php使用NuSoap产生webservice结合WSDL让asp.net调用
<?php
require_once("nusoap-0.9.5/lib/nusoap.php");
//定义服务程序
function Add($a,$b)
{
return $a+$b;
}
//初始化服务对象 , 这个对象是类 soap_server 的一个实例
$soap = new soap_server;
//调用服务对象的 register 方法注册需要被客户端访问的程序。
//只有注册过的程序,才能被远程客户端访问到。
$soap->configureWSDL('EventWSDL', 'http://tempuri.org/');
$soap->register('Add', array("a"=>"xsd:string","b"=>"xsd:string"), // 输入参数的定义
array("return"=>"xsd:string") // 返回参数的定义
);
//最后一步,把客户端通过 post 方式提交的数据,传递给服务对象的 service 方法。
//service 方法处理输入的数据,调用相应的函数或方法,并且生成正确的反馈,传回给客户端。
$HTTP_RAW_POST_DATA = isset($HTTP_RAW_POST_DATA) ? $HTTP_RAW_POST_DATA : '';
$soap->service($HTTP_RAW_POST_DATA);
?>
asp.net调用
lt.EventWSDL ew =new webserviceTest.lt.EventWSDL();
Response.Write(ew.Add("1","7").ToString());
=================================
参考:
这篇文章是接着 Introduction to NuSOAP, Programming with NuSOAP 和 Programming with NuSOAP Part 2 这三篇,增加了一些实例来说明如何使用 NuSOAP 结合 WSDL 来创建和使用 SOAP web service。
Hello, World Redux
The New Client
Defining New Data Structures
Hello, World Redux
我在 Introduction to NuSOAP 使用普遍的 “Hello,World” 实例,在那篇文章中,我演示了客户端和服务器端的请求和响应的交互,这里,我将使用 WSDL 来扩展那个实例。WSDL 文件为 service 提供了 metadata,NuSOAP 允许程序员指定使用 soap_server 类的附加字段和方法的 service 创建的 WSDL。
Service 的代码必须依照产生的正确的 WSDL 的顺序做很多事情。service 的信息通过调用 configureWSDL 方法来指定,每个方法的信息也通过提供 register 方法的附加参数来指定,使用 WSDL 的 service 代码在下面的实例中演示:
<?php
// Pull in the NuSOAP code
require_once('nusoap.php');
// Create the server instance
$server = new soap_server();
// Initialize WSDL support
$server->configureWSDL('hellowsdl', 'urn:hellowsdl');
// Register the method to expose
$server->register('hello', // method name
array('name' => 'xsd:string'), // input parameters
array('return' => 'xsd:string'), // output parameters
'urn:hellowsdl', // namespace
'urn:hellowsdl#hello', // soapaction
'rpc', // style
'encoded', // use
'Says hello to the caller' // documentation
);
// Define the method as a PHP function
function hello($name) {
return 'Hello, ' . $name;
}
// Use the request to (try to) invoke the service
$HTTP_RAW_POST_DATA = isset($HTTP_RAW_POST_DATA) ? $HTTP_RAW_POST_DATA : '';
$server->service($HTTP_RAW_POST_DATA);
?>
现在有些魔幻了,在你的浏览器上打开 service 的地址,在我的环境上是 http://localhost/phphack/hellowsdl.php,页面返回的内容提供了可以查看 service 的 WSDL 或者 查看每个方法信息的链接,这个实例是 hello 方法,页面显示的内容类似下图:
<?xml version="1.0"?>
<definitions xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:si="http://soapinterop.org/xsd"
xmlns:tns="urn:hellowsdl"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/"
xmlns="http://schemas.xmlsoap.org/wsdl/"
targetNamespace="urn:hellowsdl">
<types>
<xsd:schema targetNamespace="urn:hellowsdl">
<xsd:import namespace="http://schemas.xmlsoap.org/soap/encoding/" />
<xsd:import namespace="http://schemas.xmlsoap.org/wsdl/" />
</xsd:schema>
</types>
<message name="helloRequest">
<part name="name" type="xsd:string" />
</message>
<message name="helloResponse">
<part name="return" type="xsd:string" />
</message>
<portType name="hellowsdlPortType">
<operation name="hello">
<documentation>Says hello to the caller</documentation>
