SpringBoot b2b2c 多用户商城系统(十五)Springboot整合RabbitMQ...
这篇文章带你了解怎么整合RabbitMQ服务器,并且通过它怎么去发送和接收消息。我将构建一个springboot工程,通过RabbitTemplate去通过MessageListenerAdapter去订阅一个POJO类型的消息。
准备工作
15min
IDEA
maven 3.0
在开始构建项目之前,机器需要安装rabbitmq,你可以去官网下载,http://www.rabbitmq.com/download.html ,如果你是用的Mac,你可以这样下载:
brew install rabbitmq
安装完成后开启服务器:
rabbitmq-server
开启服务器成功,你可以看到以下信息:
RabbitMQ 3.1.3. Copyright (C) 2007-2013 VMware, Inc.Licensed under the MPL. See http://www.rabbitmq.com/Logs: /usr/local/var/log/rabbitmq/rabbit@localhost.log/usr/local/var/log/rabbitmq/rabbit@localhost-sasl.logStarting broker... completed with 6 plugins.
构建工程
构架一个SpringBoot工程,其pom文件依赖加上spring-boot-starter-amqp的起步依赖:
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-amqp</artifactId></dependency>
创建消息接收者
在任何的消息队列程序中,你需要创建一个消息接收者,用于响应发送的消息。
@Component
public class Receiver {private CountDownLatch latch = new CountDownLatch(1);public void receiveMessage(String message) {System.out.println("Received <" + message + ">");latch.countDown();}public CountDownLatch getLatch() {return latch;}}
消息接收者是一个简单的POJO类,它定义了一个方法去接收消息,当你注册它去接收消息,你可以给它取任何的名字。其中,它有CountDownLatch这样的一个类,它是用于告诉发送者消息已经收到了,你不需要在应用程序中具体实现它,只需要latch.countDown()就行了。
创建消息监听,并发送一条消息
在spring程序中,RabbitTemplate提供了发送消息和接收消息的所有方法。你只需简单的配置下就行了:
需要一个消息监听容器
声明一个quene,一个exchange,并且绑定它们
一个组件去发送消息
代码清单如下:
package com.forezp;import com.forezp.message.Receiver;
import org.springframework.amqp.core.Binding;
import org.springframework.amqp.core.BindingBuilder;
import org.springframework.amqp.core.Queue;
import org.springframework.amqp.core.TopicExchange;
import org.springframework.amqp.rabbit.connection.ConnectionFactory;
import org.springframework.amqp.rabbit.listener.SimpleMessageListenerContainer;
import org.springframework.amqp.rabbit.listener.adapter.MessageListenerAdapter;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;@SpringBootApplication
public class SpringbootRabbitmqApplication {final static String queueName = "spring-boot";@BeanQueue queue() {return new Queue(queueName, false);}@BeanTopicExchange exchange() {return new TopicExchange("spring-boot-exchange");}@BeanBinding binding(Queue queue, TopicExchange exchange) {return BindingBuilder.bind(queue).to(exchange).with(queueName);}@BeanSimpleMessageListenerContainer container(ConnectionFactory connectionFactory,MessageListenerAdapter listenerAdapter) {SimpleMessageListenerContainer container = new