几年了(2016年就诞生了),但是这两年尤为火爆。本人愚钝,一直没能搞懂这个GCN为何物,最开始是看清华写的一篇三四十页的综述,读了几页就没读了&a…

Java数据结构一 —— Java Collections API中的表
1.Collection接口 位于java.util包中,以下是重要的部分。 1 public interface Collection<AnyType> extends Iterable<AnyType> 2 { 3 int size(); 4 boolean isEmpty(); 5 void clear(); 6 boolean add(AnyType x); 7 …

Swift 中的内存管理详解
这篇文章是在阅读《The Swift Programming Language》Automatic Reference Counting(ARC,自动引用计数)一章时做的一些笔记,同时参考了其他的一些资料。 在早期的 iOS 开发中,内存管理是由开发者手动来完成的。因为传统…
Ubuntu14.04 64位机上配置OpenCV3.4.2+OpenCV_Contrib3.4.2+Python3.4.3操作步骤
Ubuntu 14.04 64位上默认安装了两个版本的python,一个是python2.7.6,另外一个是python3.4.3。这里使用OpenCV最新的稳定版本3.4.2在Ubuntu上安装python3.4.3支持OpenCV的操作步骤如下: 1. 更新包,执行: sudo apt-get update sud…
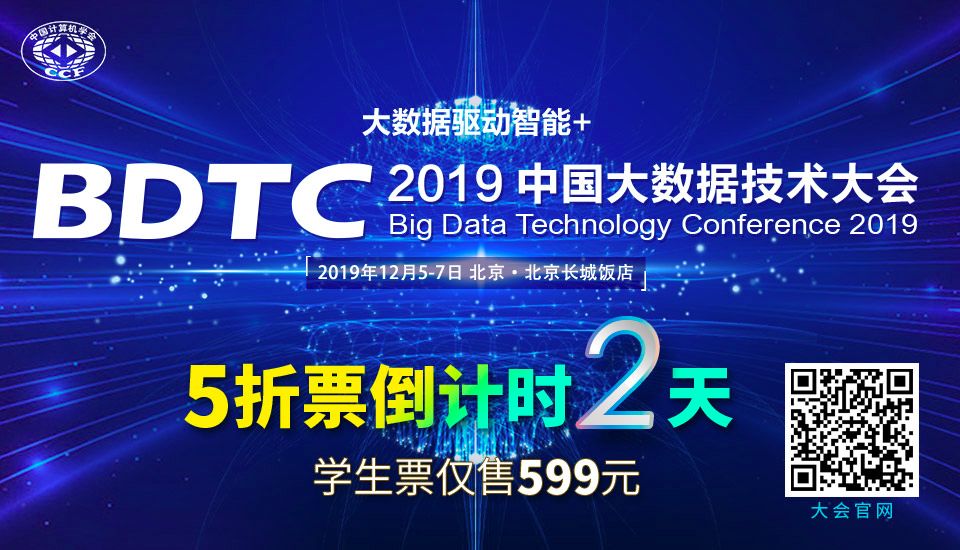
“Python之父”从Dropbox退休
作者 | 若名出品 | AI科技大本营(ID:rgznai100)10 月 30 日,Python 之父 Guido Van Rossum 宣布将从工作六年的 Dropbox 公司退休,他在 Twitter 上转发了 Dropbox 团队写的《Thank you, Guido》公开信长文。Guido 表示,…

谭浩强《C++程序设计》书后习题 第十三章-第十四章
2019独角兽企业重金招聘Python工程师标准>>> 最近要复习一下C和C的基础知识,于是计划把之前学过的谭浩强的《C程序设计》和《C程序设计》习题重新做一遍。 编译环境为:操作系统32位Win7,编译工具VC6.0 第十三章:输入输…
图像处理库(fbc_cv):源自OpenCV代码提取
在实际项目中会经常用到一些基本的图像处理操作,而且经常拿OpenCV进行结果对比,因此这里从OpenCV中提取了一些代码组织成fbc_cv库。项目fbc_cv所有的代码已放到GitHub中,地址为 https://github.com/fengbingchun/OpenCV_Test ,它…