<input message="tns:helloRequest"/>
<output message="tns:helloResponse"/>
</operation>
</portType>
<binding name="hellowsdlBinding" type="tns:hellowsdlPortType">
<soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="hello">
<soap:operation soapAction="urn:hellowsdl#hello" style="rpc"/>
<input>
<soap:body use="encoded" namespace="urn:hellowsdl"
encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</input>
<output>
<soap:body use="encoded" namespace="urn:hellowsdl"
encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</output>
</operation>
</binding>
<service name="hellowsdl">
<port name="hellowsdlPort" binding="tns:hellowsdlBinding">
<soap:address location="http://localhost/phphack/hellowsdl.php"/>
</port>
</service>
</definitions>
The New Client
在 service 中加入一些 NuSOAP WSDL 调用让它产生 WSDL 和其它的文档。相比之下,支持 WSDL 的客户端是突减的(anti-climactic),是少在这个简单的例子是。下面这个简单的例子和之前没有 WSDL 的客户端代码没有什么不同,唯一的不同是 soapclient 类的构造函数提供了一个 WSDL 的 URL 作为参数,而不是service 的地址。<?php
// Pull in the NuSOAP code
require_once('nusoap.php');
// Create the client instance
$client = new soapclient('http://localhost/phphack/hellowsdl.php?wsdl', true);
// Check for an error
$err = $client->getError();
if ($err) {
// Display the error
echo '<h2>Constructor error</h2><pre>' . $err . '</pre>';
// At this point, you know the call that follows will fail
}
// Call the SOAP method
$result = $client->call('hello', array('name' => 'Scott'));
// Check for a fault
if ($client->fault) {
echo '<h2>Fault</h2><pre>';
print_r($result);
echo '</pre>';
} else {
// Check for errors
$err = $client->getError();
if ($err) {
// Display the error
echo '<h2>Error</h2><pre>' . $err . '</pre>';
} else {
// Display the result
echo '<h2>Result</h2><pre>';
print_r($result);
echo '</pre>';
}
}
// Display the request and response
echo '<h2>Request</h2>';
echo '<pre>' . htmlspecialchars($client->request, ENT_QUOTES) . '</pre>';
echo '<h2>Response</h2>';
echo '<pre>' . htmlspecialchars($client->response, ENT_QUOTES) . '</pre>';
// Display the debug messages
echo '<h2>Debug</h2>';
echo '<pre>' . htmlspecialchars($client->debug_str, ENT_QUOTES) . '</pre>';
?>
这里是 WSDL 实现的请求和响应信息:
POST /phphack/hellowsdl.php HTTP/1.0
Host: localhost
User-Agent: NuSOAP/0.6.8 (1.81)
Content-Type: text/xml; charset=ISO-8859-1
SOAPAction: "urn:hellowsdl#hello"
Content-Length: 550
<?xml version="1.0" encoding="ISO-8859-1"?>
<SOAP-ENV:Envelope SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:si="http://soapinterop.org/xsd"
xmlns:tns="urn:hellowsdl">
<SOAP-ENV:Body>
<tns:hello xmlns:tns="urn:hellowsdl">
<name xsi:type="xsd:string">Scott</name>
</tns:hello>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
HTTP/1.1 200 OK
Server: Microsoft-IIS/5.0
Date: Wed, 03 Nov 2004 21:05:34 GMT
X-Powered-By: ASP.NET
X-Powered-By: PHP/4.3.4
Server: NuSOAP Server v0.6.8
X-SOAP-Server: NuSOAP/0.6.8 (1.81)
Content-Type: text/xml; charset=ISO-8859-1
Content-Length: 551
<?xml version="1.0" encoding="ISO-8859-1"?>
<SOAP-ENV:Envelope SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:si="http://soapinterop.org/xsd">