SimpleMessageListenerContainer();container.setConnectionFactory(connectionFactory);container.setQueueNames(queueName);container.setMessageListener(listenerAdapter);return container;}@BeanMessageListenerAdapter listenerAdapter(Receiver receiver) {return new MessageListenerAdapter(receiver, "receiveMessage");}public static void main(String[] args) {SpringApplication.run(SpringbootRabbitmqApplication.class, args);}
}
创建一个测试方法:
@Component
public class Runner implements CommandLineRunner {private final RabbitTemplate rabbitTemplate;private final Receiver receiver;private final ConfigurableApplicationContext context;public Runner(Receiver receiver, RabbitTemplate rabbitTemplate,ConfigurableApplicationContext context) {this.receiver = receiver;this.rabbitTemplate = rabbitTemplate;this.context = context;}@Overridepublic void run(String... args) throws Exception {System.out.println("Sending message...");rabbitTemplate.convertAndSend(Application.queueName, "Hello from RabbitMQ!");receiver.getLatch().await(10000, TimeUnit.MILLISECONDS);context.close();}}
相关文章:
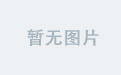
SQLite 日期类型(转)
SQLite日期类型 简单示例: SELECT datetime(CHANGE_DATE,localtime), strftime(%Y-%m-%d,CHANGE_DATE,localtime), datetime(now,localtime), strftime(%Y-%m-%d,now,localtime), DATE(now,localtime), time(now,Localtime), time(2010-11-27 01:…
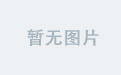
Visual Studio Code常用插件
名称 功能 Chinese (Simplified) Language Pack for Visual Studio Code 汉化 VSCode Auto Close Tag 自动为写的html标签进行闭 合 Beautify 代码格式化 Element UI Snippets Element UI的代码提示功能 Material Icon Theme 为项目换上相应的图标 Vetur对vue代码进行高亮 View…
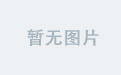
sqlserver导入excel的电话号码(身份证)变为科学计数解决方式
如果excel中有一列存的是手机号码或者身份证号码,那么导入到sql中时,会把手机或者身份证当作数字格式对待,因而会以科学记数法的形式存在sqlserver表中,解决方式,先将excel文件另存为文本文件(制表符&#…

Git的其他用法
2019独角兽企业重金招聘Python工程师标准>>> 目录: 减少【.git】文件夹的大小和文件数更换git for windows的文本编辑器修改已经提交的commit说明合并commit解决merge时出现的冲突回退一个merge获取某一commit的修改将低版本push到Github(删掉…

UE中的几个极有用功能
1. 指定目录和文件类型批量查找目标字符串 示例:在H:\qtdemo目录(含子目录)中下的*.h和*.cpp中,查找“main”字符串 查找结果: 2. 在当前活动窗口中查找目标字符串(Ctrl F) (1&…
Missing space before value for key ‘routes‘ key-spacing
vue项目报错: Missing space before value for key ‘routes’ key-spacing 解决办法: 字母new前面应该是1个空格,我写了两个空格,删去一个空格即可
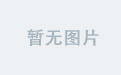
Oracle Mutex 机制 说明
之前也整理过一篇文章来说明Oracle Lock的,参考: 锁 死锁 阻塞 Latch 等待 详解 http://blog.csdn.net/tianlesoftware/archive/2010/08/19/5822674.aspx 在这篇文章里,提到了System Locks,它包含: (1&…