Swift2.x编写NavigationController动态缩放titleView
这两天看到一篇文章iOS 关于navigationBar的一些..中的动态缩放比较有意思,看了一下源码,然后用Swift写了一下,使用storyboard实现. 效果图: 部分代码: 设置滑动代理 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26/**设置滑动代理- parameter scrollV…
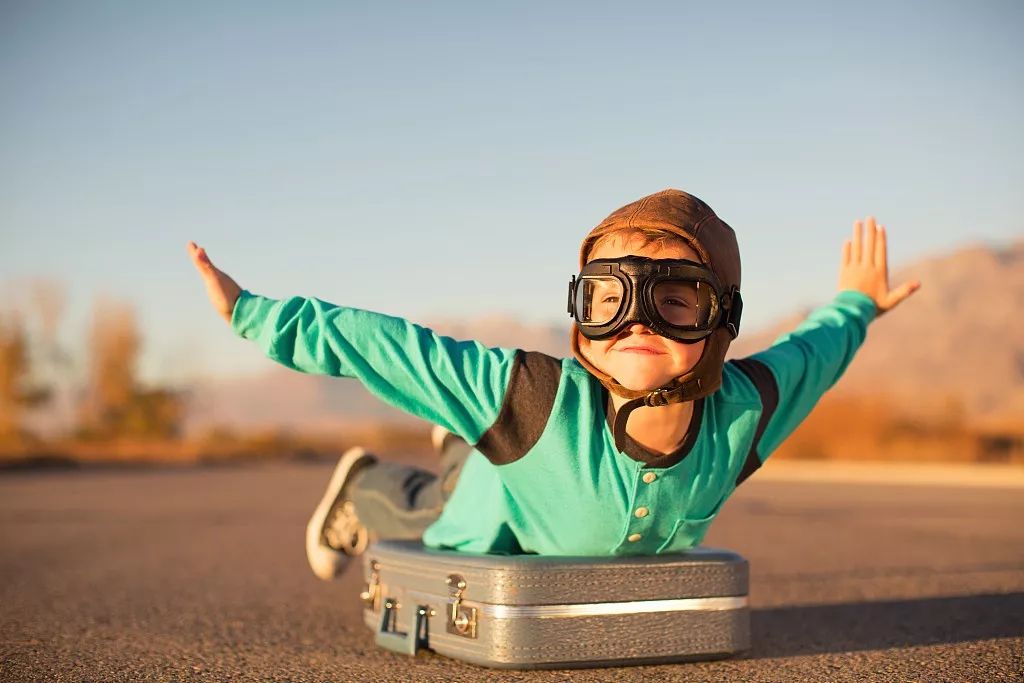
云厂商和开源厂商“鹬蚌相争”,他却看到了开发者的新机会
作者 | 夕颜出品 | AI科技大本营(ID:rgznai100)【导读】过去一年,开发者生态发生了一些或巨大、或微妙的变化,大的变化如巨头云厂商正在通过开源、收购等方式争夺开发者生态,比如微软以 75 亿美金收购 GitHubÿ…
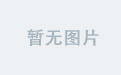
Error: could not open 'D:\Program Files\Java\jre7\lib\amd64\jvm.cfg'
重装JDK后,因为没有装在以前的目录,运行java命令后报错,环境变量的设置都没有问题。解决方法:删除c:/windows/system32/目录下的java.exe 、javaw.exe、javaws.exe,找不到的话在C:\Windows\SysWOW64下找。删除三个文件…
循环神经网络(RNN)简介
人工神经网络介绍参考: https://blog.csdn.net/fengbingchun/article/details/50274471 卷积神经网络介绍参考: https://blog.csdn.net/fengbingchun/article/details/50529500 这里在以上两篇基础上整理介绍循环神经网络: 前馈网络可以…
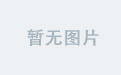
Swift 中 10 个震惊小伙伴的单行代码
几年前,函数式编程的复兴正值巅峰,一篇介绍 Scala 中 10 个单行函数式代码的博文在网上走红。很快地,一系列使用其他语言实现这些单行代码的文章也随之出现,比如 Haskell,Ruby,Groovy,Clojure&a…

满12万送Mate 30 Pro?华为云“双十一”20+款明星产品齐上线
双十一这次是真的真的真真真来了,华为云11.11血拼风暴一促即发!想好怎么玩转双十一了嘛?怎么买到低价高性价比的云主机?怎么抽到100%中奖的礼品?怎么当欧皇被免单?不仅红包、折扣、特惠、满赠、抽奖一样都没…