<SOAP-ENV:Body>
<ns1:helloResponse xmlns:ns1="urn:hellowsdl">
<return xsi:type="xsd:string">Hello, Scott</return>
</helloResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
Defining New Data Structures
WSDL 一个重要的方面是它封装了一个或多个 XML 结构,允许程序员通过 service 来描述数据结构,为了说明 NuSOAP 如何支持这个,我会在 Programming with NuSOAP Part 2 文章中的 SOAP struct 实例中加入 WSDL 代码。service 代码的改变已经显示在 Hello, World 实例中,但是它也包含了定义 Person 数据结构的代码:
<?php // Pull in the NuSOAP code require_once('nusoap.php'); // Create the server instance $server = new soap_server(); // Initialize WSDL support $server->configureWSDL('hellowsdl2', 'urn:hellowsdl2'); // Register the data structures used by the service $server->wsdl->addComplexType( 'Person', 'complexType', 'struct', 'all', '', array( 'firstname' => array('name' => 'firstname', 'type' => 'xsd:string'), 'age' => array('name' => 'age', 'type' => 'xsd:int'), 'gender' => array('name' => 'gender', 'type' => 'xsd:string') ) ); $server->wsdl->addComplexType( 'SweepstakesGreeting', 'complexType', 'struct', 'all', '', array( 'greeting' => array('name' => 'greeting', 'type' => 'xsd:string'), 'winner' => array('name' => 'winner', 'type' => 'xsd:boolean') ) ); // Register the method to expose $server->register('hello', // method name array('person' => 'tns:Person'), // input parameters array('return' => 'tns:SweepstakesGreeting'), // output parameters 'urn:hellowsdl2', // namespace 'urn:hellowsdl2#hello', // soapaction 'rpc', // style 'encoded', // use 'Greet a person entering the sweepstakes' // documentation ); // Define the method as a PHP function function hello($person) { $greeting = 'Hello, ' . $person['firstname'] . '. It is nice to meet a ' . $person['age'] . ' year old ' . $person['gender'] . '.'; $winner = $person['firstname'] == 'Scott'; return array( 'greeting' => $greeting, 'winner' => $winner ); } // Use the request to (try to) invoke the service $HTTP_RAW_POST_DATA = isset($HTTP_RAW_POST_DATA) ? $HTTP_RAW_POST_DATA : ''; $server->service($HTTP_RAW_POST_DATA); ?> 除了支持 WSDL 的附加代码之外,service 方法的代码本身也有一点改变,使用 WSDL ,不再需要使用 soapval 对象来为返回值指定名称和数据类型。相似的, WSDL 客户端不需要使用 soapval 指定参数的名称和数据类型,演示代码如下:
<?php
// Pull in the NuSOAP code
require_once('nusoap.php');
// Create the client instance
$client = new soapclient('http://localhost/phphack/hellowsdl2.php?wsdl', true);
// Check for an error
$err = $client->getError();
if ($err) {
// Display the error
echo '<h2>Constructor error</h2><pre>' . $err . '</pre>';
// At this point, you know the call that follows will fail
}
// Call the SOAP method
$person = array('firstname' => 'Willi', 'age' => 22, 'gender' => 'male');
$result = $client->call('hello', array('person' => $person));
// Check for a fault
if ($client->fault) {
echo '<h2>Fault</h2><pre>';
print_r($result);
echo '</pre>';
} else {
// Check for errors
$err = $client->getError();
if ($err) {
// Display the error
echo '<h2>Error</h2><pre>' . $err . '</pre>';
} else {
// Display the result
echo '<h2>Result</h2><pre>';
print_r($result);
echo '</pre>';
}
}
// Display the request and response