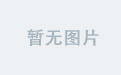
POJ-1860-Currency Exchange
链接:https://vjudge.net/problem/POJ-1860 题意: 有N个点,支持货币兑换,从货币a->b手续费c,汇率r。 求能否换一圈使总净额增加。 思路: bellman-ford。 找一个正权回路。 代码: #include &l…
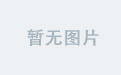
如果asp.net mvc中某个action被执行了两次,请检查是不是以下的原因
注释 <link rel"icon" href"#"> 这一句后试试转载于:https://www.cnblogs.com/lummon/p/4559185.html
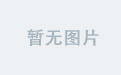
vue常见错误汇总(自看)
解决办法汇总 eslint: Expected indentation of 2 spaces but found 4缩进报错 ,所有缩进只能用两个空格 Newline required at end of file but not found需要在最后的后面再加一行!!! Missing space before value for key ‘name’在关键字“值”之前缺少空格 …
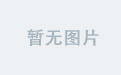
IOS的钥匙串,确保本地隐私数据的安全
* 苹果的"生态圈",钥匙串访问,使用 AES 256 加密算法,能够保证用户密码的安全 * 钥匙串访问SDK,是苹果在 iOS 7.0.3 版本以后公布的 * 钥匙串访问的接口是纯 C 语言的,但是,网络上有框架把它封装…
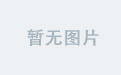
【转】PendingIntent的总结
Intent和PendingIntent的关系,初学的时候很迷惑,用PendingIntent的时候,还会出现奇怪的问题,比如无法传递数据,无法更新数据,所以我集众家之长,加上我个人的一些实践,总结如下&#…
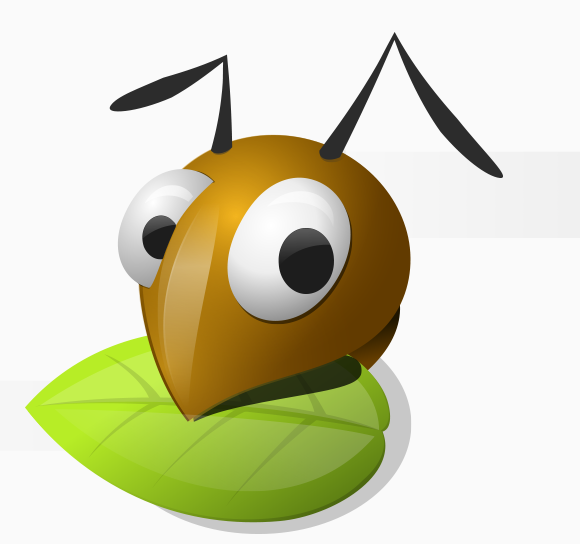
CentOS 7 安装 GlusterFS
目录 环境说明: 3台机器安装 GlusterFS 组成一个集群。 使用 docker volume plugin GlusterFS 服务器: 10.6.0.140 10.6.0.192 10.6.0.196转载于:https://www.cnblogs.com/MeiCheng/p/10274222.html
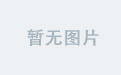
npm安装less报错 rollbackFailedOptional: verb npm-session
解决办法 在cmd中依次输入然后回车 (1) npm install -g cnpm --registryhttps://registry.npm.taobao.org (2) cnpm install less less-loader --save-dev
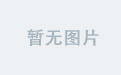
Online Judge上陪审团选人问题用Java实现的一个AC解
原问题位于:http://poj.org/problem?id1015 以下为问题描述的摘录: In Frobnia, a far-away country, the verdicts in court trials are determined by a jury consisting of members of the general public. Every time a trial is set to begin, a j…
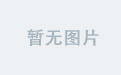
【D3】transition API
摘要: 动画类API 一、API 使用 1. 1 d3.ease 1.2 d3.timer Start a custom animation timer, invoking the specified function repeatedly until it returns true. There is no way to cancel the timer after it starts, so make sure your timer function return…
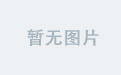
微信小程序 - 富文本图片宽度自适应(正则)
引言:在微信小程序里,比如商品展示页面的商品详情会有图片展示,PC端设置的商品详情是PC端的宽度,所以在小程序里图片会显示不全,这时就应该做相应的处理,使小程序里图片显示正确 思路 把图片的宽度改为手机…
(1)01背包问题
#include <stdio.h> #define N 6 #define M 21 #define W 20 int dp[N][M]; int w[N] {0}; int v[N] {0}; void knapsack(){int i, j;int value1, value2;for(i 1; i < N; i){ // 前i件物品for(j 1; j < N; j){ // 背包剩余空间if(w[i] > j){ // 第i件物品太…