echo '<h2>Request</h2>';
echo '<pre>' . htmlspecialchars($client->request, ENT_QUOTES) . '</pre>';
echo '<h2>Response</h2>';
echo '<pre>' . htmlspecialchars($client->response, ENT_QUOTES) . '</pre>';
// Display the debug messages
echo '<h2>Debug</h2>';
echo '<pre>' . htmlspecialchars($client->debug_str, ENT_QUOTES) . '</pre>';
?>
WSDL 是客户端多于一个功能,使用代理而不是用 soapclinet 类的 call 方法。代理(proxy)是一个类,它映射到 service 。因此,它具备了与 service 相同参数的相同方法,一些程序员更喜欢使用代理因为方法是作为用户一个实例的方法来调用的,而不是通过 call 方法,一个使用代理的实例如下:
<?php
// Pull in the NuSOAP code
require_once('nusoap.php');
// Create the client instance
$client = new soapclient('http://localhost/phphack/hellowsdl2.php?wsdl', true);
// Check for an error
$err = $client->getError();
if ($err) {
// Display the error
echo '<h2>Constructor error</h2><pre>' . $err . '</pre>';
// At this point, you know the call that follows will fail
}
// Create the proxy
$proxy = $client->getProxy();
// Call the SOAP method
$person = array('firstname' => 'Willi', 'age' => 22, 'gender' => 'male');
$result = $proxy->hello($person);
// Check for a fault
if ($proxy->fault) {
echo '<h2>Fault</h2><pre>';
print_r($result);
echo '</pre>';
} else {
// Check for errors
$err = $proxy->getError();
if ($err) {
// Display the error
echo '<h2>Error</h2><pre>' . $err . '</pre>';
} else {
// Display the result
echo '<h2>Result</h2><pre>';
print_r($result);
echo '</pre>';
}
}
// Display the request and response
echo '<h2>Request</h2>';
echo '<pre>' . htmlspecialchars($proxy->request, ENT_QUOTES) . '</pre>';
echo '<h2>Response</h2>';
echo '<pre>' . htmlspecialchars($proxy->response, ENT_QUOTES) . '</pre>';
// Display the debug messages
echo '<h2>Debug</h2>';
echo '<pre>' . htmlspecialchars($proxy->debug_str, ENT_QUOTES) . '</pre>';
?>
尽管可以使用常规的和代理的编码风格,但是请求和响应的信息是相同的。
POST /phphack/hellowsdl2.php HTTP/1.0
Host: localhost
User-Agent: NuSOAP/0.6.8 (1.81)
Content-Type: text/xml; charset=ISO-8859-1
SOAPAction: "urn:hellowsdl2#hello"
Content-Length: 676
<?xml version="1.0" encoding="ISO-8859-1"?>
<SOAP-ENV:Envelope SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:si="http://soapinterop.org/xsd"
xmlns:tns="urn:hellowsdl2">
<SOAP-ENV:Body>
<tns:hello xmlns:tns="urn:hellowsdl2">
<person xsi:type="tns:Person">
<firstname xsi:type="xsd:string">Willi</firstname>
<age xsi:type="xsd:int">22</age>
<gender xsi:type="xsd:string">male</gender>
</person>
</tns:hello>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
HTTP/1.1 200 OK
Server: Microsoft-IIS/5.0
Date: Wed, 03 Nov 2004 21:20:44 GMT
X-Powered-By: ASP.NET
X-Powered-By: PHP/4.3.4
Server: NuSOAP Server v0.6.8
X-SOAP-Server: NuSOAP/0.6.8 (1.81)
Content-Type: text/xml; charset=ISO-8859-1
Content-Length: 720
<?xml version="1.0" encoding="ISO-8859-1"?>
<SOAP-ENV:Envelope SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:si="http://soapinterop.org/xsd"
xmlns:tns="urn:hellowsdl2">
<SOAP-ENV:Body>
<ns1:helloResponse xmlns:ns1="urn:hellowsdl2">
<return xsi:type="tns:SweepstakesGreeting">
<greeting xsi:type="xsd:string">
Hello, Willi. It is nice to meet a 22 year old male.
</greeting>
<winner xsi:type="xsd:boolean">0</winner>
</return>
</helloResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
相关文章:

分享 10 个超实用的 Python 编程技巧
作者 | 欣一来源 | Python爱好者集中营今天小编来给大家分享几个Python的编程技巧,帮助你迅速完成地从小白到Python大神的蜕变。字典翻转首先我们来看字典的翻转,假设我们有下面类似的一个字典对象与数据car_dict { "brand":"Tesla"…
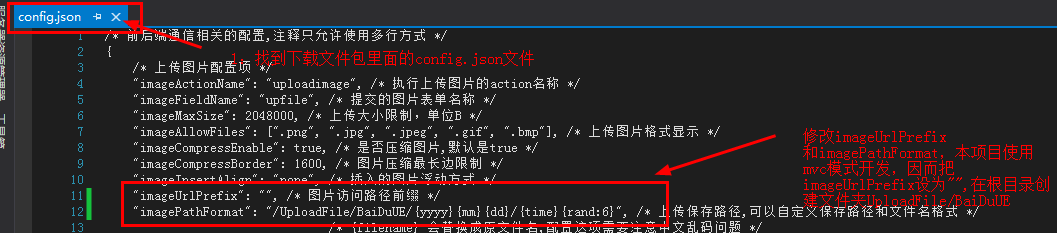
百度编辑器(1.4.3—net版)上传图片路径及其他配置
1:文件配置图: 2:文件夹配置图: 3:多余的功能删除(懒人不想使用百度编辑器官网的自定义,而选择全部功能的直接下载,对于没用的功能可以注释掉,以后有需要就可以再拿出来用…
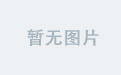
windows下配置redis集群,启动节点报错:createing server TCP listening socket *:7000:listen:Unknown error...
windows下配置redis集群,启动节点报错:createing server TCP listening socket *:7000:listen:Unknown error 学习了:https://blog.csdn.net/u014652744/article/details/71774171 竟然真的是需要bind 127.0.0.1 不同的机器为啥就不一样呢&am…
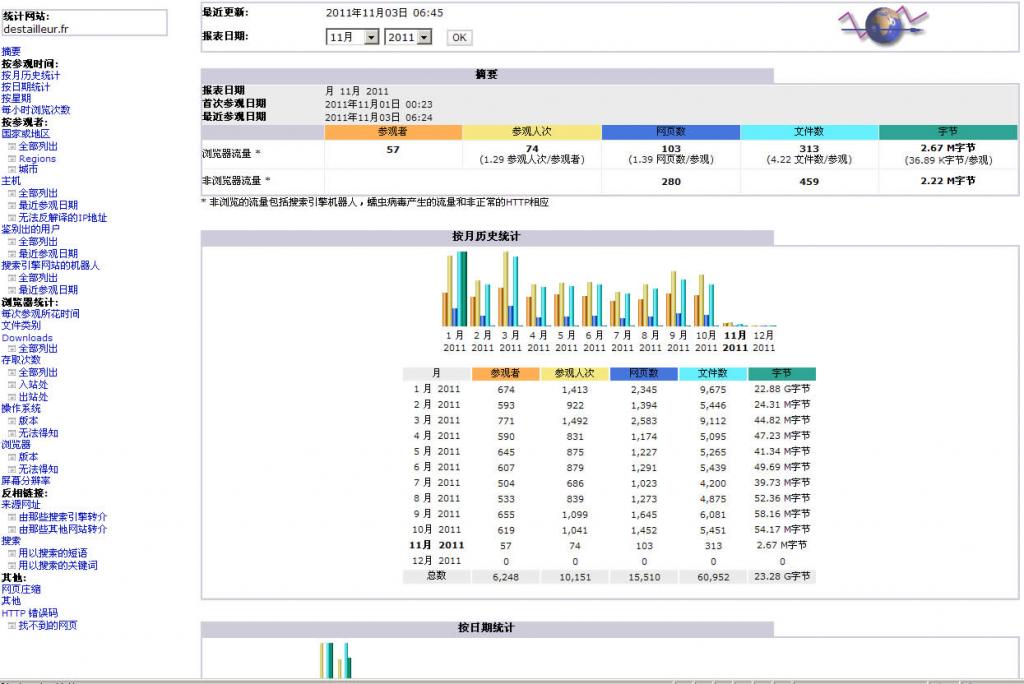
apache日志分析简介
对apache的日志分析做下简单的介绍,主要参考apache官网的Log Files,手册参照 http://httpd.apache.org/docs/2.2/logs.html一.日志分析 如果apache的安装时采用默认的配置,那么在/logs目录下就会生成两个文件,分别是access_log和error_log1.access_log access_log为访问日志,记…
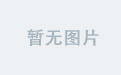
Kotlin语法(基础)
一、基础语法: 1. 定义包名: 包名应该在源文件的最开头,包名不必和文件夹路径一致:源文件可以放在任意位置。 package my.demo 2. 定义函数: fun sum(a: Int , b: Int) : Int{return a b } 表达式函数体自动推断型的返…

未来十年,人机交互将是重要的发展
编译 | 禾木木出品 | AI科技大本营(ID:rgznai100)机器人市场包括广泛且不断扩大的产品范围。经过多年的合作,可以预测机器人技术和机器人行业的未来发展。根据需求,专业服务应用将占据主要市场份额。客户行为的变化已成为行业发展…

20170507Linux七周二次课 io监控free ps 网络状态 抓包
七周二次课(5月7日)10.6 监控io性能10.7 free命令10.8 ps命令10.9 查看网络状态10.10 linux下抓包扩展tcp三次握手四次挥手 http://www.doc88.com/p-9913773324388.htmltshark几个用法:http://www.aminglinux.com/bbs/thread-995-1-1.html监控…
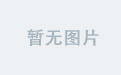
navicat for mysql导出表结构
show create table cm_events;

《新程序员003》正式上市!华为、阿里等 30+ 公司的云原生及数字化实战经验...