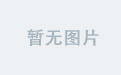
Web.Config文件配置之限制上传文件大小和时间
在邮件发送系统或者其他一些传送文件的网站中,用户传送文件的大小是有限制的,因为这样不但可以节省服务器的空间,还可以提高传送文件的速度。下面介绍如何在Web.Config文件中配置限制上传文件大小与时间。 在Web.Config文件中配置限制上传文件…
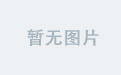
成为软件高手的几个忌讳
1) 不会英语:CS源于美国,重量级的文档都是英文的。不会英语,那么你只能忍受拙劣的翻译和大延迟的文档(翻译出来的文档几乎都是很久以前出版的东西)。2) 急于求成:什么都没学习就开始…
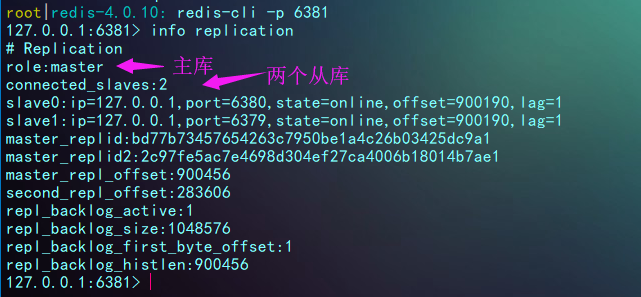
Linux下的redis的持久化,主从同步及哨兵
redis持久化 Redis是一种内存型数据库,一旦服务器进程退出,数据库的数据就会丢失, 为了解决这个问题,Redis提供了两种持久化的方案,将内存中的数据保存到磁盘中,避免数据的丢失。 RDB持久化 redis提供了RDB…
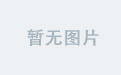
vue/require-v-for-key]Elements in iteration expect to have ‘v-bind:key‘ directives
报错内容:[vue/require-v-for-key]Elements in iteration expect to have v-bind:key directives.解决:加上v-bind:key"index"<p>主演:<!--显示电影主演,循环--><span v-for"(actor, index) in scope.row.act…
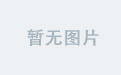
@Ignore_JUnit - Ignore Test
Ignore 用法很简单, 如果你的测试用例还没有准备好而不想被执行, 又不想删掉或注释掉, 可以使用 Ignore 标注来忽略测试。 方法一旦用 Ignore 注解了将不会被执行. 如果一个类用 Ignore 注解了 他下面的所有测试方法将不会被执行. 看个应用 Create a Class Create a java clas…

Redis5.0之Stream案例应用解读
2019独角兽企业重金招聘Python工程师标准>>> 非常高兴有机会和大家在这里交流Redis5.0之Stream应用。今天的分享更多的是一个抛砖引玉,欢迎大家提出更多关于Redis的思考。 首先,我们来个假设,这里有个杯子,这个杯子是去…
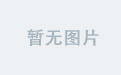
往阿里云服务器上安装Mysql
在安装完Mysql后要进行登录,这时候显示让你输密码, 我点击键盘发现怎么没有密码出现, 然后我就把Mysql卸载了重新装的,折腾了2个多小时, 最后,我突然想试试直接输密码然后就回车, 发现成功进入数…
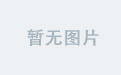
PHP中spl_autoload_register函数的用法
spl_autoload_register(PHP 5 > 5.1.2)spl_autoload_register — 注册__autoload()函数说明bool spl_autoload_register ([ callback $autoload_function ] )将函数注册到SPL __autoload函数栈中。如果该栈中的函数尚未激活,则激活它们。如果在你的程序中已经实现…
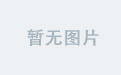
Linux初步——常用简单命令
散乱的记录,目前是边学边用,以后有机会再整理 curl命令 发起一个HTTP请求,如:curl "http://www.baidu.com" 加上-I选项查看HTTP协议头的信息,如:curl "http://www.baidu.com" -I Linux…
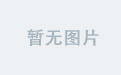
Centos-Mysql配置my.cnf内容
#v1.0 [mysqld] #通用 #skip-grant-tables 跳过授权密码登录 port3306 #使用mysql系统账号操作进程 usermysql socket/var/lib/mysql/mysql.sock #basedir/usr datadir/var/lib/mysql #mysql错误日志 log_error /tmp/ch_mysql_log/error.log #mysql所有操作日志 生产服务器不…
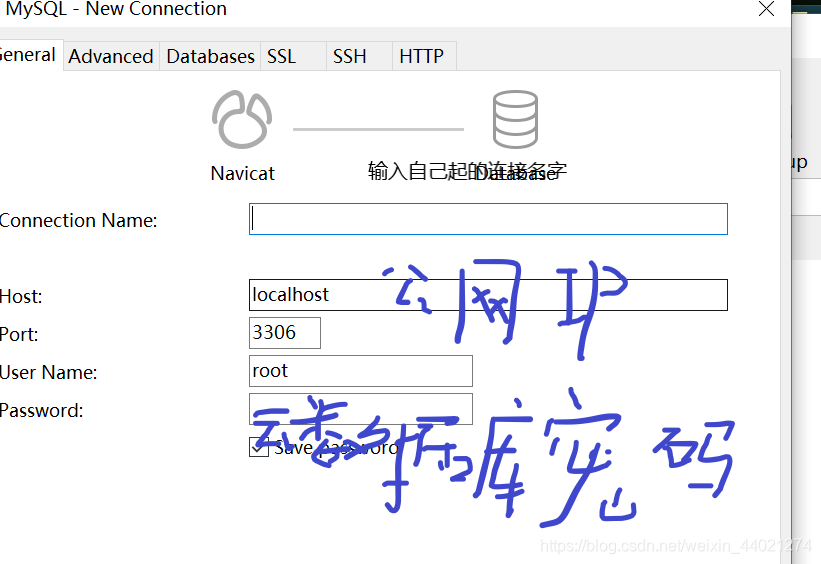
本地navicat连接阿里云数据库
自己起连接名字; prot:填公网IP(服务器给的) password:填阿里云数据库的密码
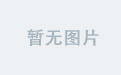
adb logcat命令查看并过滤android输出log
adb logcat命令查看并过滤android输出log cmd命令行中使用adb logcat命令查看android系统和应用的log,dos窗口按ctrlc中断输出log记录。 logcat日志中的优先级/tag标记: android输出的每一条日志都有一个标记和优先级与其关联。 优先级是下面的字符&…