作者 | 唐小引出品 | 《新程序员》编辑部《新程序员 003:云原生和全面数字化实践》图书今日正式上市,纸书和电子书同步上架 CSDN 商城、New 程序员小程序、京东、当当等平台。这是由 50 余位技术专家共同创作,写给所有关注云原生和数字化的开…
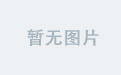
sed及awk显示指定行内容
文件内容为[roottest1 test]# cat file.test 1 2 3 4 5 6 7 8 9 101. 显示第二行内容(指定行)1)sed[roottest1 test]# sed -n 2p file.test 22)awk[roottest1 test]# awk NR2 {print $0} file.test 2 [roottest1 test]# awk {if(NR2)print $0} file.t…
win10 spark+scala+eclipse+sbt 安装配置
转载请务必注明原创地址为:http://dongkelun.com/2018/03/... 1、首先安装配置jdk1.8以上,建议全部的安装路径不要有空格 2、安装spark 2.1 下载 下载地址:http://spark.apache.org/downloads.html,我下载的是 spark-2.2.1-bin-hadoop2.7.tgz…
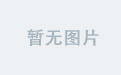
jquery的live方法
live(type, [data], fn)手册API的介绍 jQuery 给所有匹配的元素附加一个事件处理函数,即使这个元素是以后再添加进来的也有效。 如下用 live给classclickme的元素绑定一个click事件: $(.clickme).live(click, function() { alert("Live handler ca…
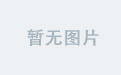
碱基序列的儿子最长上涨
Font Size:Aa Aa AaDescription 给出一个由n个数组成的序列x[1..n],找出它的最长单调上升子序列的长度。即找出最大的长度m和a1, a2……,am,使得 a1 < a2 < … … < am 且 x[a1] < x[a2] < … … < x[am]。Input 先输入一个整数t&…

用 Python 写 3D 游戏,太赞了
作者 | 可可卷CSDN博客 | 可可卷vizard介绍Vizard是一款虚拟现实开发平台软件,从开发至今已走过十个年头。它基于C/C,运用新近OpenGL拓展模块开发出的高性能图形引擎。当运用Python语言执行开发时,Vizard同时自动将编写的程式转换为字节码抽象…
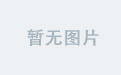
人人都能学会的python编程教程3:字符串和编码
字符串 在python3中已经全面支持中文。 由于Python源代码也是一个文本文件,所以,当你的源代码中包含中文的时候,在保存源代码时,就需要务必指定保存为UTF-8编码。当Python解释器读取源代码时,为了让它按UTF-8编码读取&…
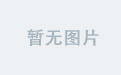
基本MVC原理
参考《Pro PHP》 简单实现了一个mvc框架。 地址http://code.google.com/p/smallframework/自动加载的问题<?php function __autoload($class) { if(file_exists($class.".php")) { require_once($class.".php"); } } class autoload{ public static fu…

31个好用的 Python 字符串方法,建议收藏!
作者 | 小F来源 | 法纳斯特字符串是Python中基本的数据类型,几乎在每个Python程序中都会使用到它。今天,就带大家学习一下31个最重要的内置字符串方法。希望大家能从中找到对自己有帮助的技巧。▍1、Slicingslicing切片,按照一定条件从列表或…
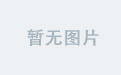
《深入理解计算机系统》读书随笔-位操作
最近开始读《深入理解计算机系统》这本书。对于书中提到的从程序员的角度解读计算机系统这一说法非常感兴趣,所以决定好好读一读。从开始接触计算机编程就是站在一个高级语言的层次,虽然对编译原理,操作系统,汇编语言和计算机组成…

专访小邪:从十年技术之路看阿里技术体系的变革
2019独角兽企业重金招聘Python工程师标准>>> 摘要: 从2008年到2018年,从阿里巴巴中间件团队到飞天八部——小邪与阿里的十年。 编者按:从2008年到2018年,从阿里巴巴中间件团队到飞天八部——小邪与阿里的十年。 2008年…
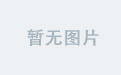
PHP SPL笔记
PHP SPL笔记作者: 阮一峰日期: 2008年7月 8日这几天,我在学习PHP语言中的SPL。这个东西应该属于PHP中的高级内容,看上去很复杂,但是非常有用,所以我做了长篇笔记。不然记不住,以后要用的时候&am…

算力超越 iPhone,芯片堪比Mac,网友:“买来能干啥?”
整理 | 郑丽媛出品 | CSDN(ID:CSDNnews)自去年“元宇宙”概念突然爆火,作为其“入门钥匙”的 AR/VR 设备也顺势成为了话题焦点,尤其在多家外媒爆料苹果也在为此发力、甚至从 Meta 挖人以争取在 2022 年正式推出时&…
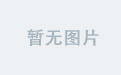
ios开发日记- 5 屏幕截图
-(void)fullScreenshots{UIWindow *screenWindow [[UIApplication sharedApplication] keyWindow]; UIGraphicsBeginImageContext(screenWindow.frame.size);//全屏截图,包括window [screenWindow.layer renderInContext:UIGraphicsGetCurrentContext()]; UIImage …
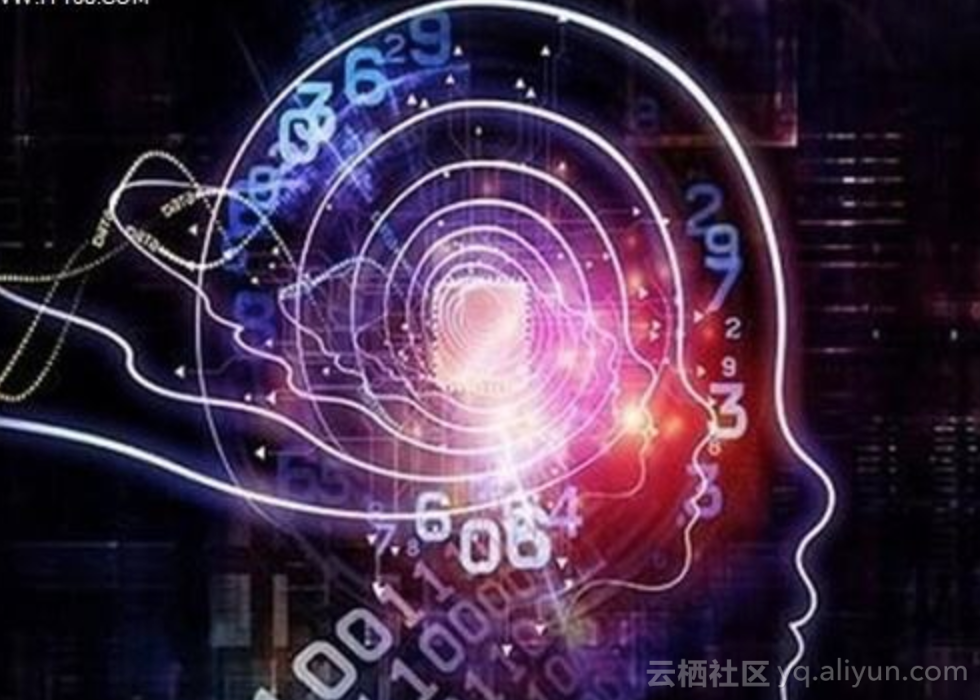
MaxCompute助力OSS支持EB级计算力
一、 MaxCompute是什么? 你的OSS数据是否作堆积在一旁沉睡已久,存储成本变为企业负担?你是否想唤醒沉睡的数据,驱动你的业务前行?MaxCompute可以帮助你高效且低成本的解决这些问题,通过对海量数据进行分析和…
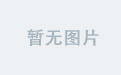
php自动加载
很多开发者写面向对象的应用程序时对每个类的定义建立一个 PHP 源文件。一个很大的烦恼是不得不在每个脚本(每个类一个文件)开头写一个长长的包含文件列表。 在 PHP 5 中,不再需要这样了。可以定义一个 __autoload 函数,它会在试…

22个案例详解 Pandas 数据分析/预处理时的实用技巧,超简单
作者 | 俊欣来源 | 关于数据分析与可视化今天小编打算来讲一讲数据分析方面的内容,整理和总结一下Pandas在数据预处理和数据分析方面的硬核干货,我们大致会说Pandas计算交叉列表Pandas将字符串与数值转化成时间类型Pandas将字符串转化成数值类型Pandas当…

《mysql性能调优与架构设计》笔记: 一mysql 架构组成
2019独角兽企业重金招聘Python工程师标准>>> 2.1mysql物理文件组成 2.1.1日志文件: 1,查看mysql配置文件:mysql --verbose --help | grep -A 1 Default options; 1,错误日志:--log-error[file_name] 指定错…
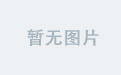
发现一个可以搜索常用rpm包的地址(http://www.rpmfind.net/)
http://www.rpmfind.net/ 虽然资源不多,但也够用。 >如有问题,请联系我:easonjim#163.com,或者下方发表评论。<
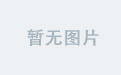
PHP版UTF-8文件BOM自动检测移除程序
BOM信息是文件开头的一串隐藏的字符,用于让某些编辑器识别这是个UTF-8编码的文件。但PHP在读取文件时会把这些字符读出,从而形成了文件 开头含有一些无法识别的字符的问题。比如用UTF-8格式保存的生成图片的PHP文件,因为文件头隐藏的BOM信息也…
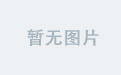
java: web应用中不经意的内存泄露
前面有一篇讲解如何在spring mvc web应用中一启动就执行某些逻辑,今天无意发现如果使用不当,很容易引起内存泄露,测试代码如下: 1、定义一个类App package com.cnblogs.yjmyzz.web.controller;import java.util.Date;public class…

「游戏圈地震级消息」687亿美元,微软收购游戏巨头动视暴雪
整理 | 苏宓、禾木木 出品 | CSDN 2022年1月18日晚,一条热搜刷爆了朋友圈: 继 2018 年,微软以 75 亿美元收购全球知名的代码托管平台 GitHub 后,2022 年 1 月 18 日,微软将以 687 亿美元的价格收购著名游戏制作和发行公…